Performance in JavaScript

Introduction
Andrii Zhukov
Senior Software Engineer
at GlobalLogic

Agenda
- Best Practises
- Code minimization, Tree shaking
- Dynamic import / Lazy loading / Code splitting
- Working with memory
- Code review
- Horror code optimization
- Third party libraries
- Working with Event Loop
- Web Workers
- Memoization
- Server side computing
- Other ways to optimize
- You don't need optimization

Performance in JS

What is performance?
- Application performance
- Development productivity
- Customer satisfaction
- Your guess

Use Best Practises
const someArray = [1, 2, 3, 4, 5];
someArray.map(...).filter(...);
VS
someArray.forEach(...);
Use Best Practises
See previous episode:
Hot or Not:
Algorithms for JS devs

Some examples

import React from 'react';
class Greeting extends React.Component {
render() {
return (<div>{this.state.count}</div>);
}
}
Some examples

import React, { useState } from 'react';
function Greeting() {
const [count, setCount] = useState(0);
return (<div>{count}</div>);
}
Some examples

import Vue from 'vue';
import App from './App.vue';
new Vue({
render: h => h(App),
}).$mount('#app');
Some examples

import { createApp } from 'vue';
import App from './App.vue';
createApp(App).mount('#app');
Don't be a hostage of the tool, but use good solutions
export class DoEverything {
showSomeone() {...}
hideSomeone() {...}
saveSomeone() {...}
doNotMixinThis() {...}
makeSomeCofee() {...}
}
Don't be a hostage of the tool, but use good solutions
export showSomeone() {...}
export hideSomeone() {...}
export saveSomeone() {...}
export doNotMixinThis() {...}
export makeSomeCofee() {...}
Don't be a hostage of the tool, but use good solutions
Tree shaking
Code minimization

Some more good solutions
Dynamic import
Lazy loading
Code splitting

const route = () =>
import(somePath);
Working with memory
Garbage Collector clears memory when there is no
Object reference

Map VS WeakMap
Working with memory
Garbage Collector clears memory when there is no
Object reference
Map VS WeakMap
WeakRef
ImmutableJS



Code Review
Your code needs to be checked and that's it.

- Not to humiliate your dignity
- No one writes optimized code straight away
- Optimized code can be optimized
- One head is good and so on
Horror code optimization
const someArray = [2, 3, 1, 7, 5, 6, 4, 9, 10, 8];
const newArray = someArray
.sort((a, b) => a - b)
.filter((item) => item % 2)
.map((item) => item * 2);

Horror code optimization
function changeArrayOrReturnDefault(
someArray,
defaultArray = []
){
// do some operations
const newArray = someArray.map(...);
// do something else
if (someArray.length === 0) {
return defaultArray;
}
return newArray;
}

Horror code optimization
const someArray = [1, 2, ..., 99, 100];
function makeOnlyFiveEmployeers(someArray)
{
let employeers = "", count = 0;
someArray.forEach((i) => {
count++;
if (count < 6) {
employeers +=
`Employee ${i}`;
}
});
}

Horror code optimization
function makeAndRender(arrayHTMLElements) {
arrayHTMLElements.forEach(
(htmlElement) => {
const someInnerText =
`<div>${htmlElement}</div>`;
const blog = document
.querySelector("#blog");
blog.innerHTML = someInnerText;
}
);
}

Third party libraries
- The code doesn't belong to you and can use any horror stories mentioned before

- Most of the code you may not need at all
- May use legacy code and not have code support
- Vulnerability, versioning and other dangerous things
- Can use other libs with problems above
Third party libraries
Solutions:
- Write your own code and use your own libs :)

- If you can't write the code, use only reliable, proven libraries
Event Loop

Event Loop

Event Loop

Event Loop

Event Loop

Event Loop
const bigData = [1, 2, 3, ..., 1000000000000];
let numberIteration = 0
function doSmallChanksOfTasks(bigData) {
do {
doOneTask(bigData[numberIteration]);
numberIteration++;
} while(numberIteration % 1e6 !== 0);
if (numberIteration < bigData.length) {
setTimeout(doSmallTasks); // or queueMicrotask
}
}
Web Workers

const myWorker = new Worker("worker.js");
Memoization
Vue, React - useMemo
React - useCallback, resellect, etc.

Server side computing

Other ways to optimize
If the previous methods do not help,
you need to redevelop your application completely

Other ways to optimize
Keep the client busy with something else,
organize the active work of the brain

Other ways to optimize
You don't need optimization

That's all
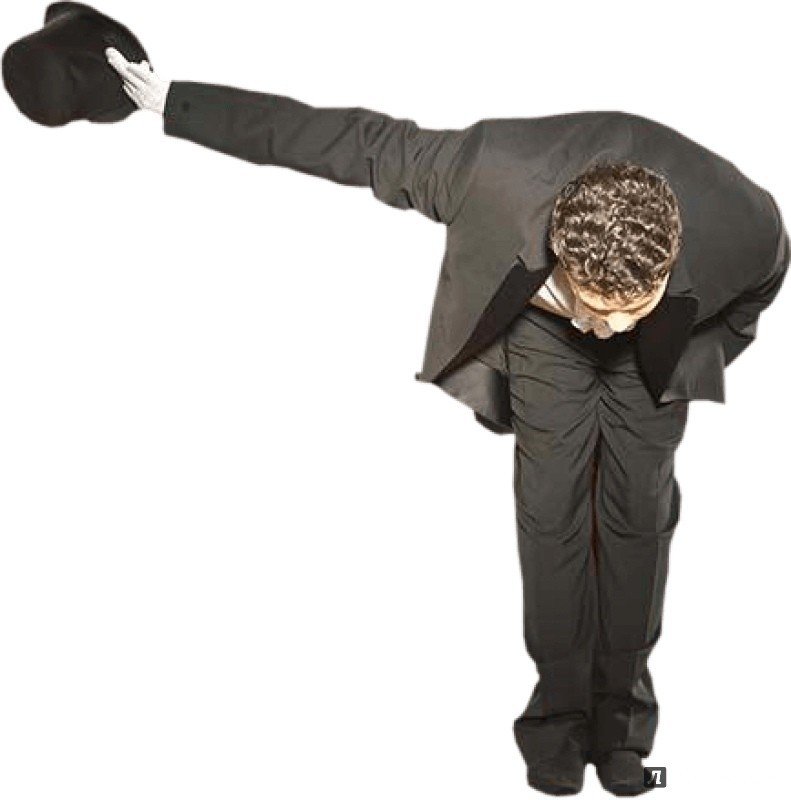
Questions

performanceInJS
By andreyvalerievich
performanceInJS
- 142