Immutable Flux
Adventures in
Adam MIskiewicz
Interactive Director and Founder
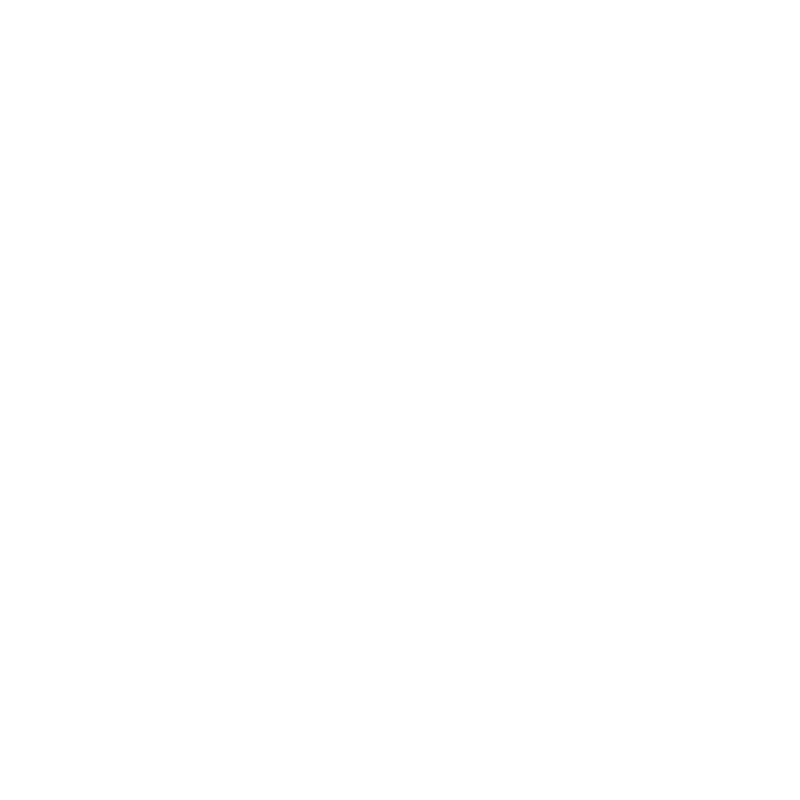
IMMUTABILITY
REACT.JS
FLUX
===
+
+
Immutability...????
immutable object
an object that cannot be modified after it’s initial creation.
noun
STRINGS
NUMBERS
BOOLEANS
NULL
UNDEFINED
"a string" = "another string"
2 = 3
true = false
null = "i'm not null"
undefined = null
"a really cool string" === "a really cool string"
2 === 2
2 = 3 ?
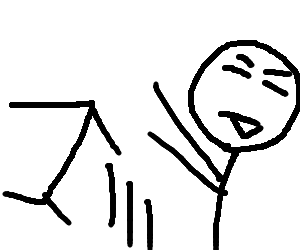
