Object Tracking
-Akshat
Object tracking is the process of locating a moving object (or multiple objects) over time using a camera. It has a variety of uses, some of which are: human-computer interaction, security and surveillance, augmented reality, traffic control, medical imaging and video editing.
Akshat

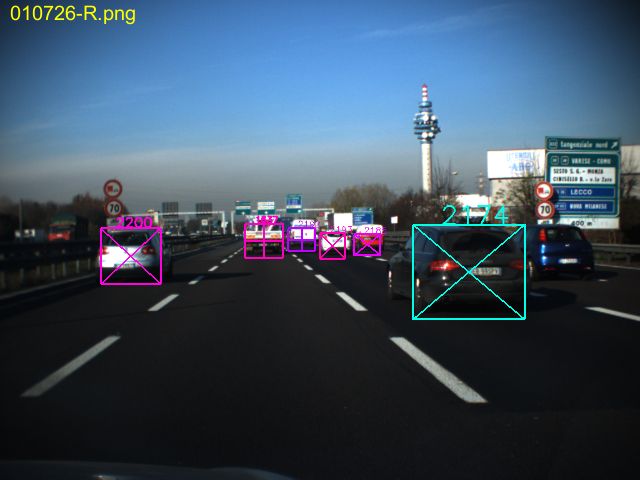
Some Day2Day Examples
Akshat
It seems interesting
?
Akshat
so for next 80 mins..
a short introduction on video capture
describe the object to be tracked
contour extraction
sketch the frame
Akshat


Some
Results
Akshat
before we do anything
import cv2
import numpy as np
Akshat
let' s capture a video
import cv2
import numpy as np
cap = cv2.VideoCapture(0)
while True:
# Let's capture/read the frames for the video
_, frame = cap.read()
# This frame captured is flipped, Lets's get the mirror effect
frame = cv2.flip(frame, 1)
# Any operations on the frame captured will be processed in the operate(frame) method
final_frame = operate(frame)
# Showing the captured frame
cv2.imshow('garrix', frame)
cv2.imshow('martin', final_frame)
# Continuous, Large amount of frames produce a video
# waitkey(value), value is the ammount of millisecs a frame must be displayed
# 0xff represents the ASCII value for the key, 27 is for ESC
# waitKey is necessary to show a frame
if cv2.waitKey(1) & 0xff == 27:
break
cap.release()
cv2.destroyAllWindows()
Akshat
let' s describe the object
# Info : ZOOM in the frame to get the RGB value of the corresponding pixel
# The range of color for the object to be detected in HSV
hsv_supremum = np.array([172, 221, 255])
hsv_infinum = np.array([150, 40, 130])
def operate(frame):
frame_copy = frame.copy()
frame_ret = frame.copy()
# Converting the frame from BGR format to HSV format
frame_copy = cv2.cvtColor(frame, cv2.COLOR_BGR2HSV)
# Thresholding the frame to get our object(color) tracked
mask = cv2.inRange(frame_copy, hsv_infinum, hsv_supremum)
mask = cv2.medianBlur(mask, 5)
mask = cv2.erode(mask, None, iterations=2)
mask = cv2.dilate(mask, None, iterations=2)
#cv2.imshow('mask', mask)
# Obtaining the binary Image for the corresponding mask
res = cv2.bitwise_and(frame_ret,frame_ret, mask= mask)
#cv2.imshow('res', res)
# The result can be improved by smoothening the frame
# To be contd...
Akshat
my object
Akshat







object configuration

Akshat
object configuration
hsv_supremum = np.array([172, 221, 255])
hsv_infinum = np.array([150, 40, 130])
def operate(frame):
# Some code above
# Converting the frame from BGR format to HSV format, Hue Saturation Value GOOGle for more
frame_copy = cv2.cvtColor(frame, cv2.COLOR_BGR2HSV)
# Thresholding the frame to get our object(color) tracked
mask = cv2.inRange(frame_copy, hsv_infinum, hsv_supremum)
# Some code below
Akshat
object configuration
# In the operate function
mask = cv2.medianBlur(mask, 5)
mask = cv2.erode(mask, None, iterations=2)
mask = cv2.dilate(mask, None, iterations=2)
# The result can be further improved by smoothening the frame
# Issues:
# Lightning
# Variable Contour
operations to enhance the object
Akshat
dilation
Akshat

source UDACITY
erosion
Akshat

source UDACITY

Results After Dilation & Erosion in the binary Image
Akshat
contour extraction
# In the same operate function
mask_copy = mask.copy()
cnts = cv2.findContours(mask.copy(), cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)[-2]
center = None
if len(cnts) > 0:
c = max(cnts, key = cv2.contourArea)
((x, y), radius) = cv2.minEnclosingCircle(c)
M = cv2.moments(c)
center = (int(M["m10"] / M["m00"]), int(M["m01"] / M["m00"]))
if radius > 10:
cv2.circle(frame_ret, (int(x), int(y)), int(radius), (0, 150, 255), 2)
cv2.circle(frame_ret, center, 5, (0, 0, 255), -1)
operations to extract the contour
Akshat

Object Contour Extracted
Object Mask Extracted
Video Capture
Akshat
sketch the frame
import cv2
import numpy as np
from collections import deque # <--
pts = deque() # <--
cap = cv2.VideoCapture(0)
# In the operate function
pts.appendleft(center)
#print(pts[0])
for i in range(1 , len(pts)):
if pts[i-1] is None or pts[i] is None:
continue
thickness = 6
cv2.line(frame_ret, pts[i-1], pts[i], (0, 0, 255), thickness)
return frame_ret
operations to sketch on the frame
Akshat

Sample Sketch
Akshat

Sample Sketch
Akshat
Code
Implementation
Lets Begin
[Quest]ions
?
Thank you
Akshat Sharma
Sophomore
Phoenix | CV Last Lecture
By Akshat Sharma
Phoenix | CV Last Lecture
Project Based session on Computer Vision, covering Object Tracking & Webcam Drawing.
- 388