INTRO O
PROBLEM SOLVING AND
PROGRAMMING IN PYTHON
(use the Space key to navigate through all slides)
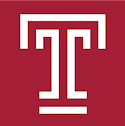
Prof. Andrea Gallegati |
Prof. Dario Abbondanza |
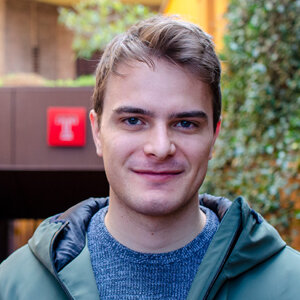
CIS 1051 - IF STATEMENT
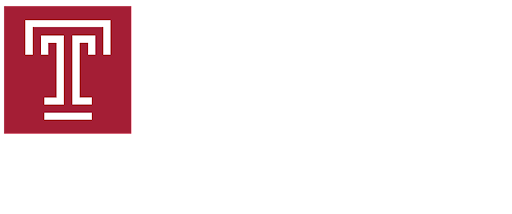
Understanding the
statement
MAKING Decisions in Code
if
Every day, we make decisions based on conditions:
- If it’s sunny, we wear sunglasses.
- If we’re hungry, we eat.
- If our phone battery is low, we charge it.
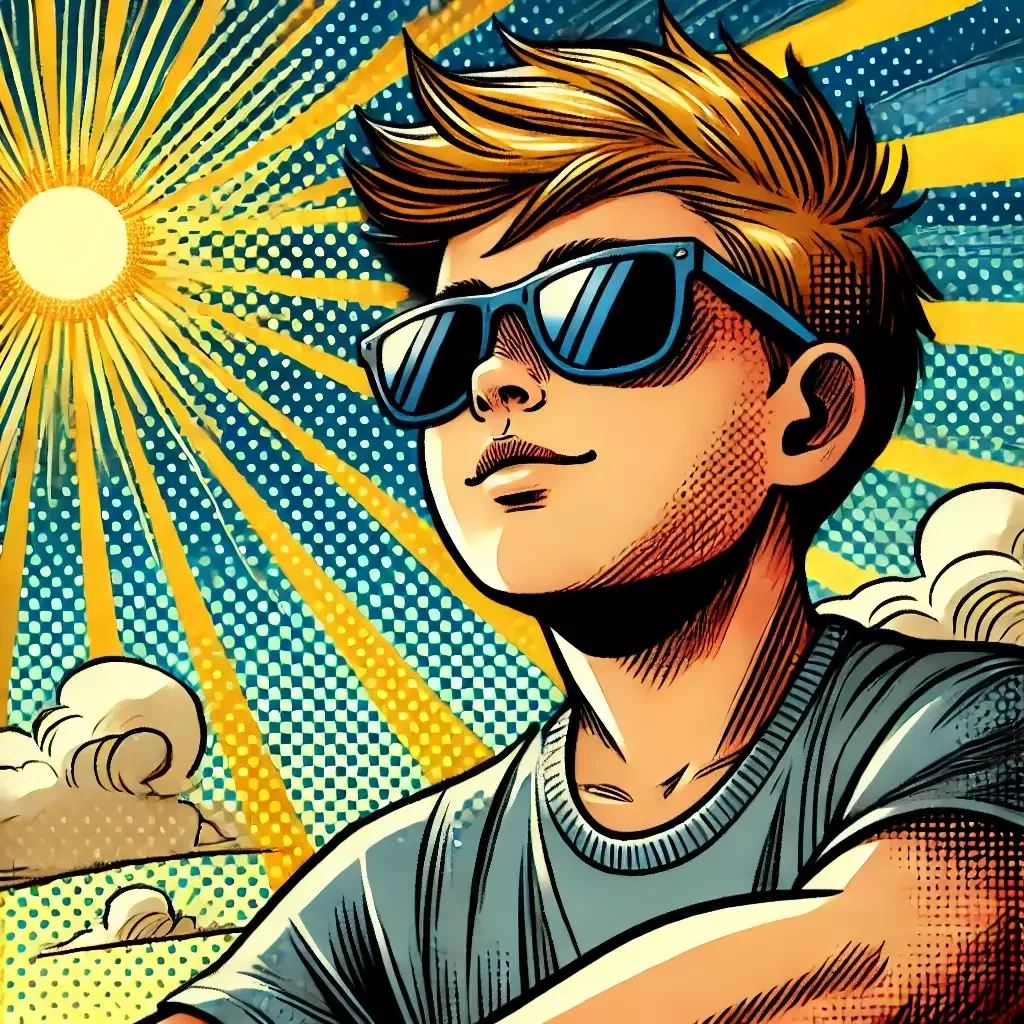
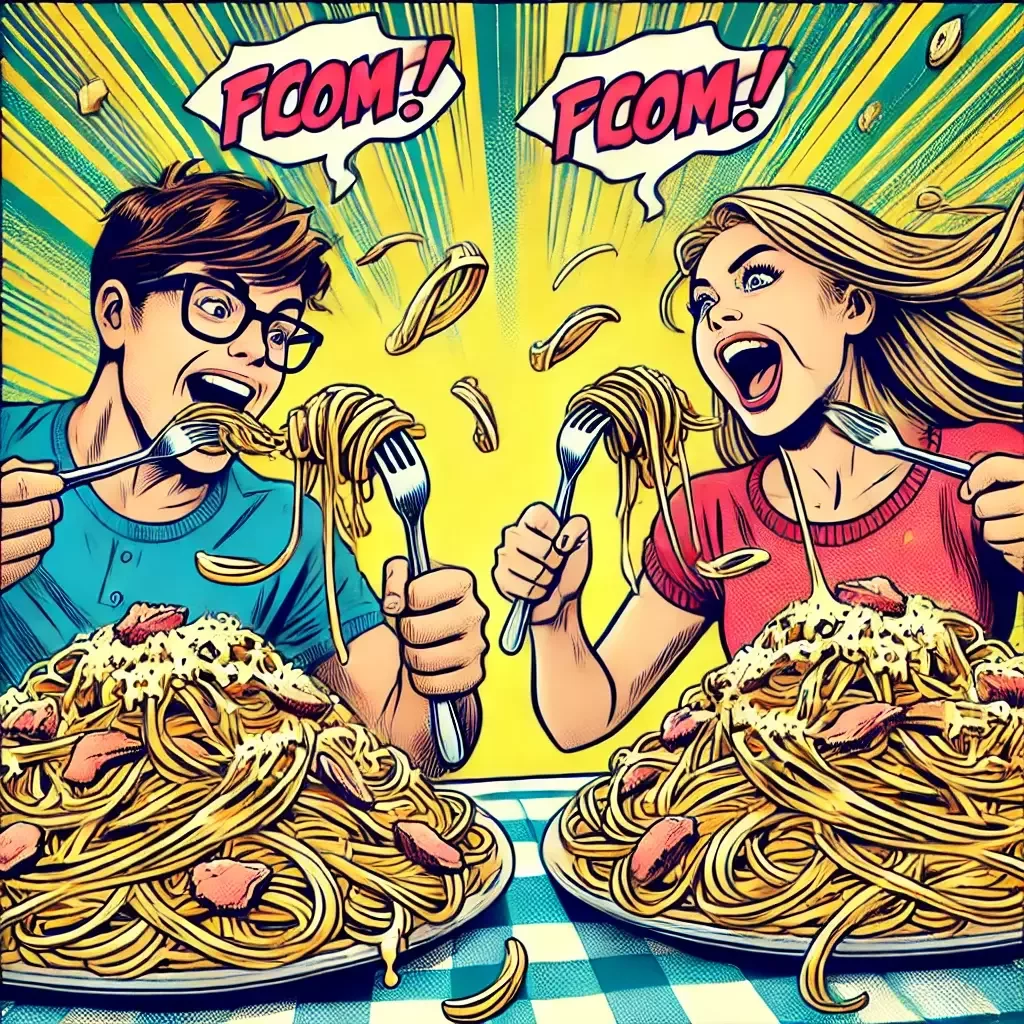
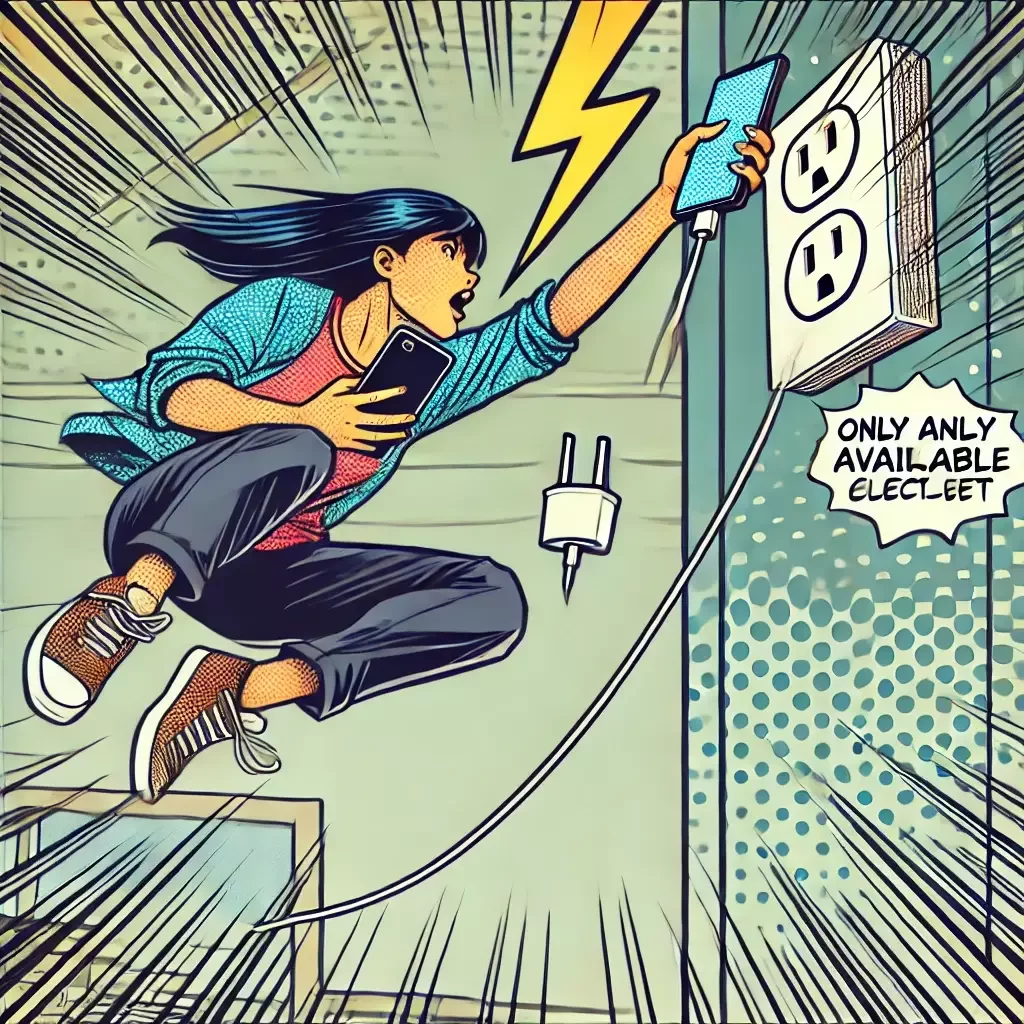
Syntax:
write Python programs that react to different conditions
if condition:
# Code that runs if
# the condition is True
pass
it allows us to execute a block of code only when a
specific condition is met.
- An expression that evaluates to
True
orFalse
. - A colon (
:
) fends the header. - The indented block below is the body.