What about
Objects and Arrays?
Object.freeze()
Vector.prototype.push = function(newEl) {
var newVector = deepCopy(this) //step 1
newVector.push(newEl) //step 2
return newVector //step 3
}
That must be slowwwwwwwwwwwwwwww
STRUCTURAL SHARING
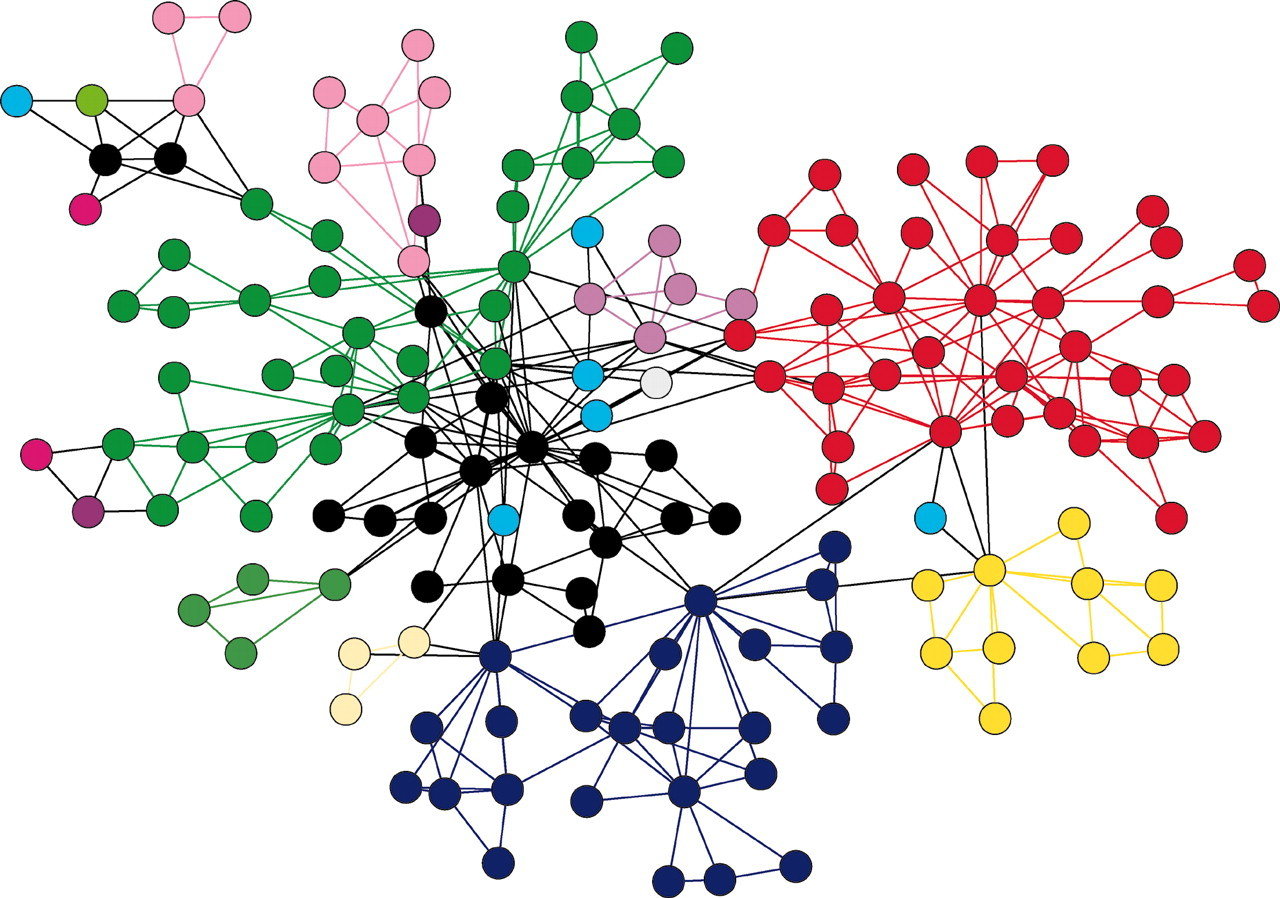
DIRECTED
ACYCLIC
GRAPH
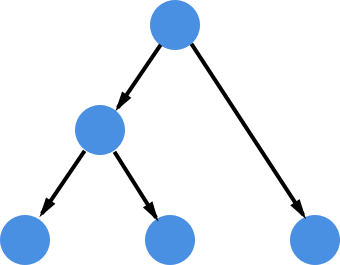
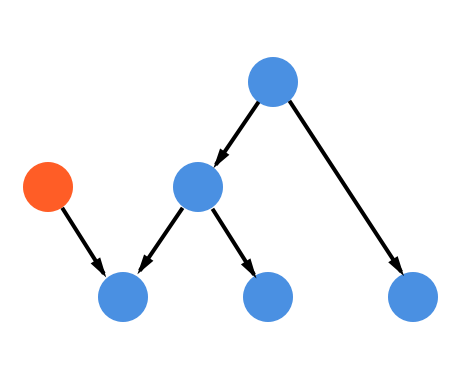
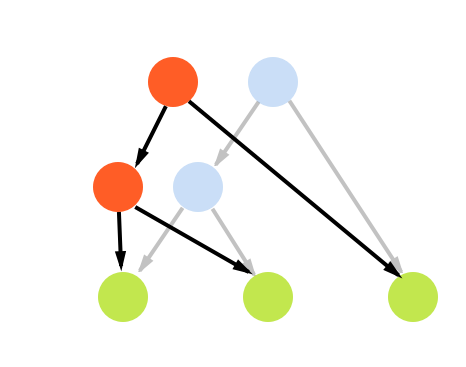
Ain't nobody got time for that.
