Backend Crash Course
Leon Noel

#100Devs
Pooh Shiesty, that's my dawg,
but Pooh, you know I'm really shiesty
Agenda
-
Questions?
-
Review - JS Fundamentals
-
Review - Promises + Async / Await
-
Review - Node Fundamentals
-
Review - CRUD w/ Express
-
Review - Local Auth
-
Homework - Review Binary Upload Boom
Questions
About last class or life

Active Recall

Ali Abdaal: https://youtu.be/ukLnPbIffxE
Forgetting Curve*

https://intelalearning.wordpress.com
Resetting Forgetting Curve

https://www.pearsoned.com/three-simple-research-based-ways-to-ace-a-test
Spaced Repetition

Ali Abdaal: https://youtu.be/Z-zNHHpXoMM
Where are you?
What do you want?


Happiest Career:

Ticketmaster Office
Baby Learns To Walk?
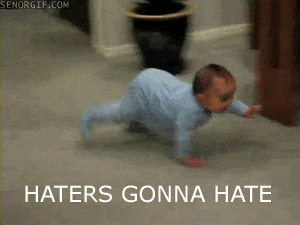
Half Hard Work and Half Believing You Can Do It
*thanks Vonds
Imposter Syndrome
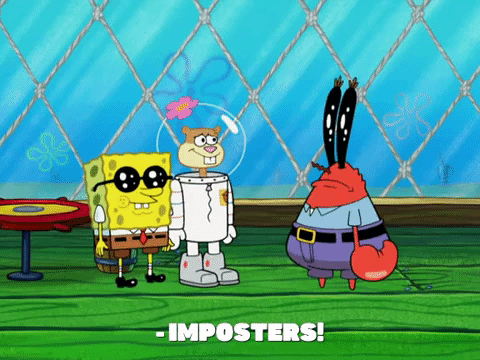
Trough Of Sorrow

Manage Frustration
Consistency
Taking Care Of Yourself
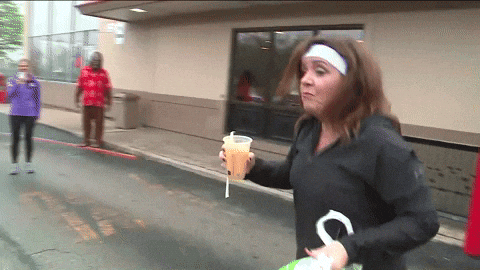
Don't let your dreams be dreams

YOU OWE YOU

Text
What is the internet?

Programming
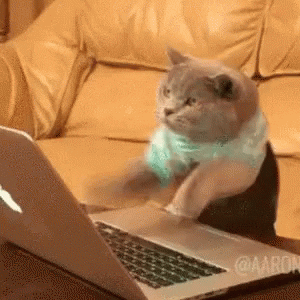
A computer will do what you tell it to do.
What is a program?
A program is a set of instructions that you write to tell a computer what to do
What is a programming?
Programming is the task of writing those instructions in a language that the computer can understand.
Simple Circut

True Story
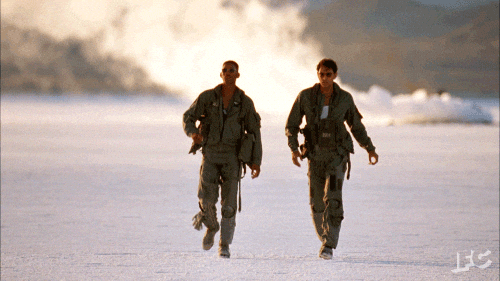
JAVASCRIPT

Variables
Declaration: let age
Assignment: age = 25
Both at the same time:
let age = 25
Conditional Syntax
if(condition is true) {
//Do cool stuff
}
Conditional Syntax
if(condition is true) {
//Do this cool stuff
}else if(condition is true){
//Do this other cool stuff
}else{
//Default cool stuff
}
Conditional Syntax
const pizza = "Dominos"
if (pizza === "Papa Johns") {
console.log("Scram!")
} else if(pizza === "Dominos") {
console.log("All aboard the train to flavor town")
} else {
console.log("What are you even doing with your life?")
}
🚨 DANGER 🚨
Assignment vs. Comparison
Multiple Conditions
if (name === "Leon" && status === "Ballin"){
//Wink at camera
}
Multiple Conditions
if (day === "Saturday" || day === "Sunday"){
//It is the weekend
}
Functions
-
Functions are simple sets of instructions!
-
Functions are reusable
-
Functions perform one action as a best practice
-
They form the basic "building blocks" of a program
Functions
function name(parameters){
//body
}
//call
name(arguments)
Functions
function yell(word){
alert(word)
}
yell("HELLO")
Functions
function yell(word, otherWord){
alert(word)
alert(otherWord)
}
yell("HELLO","GOODBYE")
Loops
-
Repeat an action some number of times!
-
Three main types of loops in Javascript
-
For, while, and do while loops
-
Each type offers a different way to determine the start and end points of a loop
Loops - For
for (let i = 1; i < 5; i++) {
console.log(i)
}
Arrays
-
A data structure to store ordered collections!
-
Array elements are numbered starting with zero
-
Arrays have many methods to manage the order of elements
-
Can be created by a constructor or literal notation
Toasters
Declaring Arrays
let newArr = []
Literal Notation
Declaring Arrays
newArr = ['Zebra',true,21]
Arrays are populated with elements
Elements can be of any type
Array Indexing

Array Indexing
newArr = ['Zebra',,true,21]
console.log( newArr[0] ) //Zebra
console.log( newArr[1] ) //undefined
console.log( newArr[2] ) //true
console.log( newArr[3] ) //21
Elements can be accessed by their index numbers
Array Indexing
newArr = ['Zebra',,true,21]
newArr[1] = 'Bob'
console.log( newArr )
// ['Zebra','Bob',true,21]
Elements can be updated by using the index at that position
Objects
-
Objects are a collection of variables and functions!
-
Objects represent the attributes and behavior of something used in a program
-
Object variables are called properties and object functions are called methods
-
Objects store "keyed" collections
Think of a physical object

What are it's attributes and behaviors?
Stopwatch Object
let stopwatch = {}
stopwatch.currentTime = 12
stopwatch.tellTime = function(time){
console.log(`The current time is ${time}.`)
}
stopwatch.tellTime(stopwatch.currentTime)
Car Factory
Look Ma! New syntax!
class MakeCar{
constructor(carMake,carModel,carColor,numOfDoors){
this.make = carMake
this.model = carModel
this.color = carColor
this.doors = numOfDoors
}
honk(){
alert('BEEP BEEP FUCKER')
}
lock(){
alert(`Locked ${this.doors} doors!`)
}
}
let hondaCivic = new MakeCar('Honda','Civic','Silver', 4)
let teslaRoadster = new MakeCar('Tesla','Roadster', 'Red', 2)
Classes are like templates for objects!
APIs
What are APIs?
APIs
-
A simple interface for some complex action!
-
Think of a restaurant menu! Remember those...
-
Lets one thing communicate with another thing without having to know how things are implemented.
APIs

