G12_C6_For_Teacher
Bullet Firing

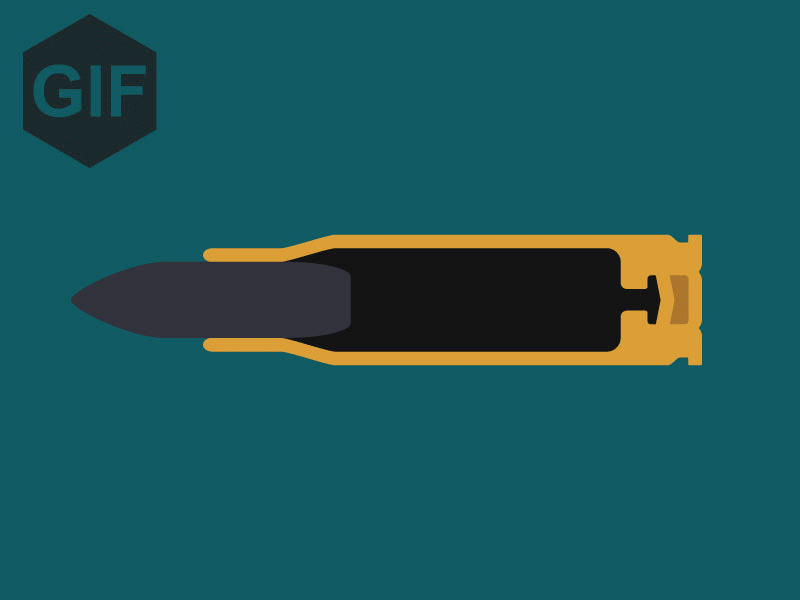
Activity Flow | Slide No. | Topic | Time |
---|---|---|---|
TA | 4-8 | Last class revision+quiz | 1 min |
9-13 | State of Bullets | 6 min | |
14-18 | TA1: Adding bullets to Spaceship | 6 min | |
19-21 | Firing of Bullets | 8 min | |
SA | 22-26 | SA1: Fire the bullets, when bullets are in ready state | 8 min |
Wrap Up | 27-30 | Wrap Up Quiz | 5 min |
31-32 | SAA1: Resolve swinging problem of Bullets | ||
33-34 | Creation of application that shows time table of daily routine of students | ||
35 | Thank You! |

Class Structure
Slide No. | Topic |
---|---|
9-13 | State of Bullets |
14-18 | TA1: Adding bullets to Spaceship |
19-21 | Firing of Bullets |
25 | SA1 solution: Fire the bullets, when bullets are in ready state |
32 | SAA1 solution: Resolve swinging problem of Bullets |
34 | SAA2 solution |
Preparation and Reference

Prerequisites
FOR STUDENTS
-
Computer with Internet connection.
-
Spyder IDE installed.
-
"pygame" package installed.
FOR TEACHER
-
Computer with Internet connection.
-
Latest browser installed.
-
"pygame" package installed.
-
Spyder IDE installed.


What we did in the last class?
Same images


(WARM-UP QUIZ)
Same images
Which syntax is correct to add a new value at the end of list?
A
list.append(value)
list.insert(value)
Q.1
A
list.add(value)
B
C

append function of the list is used to add the items at the end of the list. So option A is correct.
D
list.addItem(value)
What would be the output of the following?
B
Integer number between 4 to 10
decimal number
between 3.5 to 10.0
Q.2
A
Error
B
C

randint() generates only integral numbers. It never works with real values. So it will throw an error.
D
Combination of integral and decimal value from 3.5 to 10
import random
print(random.randint(3.5,10))

Same images
Game Feature to be added


In today's class, we will add bullets to the spaceship and create a firing feature.
Bullet has two states



The Game State that we are going to develop:
1. Creation of Bullet (that is Ready State)
2. Firing of Bullet
What is State?

What is State?
Ready State


Moving State
When an object changes its position/behavior it is called changing of state.
Flow of program
1. Drawing a rectangle as a bullet.
2. Change it to ready state.
3. Set its position near the spaceship/player.
4. Add an event on which bullet will fire so change the state of the bullet to fire state.
5.If the bullet is in a fire state move the location of the bullet.
6. If the bullet crosses the boundary of the game window, set the bullet in a ready state.



GREAT!

Same images

Same images
Teacher Activity
Step 1 - Bullet creation
Game loop
Same images
enemycount=10
enemies=[]
evlx=[]
evly=[]
bullet=pygame.Rect(200,200,5,5)
bulletState="ready"
for i in range(1,enemycount):
Creating rectangle for bullet
Set the state of the bullet to ready. That means bullet is ready to fire.

