High Voltage Python

Andrea Stagi Develover @ Nephila



SHIELDS

Arduino IDE

PRogramming With ARduino
#include <LiquidCrystal.h>
LiquidCrystal lcd(12, 11, 5, 4, 3, 2);
void setup() {
lcd.begin(16, 2);
lcd.print("hello, world!");
}
void loop() {
lcd.setCursor(0, 1);
lcd.print(millis()/1000);
}
PRogramming With ARduino
// Pin 13 has an LED connected on most Arduino boards.
// give it a name:
int led = 13;
// the setup routine runs once when you press reset:
void setup() {
// initialize the digital pin as an output.
pinMode(led, OUTPUT);
}
// the loop routine runs over and over again forever:
void loop() {
digitalWrite(led, HIGH); // turn the LED on (HIGH is the voltage level)
delay(1000); // wait for a second
digitalWrite(led, LOW); // turn the LED off by making the voltage LOW
delay(1000); // wait for a second
}

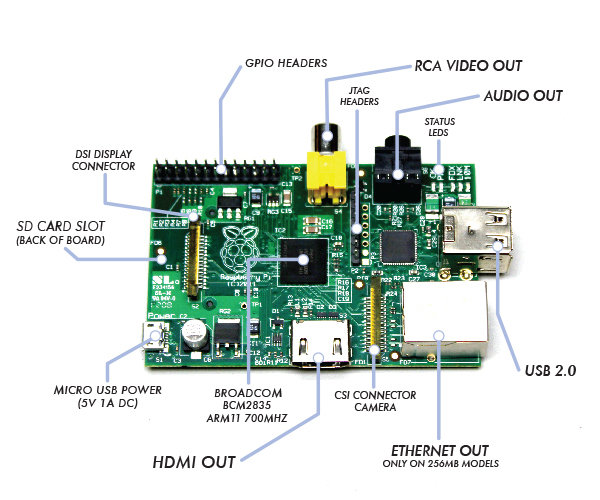

Raspberry PI GPIO


Raspberry hats

Programming With Raspberry PI
import RPi.GPIO as GPIO
GPIO.setmode(GPIO.BOARD)
GPIO.setup(7, GPIO.OUT)
GPIO.output(7,True)
The best of both worlds
USB connection

Duino
Nanpy
Firmata
How do they work?
Arduino
Duino
int led = 13;
void setup() {
pinMode(led, OUTPUT);
}
void loop() {
digitalWrite(led, HIGH);
delay(1000);
digitalWrite(led, LOW);
delay(1000);
}
var arduino = require('duino'),
board = new arduino.Board();
var led = new arduino.Led({
board: board,
pin: 13
});
led.blink(1000);
ಠ_ಠ
Arduino
Nanpy
int led = 13;
void setup() {
pinMode(led, OUTPUT);
}
void loop() {
digitalWrite(led, HIGH);
delay(1000);
digitalWrite(led, LOW);
delay(1000);
}
from nanpy import ArduinoApi, SerialManager
from time import sleep
a = ArduinoApi(connection=SerialManager())
a.pinMode(13, a.OUTPUT)
for i in range(10000):
a.digitalWrite(13, (i + 1) % 2)
sleep(1)

More

Objects MApping
1 Nanpy Object -> 1 Arduino Object
from nanpy import Servo
import time
servo = Servo(7)
for move in [0, 90, 180, 90, 0]:
servo.write(move)
time.sleep(1)
#ifndef SERVO_CLASS
#define SERVO_CLASS
#include "BaseClass.h"
#include "MethodDescriptor.h"
class Servo;
namespace nanpy {
class ServoClass: public ObjectsManager<Servo> {
public:
void elaborate( nanpy::MethodDescriptor* m );
const char* get_firmware_id();
};
}
#endif
Objects MApping
void nanpy::ServoClass::elaborate( nanpy::MethodDescriptor* m ) {
ObjectsManager<Servo>::elaborate(m);
if (strcmp(m->getName(),"new") == 0) {
v.insert(new Servo());
v[v.getLastIndex()]->attach(m->getInt(0));
m->returns(v.getLastIndex());
}
if (strcmp(m->getName(), "write") == 0) {
v[m->getObjectId()]->write(m->getInt(0));
m->returns(0);
}
if (strcmp(m->getName(), "read") == 0) {
m->returns(v[m->getObjectId()]->read());
}
if (strcmp(m->getName(), "writeMicroseconds") == 0) {
v[m->getObjectId()]->writeMicroseconds(m->getInt(0));
m->returns(0);
}
if (strcmp(m->getName(), "readMicroseconds") == 0) {
m->returns(v[m->getObjectId()]->readMicroseconds());
}
// ...
}
from nanpy import DallasTemperature
sensors = DallasTemperature(5)
n_sensors = sensors.getDeviceCount()
print("There are %d devices connected on pin %d" % (n_sensors, sensors.pin))
addresses = []
for i in range(n_sensors):
addresses.append(sensors.getAddress(i))
sensors.setResolution(12)
while True:
sensors.requestTemperatures()
for i in range(n_sensors):
temp = sensors.getTempC(i)
print("Device %d (%s) temperature is %0.2f °C" % (i, addresses[i], temp))
print("\n")
Create your own Arduino framework
Protocol definition
FEW RAM & No GC
Memory fragmentation
First allocation
#define K (1024)
char *p1, *p2;
p1 = malloc(3*K);
p2 = malloc(4*K);
p2
p1
4K
3K FREE
3K
Deallocation
...
free(p1);
p1 = malloc(4*K);
...
p2
4K
3K FREE
3K FREE

Servo motor

Connection with Arduino

Altimeter
from mcpi import minecraft
from nanpy import Servo, SerialManager
import time
mc = minecraft.Minecraft.create()
def get_angle(pos_player, max_alt=180):
angle = pos_player.y * (90.0/max_alt) + 90
return int(angle)
connection = SerialManager(
device='/dev/ttyACM0'
)
servo = Servo(
7,
connection=connection,
rtscts=True
)
while True:
pos_player = mc.player.getPos()
angle = get_angle(pos_player)
servo.write(angle)
https://github.com/djangobeer/minecraftpi-hack
Django Rest Framework
DHT

Connection with Arduino

STAGI.ANDREA@GMAIL.COM
GITHUB.COM/ASTAGI
TWITTER: @4STAGI

High Voltage Python
By Andrea Stagi
High Voltage Python
- 2,028