APIs
Fetch Fido, Fetch!
fetch("https://dog.ceo/api/breeds/image/random")
.then(res => res.json()) // parse response as JSON
.then(data => {
console.log(data)
})
.catch(err => {
console.log(`error ${err}`)
});
API returns a JSON object that we can use within our apps
Backend!
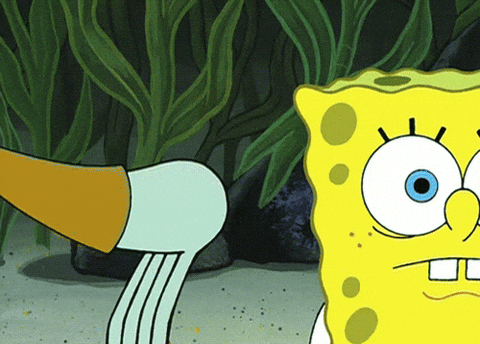
Butt first!
Javascript is
single-threaded
Synchronous aka processes
one operation at a time
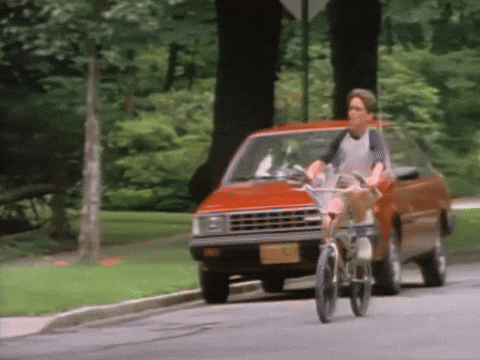
vs
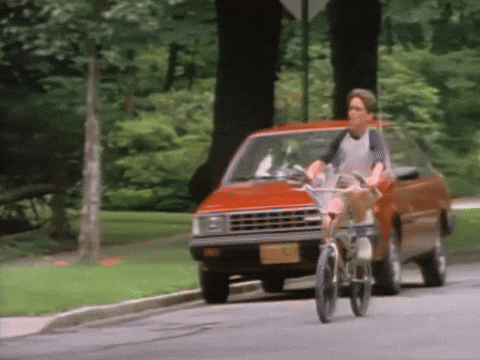
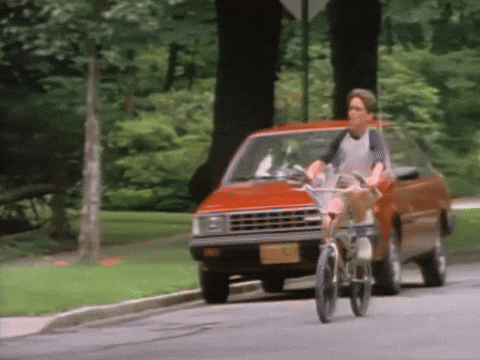
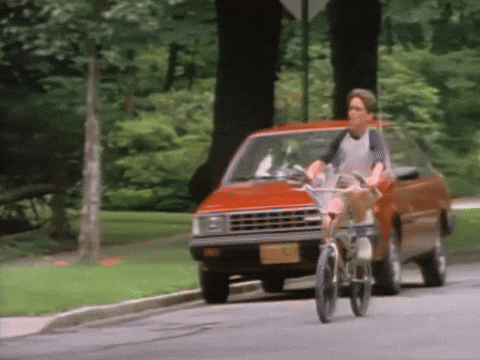
If synchronous, how do we do stuff like make an api request and keep scrolling or clicking
Things should block
THE ENVIRONMENT
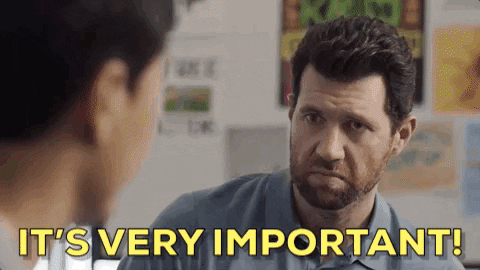
Not This
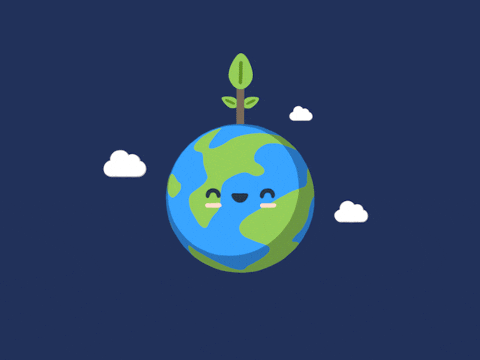
THIS
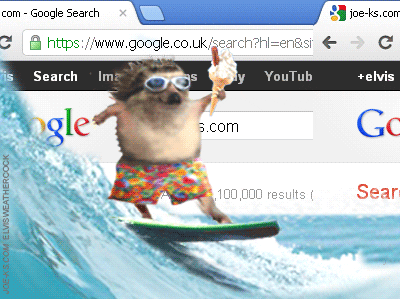
Our JS is running in
a browser
Browsers have a BUNCH of APIs we can use that are async and enable us to keeping looking a cute cat photos while those operations are being processed asynchronously
Common browser APIs
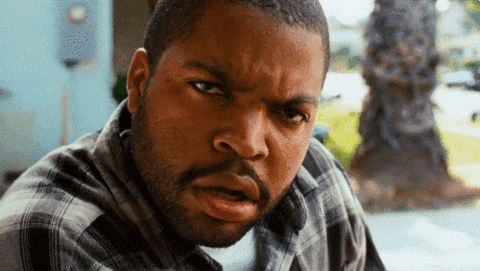
WAIT
WHAT THE FUCK
Actual words Leon said when figuring all this shit out...

So, yeah, JS can do a lot of "blocking" stuff in the browser because it is handing that stuff off to async
Web APIs
BUT
We are going to need to know how to handle responses coming back from those Web APIs
JS does this with callbacks, promises,
and eventually async/await
The Old School Way
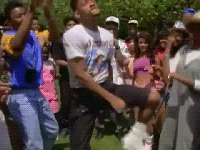
Callbacks
You can have a function that takes another function as an argument
aka Higher Order Function

