Rails Decal
Lecture 4 - Models & Migrations
Charles X.
Administrivia
- < 1 hrs
- 1 - 3 hrs
- 3 - 5 hrs
- 5+ hrs
Homework 2 - How long?
Administrivia
Starting from HW2, homework due on the following Fridays
Today:
- Database Introduction
- What are Models?
- Creating Models
- Migrations
- Querying the database
How do we persist data?
DATABASES!
What are databases?

Text
WAT
What are databases?
- Used to store/retrieve information
- Database is composed of many tables
- Each table corresponds to a Model (explain later)
- Each table has its own set of attributes in columns
- For more in-depth: CS186

Database for sample_app
Databases
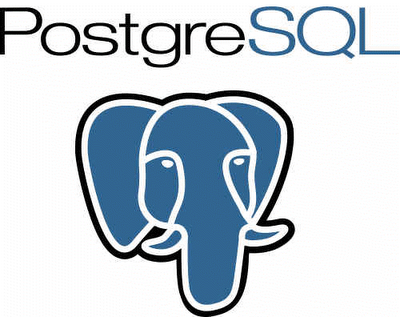


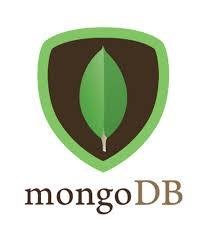
Our database of choice

MVC

Models in Rails!
- Models are built in class frameworks
- Have methods that you don't need to define
- Interact with the database automatically for you***
- They do a bunch of other cool things like relationships and validations that will be covered later
- They can do all of that because of this:
class <classname> < ActiveRecord::Base
Why models are important
- Models are the core of Rails apps
- Represents all the information you need
- This allows controllers to work with the model
- Represents all the information you need
Future Lectures:
- Validations
- Relationships
- Add more abilities (methods)
- and more!
Creating Models
rails generate model model_name column_name:column_type
Example
rails generate model User name:string age:integer
Migrations
- Migrations change the structure of the database
- Allows you to add/remove/rename columns
- Can also create/delete tables
rails generate migration migration_name
Update your database!
rake db:migrate
NEVER CHANGE MIGRATED MIGRATIONS
Types of migrations
Adding columns
Assume we have table called User
add_column :users, :nickname, :string
Removing columns
remove_column :users, :nickname
Renaming columns
rename_column :users, :name, :nickname
Querying our database
Model.find id
Model.find_by attribute: something
Model.where attribute: something
Model.first/last
Model.all
So what can I do with a model?
-
CRUD
- Create
- Read (Show)
- Update (Edit)
- Destroy
CRUD
Today we'll focus on
- Create
CRUD - Create
- Usually done through forms (coming soon!)
- A person on a Rails app will pass in values like
- Name: Howard
- Email: swag@swag.com
- Age: 9001
- And the controller will create a Model Instance
- And add that to the database


Creating our User
Making users locally - Loads our app
rails console OR rails c
Making a new User
user = User.new
user.save!
user = User.create name: "hello", age: 5
`
user.name = "Charles"
OR
Live Demo
- Create user model
- Run migrations to have these work
- Utilize Rails console
Thats cool and all but..
- How do we let the users do it instead of running rails c?
- How to stop erroneous input?
Next time...
- RUD - (Read, Update, Destroy)
- Forms
- Validations
Homework time!
Fork: https://github.com/rails-decal/sp15-hw3
Check in code
makingUsers
Spring 2015 - Week 4: Models & Migrations
By Rails Decal
Spring 2015 - Week 4: Models & Migrations
- 1,128