CSS


Cascading Style Sheets
Styling our webpages
The Main Rule
selector {
property: value;
}
We select element and then apply some styles to that.
Ex) Select h1
set h1 color to red
Some Examples
p {
color: red;
font-size: 18px;
}
img {
border-width: 5px;
border-color: purple;
}
make all p's red and 18px
give all img's a 5px purple border
Where do we write our styles?
<h1 style="color: red;">blah blah blah</h1>
<p style="color: blue;">blah blah blah</p>
<head>
<title>Document</title>
<style>
h1 {
color: red;
}
p {
color: blue;
}
</style>
</head>
inline
Style Tag
Where do we write our styles?
<h1 style="color: red;">blah blah blah</h1>
<p style="color: blue;">blah blah blah</p>
<head>
<title>Document</title>
<style>
h1 {
color: red;
}
p {
color: blue;
}
</style>
</head>
inline
Style Tag
Write our css in a separate .css file
<head>
<title>Document</title>
<link rel="stylesheet" href="style.css" />
</head>
Link Tag
Colors In CSS 🎨
What should we do? 🤔
Built in colors
<h1>I'm a Red H1</h1>
<h2>I'm a Blue H2</h2>
<h3>I'm a Purple H3</h3>
h1 {
color: red;
}
h2 {
color: blue;
}
h3 {
color: purple;
}

style.css
index.html
Hexadecimal
<h1>I'm a Red H1</h1>
<h2>I'm a Blue H2</h2>
<h3>I'm a Purple H3</h3>
h1 {
color: #000000;
}
h2 {
color: #59FF00;
}
h3 {
color: #AA00FF;
}
style.css
index.html
# + 6-digit hexadecimal number

RGB
<h1>I'm a Red H1</h1>
<h2>I'm a Blue H2</h2>
<h3>I'm a Purple H3</h3>
h1 {
color: rgb(255, 108, 0);
}
h2 {
color:rgb(0, 193, 255 );
}
h3 {
color: rgb(32, 5, 42 );
}
style.css
index.html
Red, Green and Blue: 0 - 255

RGBA
<h1>I'm a H1</h1>
<h2>I'm a H2</h2>
<h3>I'm a H3</h3>
h1 {
color: rgba(5, 44, 120, 1);
}
h2 {
color: rgba(5, 44, 120, 0.5);
}
h3 {
color: rgba(5, 44, 120, 0.2);
}
style.css
index.html
RGB + Transparency Channel : 0.0 - 1.0

Color and Background
<div>
<p>Hello 👋</p>
<p>Goodbye 🏃🏻♂️</p>
</div>
body {
background: #32D468;
}
div {
background: #324FD4;
}
style.css
index.html
'color' for text color and 'background' for background color

Background Image
<div>
<h1>rock and roll ain't noise pollution</h1>
<p>-AC/DC</p>
</div>
body {
background-image: url(./img/1.jpg);
color: #ffffff;
}
div {
background-color: rgba(255, 255, 255, 0.3);
}
style.css
index.html
Use images as background

Border
<div>
<h1>rock and roll is not voice puution</h1>
<p>-AC/DC</p>
</div>
body {
background: #000000;
color: #ffffff;
}
div {
border-color: #ffffff;
border-width: 1px;
border-style: solid;
}
style.css
index.html
'color' for text color and 'background' for background color

div {
border: solid 1px #ffffff;
}
Selectors
Element, ID and Classes

<div>
<p><b>He:</b> You are the ";" to my code!!</p>
<p><b>She:</b> Sorry, I code in Python 💔</p>
</div>
<div>
<p><b>She:</b> Can you tell me a joke baby?</p>
<p><b>He:</b> Error 404, joke not found... 😂</p>
</div>
div {
background: #28B463;
width: 300px;
}
p {
color: #5B2C6F;
}
Element Selector
Selects all elements that have the given node name.

<div>
<p><b>He:</b> You are the ";" to my code!!</p>
<p id="mute"><b>She:</b> Sorry, I code in Python 💔</p>
</div>
<div>
<p><b>She:</b> Can you tell me a joke baby?</p>
<p><b>He:</b> Error 404, joke not found... 😂</p>
</div>
div {
background: #28b463;
width: 300px;
}
p {
color: #5b2c6f;
}
#mute {
color: rgba(91, 44, 111, 0.2);
}
ID Selector
Selects an element based on the value of its id attribute. There should be only one element with a given ID in a document.

<div>
<p class="she"><b>He:</b> You are the ";" to my code!!</p>
<p class="he"><b>She:</b> Sorry, I code in Python 💔</p>
</div>
<div>
<p class="he"><b>She:</b> Can you tell me a joke baby?</p>
<p class="she"><b>He:</b> Error 404, joke not found... 😂</p>
</div>
div {
background: #28b463;
width: 300px;
}
p {
color: #ffffff;
}
.he {
background: red;
}
.she {
background: blue;
}
Class Selector
Selects all elements that have the given class attribute.
More Selectors
🤓
Css Specificity
- IDs
- Classes, attributes
- Elements and pseudo-elements
Text and Fonts ✏️
- font-family (https://www.cssfontstack.com/)
- font-size
- font-weight
-
font-height
-
text-align
-
text-decoration
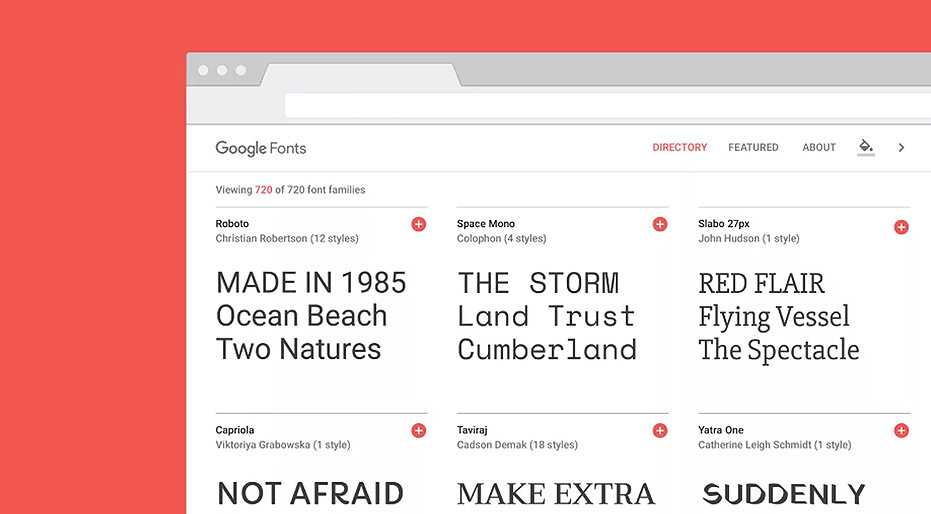
Google Fonts
Box Model
☠️
CSS
By Ali Montajebi
CSS
- 618