You have seen this a million times
addEventListener('click', callback)
A Callback is the function that has been passed as an argument
Callbacks are not really "a thing" in JS
just a convention
Callback fires when async task or another function
is done
function houseOne(){
setTimeout(() => {
console.log('Paper delivered to house 1')
setTimeout(() => {
console.log('Paper delivered to house 2')
setTimeout(() => {
console.log('Paper delivered to house 3')
}, 3000)
}, 4000)
}, 5000)
}
houseOne()
Welcome To Hell
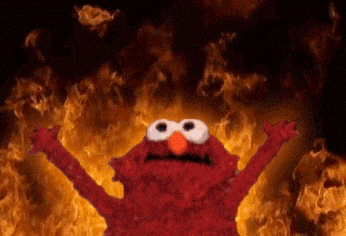
Callback Hell
What if there was a more readable way to handle async code
Promise
An object that MAY have a value in
the future
.then()
A promise object method that runs after the promise "resolves"
.then(value)
Whatever value the promise object has gets passed as an argument
We've Seen This Before
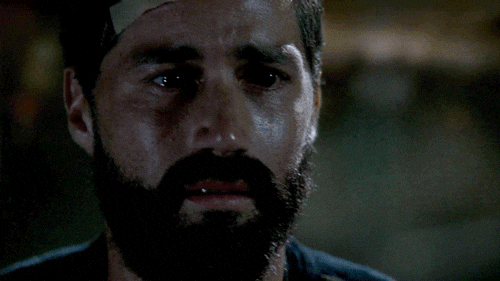
APIs
Fetch Fido, Fetch!
fetch("https://dog.ceo/api/breeds/image/random")
.then(res => res.json()) // parse response as JSON
.then(data => {
console.log(data)
})
.catch(err => {
console.log(`error ${err}`)
});
Fetch returns a Promise
Like a bunch of Web APIs running async code
function houseOne(){
return new Promise((resolve, reject) => {
setTimeout(() => {
resolve('Paper delivered to house 1')
}, 1000)
})
}
function houseTwo(){
return new Promise((resolve, reject) => {
setTimeout(() => {
resolve('Paper delivered to house 2')
}, 5000)
})
}
function houseThree(){
return new Promise((resolve, reject) => {
setTimeout(() => {
resolve('Paper delivered to house 3')
}, 2000)
})
}
houseOne()
.then(data => console.log(data))
.then(houseTwo)
.then(data => console.log(data))
.then(houseThree)
.then(data => console.log(data))
.catch(err => console.log(err))
Chaining Don't Read Good
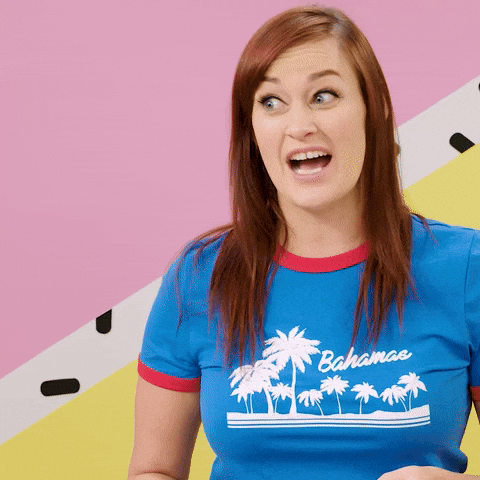
I want my asynchronous code to look sychronous
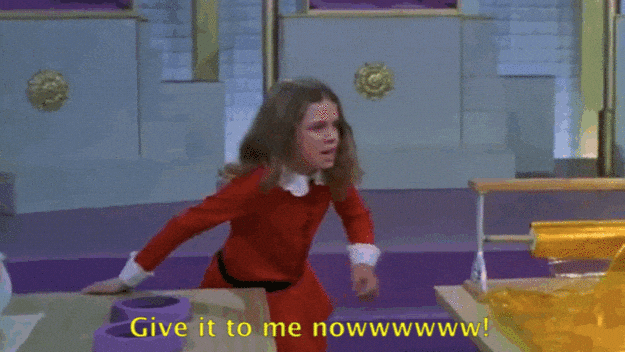
Async / Await
A way to handle async responses
Promises Under The Hood
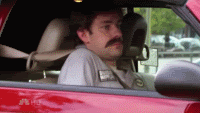
Await waits for an async process to complete inside an Async Function
function houseOne(){
return new Promise((resolve, reject) => {
setTimeout(() => {
resolve('Paper delivered to house 1')
}, 1000)
})
}
function houseTwo(){
return new Promise((resolve, reject) => {
setTimeout(() => {
resolve('Paper delivered to house 2')
}, 5000)
})
}
function houseThree(){
return new Promise((resolve, reject) => {
setTimeout(() => {
resolve('Paper delivered to house 3')
}, 2000)
})
}
async function getPaid(){
const houseOneWait = await houseOne()
const houseTwoWait = await houseTwo()
const houseThreeWait = await houseThree()
console.log(houseOneWait)
console.log(houseTwoWait)
console.log(houseThreeWait)
}
getPaid()
async function getPaid(){
const houseOneWait = await houseOne()
const houseTwoWait = await houseTwo()
const houseThreeWait = await houseThree()
console.log(houseOneWait)
console.log(houseTwoWait)
console.log(houseThreeWait)
}
getPaid()
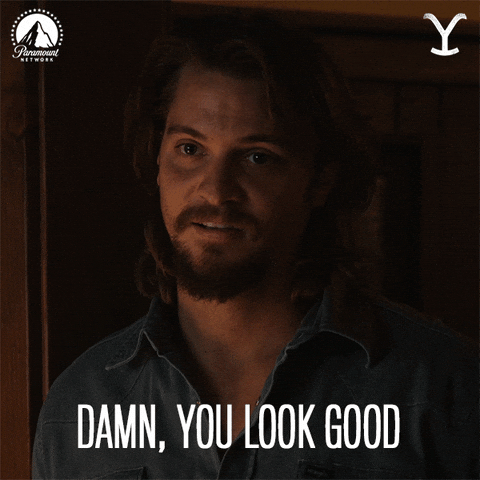
I Need Something Real
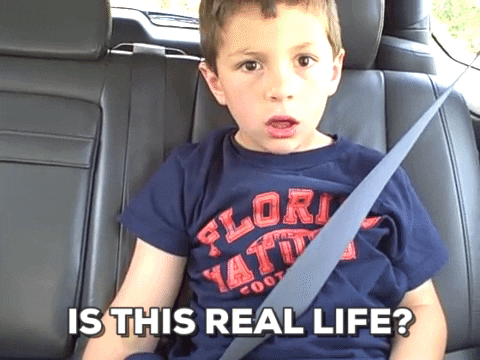
APIs
Fetch Fido, Fetch!
async function getACuteDogPhoto(){
const res = await fetch('https://dog.ceo/api/breeds/image/random')
const data = await res.json()
console.log(data)
}
getACuteDogPhoto()
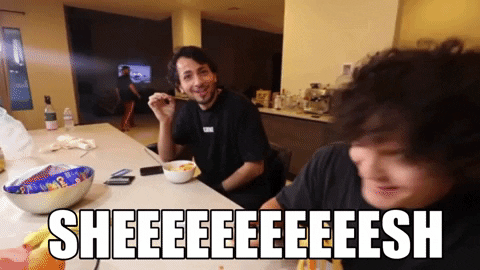
Let's Use A Web API
setTimeout()
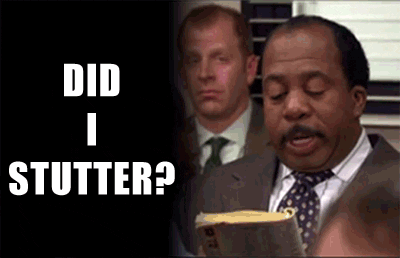
setTimeout and setInterval are not part of the Javascript specification...
Most environments include them...
like all browsers and Node.js
function houseOne(){
console.log('Paper delivered to house 1')
}
function houseTwo(){
setTimeout(() => console.log('Paper delivered to house 2'), 3000)
}
function houseThree(){
console.log('Paper delivered to house 3')
}
houseOne()
houseTwo()
houseThree()
function houseOne(){
console.log('Paper delivered to house 1')
}
function houseTwo(){
setTimeout(() => console.log('Paper delivered to house 2'), 0)
}
function houseThree(){
console.log('Paper delivered to house 3')
}
houseOne()
houseTwo()
houseThree()
EVENT LOOP
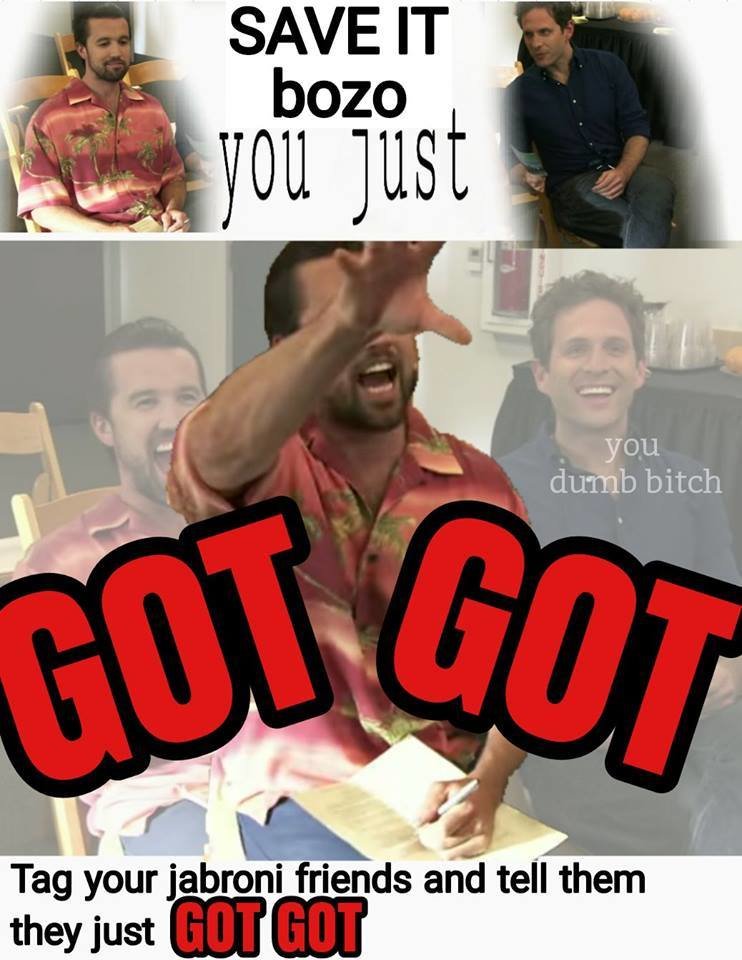
Time for some data structures
Queue

