BBC Micro:Bit
Lesson Objectives
To be able to:
- Understand how a while loop is used to repeat code forever.
- Understand the use of indentation in code
- Use IF statements in code
While Loops
What process do you repeat when you walk?
One foot in-front of the other
left foot up
left foot forwards
left foot down
right foot up
right foot forwards
right foot down
left foot up
left foot forwards
left foot down
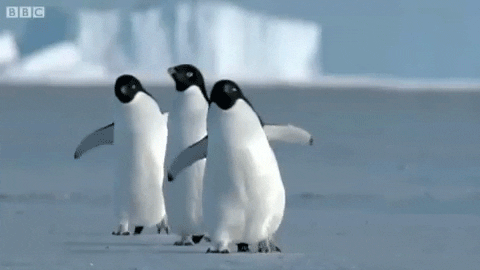
Do we need to repeat every single instruction over and over and over again?
While Loops
left foot up
left foot forwards
left foot down
right foot up
right foot forwards
right foot down
left foot up
left foot forwards
left foot down
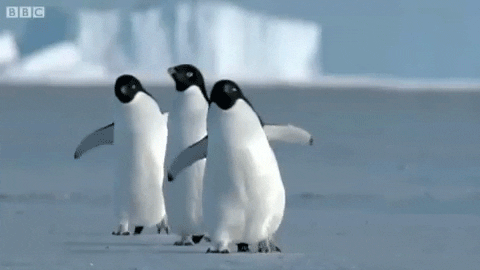
while walking:
left foot up
left foot forwards
left foot down
right foot up
right foot forwards
right foot down
Instead of saying left and right and left and right ect., we can use a loop and only mention each foots process once

While Loops
How many times is this code going to show the happy face?
from microbit import *
display.show(Image.HAPPY)
sleep(1000)
display.show(Image.MEH)
sleep(1500)
display.show(Image.SAD)
sleep(1000)
While Loops
How many times will a happy face appear in this code?
from microbit import *
while True:
display.show(Image.HAPPY)
sleep(1000)
display.show(Image.MEH)
sleep(1500)
display.show(Image.SAD)
sleep(1000)
While Loops
How does the program know what code to repeat?
from microbit import *
while True:
display.show(Image.HAPPY)
sleep(1000)
display.show(Image.MEH)
sleep(1500)
display.show(Image.SAD)
sleep(1000)
display.show(Image.ASLEEP)
While Loops
from microbit import *
while True:
display.show(Image.HAPPY)
sleep(1000)
display.show(Image.MEH)
sleep(1500)
display.show(Image.SAD)
sleep(1000)
display.show(Image.ASLEEP)
When you write line of code with a colon, there MUST be at least one line of code beneath with an indent.
IF Statement
from microbit import *
while True:
if button_a.is_pressed():
display.show(Image.HAPPY)
elif button_b.is_pressed():
display.show(Image.MEH)
else:
display.show(Image.SAD)
An IF Statement will check the condition of something before making a decision on what to do next.
When you first press run, what face will appear on your Micro:Bit?
IF Statement
from microbit import *
while True:
if button_a.is_pressed():
display.show(Image.HAPPY)
elif button_b.is_pressed():
display.show(Image.MEH)
else:
display.show(Image.SAD)
An IF Statement will check the condition of something before making a decision on what to do next.
If you press button A, what face will appear?
IF Statement
from microbit import *
while True:
if button_a.is_pressed():
display.show(Image.HAPPY)
elif button_b.is_pressed():
display.show(Image.MEH)
else:
display.show(Image.SAD)
An IF Statement will check the condition of something before making a decision on what to do next.
If you press button A and B, what face will appear?
IF Statement
from microbit import *
while True:
if button_a.is_pressed():
display.show(Image.HAPPY)
elif button_b.is_pressed():
display.show(Image.MEH)
elif button_a.is_pressed() and button_b.is_pressed():
display.show(Image.PACMAN)
else:
display.show(Image.SAD)
If you press button A and B, what face will appear?
Continue where you left off on Grok Learning
Activity
Complete the quiz in your Google Classroom
Plenary
Micro:Bit L3 - Student
By CJackson
Micro:Bit L3 - Student
- 93