Same images
Step 2 - Check the ready state of bullet
if event.key ==pygame.K_RIGHT:
change = -6
if event.key == pygame.K_UP:
forward = True
if bulletState == "ready":
bullet.x=player.x
bullet.y=player.y
pygame.draw.rect(screen,(225,225,15),bullet)
Check the state of the bullet, if it is in ready state
Set the x position of the bullet close to position of player's x and y-axis
Draw the rectangle to show the bullet on screen
Output Bullet in ready state



The bullet is not close to player. How to fix this?
if bulletState == "ready":
bullet.x=player.x+20
bullet.y=player.y+24
Add a number in x and y axis to hide the bullet

Step 3: Set the location of bullet near spaceship
if bulletState == "ready":
bullet.x=player.x+15
bullet.y=player.y+15
if bulletState == "ready":
bullet.x=player.x+20
bullet.y=player.y+20







How to fire a bullet?
That's great! When the user presses the space bar and the bullet is in a ready state.


Step 4 - Fire bullet on event
if event.key == pygame.K_UP:
forward = True
if event.key == pygame.K_SPACE and bulletState=="ready":
bulletState="fired"
if bulletState == "ready":
bullet.x=player.x+20
bullet.y=player.y+24
if bulletState=="fired":
bullet.x ,bullet.y = newxy(bullet.x, bullet.y, 20 , angle)
Check if the user presses the space bar and state
of the bullet is in ready.
Check if this is fired state, call newxy(), and find the new position of the bullet.

Output


Hey, after firing once,
the bullet is not going back in the ready state. So it is not firing again. How to solve this?

Same images


B
Hint 1: Check the boundaries when we fire the bullet. The bullet's x and y-axis should neither be less than 0 nor more than the game window, 400x600.
Task: Fire the bullets when bulltes is in ready state.
Same images
Hint 3: Add a number for the y-axis to hide the bullet similar to x-axis as shown here.
if bulletState == "ready":
bullet.x=player.x+20
Hint 2: Change the state of bullet from fire to ready state after hitting the boundaries like bulletstate="ready"
#in place of "_" Write your number
if bullet.y< _ or bullet.x< _ or bullet.y>_ or bullet.x>_:

Output of Student Activity
Same images
if bulletState=="fired":
bullet.x ,bullet.y = newxy(bullet.x, bullet.y, 20 , bangle)
#change bullet state back to ready when it moves out
if bullet.y<0 or bullet.x<0 or bullet.y>600 or bullet.x>400:
bulletState="ready"
pygame.draw.rect(screen,(225,225,15),bullet)
i=0
if bulletState == "ready":
bullet.x=player.x+20
bullet.y=player.y+24



What will change for an object when its state changes?
Velocity
Life of object
Q.1

Behaviour
A
B
D
Object Type
C

When an object changes its state, its behaviour changes. Just like when we fire the bullet, it changes its state from ready to fire state.
1
Q
Suppose the window size of the game is 400x600.
The object should return after hitting the boundaries of the game window.
What would be the condition for the same?
object.y<0 or object.x<0 or object.y>600 or object.x>400
object.y>0 or object.x<0 or object.y>600 or object.x<400
object.y<0 or object.x>0 or object.y>600 or object.x<400
object.y>0 or object.x>0 or object.y<600 or object.x<400
A
B
C
D
Q 2
0 400
0 600



B
Hint:
1. Check the angle of the spaceship.
2. Prepare a new angle of the bullet. Depending angle of spaceship
3. call newxy(bullet.x, bullet.y, 20 , angle), with new angle.
Task: In the given student activity, we faced a problem that when the spaceship was rotating frequently and firing, the bullet was swinging. So how to resolve the problem?
Same images
Output :


ADDITIONAL ACTIVITY - 1


B
Same images

ADDITIONAL ACTIVITY - 1 SOLUTION
if bulletState == "ready":
bullet.x=player.x+20
bullet.y=player.y+24
bangle=angle
if bulletState=="fired":
bullet.x ,bullet.y = newxy(bullet.x, bullet.y, 20 , bangle)

Solution : https://bit.ly/3A5pYIy


B
Hint:
1.Create a list of time-table
2. run for loop and fetch time from time table list
3. check fetched data from timetable
4. Print appropriate message
Task: Create an application where the application will show time-table of daily routine of students.
Same images
ADDITIONAL ACTIVITY - 2




B
Same images
ADDITIONAL ACTIVITY - 2

ADDITIONAL ACTIVITY - 2
ADDITIONAL ACTIVITY - 2 SOLUTION
timetable=["21-5","6-7","8-15",'16-17','18-20','20-21']
for i in timetable:
if i=="21-5":
print("Sleeping Time "+i)
elif i=="6-7":
print("WakeUp Time "+i)
elif i=="8-15" :
print("School Time "+i)
elif i=="16-17":
print("Rest Time " +i)
elif i=="18-20":
print("Playing Time "+ i)
elif i=="20-21":
print("Home and Dinner Time "+ i)



Same images
Activity | Activity Name | Link |
---|---|---|
Teacher Activity 1 | Bullet Creation | |
Teacher Activity 2 | Bullet Firing | |
Student Template | Bullet Creation | |
AA1 | Bullet Swing | |
AA2 | Function firing | |
Links Table
Copy of G12_C6_New_For_Teacher
By anjali_sharma
Copy of G12_C6_New_For_Teacher
- 127