Like a real queue, the first element which was added to the list, will be the first element out.
This is called a FIFO (First In First Out) structure.
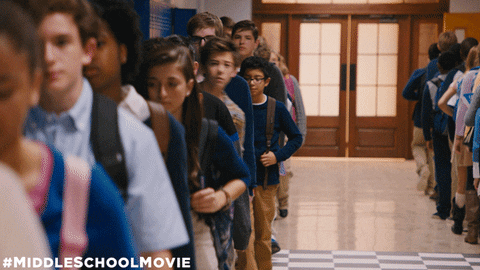
let queue = []
queue.push(2) // queue is now [2]
queue.push(5) // queue is now [2, 5]
let i = queue.shift() // queue is now [5]
alert(i) // displays 2
Queue Example
Stack
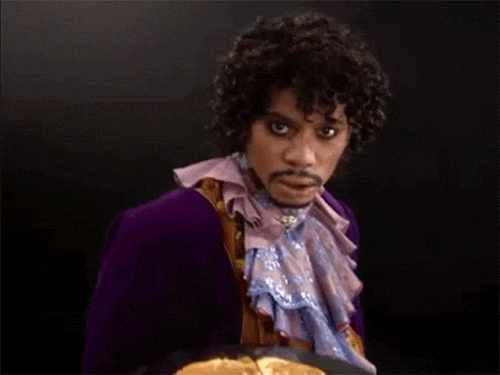
The first pancake made, is the last pancake served.
This is called a stack.
The first element which was added to the list, will be the last one out. This is called a LIFO (Last In First Out) structure.
let stack = []
stack.push(2) // stack is now [2]
stack.push(5) // stack is now [2, 5]
let = stack.pop() // stack is now [2]
alert(i) // displays 5
Stack Example
Back To Getting Got
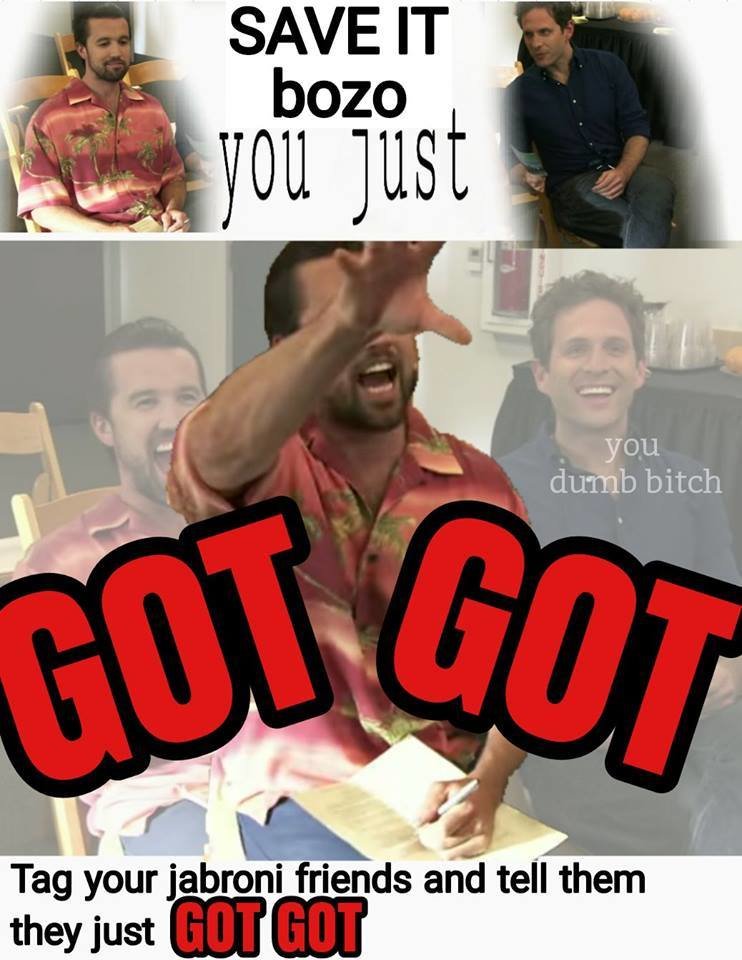
JS IS RUNNING IN THE BROWSER
V8 Engine
(Parse Code > Runnable Commands)
Window Runtime (Hosting Environment)
Gives Us Access To Web APIs
Passes stuff to Libevent (Event Loop)
The Event Loop monitors the Callback Queue and Job Queue and decides what needs to be pushed to the Call Stack.
Call Stack
Web APIs
Task Queue
(Also, a job queue)
console.log('Paper delivered to house 1')
setTimeout(() => {
console.log('Paper delivered to house 2'), 0)
}
console.log('Paper delivered to house 3')
Console
main()
Call Stack
Web APIs
Task Queue
(Also, a job queue)
console.log('Paper delivered to house 1')
setTimeout(() => {
console.log('Paper delivered to house 2'), 0)
}
console.log('Paper delivered to house 3')
Console
main()
log('house 1')
Call Stack
Web APIs
Task Queue
(Also, a job queue)
console.log('Paper delivered to house 1')
setTimeout(() => {
console.log('Paper delivered to house 2'), 0)
}
console.log('Paper delivered to house 3')
Console
main()
log('house 1')
Call Stack
Web APIs
Task Queue
(Also, a job queue)
console.log('Paper delivered to house 1')
setTimeout(() => {
console.log('Paper delivered to house 2'), 0)
}
console.log('Paper delivered to house 3')
Console
main()
log('house 1')
setTimeout()
Call Stack
Web APIs
Task Queue
(Also, a job queue)
console.log('Paper delivered to house 1')
setTimeout(() => {
console.log('Paper delivered to house 2'), 0)
}
console.log('Paper delivered to house 3')
Console
main()
log('house 1')
setTimeout()
Call Stack
Web APIs
Task Queue
(Also, a job queue)
console.log('Paper delivered to house 1')
setTimeout(() => {
console.log('Paper delivered to house 2'), 0)
}
console.log('Paper delivered to house 3')
Console
main()
log('house 1')
setTimeout() - cb or promise...
log('house 3')
Call Stack
Web APIs
Task Queue
(Also, a job queue)
console.log('Paper delivered to house 1')
setTimeout(() => {
console.log('Paper delivered to house 2'), 0)
}
console.log('Paper delivered to house 3')
Console
log('house 1')
setTimeout() - cb or promise...
log('house 3')
Call Stack
Web APIs
Task Queue
(Also, a job queue)
console.log('Paper delivered to house 1')
setTimeout(() => {
console.log('Paper delivered to house 2'), 0)
}
console.log('Paper delivered to house 3')
Console
cb / promise
log('house 1')
log('house 3')
Call Stack
Web APIs
Task Queue
(Also, a job queue)
console.log('Paper delivered to house 1')
setTimeout(() => {
console.log('Paper delivered to house 2'), 0)
}
console.log('Paper delivered to house 3')
Console
log('house 1')
log('house 3')
log('house 2')
Backend BABY
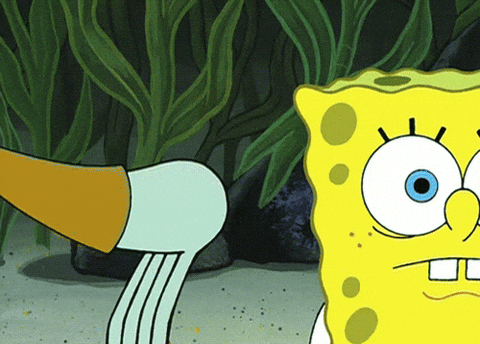
Does Javascript have access to the DOM natively (built in)?
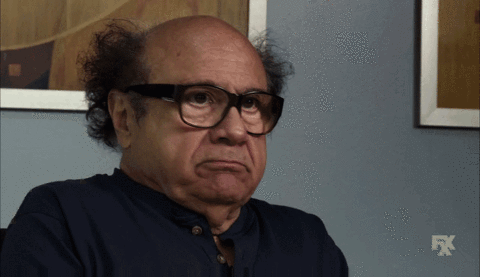
Javascript needed Web APIs to handle async and a bunch of stuff in the Browser
JS is a language that can only do what the hosting environment allows
What Do Servers Need?
Disk Access
(hardrive/ssd)
&&
Network Access
(internet, request / responses)
What if there was a hosting environment that allowed JS to have disk and network access
Music & Light Warning - Next Slide
NODE.js BABY
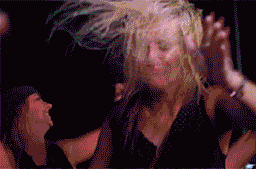
Node.js is a JavaScript runtime built on Chrome's V8 JavaScript engine.


