Python 101
Python Pune
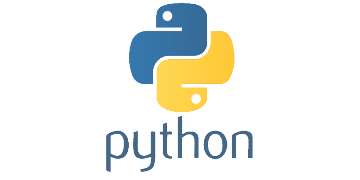
Time to Brush up your Basics &
Learn Python 3 skills
Using Interpreter.
>>> print("Hello World")
Hello World
Comments
>>> # This is a comment
>>> # print("Hello World!")
...
>>>
Variables and Datatypes
Read User Input.
>>> number = int(input("Enter a number: "))
Enter a number: 5
>>> print(number)
5
>>> name = input("Enter your name: ")
Enter your name: Aditya
>>> print(name)
'Aditya'
Decision Making and Flow Control
If statement
if expression:
do this
elif expression:
do this
else:
do this
for loop
for var in sequence:
statement
List
supports multiple data types
>>> list = [1, 2, "hello", 1.0]
>>> list
[1, 2, 'hello', 1.0]
Lists Comprehensions
>>> a = [1, 2, 3]
>>> [x ** 2 for x in a]
[1, 4, 9]
Tuple
>>> tuple = ('Fedora', 'Debian', 10, 12)
>>> tuple
('Fedora', 'Debian', 10, 12)
Dictionary
>>> data = {'os':'Fedora', 'kart':'Debian', 'Jace':'Mac'}
>>> data
{'os': 'Fedora', 'Jace': 'Mac', 'kart': 'Debian'}
Set
>>> a = set('abcthabcjwethddda')
>>> a
{'a', 'c', 'b', 'e', 'd', 'h', 'j', 't', 'w'}
Function
>>> def function(params):
... statement
>>> def sum(a, b):
... return a + b
...
>>> res = sum(2, 3)
>>> res
5
Lambda Expression
>>> sum = lambda x, y: x+y
>>> sum(2, 3)
5
Class
>>> class ClassName:
... """ Optional class documentation string. """
... class_suite
>>>
File Handling.
>>> f = open("abc.txt", "w") # Open file in written mode.
>>> f.write("Programming with Python id Fun! ")
>>> f.close() # Closed opened file.
>>>
Questions?
Hack and Learn
By Aditya Ramteke
Hack and Learn
Python basics to advance.
- 174