.Net 3.5/C# 3.0 / VS
As you might heard
We've recently updated to .Net 3.5

History
- 1.0 - Published in 2002
- 1.1 - Published in 2003
- 2.0 - Published in 2005
- 3.0 - Published in 2006
- 3.5 - Published in 2007
- 4.0 - Published in 2010
- 4.5 - Published in 2012
- 4.6 - Published in 2015

What's new ?
So much...
We can't cover everything...
Still
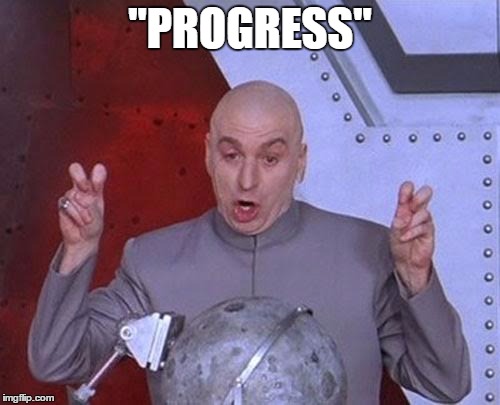
Let's see what we got
SYNTACTIC SUGAR
- Automatic Properties
- Object Initializers
- Collection Initializers
- Extension Methods
- Implicitly Typed Variable
- Anonymous Types
LINQ - intro to...
LAMBDA EXPRESSION
What we're gonna cover
INTRODUCTION
Not Covered
WPF
WCF
WF
Allot of Frameworks Classes introduced in .Net 3.5
- new presentation FW
- new communication FW
- no clue
Another day
Introduction
RESEMBLES JAVA
NOT JAVA!
Introduction - Proprties
Let's look at "plain old C# object"
class Customer
{
string id;
string name;
// ...
}
How to access these fields ?
JAVA has the "getX"/"setX"/"isX" notation used as convention (over configuration)
class Customer {
String getId() {
return id;
}
void setId(String id) {
this.id = id;
}
}
C# decided on a less cumbersome notation (still more configurable than you think)
class Customer
{
string Id
{
get { return id; }
set { id = value; }
}
}
Introduction - Proprties
Annotations / Attributes
Say we have a requirement for "Author" metadata
// Where do you set the attribute ?
@Author(Name = "Amir")
String id;
// -> or here
String getId() {}
// -> or here
void setId(String id) {}
[Author("Amir")]
string Id
{
get { return id; }
set { id = value; }
}
Automatic Properties
nail #1 in the java coffin ?
public class Customer
{
private int _customerID;
private string _companyName;
private Address _businessAddress;
public int CustomerID
{
get { return _customerID; }
set { _customerID = value; }
}
public string CompanyName
{
get { return _companyName; }
set { _companyName = value; }
}
public Address BusinessAddress
{
get { return _businessAddress; }
set { _businessAddress = value; }
}
}
public class Customer
{
public int CustomerID
{
get; set;
}
public string CompanyName
{
get; set;
}
public Address BusinessAddress
{
get; set;
}
}
This is soooooo redundant...
JUST WRITE
.Net generates the private fields for us...
// NO NEED
// NO NEED
// NO NEED
// NO NEED
// NO NEED
// NO NEED
// NO NEED
// NO NEED
// NO NEED
Automatic Properties
Or - nail #1 in the java coffin
public class Customer
{
public int CustomerID
{
get; private set;
}
public string CompanyName
{
get; private set;
}
public Address BusinessAddress
{
get; private set;
}
}
This should also be cool
Great support by VS
LET'S SEE AN EXAMPLE
Object Initilizers
Looking at POCO (plain-old-c#-object)
It's very convenient to have C'tor
public Customer(int customerID,
string companyName,
Address businessAddress,
string phone) {}
Or - have many combinations...
public class Customer
{
private int _customerID;
private string _companyName;
private Address _businessAddress;
public int CustomerID
{
get { return _customerID; }
set { _customerID = value; }
}
public string CompanyName
{
get { return _companyName; }
set { _companyName = value; }
}
public Address BusinessAddress
{
get { return _businessAddress; }
set { _businessAddress = value; }
}
}
public Customer(int customerID,
string companyName,
string phone) {}
public Customer(int customerID,
string companyName,
Address businessAddress) {}
public Customer(int customerID,
string companyName) {}
Object Initilizers
Than... There's the "Builder" pattern
- More fields - More Permutations
- C'tors get "telescopical" !
public class Customer
{
public class Builder
{
private Builder(); // PRIVATE C'TOR
private String _name;
public Builder Name(String name) { _name = name; return this; } // RETURNING THE SAME BUILDER
}
public static Builder Builder() { return new Builder() }
}
Customer.Builder().Name("Amir").Phone("000-000-000").generate()
so you can write...
The C'tor Pattern
Object Initilizers
Customer customer = new Customer();
customer.CustomerID = 101;
customer.CompanyName = "Foo Company";
customer.BusinessAddress = new Address();
customer.Phone = "555-555-1212";
Customer customer = new Customer
{
CustomerID = 101,
CompanyName = "Foo Company",
BusinessAddress = new Address
{ City="Somewhere", State="FL" },
Phone = "555-555-1212"
};
With out C'tor or Builder Patterns
Or you could write
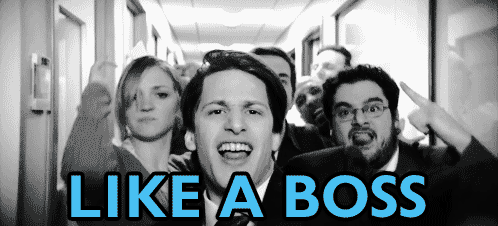
Object Initilizers
Let's see an example
Collection Initilizers
Same "shtik" - very cool...
List<Customer> custList = new List<Customer>
{
new Customer {ID = 101, CompanyName = "Foo Company"},
new Customer {ID = 102, CompanyName = "Goo Company"},
new Customer {ID = 103, CompanyName = "Hoo Company"}
};
Hate creating collections than add items one by one ?
write it
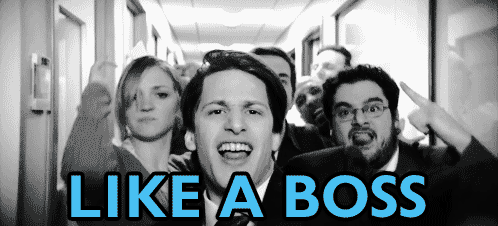
Customer customerFoo = new Customer();
customerFoo.ID = 101;
customerFoo.CompanyName = "Foo Company";
Customer customerGoo = new Customer();
customerGoo.ID = 102;
customerGoo.CompanyName = "Goo Company";
Customer customerHoo = new Customer();
customerHoo.ID = 103;
customerHoo.CompanyName = "Hoo Company";
List<Customer> customerList3 = new List<Customer>();
customerList3.Add(customerFoo);
customerList3.Add(customerGoo);
customerList3.Add(customerHoo);
Collection Initilizers
Let's see an example
Extension Methods
missing some functionality on a known class ?
public static class MyExtensions
{
public static string SayIm(this string str)
{
return "I'm" + str.ToString();
}
}
What if string could say more ?
//...
string batman= "Batman"
//...
batman.SayIm()