The same shit that lets you run JS in the browser can now be used to run JS on Servers, Desktops, and elsewhere
True Story
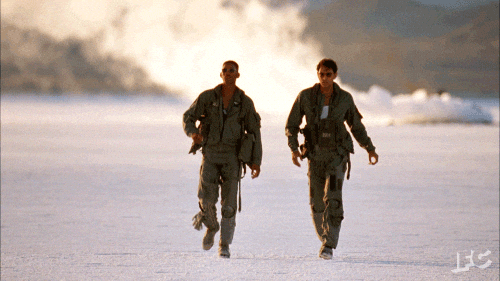
V8 Engine Does All The Heavy Lifting
Engine Vs. Compiler
And just like the browser's Web APIs Node come with a bunch of stuff
Built in Modules
(libraries or collections of functions)
HTTP (network access)
FS (file system access)
Access to millions of packages via NPM
(groupings of one or more custom modules)
Not Web APIs, but C/C++ APIs

sorry, don't remember the source
Releases?
LTS, Current, Nightly?

Let's Code
Simple Node Server

Just
HTTP & FS
const http = require('http')
const fs = require('fs')
http.createServer((req, res) => {
fs.readFile('demofile.html', (err, data) => {
res.writeHead(200, {'Content-Type': 'text/html'})
res.write(data)
res.end()
})
}).listen(8000)
Music & Light Warning - Next Slide
Let's Look
More Complex Backend

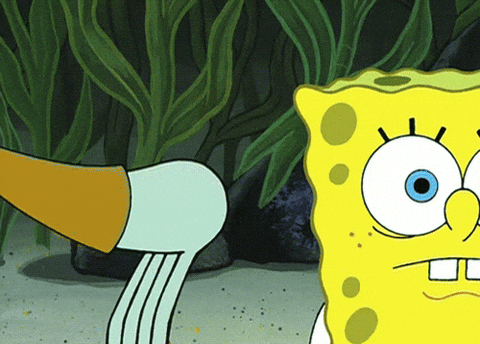
How could we clean this up?
Express
Express
Fast, unopinionated, minimalist web framework for Node.js
With a myriad of HTTP utility methods and middleware at your disposal, creating a robust API is quick and easy.

But First
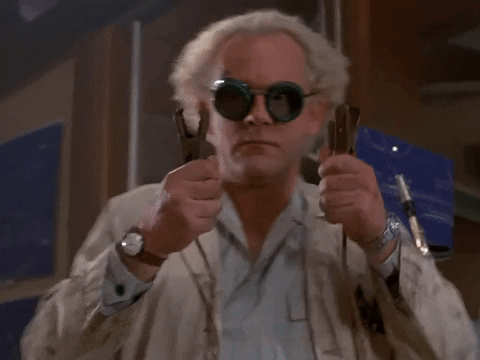
Blast To The Past
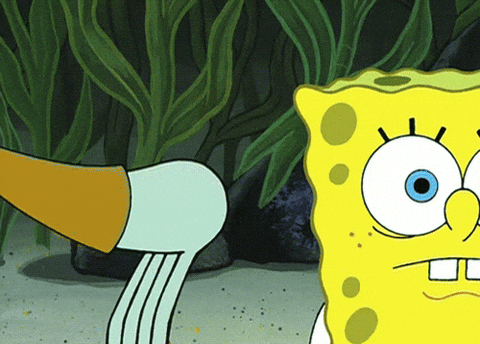
How Does The Internet Work
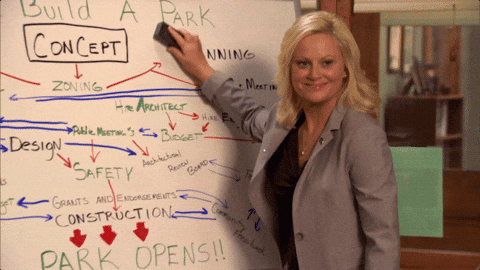
CRUD
Create (post) - Make something
Read (get) - Get Something
Update (put) - Change something
Delete (delete) - Remove something
Mongo DB
Collection
document
document
document
document
EJS
(Embedded Javascript Templates)
<h1>Current Rappers</h1>
<ul class="rappers">
<% for(let i=0; i < info.length; i++) {%>
<li class="rapper">
<span><%= info[i].stageName %></span>
<span><%= info[i].birthName %></span>
<span class='del'>delete</span>
</li>
<% } %>
</ul>
<h2>Add A Rapper:</h2>
Client (Laptop)
Browser
URL
Server
Disk
API Code
Files
Mongo
Database
Collection
Collection
document
document
document
document
app.get('/')
app.post('/form')
app.put('/info')
app.delete('/info')
/views
/public
index.ejs
main.js
style.css
Fonts
Images
Rap Names
What are some Create (post) requests?
Rap Names
What are some Read (get) requests?
Rap Names
What are some Update (put) requests?
Rap Names
What are some Delete (delete) requests?
Client (Laptop)
Browser
URL
Server
Disk
API Code
Files
Mongo
Database
Collection
Collection
document
document
document
document
app.get('/')
app.post('/form')
app.put('/info')
app.delete('/info')
/views
/public
index.ejs
main.js
style.css
Fonts
Images
Let's Build An App with Express
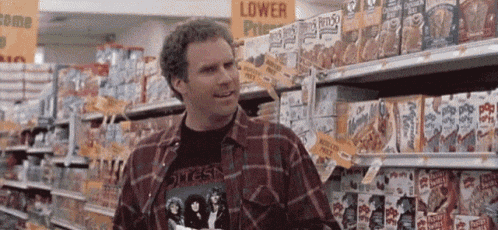
Key Steps
mkdir api-project
cd api-project
npm init
npm install express --save
npm install mongodb --save
npm install ejs --save
Setting Up The Project
let db,
dbConnectionStr = 'mongodb+srv://demo:demo@cluster0
.2wapm.mongodb.net/rap?retryWrites=true&w=majority',
dbName = 'rap'
MongoClient.connect(dbConnectionStr, { useUnifiedTopology: true })
.then(client => {
console.log(`Connected to ${dbName} Database`)
db = client.db(dbName)
})
Connect To DB
app.set('view engine', 'ejs')
app.use(express.static('public'))
app.use(express.urlencoded({ extended: true }))
app.use(express.json())
app.listen(process.env.PORT || PORT, ()=>{
console.log(`Server running on port ${PORT}`)
})
Setup Server
app.get('/',(request, response)=>{
db.collection('rappers').find().toArray()
.then(data => {
response.render('index.ejs', { info: data })
})
.catch(error => console.error(error))
})
API - GET
app.post('/addRapper', (request, response) => {
db.collection('rappers').insertOne(request.body)
.then(result => {
console.log('Rapper Added')
response.redirect('/')
})
.catch(error => console.error(error))
})
API - POST
Create EJS File
<h1>Current Rappers</h1>
<ul class="rappers">
<% for(let i=0; i < info.length; i++) {%>
<li class="rapper">
<span><%= info[i].stageName %></span>
<span><%= info[i].birthName %></span>
<span class='del'>delete</span>
</li>
<% } %>
</ul>
<h2>Add A Rapper:</h2>
Create Public Folder/Files
app.delete('/deleteRapper', (request, response) => {
db.collection('rappers').deleteOne({stageName: request.body.stageNameS})
.then(result => {
console.log('Rapper Deleted')
response.json('Rapper Deleted')
})
.catch(error => console.error(error))
})
API - DELETE
heroku login -i
heroku create simple-rap-api
echo "web: node server.js" > Procfile
git add .
git commit -m "changes"
git push heroku main
Push To Heroku
Let's Code
Rap Names App

