Todo List CRUD Express
Leon Noel

#100Devs
I don't give a fuck about jeans and crep
Or goin' to Milan or goin' to the Met
I just wanna make these songs for the set
Agenda
-
Questions?
-
Let's Talk - #100Devs
-
Review - CRUD
-
Review - Express
-
Learn - Todo List
-
Together - Let's Build
-
Homework - Build your own APP
Questions
About last class or life

Checking In
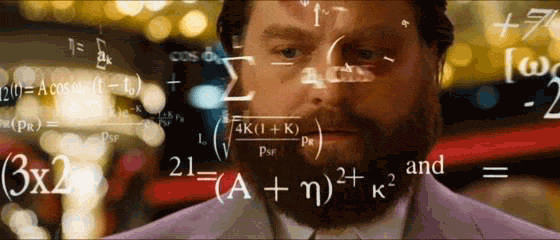
Like and Retweet the Tweet
!checkin
Health First
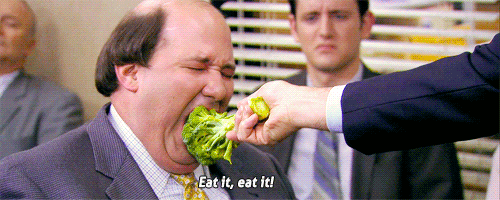
What's Next
-
Aug - Fullstack Web Apps
-
Sept - React + DS/Algorithms
THE HUNT BEGINS
-
Oct - PROJECTS, PREP, MEGA
-
Nov - Agency Life
YOU ALREADY KNOW ENOUGH