I ACTUALLY DON'T HAVE ANY GOOD EXAMPLE FOR THIS
:)
// OUTPUT: I'm Batman
Extension Methods
Let's see an example
Implicit Types
Or - How .Net learnt to be less tedious !!!
//..
String name = "Yo oh oh";
List<int> ids = new List<int>();
IEnumerable<String> items = ids.Where(x => x.id = 0); // We will get to this...
Dictionary<String, List<Pair<String, int>> current = new Dictionary<String, List<Pair<String, int>>();
//..
var name = "Yo oh oh";
var ids = new List<int>();
var items = ids.Where(x => x.id = 0);
var current = new Dictionary<String, List<Pair<String, int>>();
Suddenly - type deceleration it less tedious...
But is it good practice ?
Actually - Compiler can infer the type for us...
Implicit Types
Some rules...
var a;
a = "OH NO YOU DIDN'T"
Implicitly-typed local variables must be initilized
// BAD PROGRAMMER
class Program
{
var str = "";
static var number = 5;
static void DoNothing(string[] args)
{
// Some code
}
}
// BAD PROGRAMMER - local scope only
// BAD PROGRAMMER
Inferred type "var" can only be defined locally
Implicit Types
YES ? NO ?
- Required for "Anonymous Types"
- Induce better naming for local variable
IUnitTestElement current
var currentUnitTest
VS.
- removes code noise
- does not require "using" directive
- Induces variable initialization
- Better API - better names for members
// get the xml node name
var string = tree.XmlNodeName
var current = new Dictionary<String, List<Pair<String, int>>();
var current; // COMPILER ERROR
Implicit Types
YES ? NO ?
Most benefits mentioned - ARE STYLISTIC
"better naming":
- longer (Hungarian style) variable names
- will show up everywhere...
"better API", let's say in properties
- XmlNode.XmlNodeName - SUCKS
- XmlNode.Name
Implicit Types
RULE OF THUMB
When the type is obvious from the right hand side expression
C# team Quote:
"Overuse of var can make code less readable for others..."
Implicit Types
Let's see an example
Anonymous Types
Basically - Create Class Structure on the fly
var dog = new
{
Bark = "woof",
Name = "Woofy"
}
Anonymous Types
In JS it is taken to extreme and the main diff's are
We already know the concept of "Dynamic Structure" from JavaScript
- "Anonymous" the types are still "strict"
- They cannot be extended further after deceleration
- "read-only" properties
- methods are not "first class citizen" - so no !
- events also (they are semantically the same)
- There are "dirty" ways to do it... let's not...
BUT IT'S NOT THE SAME
Anonymous Types
Actually - The main use for Anonymous-Types goes in correlation with LINQ, which we'll soon cover
Anonymous Types
Let's see an example
Lambda Expressions
YAY
local Anonymous-Function, to be passed around as arguments, or returned as the value of a function call
Definition
Lambda Expressions
delegate int Operator(int i);
// function
public void TravereseList(List<int> list, Operator op)
{
for (int i = 0; i < list.Count; i++)
{
list[i] = op(list[i]);
}
}
static void DoSomething()
{
Operator square = x => x * x;
int j = square (5); //j = 25
}
Simple Lambda
Pass to function ?
Lambda Expressions
Func<int, bool> myFunc = x => x == 5;
bool result = myFunc(4); // returns false of course
Do we really need delegation ?!
Lambda Expressions
EXAMPLE
Lambda Expressions
Syntax - Expression Lambda
(input parameters) => expression
// THIS
x => x * x
// SAME AS
(x) => x * x
// MORE THAN ONE PARAM
(x, y) => x == y
// ZERO INPUT
() => SomeMethod()
(int x, string s) => s.Length > x
Some times - it's impossible for the compiler to infer the input types
So - Optionally - you can add the types explicitly
Lambda Expressions
Syntax - Statment Lambda
(input parameters) => {statement;}
delegate void SomeDelegate(string s);
// ...
SomeDelegate myDel = n => { string s = n + " " + "World"; Console.WriteLine(s); };
// ...
myDel("Hello");
Almost the same - but body can consist of several statments
Lambda Expressions
Syntax - asynchronous lambdas