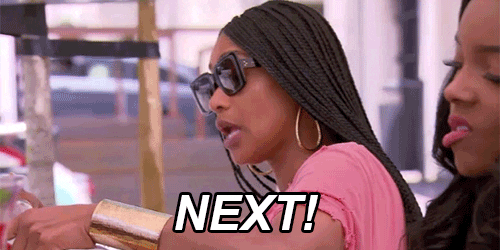
Let's Plan A Todolist
Todo List
What are some Create (post) requests?
Todo List
What are some Read (get) requests?
Todo List
What are some Update (put) requests?
Todo List
What are some Delete (delete) requests?
Client (Laptop)
Browser
URL
Server
Disk
API Code
Files
Mongo
Database
Collection
Collection
document
document
document
document
app.get('/')
app.post('/form')
app.put('/info')
app.delete('/info')
/views
/public
index.ejs
main.js
style.css
Fonts
Images
Let's Look At Our App With Authentication
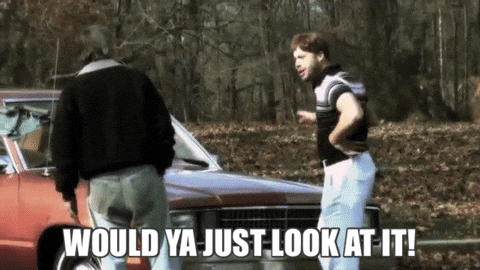
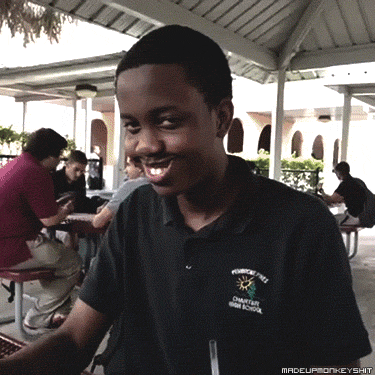
Live footage of me after adding auth
Authentication? Logged In Users? HOW?
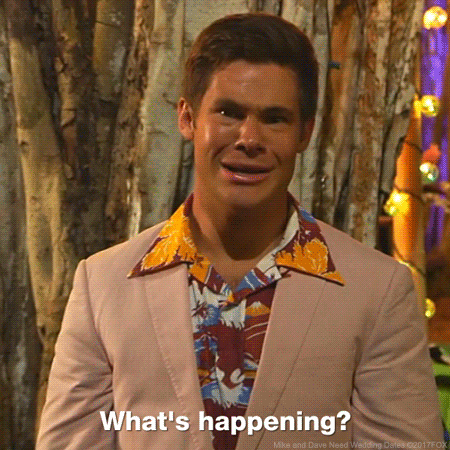
Passport is authentication middleware for Node.js. Extremely flexible and modular, Passport can be unobtrusively dropped in to any Express-based web application.
Passport.js
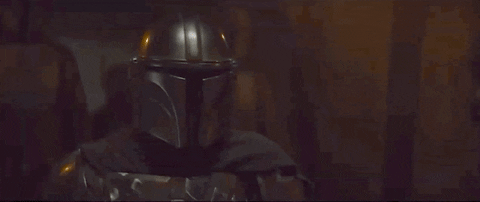
I'm going to embrace the magic
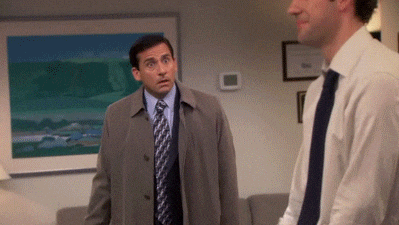
Live footage of me using passport and AAD
Passport Has Strategies
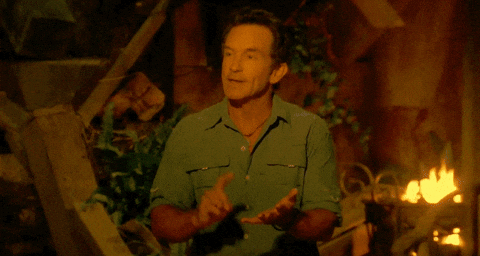
Each application has unique authentication requirements. Authentication mechanisms, known as strategies, are packaged as individual modules.
It's Really Just Magic
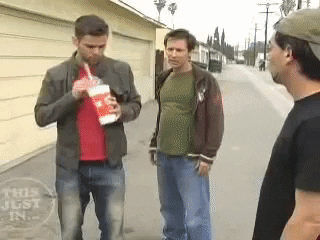
Music & Light Warning - Next Slide
How Do We Stay Signed In?
Express.js uses a cookie to store a session id (with an encryption signature) in the user's browser and then, on subsequent requests, uses the value of that cookie to retrieve session information stored on the server.
This server side storage can be a memory store (default) or any other store which implements the required methods

Let's Find The Cookies
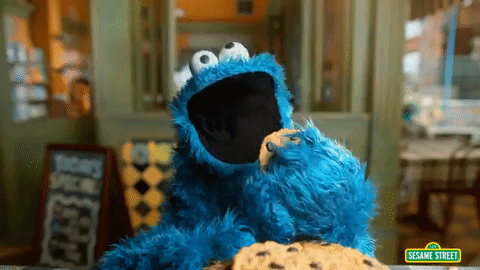
Let's Find The Store
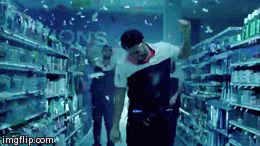
req.user

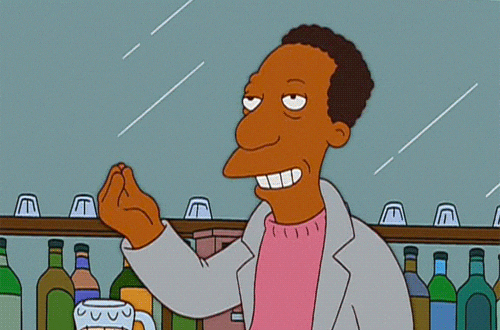
Ok, so...
Our Users Are Logged In
BUT WHY?
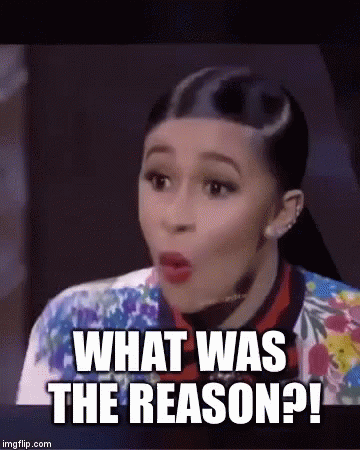
Let Me Show You
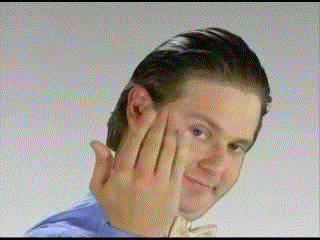
EnsureAuth, BABY!