INTERFACE
vs.
IMPLEMENTATION
reTrieve
TRIE
BITMAPPED VecTor Trie
HASH ARRAY MAPPED TRIE
List
HashMap
WHY?
Concurrency?
But JS is single threaded...
Less complex
Mutable state is the root of all evil.
- Pete Hunt
var a = [0, 1, 2, 3, 4]
var b = a
a.push(5)
console.log(a) // [0, 1, 2, 3, 4, 5]
console.log(b) // [0, 1, 2, 3, 4, 5]
function someFunc(anotherFunc) {
var anObject = {
a: 1,
b: 2
}
anotherFunc(anObject)
console.log(anObject) // I have no idea.
}
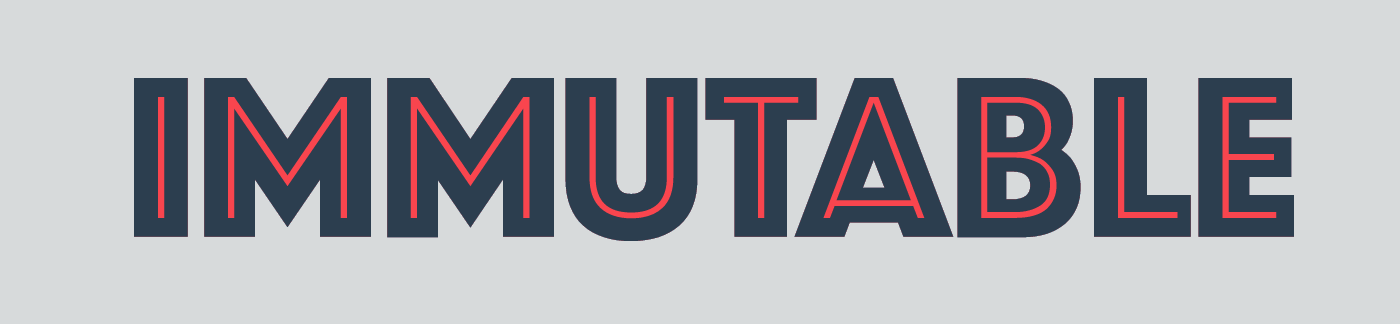
import {List, Map} from "immutable"
var list1 = List([0, 1, 2, 3, 4])
var list2 = list1.push(5)
console.log(list1 === list2) // false
var map1 = Map({ a: 1, b: 2 })
var map2 = map1.set("c", 3)
console.log(map1 === map2) // false
console.log(map1) // { a: 1, b: 2 }
console.log(map2) // { a: 1, b: 2, c: 3 }

shouldComponentUpdate()
Fast reconciliation
PureRenderMixin
return !shallowEqual(this.props, nextProps) ||
!shallowEqual(this.state, nextState);
===
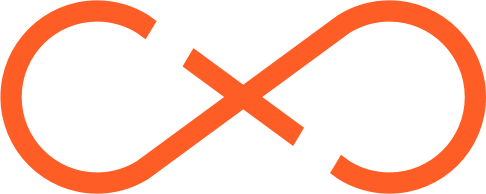

ONE POSSIBILITY...