Visual Studio Snippets
?
Visual Studio Snippets
CODE SNIPPETS are ready-made snippets of cod you can quickly add into your code
Visual Studio Snippets
Two types
Type 1: Insertion
Type 2: Surround
Visual Studio Snippets
Two types
Type 1: Insertion
Type 2: Surround
Both can be added, either while typing with "tab-tab", or with Ctrl+k than X, Ctrl+k than S respectivily
Visual Studio Snippets
Let's see an examples
LINQ &
Extension Methods
for collections
Language Integrated Query

LINQ
int[] numbers = { 5, 4, 1, 3, 9, 8, 6, 7, 2, 0 };
int j = 0;
for(int i = 0; i < numbers.Count ; i++)
{
if(numbers[i] > 5)
{
j++;
}
}
Let's assume we have the following:
And we want to count the number of items larger than 5

LINQ
int[] numbers = { 5, 4, 1, 3, 9, 8, 6, 7, 2, 0 };
Let's assume we have the following:
And we want to count the number of items larger than 5
int oddNumbers = numbers.Count(n => n > 5);

LINQ
int[] numbers = { 5, 4, 1, 3, 9, 8, 6, 7, 2, 0 };
Let's assume we have the following:
What more can we do
MORE
- GENERICS - JAVA vs. .NET
- Coding Conventions
- https://msdn.microsoft.com/en-us/library/ff926074.aspx
.Net 3.5/C#3.0/VS
By Amir Gal-Or
.Net 3.5/C#3.0/VS
- 841