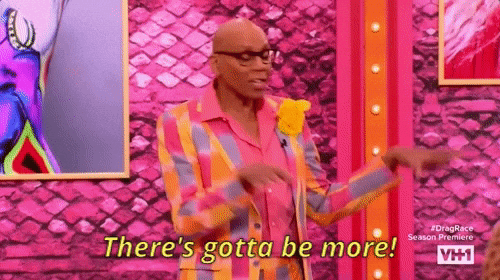
If we have logged in users, what can we dream up?
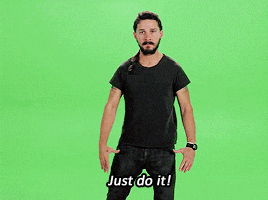
Let's Build An App with Passport & Express
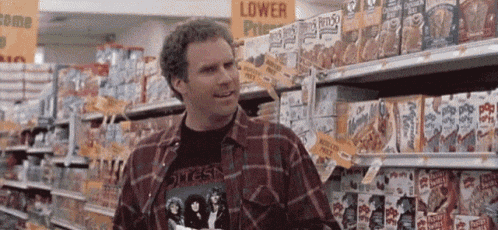
Real Quick
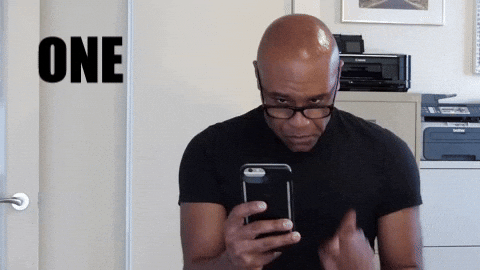
Mongoose
Collection
document
document
document
document
Mongoose provides a straight-forward, schema-based solution to model your application data.
Mongoose
Collection
document
document
document
document
Each schema maps to a MongoDB collection and defines the shape of the documents within that collection.
const UserSchema = new mongoose.Schema({
microsoftId: {
type: String,
required: true,
},
displayName: {
type: String,
required: true,
}
})
Mongoose
Collection
document
document
document
document
Models are fancy constructors compiled from Schema definitions. An instance of a model is called a document.
Models are responsible for creating and reading documents from the underlying MongoDB database.
module.exports =
mongoose.model('User', UserSchema)
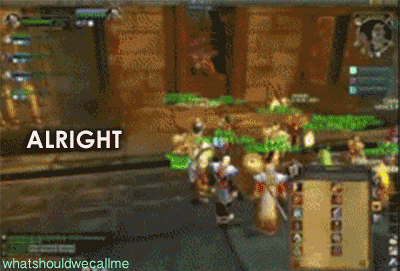
mkdir todo-mvc-auth-microsoft
cd todo-mvc-auth-microsoft
npm init
npm i connect-mongo dotenv ejs
express express-session mongodb mongoose
nodemon passport passport-azure-ad --save
Setting Up The Project
DB_STRING =
mongodb+srv://demo:demo@cluster0.2wapm.mongodb.net/rap?retryWrites=true&w=majority
Using .env
.env file
add .env and config to .gitignore
.env
node_modules
config/config.js
exports.destroySessionUrl = 'http://localhost:2121'
exports.databaseUri = 'mongodb://localhost/OIDCStrategy'
Using config
config.js file
const mongoose = require('mongoose')
const connectDB = async () => {
try {
const conn = await mongoose.connect(process.env.DB_STRING, {
useNewUrlParser: true,
useUnifiedTopology: true,
useFindAndModify: false,
})
console.log(`MongoDB Connected: ${conn.connection.host}`)
} catch (err) {
console.error(err)
process.exit(1)
}
}
module.exports = connectDB
DB Setup
const express = require('express')
const app = express()
const mongoose = require('mongoose')
const passport = require('passport')
const session = require('express-session')
const MongoStore = require('connect-mongo')(session)
const connectDB = require('./config/database')
const authRoutes = require('./routes/auth')
const homeRoutes = require('./routes/home')
const todoRoutes = require('./routes/todos')
require('dotenv').config({path: './config/.env'})
require('./config/passport')(passport)
Setup Server
const connectDB = require('./config/database')
connectDB()
Connect To DB
app.set('view engine', 'ejs')
app.use(express.static('public'))
app.use(express.urlencoded({ extended: true }))
app.use(express.json())
app.listen(process.env.PORT || PORT, ()=>{
console.log(`Server running on port ${PORT}`)
})
Setup Server
// Sessions
app.use(
session({
secret: 'keyboard cat',
resave: false,
saveUninitialized: false,
store: new MongoStore({ mongooseConnection:
mongoose.connection }),
})
)
// Passport middleware
app.use(passport.initialize())
app.use(passport.session())
Setup Session & Passport
const authRoutes = require('./routes/auth')
const homeRoutes = require('./routes/home')
const todoRoutes = require('./routes/todos')
app.use('/', homeRoutes)
app.use('/auth', authRoutes)
app.use('/todos', todoRoutes)
Setup Routes
Create EJS File
<h2>
<%= user.displayName %> has <%= left %>
things left to do.
</h2>
Create Public Folder/Files
MVC