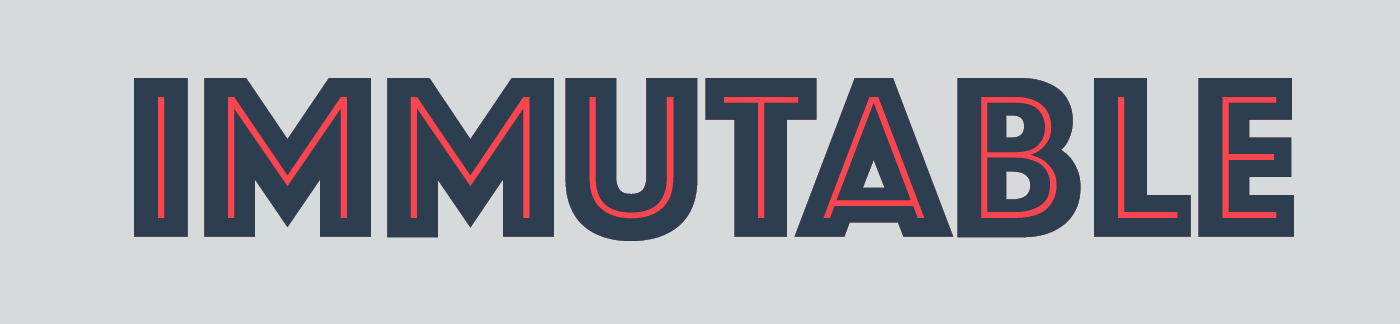
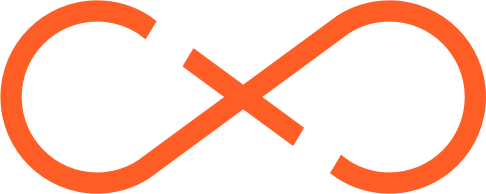
+
DATA INSIDE STORES BECOMES IMMUTABLE
SIMPLE.
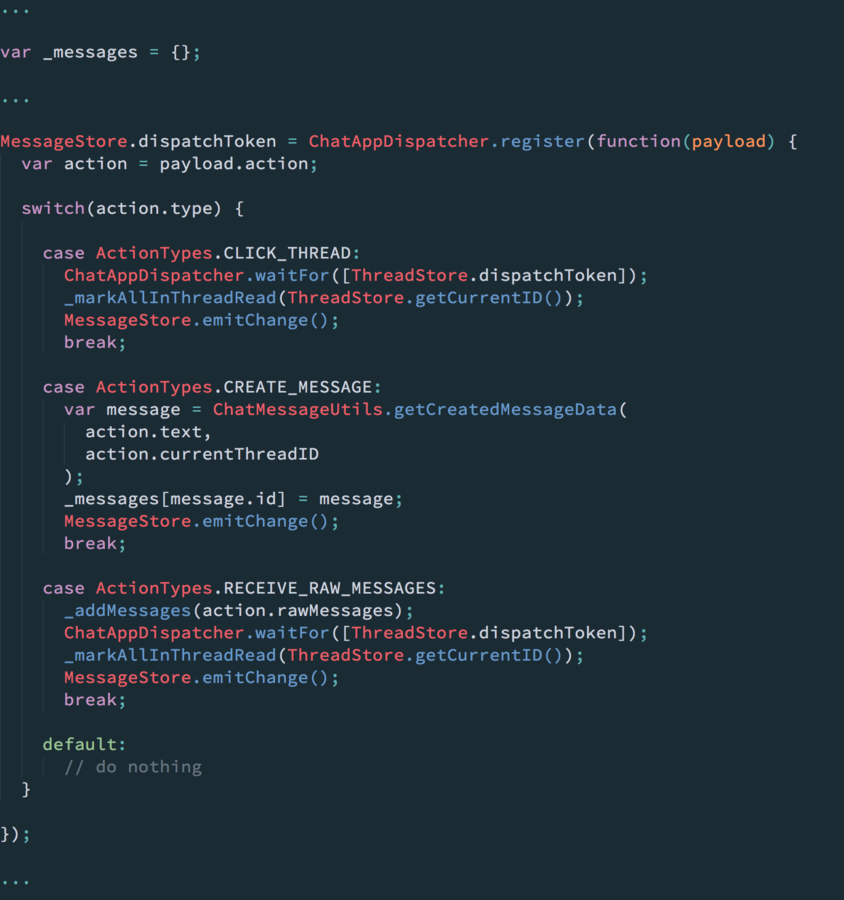

PureRenderMixin
dats it
LETS TAKE IT FURTHER....
