Smart Objects
and
IoT
vitaletti@dis.uniroma1.it
Smart Object
A Smart Object is an object that enhances the interaction with not only people but also with other Smart Objects.
Connectivity
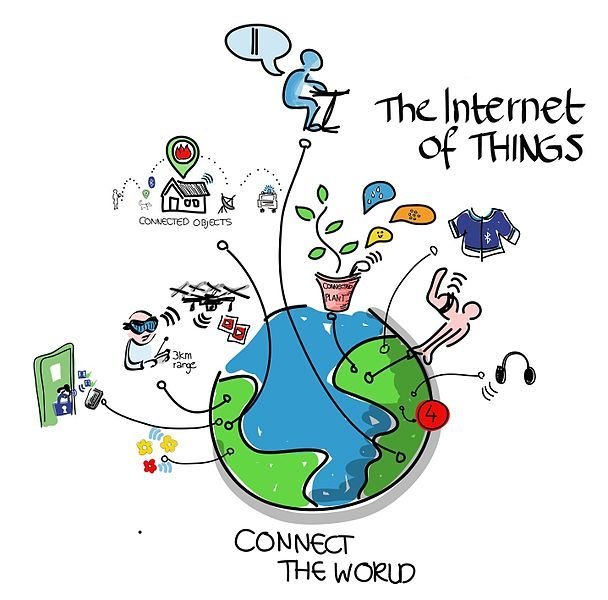
A connected world
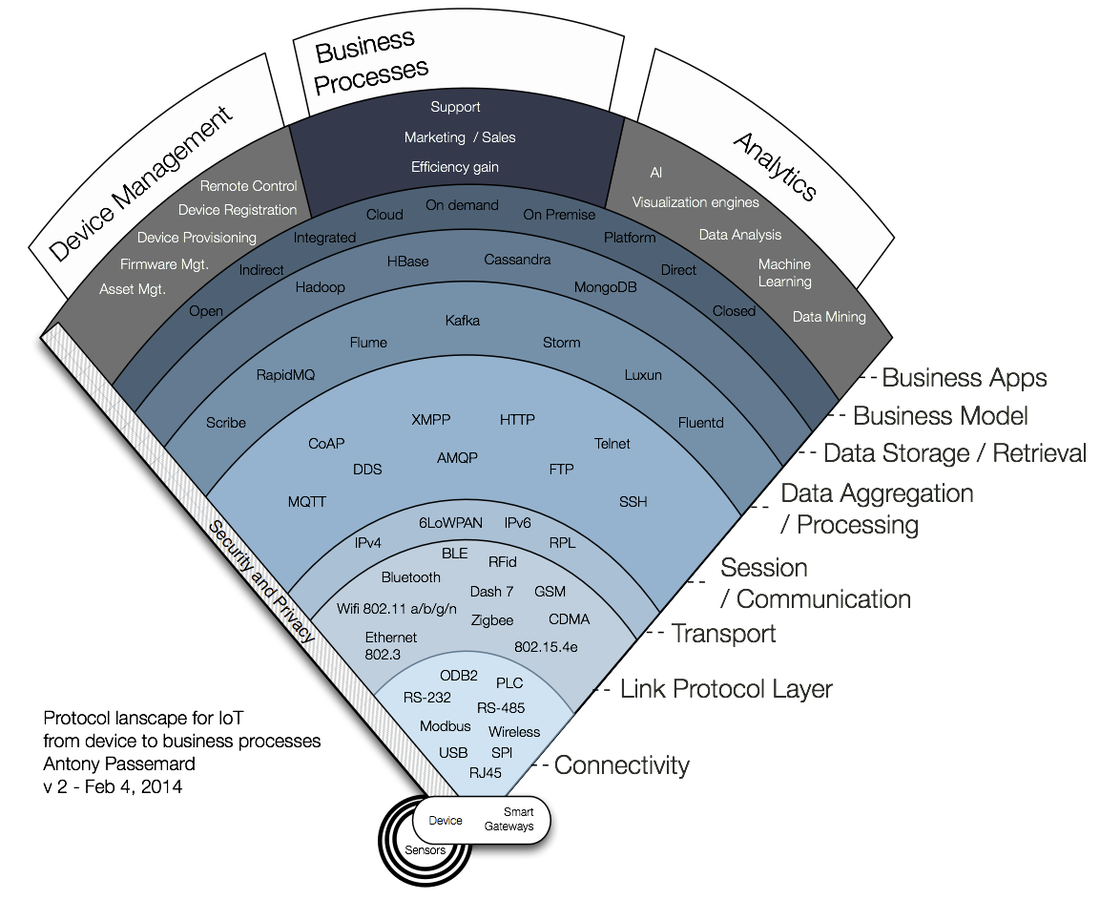
A communication protocol is a system of digital rules for data exchange within or between computers and/or
smart objects
Smart Object Abstraction
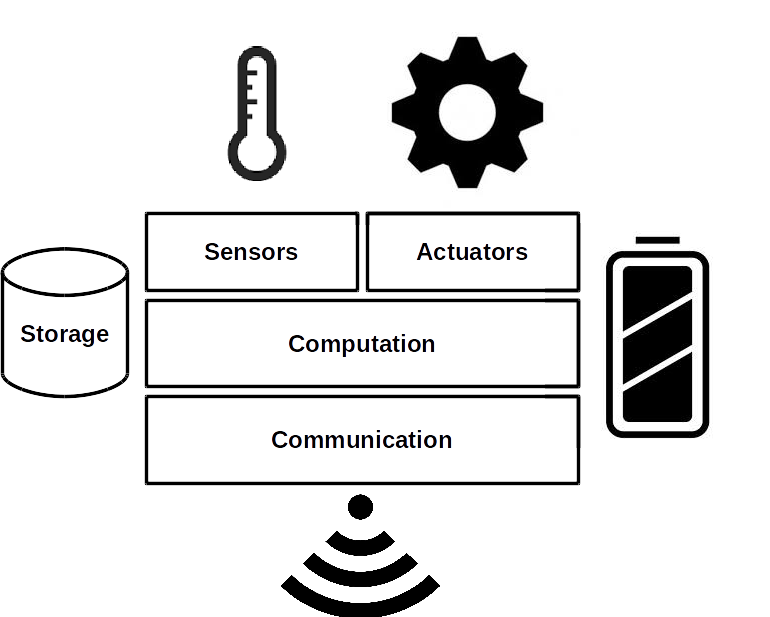
A simple example
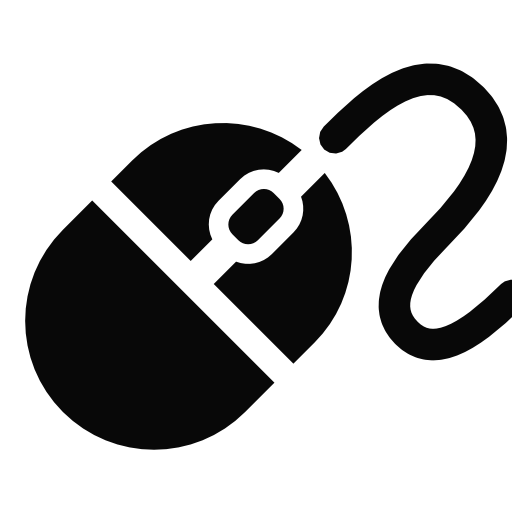
<html>
<head>
<script src='https://cdn.firebase.com/js/client/2.0.4/firebase.js'></script>
<script src='https://ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js'></script>
</head>
<body>
Temperature:
<input type="range" min="0" max="40" value="0" step="5" onchange="pubTemp(this.value)" />
<span id="range">0</span>
<script>
var myDataRef = new Firebase('https://flickering-heat-1338.firebaseio.com/');
function pubTemp(newValue)
{
myDataRef.set({temp: newValue});
document.getElementById("range").innerHTML=newValue;
}
</script>
</body>
</html>
A virtual temp. sensor
<html>
<head>
<script src='https://cdn.firebase.com/js/client/2.0.4/firebase.js'></script>
<script src='https://ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js'></script>
</head>
<body>
Temperature: <span id="range">0</span>
<script>
var myDataRef = new Firebase('https://flickering-heat-1338.firebaseio.com/');
myDataRef.on('value', function(snapshot) {
var message = snapshot.val();
document.getElementById("range").innerHTML=message.temp;
});
</script>
</body>
</html>
Get data remotely

