Container/Presentational
Pattern in React
- Richa Pujara
Intern at Back2.Dev
- Design Patterns in React
- Container/Presentational Pattern
- Benefits
- Demonstation
- My experience using it
Introduction
Container/Presentational Pattern
Container Component
Data Fetching/
other Application logic
Presentational
UI Component
Presentational
UI Component
View
Benefits
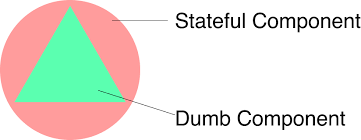
1. Separation of Concerns
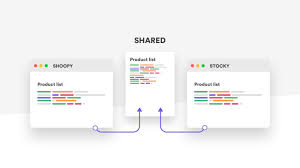
2: Sharing the states among components
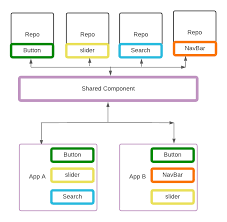
3: Reusing components, less dev time, consistency between views/layouts.
A way to do it..
// dogImages.js
import React from'react';
export default function () {
const [dogs, setDogs] = React.useState([])
React.useEffect(()=>{
fetch("https://dog.ceo/api/breed/labrador/images/random/6")
.then(res => res.json())
.then(jsonRes => setDogs(jsonRes.message));
},[])
return dogs.map((dog, i) => <img src={dog} key={i} alt="Dog" />
}
# PRESENTING CODE
A better way to do it..
// dogsContainer.js -- Container Component
import React from 'react';
import DogImages from './dogImages'
export default function DogImagesContainer() {
const [dogs, setDogs] = React.useState([])
React.useEffect(()=> {
fetch("https://dog.ceo/api/breed/labrador/images/random/6")
.then(res => res.json())
.then(jsonRes => setDogs(jsonRes.message))
},[])
return <DogImages dogs={dogs} />;
}
# PRESENTING CODE
Presentational Component
// dogIMagesDisplay.js -- Presentational COmponent
import React from "react";
export default function DogImages({ dogs }) {
return dogs.map((dog, i) => <img src={dog} key={i} alt="Dog" />);
}
# PRESENTING CODE
How did we use it?
Easier testing of Renderer components with Storybook
My Experience Using It
References:
- https://www.patterns.dev/posts/presentational-container-pattern/
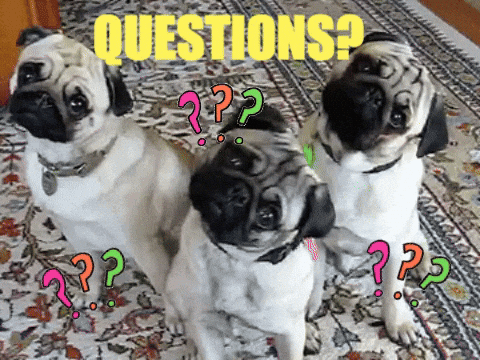
Thank You...
Introduction
- One of the most common React Design Pattern
- Used to enforce Separation of Concerns
- Separation of View from the Application Logic.
// This slide uses Auto-Animate to animate between
// two different code blocks
const distanceBetween = ( p1, p2 ) => {
// TODO
}
distanceBetween([10,10], [50,50])
# PRESENTING CODE
Text
// This slide uses Auto-Animate to animate between
// two different code blocks
const distanceBetween = ( p1, p2 ) => {
// TODO
}
distanceBetween([10,10], [50,50])
# PRESENTING CODE
Code Transitions
Container Presentational Component Pattern
What is Container-Presenter Pattern?
1. What
2. Why
Text
3. How
Text
# CHAPTER 2
// This slide uses Auto-Animate to animate between
// two different code blocks
const distanceBetween = ( p1, p2 ) => {
// TODO
}
distanceBetween([10,10], [50,50])
# PRESENTING CODE
Code Transitions
Copy of Container/Presentational Pattern in React
By Mike King
Copy of Container/Presentational Pattern in React
- 20