R
E
A
C
T
O

xamples
epeat
ode
pproach
ptimize
est
Spiral Jetty
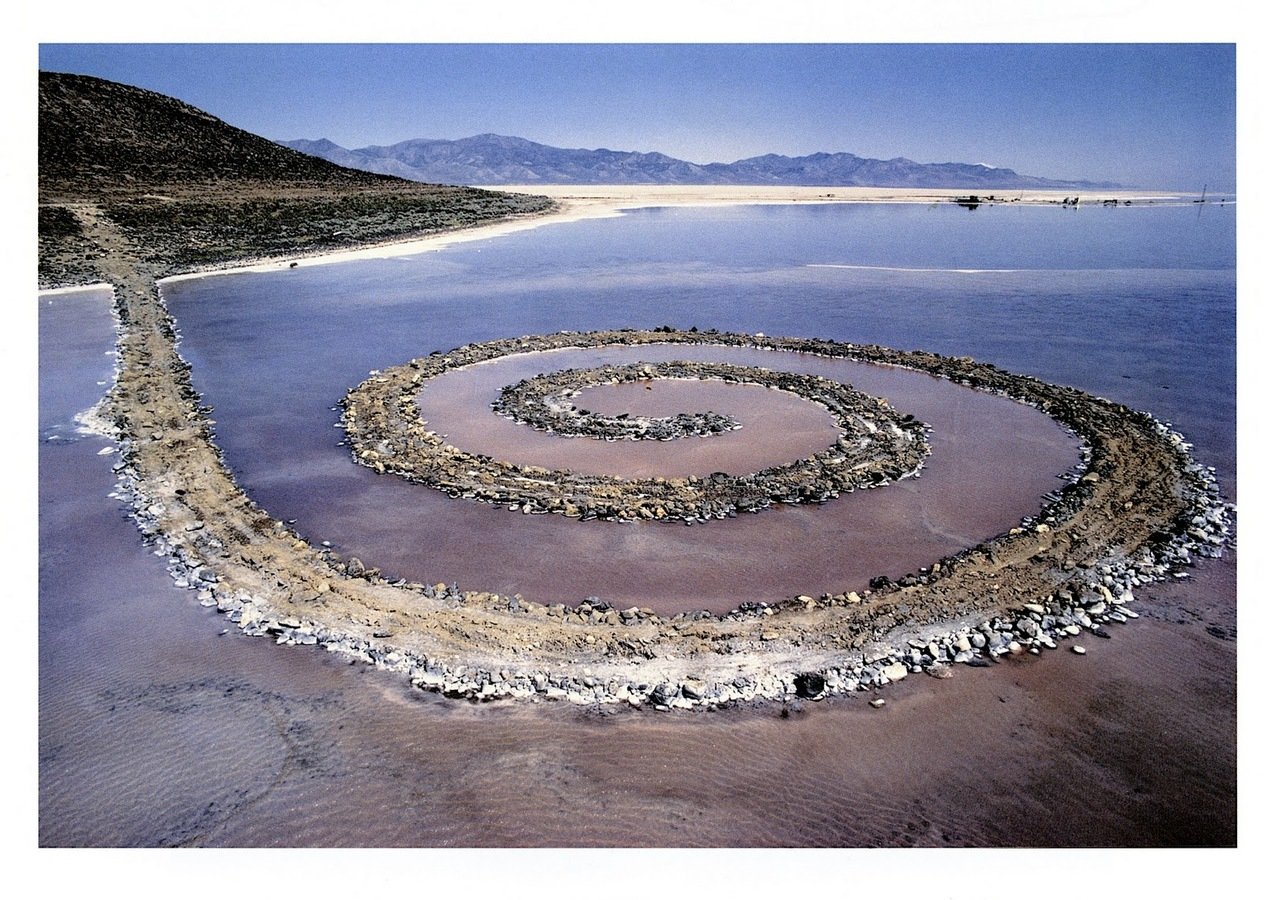

The Question
Write a function that will generate a spiral
of the form below:
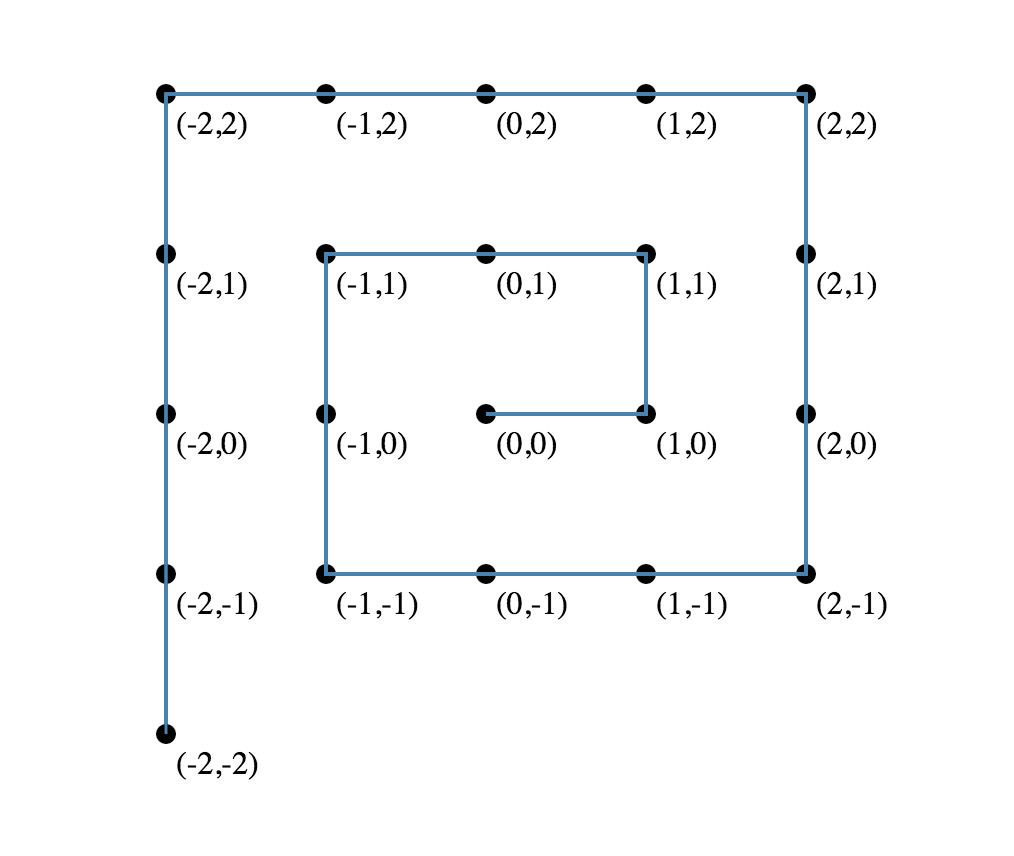
resultArray = [[0,0],[1,0],[1,1],[0,1],[-1,1],[-1,0],...,[-2,-1],[-2,-2]]
var spiral = function(n){
var direction;
x=0;
y=0;
resultArray = [[0,0]]
for (var k=1; k < n; k++){
direction = k%2;
if (direction === 1){
// Move to the right then move up
}
if (direction === 0){
// Move to the left then move down
}
}
return resultArray;
}
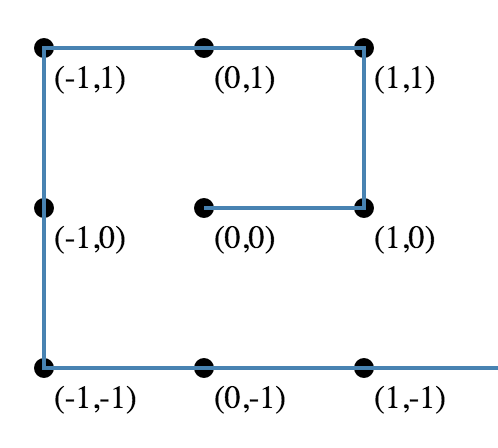
k = 1 : [[0,0],[1,0],[1,1]]
k = 2 : [[0,0],[1,0],[1,1], ]
[0,1],[-1,1],[-1,0],[-1,-1]
k=1
k=1
k=2
k=2
|
|
|
|
|
|
|
Approach
- for each k, you take
k steps in both the x
and y direction
- alternate between
+ and - direction
for (var k=1; k < n; k++){
direction = k%2;
if (direction === 1){
var i = 0;
var j = 0;
while(i < k){
x++;
resultArray.push([x,y]);
i++
}
while(j < k){
y++;
resultArray.push([x,y])
j++
}
}
if (direction === 0){
var i = 0;
var j = 0;
while (i < k){
x--;
resultArray.push([x,y]);
i++;
}
while (j < k){
y--;
resultArray.push([x,y]);
j++
}
}
}
- x and y represent
the coordinates
- i and j control the
number of steps taken
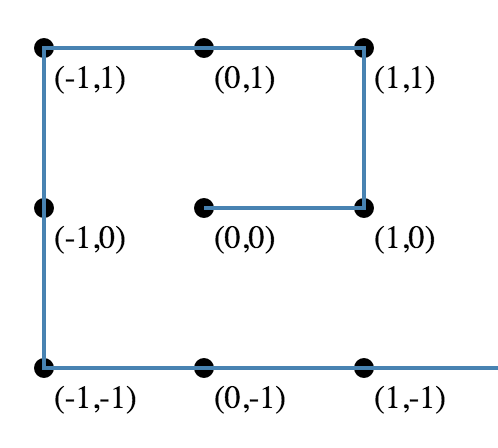
i = 0
i = 0
i = 1
j = 0
j = 0
j = 1
i = 1
i = 0
i = 2
Approach