Thank You
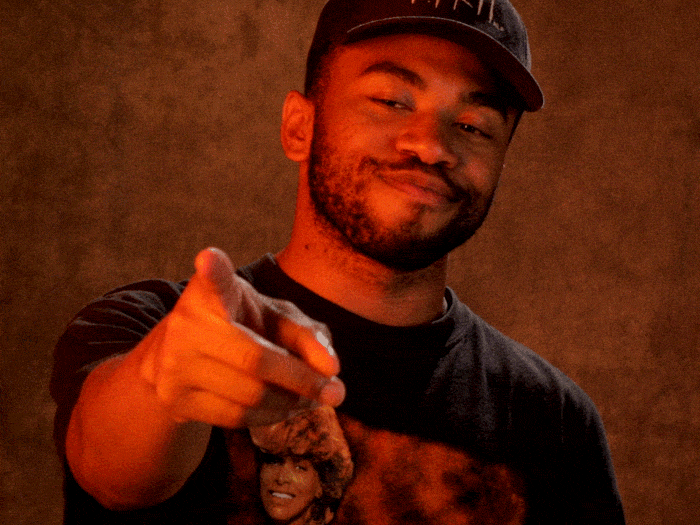
Still Just LEGO
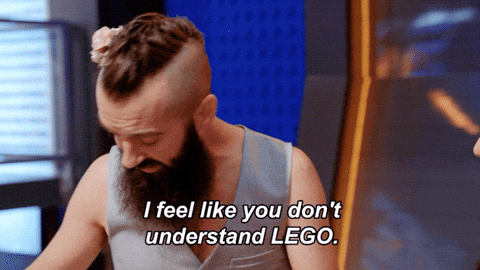
But First
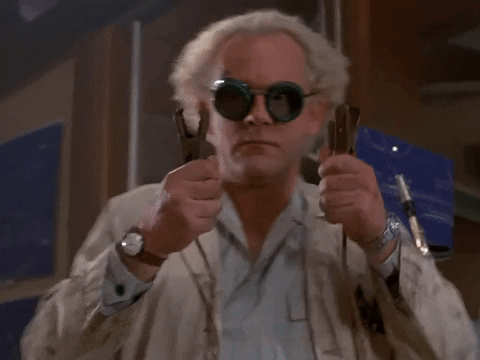
Blast To The Past
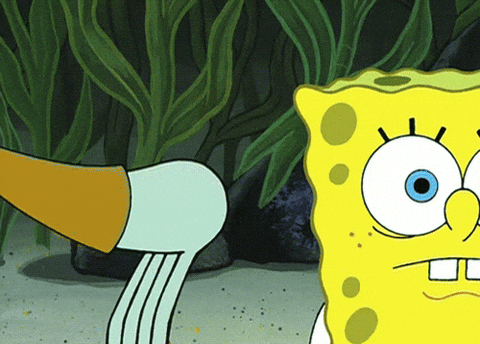
How Does The Internet Work
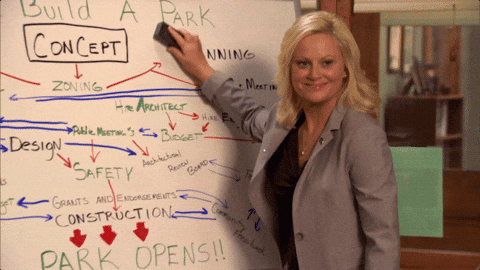
CRUD
Create (post) - Make something
Read (get) - Get Something
Update (put) - Change something
Delete (delete) - Remove something
Mongo DB
Collection
document
document
document
document
EJS
(Embedded Javascript Templates)
<h1>Current Rappers</h1>
<ul class="rappers">
<% for(let i=0; i < info.length; i++) {%>
<li class="rapper">
<span><%= info[i].stageName %></span>
<span><%= info[i].birthName %></span>
<span class='del'>delete</span>
</li>
<% } %>
</ul>
<h2>Add A Rapper:</h2>
Client (Laptop)
Browser
URL
Server
Disk
API Code
Files
Mongo
Database
Collection
Collection
document
document
document
document
app.get('/')
app.post('/form')
app.put('/info')
app.delete('/info')
/views
/public
index.ejs
main.js
style.css
Fonts
Images
Todo List
What are some Create (post) requests?
Todo List
What are some Read (get) requests?
Todo List
What are some Update (put) requests?
Todo List
What are some Delete (delete) requests?
Client (Laptop)
Browser
URL
Server
Disk
API Code
Files
Mongo
Database
Collection
Collection
document
document
document
document
app.get('/')
app.post('/form')
app.put('/info')
app.delete('/info')
/views
/public
index.ejs
main.js
style.css
Fonts
Images
Let's Build An App with Express
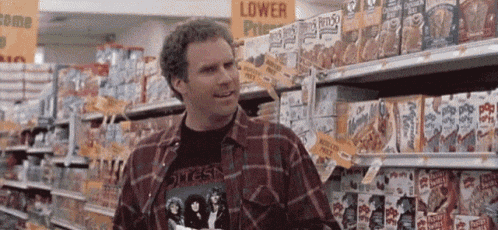
Key Steps
mkdir api-project
cd api-project
npm init
npm install express --save
npm install mongodb --save
npm install ejs --save
npm install dotenv --save
Setting Up The Project
let db,
dbConnectionStr = 'mongodb+srv://demo:demo@cluster0
.2wapm.mongodb.net/rap?retryWrites=true&w=majority',
dbName = 'rap'
MongoClient.connect(dbConnectionStr, { useUnifiedTopology: true })
.then(client => {
console.log(`Connected to ${dbName} Database`)
db = client.db(dbName)
})
Connect To DB
DB_STRING =
mongodb+srv://demo:demo@cluster0.2wapm.mongodb.net/rap?retryWrites=true&w=majority
Use .env
.env file
add .env to .gitignore
.env
node_modules
In terminal, add var to Heroku
heroku config:set DB_STRING =
mongodb+srv://demo:demo@cluster0.2wapm.mongodb.net/rap?retryWrites=true&w=majority
let db,
dbConnectionStr = process.env.DB_STRING,
dbName = 'rap'
MongoClient.connect(dbConnectionStr, { useUnifiedTopology: true })
.then(client => {
console.log(`Connected to ${dbName} Database`)
db = client.db(dbName)
})
Connect To DB
app.set('view engine', 'ejs')
app.use(express.static('public'))
app.use(express.urlencoded({ extended: true }))
app.use(express.json())
app.listen(process.env.PORT || PORT, ()=>{
console.log(`Server running on port ${PORT}`)
})
Setup Server
Create Public Folder/Files
Create EJS File
<h1>Current Rappers</h1>
<ul class="rappers">
<% for(let i=0; i < info.length; i++) {%>
<li class="rapper">
<span><%= info[i].stageName %></span>
<span><%= info[i].birthName %></span>
<span class='del'>delete</span>
</li>
<% } %>
</ul>
<h2>Add A Rapper:</h2>
app.get('/',(request, response)=>{
db.collection('rappers').find().toArray()
.then(data => {
response.render('index.ejs', { info: data })
})
.catch(error => console.error(error))
})
API - GET
app.post('/addRapper', (request, response) => {
db.collection('rappers').insertOne(request.body)
.then(result => {
console.log('Rapper Added')
response.redirect('/')
})
.catch(error => console.error(error))
})
API - POST
app.put('/addOneLike', (request, response) => {
db.collection('rappers').updateOne({stageName: request.body.stageNameS,
birthName: request.body.birthNameS,
likes: request.body.likesS},{
$set: {
likes:request.body.likesS + 1
}
},{
sort: {_id: -1},
upsert: true
})
.then(result => {
console.log('Added One Like')
response.json('Like Added')
})
.catch(error => console.error(error))
})
API - PUT
app.delete('/deleteRapper', (request, response) => {
db.collection('rappers').deleteOne({stageName: request.body.stageNameS})
.then(result => {
console.log('Rapper Deleted')
response.json('Rapper Deleted')
})
.catch(error => console.error(error))
})
API - DELETE
heroku login -i
heroku create simple-rap-api
echo "web: node server.js" > Procfile
git add .
git commit -m "changes"
git push heroku main
Push To Heroku
Let's Code
Todo List App

Homework
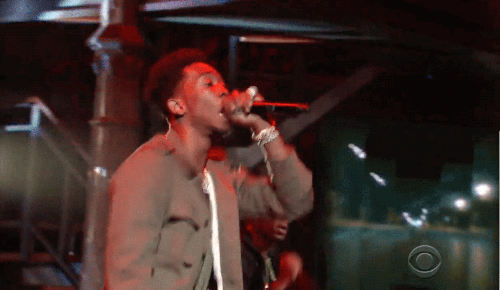
#100Devs - TODO LIST (cohort 2) take 2
By Leon Noel
#100Devs - TODO LIST (cohort 2) take 2
Class 44 of our Free Web Dev Bootcamp for folx affected by the pandemic. Join live T/Th 6:30pm ET leonnoel.com/twitch and ask questions here: leonnoel.com/discord
- 3,547