ReactJS Intro
Andrew Dacenko <asd@fb.com>
Frontend Engineer @Facebook
August 6, 2018
Library for building Interfaces
Declarative
Component-Based
Write Everywhere
<Square color="black" />

<Form>
<Input />
<Input />
<FileInput />
<Submit />
</Form>

Getting Started


Hello, World!
$ git checkout -b hello-world hello-world
$ yarn && yarn start

Source Code
class HelloMessage extends React.Component {
render() {
return <div>{'Hello, ' + this.props.message + '!'}</div>;
}
}
ReactDOM.render(
<HelloMessage message="World"/>,
document.getElementById('root')
);
class HelloMessage extends React.Component {
render() {
return React.createElement(
'div',
null,
'Hello, ' + this.props.message + '!'
);
}
}
ReactDOM.render(
React.createElement(HelloMessage, { message: 'World' }),
document.getElementById('root')
);
Transformed Code
Task
Play around with props
- display your name
- display your age
- display your hometown
Data Flow

Component State
class Clicker extends React.Component {
state = {
count: 0,
};
render() {
return (
<div className="container">
<button className="button" onClick={() => {
this.setState({
count: this.state.count + 1,
});
}}>
Increment
</button>
<Counter count={this.state.count}/>
</div>
);
}
}
Task
- add Decrement button
- do not decrement below 0
- add Max count (9)
- display '9+' on Max
$ git checkout -b state-updates state-updates
State Management Libraries
Flux

Redux

MobX

Routing
import { BrowserRouter } from 'react-router-dom'
ReactDOM.render((
<BrowserRouter>
<App/>
</BrowserRouter>
), holder)
import { Route, Switch } from 'react-router-dom'
// when location = { pathname: '/about' }
<Route path='/about' component={About}/> // renders <About/>
<Route path='/contact' component={Contact}/> // renders null
<Route component={Always}/> // renders <Always/>
<Switch>
<Route exact path='/' component={Home}/>
<Route path='/about' component={About}/>
<Route path='/contact' component={Contact}/>
{/* when none of the above match, <NoMatch> will be rendered */}
<Route component={NoMatch}/>
</Switch>
Task
- add Short Description field
- add Description field using MD
- implement adding Products
- update cards to render Short Description
- add new Route to display Product
- implement "Add to Cart" / "Remove"
- show cart items count in header
- show cart items price in header
- persist data on changes
$ git checkout -b store store
Learn More
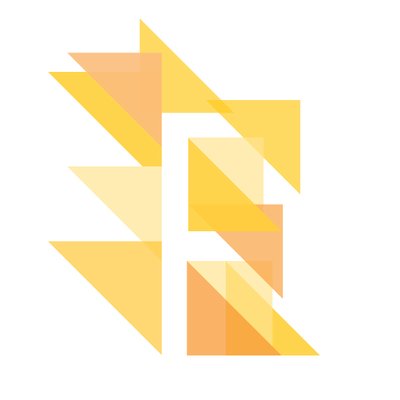
ReactJS Intro
By Andrew Dacenko
ReactJS Intro
ReactJS Intro Workshop for Coders of Colour Aug 6th, 2018
- 650