Let's make the nuns proud
app.get('/',(request, response)=>{
db.collection('rappers').find().toArray()
.then(data => {
response.render('index.ejs', { info: data })
})
.catch(error => console.error(error))
})
Old API - GET
//Routes File
app.use('/todos', todoRoutes)
//Route to Controller
router.get('/', ensureAuth, todosController.getTodos)
//Controller talking to model
getTodos: async (req,res)=>{
console.log(req.user)
try{
const todoItems = await Todo.find()
const itemsLeft = await Todo.countDocuments(
{microsoftId: req.user.microsoftId, completed: false}
)
res.render('todos.ejs',
{todos: todoItems,
left: itemsLeft,
user: req.user}
)
}catch(err){
console.log(err)
}
}
New API - GET
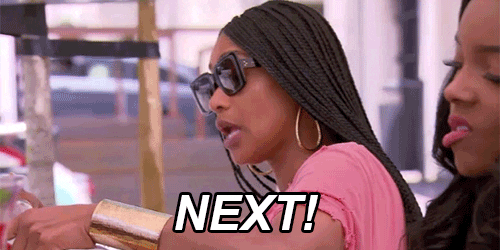
Let's Plan
A Social Network
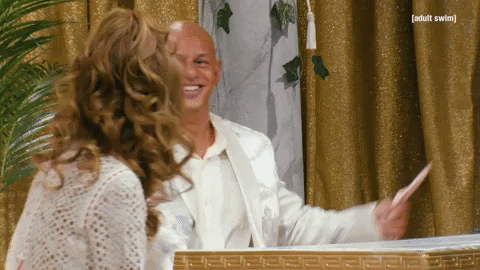
Grab The Checklist
!checklist
Passing The Sniff
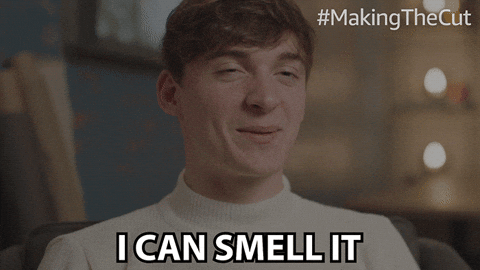
Bootcamper!
PUSH EVERY DAY
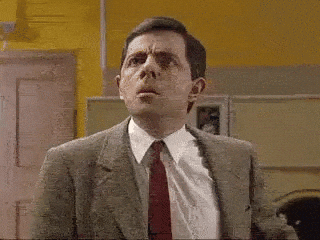
HITLIST
One of the most important things you will ever do for your career
USE THE SHEET!
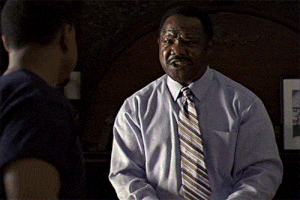
NOW WITH TWO TABS!: Google Sheet
60 Networked
RECOMMENDED
Applications
Find the hiring manager:
- Add them to the hitlist
- Follow them on Twitter
- Find their email (hunter.io)
- Ask for a coffee chat
- Send a thank you
- Get the recommendation
IF YOU EVER JUST CLICK APPLY
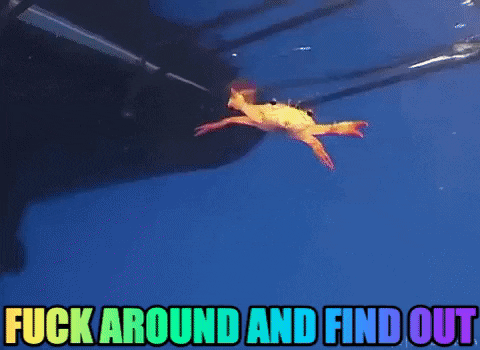
30 High Value Apps
Custom Resume, Cover Letter, and Story
10 Premium Apps
Custom everything plus, tweets, blog, and project
100Hours Project
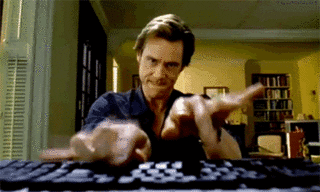
Tie your past to your present and show you can code
100 Hours Project
Description (one paragraph)
Wireframe (wireframe.cc, figma, balsamiq)
Interviewing
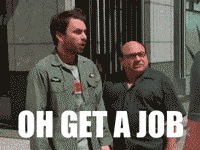
Process
- Apply / Recruited / Recommended
- Phone Screen
- Behavioral
- Technical Questions
- Take Home
- Whiteboard
- In-person
- Wine and Dine Interview
- Offer
*Every Company Is Wildly Different
Once Recommended
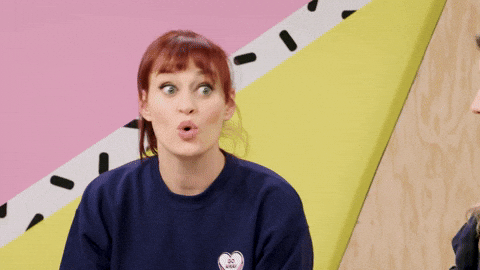
WHAT ARE THE STEPS IN THIS PROCESS?
WHAT ARE THE STEPS IN THIS PROCESS?
Once Recommended
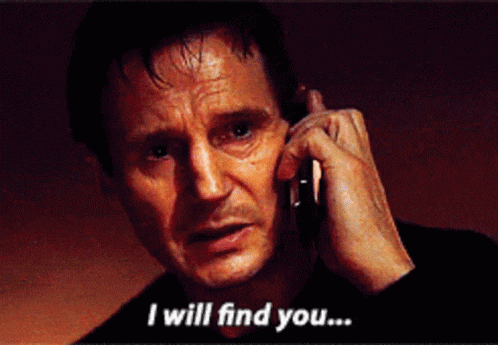
Find EVERYTHING about their process!
Glassdoor
Github
Blind
How to prepare?
The Bank
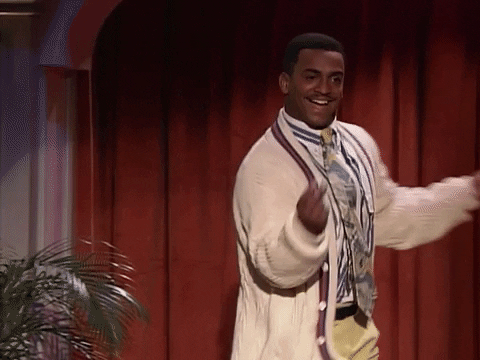
Behavioral
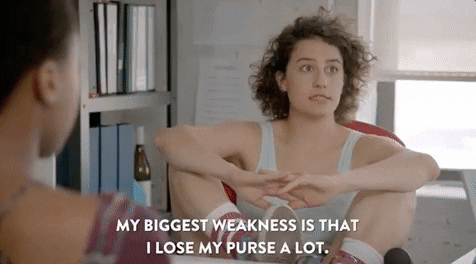
C.A.R.
Cause Action Result
CAUSE
At my last company...
At my last opportunity...
LET THEM DIG!
ACTION
Steps you took
MUST BE POSITIVE
NO TIME TO BE HUMBLE DURING THE HUNT
RESULT
How are you better because of it
How are you the best thing since sliced bread because of it
Technical Questions
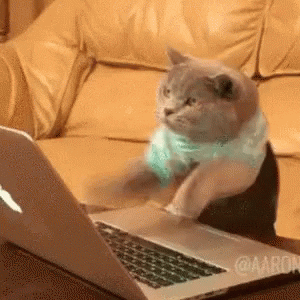
Explanation, Use, Example
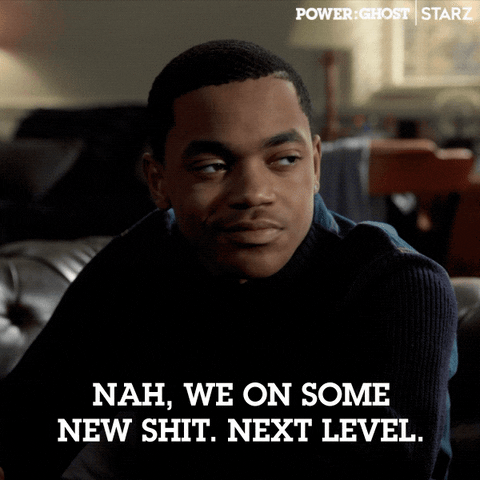
Technical Whiteboard
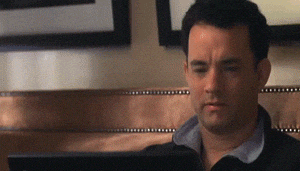
P.R.E.P
Parameters, Returns, Examples, Pseudo Code
Homework
Do: Start prepping THE BANK
Do: Complete Your HITLIST
Finish: Review Binary Upload Boom
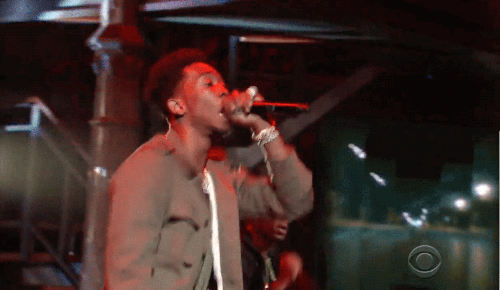
Backend Crash Course #100Devs (cohort 2)
By Leon Noel
Backend Crash Course #100Devs (cohort 2)
Backend Crash Course Review for your Free Web Dev Bootcamp for folx affected by the pandemic. Join live T/Th 6:30pm ET leonnoel.com/twitch and ask questions here: leonnoel.com/discord
- 1,684