Year 8 Robotics
Lesson 2 - Powering the Motor
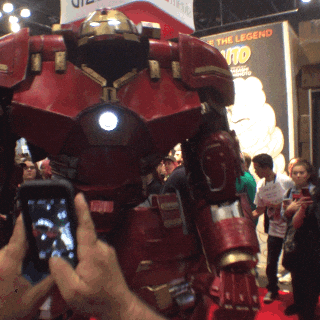
Learning Objectives
To be able to:
- Program the MiniBit to move around
- Automate moving straight and turning
Which Pins do we need?
Last week we noted that Pin 13 controlled the neopixel LED's.
Have another look at the pin chart to see which pins control the left and right motors.

Direction of the Motors
The motors have got 2 programmable pins per side, for a total of 4 pins.
These pins are pin 8 and pin 14.

Left
Dir Pin 8
Right
Dir Pin 14
Direction of the Motors
When we program the motors we use a 1 and a 0 to tell which way to rotate the motors.
0 is for forwards
1 is for reverse

Dir Pin 8
Dir Pin 14
0
0
1
1
Direction of the Motors
Which pins and values would we set for moving the vehicle in reverse?

Which pins and values would we set for moving the vehicle forwards?
Speed of the Motors
What about pin 12 and pin 16?

Now that we have told the pins which direction to go in, we have to tell it how quickly to rotate.
Left Motor
Pin 12
0 - 1023
The speed can be programmed within a range of numbers, 0 - 1023.
1023 is the highest if we are going forwards, 0 is the highest if we are in reverse.
Right Motor
Pin 16
0 - 1023
Predict

Pin 8 | 0 |
Pin 14 | 0 |
Pin 12 | 1023 |
Pin 16 | 1023 |
Pin 8 | 1 |
Pin 14 | 0 |
Pin 12 | 500 |
Pin 16 | 500 |
Pin 8 | 1 |
Pin 14 | 1 |
Pin 12 | 10 |
Pin 16 | 10 |
Pin 8 | 0 |
Pin 14 | 0 |
Pin 12 | 300 |
Pin 16 | 700 |
Look at the following algorithms. Can you predict the behaviours of the robot in each?
The Code
Your speed, pins 12 and 16, uses write_analog.
Your direction, pins 8 and 14, uses write_digital.
from microbit import *
# Left Motor
pin12.write_analog(600)
pin8.write_digital(0)
# Right Motor
pin16.write_analog(600)
pin14.write_digital(0)
Predict Code
pin12.write_analog(600)
pin8.write_digital(0)
pin16.write_analog(600)
pin14.write_digital(0)
pin12.write_analog(0)
pin16.write_analog(0)
How will the MiniBit robot behave?
Predict Code
pin12.write_analog(600)
pin8.write_digital(0)
pin16.write_analog(600)
pin14.write_digital(0)
sleep(2000)
pin12.write_analog(0)
pin16.write_analog(0)
How will the MiniBit robot behave?
Predict Code
How will the MiniBit robot behave?
pin12.write_analog(600)
pin8.write_digital(0)
pin16.write_analog(600)
pin14.write_digital(0)
sleep(2000)
pin12.write_analog(600)
pin8.write_digital(1)
pin16.write_analog(600)
pin14.write_digital(1)
sleep(2000)
pin12.write_analog(600)
pin8.write_digital(0)
pin16.write_analog(600)
pin14.write_digital(0)
Task 1 - Moving forwards
from microbit import *
# Write your code here...
pin12.write_analog(1023)
pin8.write_digital(0)
pin16.write_analog(1023)
pin14.write_digital(0)
- Write a program to make the robot
- move forwards for 3.5 seconds
- then stop
HINT:
Task 2 - Back and Forth
- Write a program to make the robot
- move forwards for 2 seconds
- turn around (180° turn) for half a second
- repeat the above steps
from microbit import *
while True:
HINT:
Task 3 - Square Dance
- Write a program to make the robot
- turn in a square pattern

Extension Task:
Use a FOR loop to reduce the amount of coding.
HINT:
from microbit import *
#Forward
pin12.write_analog(600)
pin8.write_digital(0)
pin16.write_analog(600)
pin14.write_digital(0)
sleep(2000)
#Stop Forward
pin16.write_analog(0)
pin14.write_digital(0)
sleep(250)
#Turn right
pin12.write_analog(600)
pin8.write_digital(0)
sleep(250)
#Forward
pin12.write_analog(600)
pin8.write_digital(0)
pin16.write_analog(600)
pin14.write_digital(0)
sleep(2000)
Scroll down the code
Robotics Lesson 2 - Student
By CJackson
Robotics Lesson 2 - Student
- 172