See React
Leon Noel
"Bad-ass kids, we was racein with the streetlight "

Agenda
Questions
About last class or life

Music & Light Warning - Next Slide
React
Read The Docs
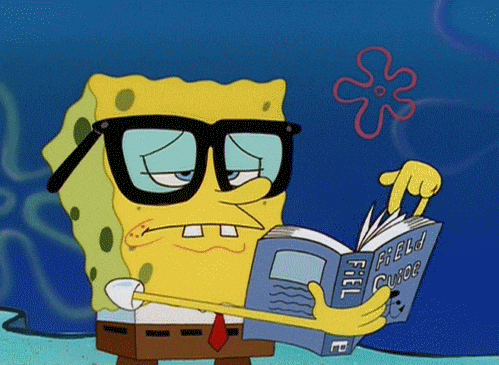
ES6+
Review
Classes
class MakeCar{
constructor(carMake,carModel,carColor,numOfDoors){
this.make = carMake
this.model = carModel
this.color = carColor
this.doors = numOfDoors
}
honk(){
alert('BEEP BEEP FUCKER')
}
lock(){
alert(`Locked ${this.doors} doors!`)
}
}
let hondaCivic = new MakeCar('Honda','Civic','Silver', 4)
let teslaRoadster = new MakeCar('Tesla','Roadster', 'Red', 2)
Classes are like templates for objects!
const foo = ['a', 'b', 'c']
const bar = ['d', 'e', 'f']
console.log(...foo)
console.log([...foo, ...bar])
"a" "b" "c"
["a", "b", "c", "d", "e", "f"]
Array Spread
const defaultStyle = {
color: 'black',
fontSize: 12,
fontWeight: 'normal'
}
const style = {
...defaultStyle,
fontWeight: 'bold',
backgroundColor: 'white'
}
console.log(style)
Object {
color: "black", fontSize: 12,
fontWeight: "bold", backgroundColor: "white"
}
Object Spread
let [name, team] = ["Bob", "RC"];
console.log(name);//"Bob"
console.log(team);//"RC"
Array Destructuring
let {name, team} = {"Bob", "RC"};
console.log(name);//"Bob"
console.log(team);//"RC"
Object Destructuring
🚨 WARNING 🚨

React
React is a library for handling your UI.
It allows you to write components which you can re-use and
extend when needed. It does not handle anything else!

React APIs
"react" contains the APIs for creating components
"react-dom" contains the APIs for rendering to the browser DOM
JSX
React components are functions which return JSX
A syntax extension to JS!
JSX is a shortcut for using the React.createElement() API, that is
much more concise, easy to read, and visually looks a little like the generated UI (as both are tree-like).
this.props
Component API
Props are arguments passed into React Components
Components can be configured upon instantiation by
passing properties to the constructor
this.state
Component API
React components have a built-in state object
This is where you store stuff related to the component
When the state object changes, the component
REACTS and re-renders!
Virtual DOM
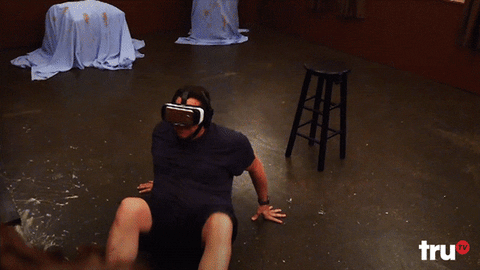
Function vs. Class Components
Function components can now have state via hooks
React Todolist
Just Add
You should have already watched Traversy Media React JS Crash Course
Questions
- What is React.js
- Why would you use React.js
- What are components
- What is JSX
- What are props
- What is state
- What are the differences btw/ functional and class based components
- Give an example of a functional and class based component
- What are React Hooks
- How does useState work
- Give an example of useState
Homework
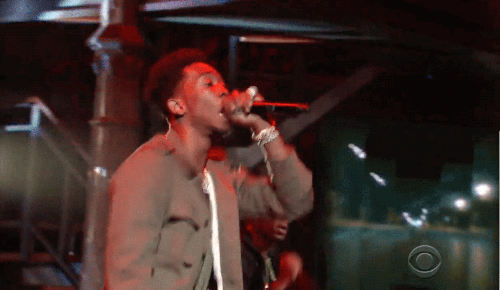
RC - See React
By Leon Noel
RC - See React
- 654