The whole process

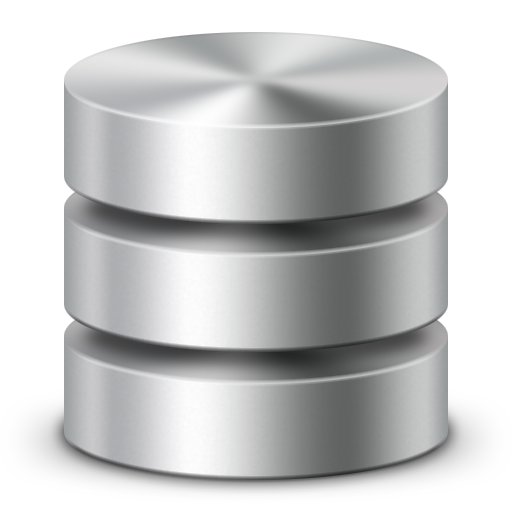

<html>
<head>
<script src='https://cdn.firebase.com/js/client/2.0.4/firebase.js'></script>
<script src='https://ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js'></script>
</head>
<body>
Temperature:
<input type="range" min="0" max="40" value="0" step="5" onchange="pubTemp(this.value)" />
<span id="range">0</span>
<script>
var myDataRef = new Firebase('https://flickering-heat-1338.firebaseio.com/');
function pubTemp(newValue)
{
myDataRef.set({temp: newValue});
document.getElementById("range").innerHTML=newValue;
}
</script>
</body>
</html>
<html>
<head>
<script src='https://cdn.firebase.com/js/client/2.0.4/firebase.js'></script>
<script src='https://ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js'></script>
</head>
<body>
Temperature:
<input type="range" min="0" max="40" value="0" step="5" onchange="pubTemp(this.value)" />
<span id="range">0</span>
<script>
var myDataRef = new Firebase('https://flickering-heat-1338.firebaseio.com/');
function pubTemp(newValue)
{
myDataRef.set({temp: newValue});
document.getElementById("range").innerHTML=newValue;
}
</script>
</body>
</html>
<html>
<head>
<meta http-equiv="content-type" content="text/html;charset=utf-8">
<script type="text/javascript" src="three.min.js"></script>
<script type="text/javascript" src="jsmodeler.js"></script>
<script src='https://cdn.firebase.com/js/client/2.0.4/firebase.js'></script>
<script src='https://ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js'></script>
<title>JSModeler Minimal</title>
<script type="text/javascript">
var body = null;
var materials = null;
var viewer = null;
var meshes = null;
function Load ()
{
var viewerSettings = {
cameraEyePosition : [-2.0, -1.5, 1.0],
cameraCenterPosition : [0.0, 0.0, 0.0],
cameraUpVector : [0.0, 0.0, 1.0]
};
viewer = new JSM.ThreeViewer ();
viewer.Start (document.getElementById ('example'), viewerSettings);
body = JSM.GenerateCuboid (1.0, 1.0, 1.0);
meshes = JSM.ConvertBodyToThreeMeshes (body);
for (var i = 0; i < meshes.length; i++) {
viewer.AddMesh (meshes[i]);
}
viewer.Draw ();
}
function Change_Color(temp,soglia)
{
viewer.RemoveMeshes ();
materials = new JSM.Materials ();
if (temp>soglia){
materials.AddMaterial (new JSM.Material ({ambient : 0xff0000, diffuse : 0xff0000}));
}
else{
materials.AddMaterial (new JSM.Material ({ambient : 0x0000ff, diffuse : 0x0000ff}));
}
body.GetPolygon (0).SetMaterialIndex (0);
meshes = JSM.ConvertBodyToThreeMeshes (body,materials);
for (var i = 0; i < meshes.length; i++) {
viewer.AddMesh (meshes[i]);
}
viewer.Draw ();
}
window.onload = function ()
{
Load ();
}
</script>
</head>
<body>
<canvas id="example" width="1000" height="500"></canvas>
<script>
var myDataRef = new Firebase('https://flickering-heat-1338.firebaseio.com/');
myDataRef.on('value', function(snapshot) {
var message = snapshot.val();
temp = parseInt(message.temp);
Change_Color(temp,30);
});
</script>
</body>
</html>
The whole process

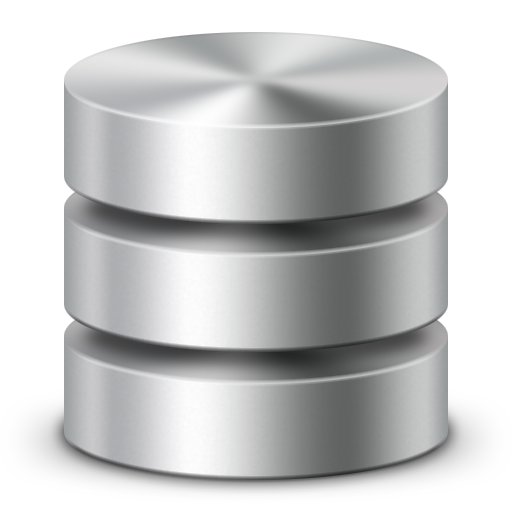

Iot and Smart Objects
By Andrea Vitaletti
Iot and Smart Objects
- 1,712