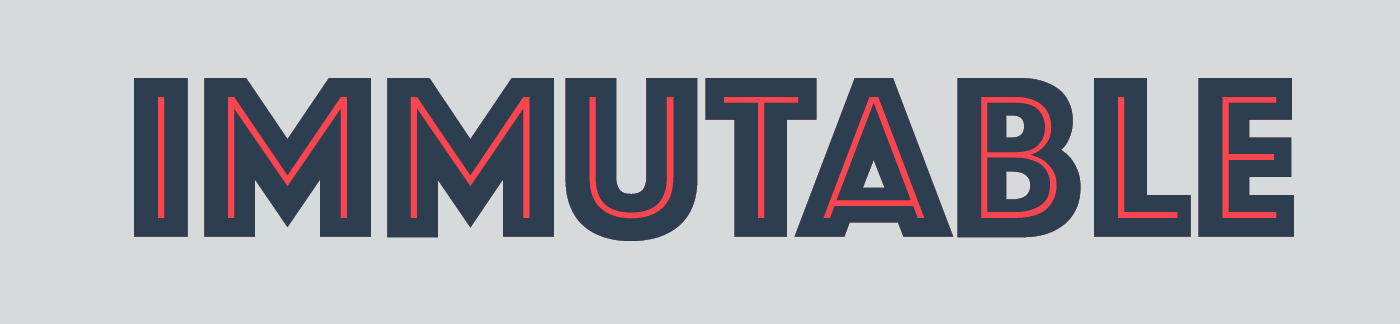
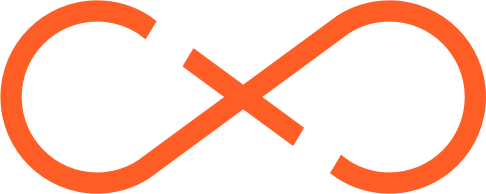
+

is fast
but it could be faster...
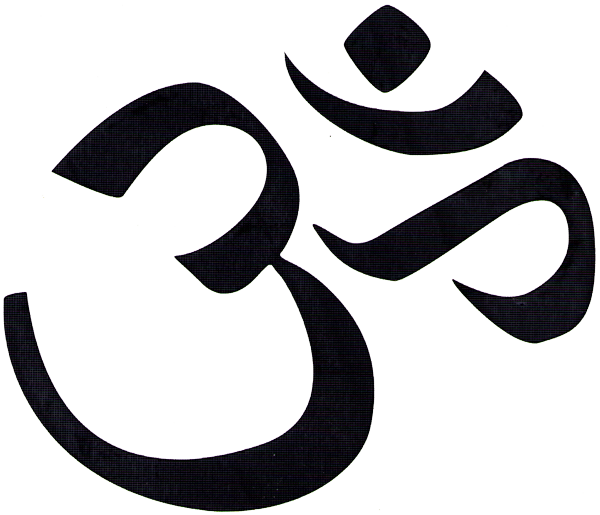
CURSORS
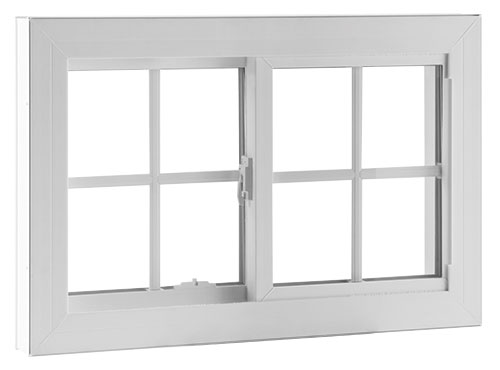
IMMUTABLE CURSORS INTO GLOBAL APP STATE
ALLOWS FOR INCREDIBLY EFFICIENT
shouldComponentUpdate()
RE-RENDER ENTIRE APP
every time the app state changes
(((((((((add 2 2)))))))))
* not actually valid Clojure
+
IMMSTRUCT
But, I want my flux back...
