Node One
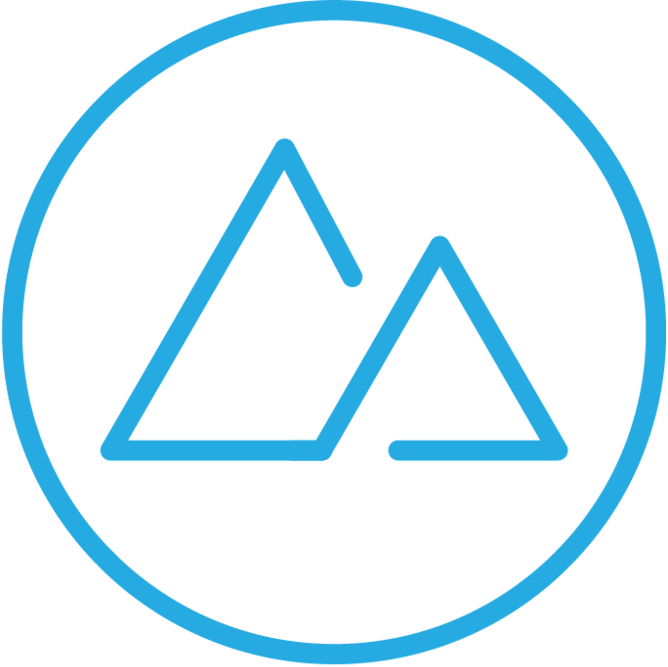
Servers and Node.js with Express
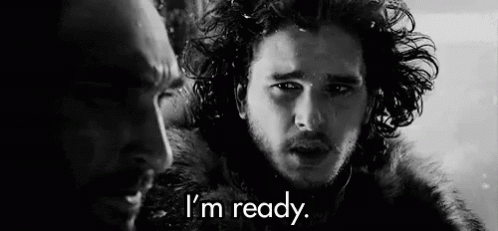
Client-Server Model
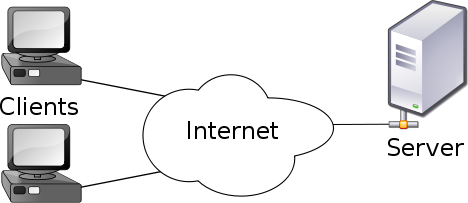
Request
Request
Response
Response
What is Node.js?
Node is a JavaScript runtime environment.
Traditionally, JavaScript could only be executed in browsers.
Node is what allows JavaScript to be able to run outside of the browser.
Node is built on Chrome's V8 JavaScript engine.
Using Node in the Terminal
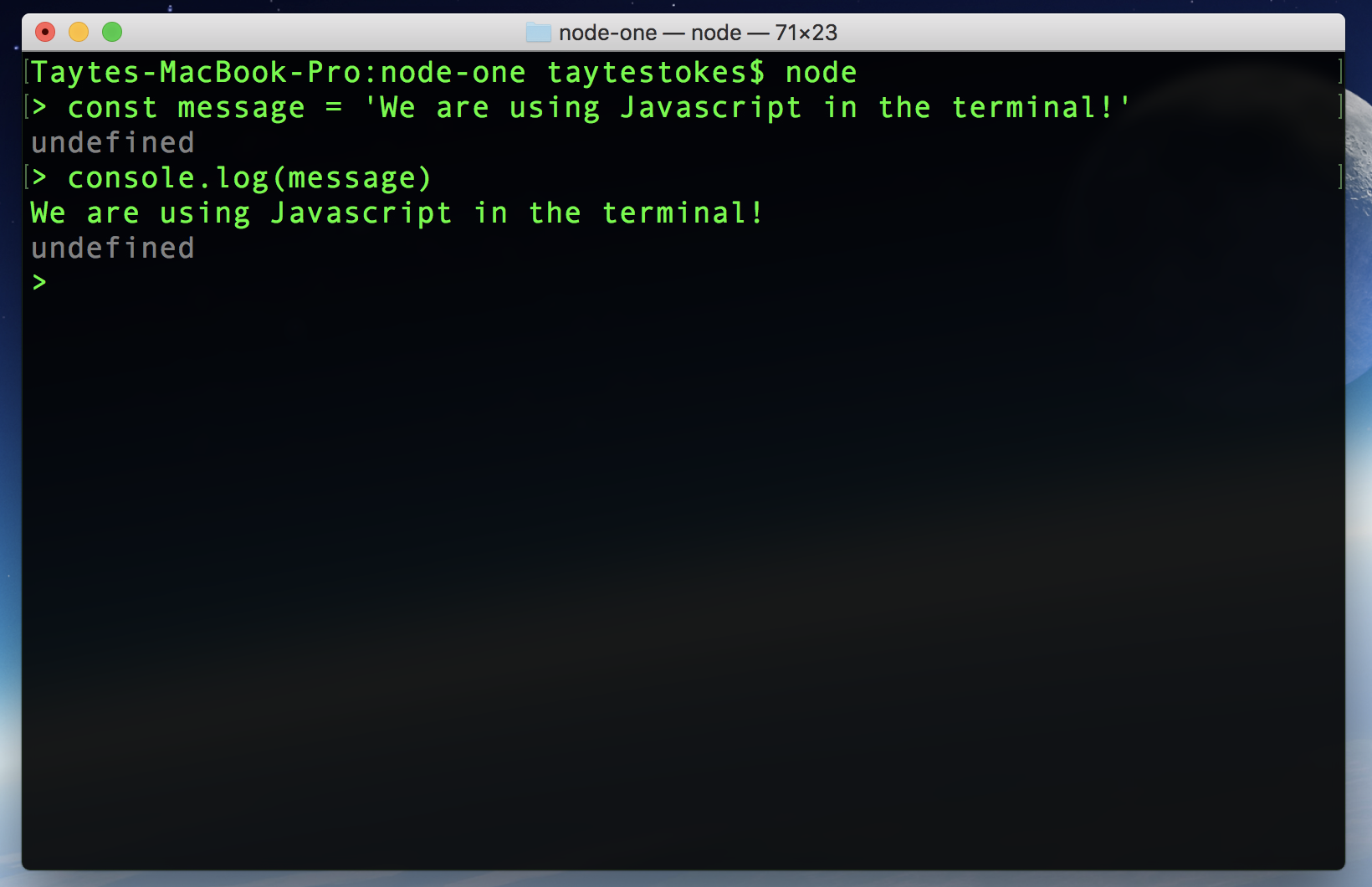
Run command 'node'
Write JavaScript
Using Node with JS Files
Node can also be used to run JavaScript files.
To do this, use the command 'node' followed by the name of the JS file you want to run.
node index.js
The command above would run JavaScript file 'index.js'.
Nodemon
Note that Node only runs the file once.
If any changes are made to your code, Node needs to be executed again.
Fortunately, there is a package called Nodemon that can 'watch' our JavaScript files, and restart Node for us.
To install Nodemon, run the following command:
npm install -g nodemon
nodemon index.js
To run Nodemon with a file, just type:
What is NPM?
We've used NPM a lot, but what is it?
NPM stands for Node Package Manager.
NPM is what gives us access to many great packages and libraries to use, such as Nodemon, create-react-app, and more.
NPM Continued
When creating a Node.js project, we may want to add packages.
To accomplish that we need a package.json and a node_modules folder.
We can create these by running the following NPM command:
npm init -y
Note: Without the '-y' flag, npm will prompt for some configuration settings. Adding the -y says yes to all the default settings.
Express
Express is a minimal, flexible Node.js framework that is great for building servers.
To install Express, run the following in your terminal:
npm install express
You're now ready to set up a server file!
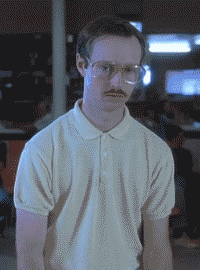
Server Setup
To set up a server, Express needs to be required (imported) to the top of the file.
Then, you need to include the following lines like so:
const express = require('express');
const app = express()
app.use(express.json())
app.listen(4040, () => console.log('Server running on 4040'))