Example: Checking the Weather
import random
# Define different weather conditions
w_conditions = ["rainy", "sunny", "cloudy"]
# Pick a random value from the above list
weather = random.choice(w_conditions)
# Print the random value
print("The weather is " + weather + ".")
if weather == "rainy":
print("Don't forget your umbrella!")
Using <!=>
Comparison Operators
Operator | Meaning | Example (x = 10 , y = 5 ) |
---|---|---|
> |
Greater than |
x > y → True
|
< |
Less than |
x < y → False
|
>= |
Greater than or equal to |
x >= 10 → True
|
<= |
Less than or equal to |
y <= 5 → True
|
== |
Equal to |
x == y → False
|
!= |
Not equal to |
x != y → True
|
in Conditions
Example: Grading Exams
import random
# Define the list of scores
grades = [20, 45, 56, 68, 75, 100]
# Pick a random value from the list
score = random.choice(grades)
# Print the random score
print("Score: " + str(score))
if score >= 60:
print("Congratulations!")
print(" You passed the exam.")
The else
Statement
an alternative
import random
# Define the range of possible scores
min_score = 20
max_score = 100
# Pick a random score
score = random.randint(min_score,
max_score)
# Print the random score
print("Score: " + str(score))
# Check if score meets the condition
if score >= 60:
message_1 = "Congratulations! "
message_2 = "You passed the exam."
else:
message_1 = "Sorry, you didn't pass."
message_2 = " Try Again!"
print(message_1 + message_2)
The elif
Statement
multiple conditions
import random
# Define the range of possible scores
min_score = 20
max_score = 100
# Pick a random score
score = random.randint(min_score,
max_score)
# Print the random score and
# the corresponding Grade
if score >= 90:
print("Grade: A")
elif score >= 80:
print("Grade: B")
elif score >= 70:
print("Grade: C")
else:
print("Grade: F")
What Happens?
- If
score >= 90
, it prints"Grade: A"
. - Otherwise, it checks if
score >= 80
... and so forth!
The program stops checking after the first True
condition.
Operator | Meaning | Example (x = 8 , y = 5 ) |
---|---|---|
and | Both conditions must be True
|
x > 5 and y > 2 → True
|
or | At least one condition is True
|
x > 10 or y < 10 → True
|
not | Negates the condition |
not (x > 10) → True
|
Logical operators
Age Verification for Discount
age = 17
is_student = True
if age < 18 and is_student:
print("You get a student discount!")
Checking for Even Numbers
number = 7
if number % 2 == 0:
print(str(number) + " is even.")
else:
print(str(number) + " is odd.")
NESTED if
Statements
age = 20
citizenship = "US"
if age >= 18:
if citizenship == "US":
print("You are eligible to vote.")
else:
print("You must be a US citizen to vote.")
else:
print("You must be at least 18 to vote.")
How It Works
- Checks if
age >= 18
isTrue.
- Checks if the person is a
US
citizen isTrue.
- If both are
True
, it prints "You are eligible to vote."
Otherwise, it prints "You must be a US citizen." - If
age < 18
, it prints "You must be at least 18 to vote."
Recap
-
if
statement executes a code block (conditionTrue)
. -
else
statement provides an alternative action. -
elif
statement allows multiple conditions.
-
Comparison operators (
==
,!=
,>
,<
,>=
,<=
)
help define conditions. -
Logical operators (
and
,or
,not
)
combine multiple conditions.
-
Nested
if
statements allow deeper decision-making.
Try It Yourself!
write a Python program that checks the temperature and prints different messages
temperature = 15 # Try changing this value!
if temperature > 30:
print("It's hot outside! Stay hydrated.")
elif temperature > 20:
print("The weather is nice and warm.")
elif temperature > 10:
print("It's a bit chilly, grab a sweater.")
else:
print("Brrr! It's freezing outside!")
Great Work! 🎉
Now you understand if statements and conditional logic in Python!
Ready to try them out? 🚀
This was crafted with
A Framework created by Hakim El Hattab and contributors
to make stunning HTML presentations
if-statement
By Andrea Gallegati
if-statement
- 21