Full Solution
Another Solution
'use strict';
var spiral = function(maxLength){
var xPos=0;
var yPos=0;
var resultArray = [[0,0]];
//each large iteration creates an 'L' of the spiral
for (let thisLength = 1; thisLength < maxLength; thisLength++){
//control ++ or -- vertically and horizontally
var goPositive = thisLength % 2;
//iterate horizontally left or right
for (let xIterator = 0; xIterator < thisLength; xIterator++){
goPositive ? xPos++ : xPos--;
//push new coordinates
resultArray.push([xPos,yPos])
}
//iterate vertically up or down
for (let yIterator = 0; yIterator < thisLength; yIterator++){
goPositive ? yPos++ : yPos--;
//push new coordinates
resultArray.push([xPos,yPos])
}
}
return resultArray;
}
Another Solution
function tropicalSpiral(n) {
let x = 0,
y = 0,
result = [[0, 0]],
z = n * -1,
k = 1;
while (x !== z && y !== z) {
// increment x positive
for (let i = 0; i < k; i++) result.push([++x, y]);
// increment y positive
for (let j = 0; j < k; j++) result.push([x, ++y]);
// increment our 'k' threshold
k++;
// decrement x negative
for (let i = 0; i < k; i++) result.push([--x, y]);
// decrement y negative
for (let j = 0; j < k; j++) result.push([x, --y]);
}
return result;
}
tropicalSpiral(1);
Copy of Copy of Spiral Jetty
By Tom Kelly
Copy of Copy of Spiral Jetty
Technical interview problem on Robert Smithson
- 1,462