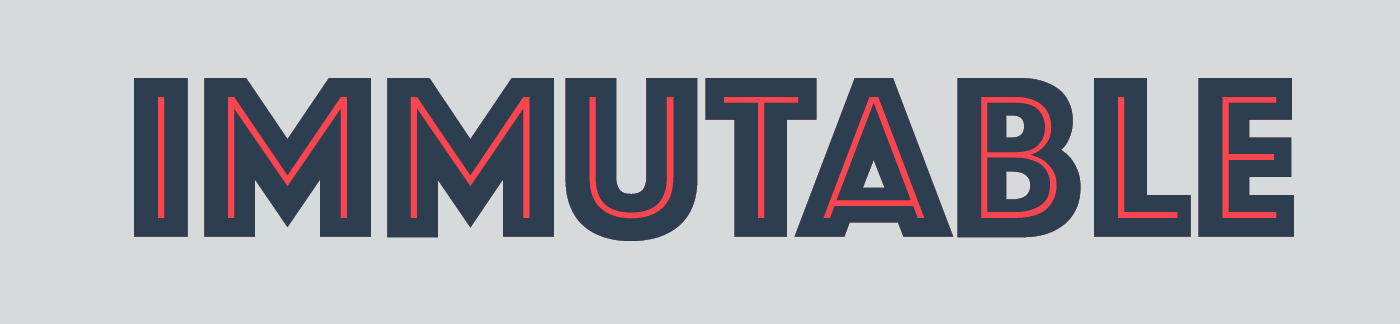
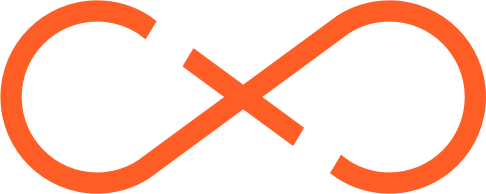
+
Do we even need Flux with all this coolness?
I think we do...

AWESOME
what do stores do?
Sure, they store data
But they also modify and prepare it for the UI
ACTION CREATOR MODEL
IS SUPER POWERFUL AS WELL
FLUX IS A FANTASTIC MODEL FOR ARCHITECTING APPLICATIONS
LETS NOT LOSE FLUX, EVEN WHEN THINKING IN TERMS OF A GLOBAL APP STATE

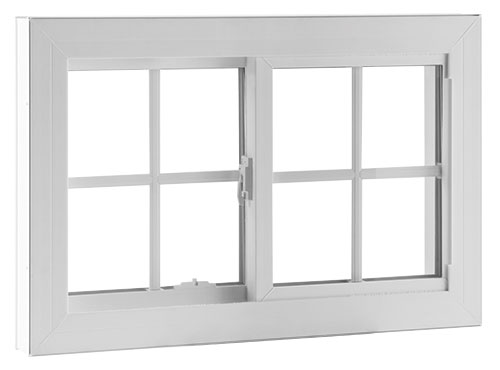
as
INTO THE GLOBAL APP STATE
STORES REGISTER THEMSELVES WITH GLOBAL STATE STRUCTURE
STORES STILL RESPOND TO DISPATCHED ACTIONS
THEY'RE JUST UPDATING THE GLOBAL APP STATE, INSTEAD OF THEiR OWN STATE
THE OTHER PARTS OF FLUX
WORK NORMALLY
WHen app state changes...
Entire app re-renders...but efficiently
REFERENCE CURSORS
huh?
FOR UPDATING COMPONENTS
Deeeeeeep in the component tree
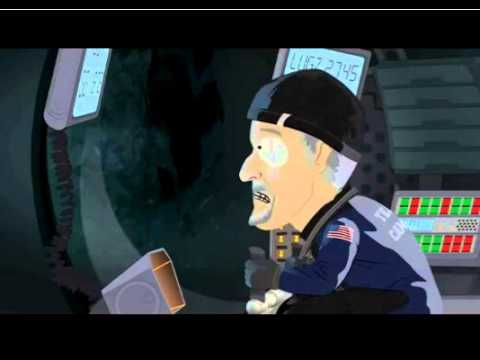
*with Randy Newman
WHY?
DO EVERYTHING YOU DO NOW
GET WHAT WE JUST WATCHED FOR (BASICALLY) FREE
UNDO + REDO
Makes server-side flux TRivial
flux.dehydrate() //outputs global app state object
flux.rehydrate(STATE) // here it is on the client!
THERE ARE LIBRARIES THAT DO THIS NOW
But adding immutable on top allows for efficient
shouldComponentUpdate()
transit.js
THANKS!
Adventures in Immutable Flux
By Adam Miskiewicz
Adventures in Immutable Flux
- 1,022