Require express
Variable equal to express invoked
Server listens to requests on specified port
Parses JSON into JavaScript
Run Nodemon with your server file to see it live!
Creating Endpoints
With a server, we can create endpoints.
Endpoints are where we define how our server, or API, can be interacted with.
To build an endpoint, we specify the type of request the server can receive, the endpoint URL for the request, and any handler functions we would like to fire when receiving that request.
app.get('/api/users', handlerFunction)
Express Invoked Variable
REST method
Endpoint
Handler Function
Endpoint Handler Functions
Handler functions determine what functionality should happen when a specific request is made to a given endpoint.
Handler functions will receive request (req) and response (res) object parameters.
app.get('/api/users', (req, res) => {
//functionality goes here
})
Using Params
To create an endpoint that accepts params, a place holder needs to be created on the server's endpoint.
app.get('/api/users/:id')
Params:
{ id: }
Using Queries
When creating an endpoint that takes queries, NOTHING is needed to specify the query object in the endpoint.
The query object can be handled in the handler function off of the request object if the user created queries on their request.
app.get('/api/users/:id')
Endpoint can receive queries without any changes server-side
The Request Object
The request object is passed in by express as an argument to handler functions.
It contains many things, but most importantly contains the params, query, and body objects that can be sent in a request.
The params, query, and body can be accessed through the request object like so:
app.put('/api/users/:id', (req, res) => {
console.log(req.params)
console.log(req.query)
console.log(req.body)
})
The Response Object
The response object is the information that the server will send back to the client making the request.
When sending a response object, it is important to include status codes.
Status codes are a standardized short-hand syntax for relaying information about requests.
app.get('/api/users', (req, res) => {
res.status(200).send(users)
})
Available status codes here:
Node 1
By matias_perez
Node 1
Servers and Node.js with Express
- 323