Debugging Like a Boss in Angular 9
Anthony Humes

This is going to rock!
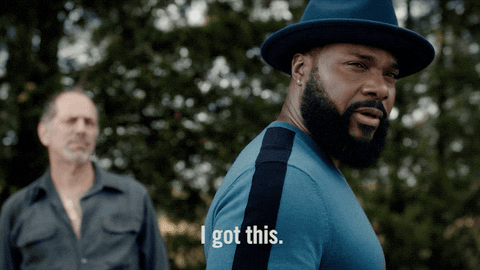
What happens next?
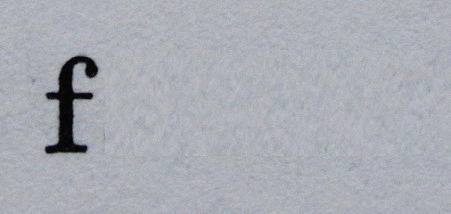
Why did this happen?
When critical production issues arose, it was hard to debug them effectively costing time and resources
What can we learn?
to build good apps, you must be able to debug them effectively
to debug effectively, you must be able to use all of your available tools
New API in Angular 9
Comes directly from Ivy internal methods

Where can I access the new methods?
// Global variable in the console
ng
{
getComponent: ƒ getComponent(element),
getContext: ƒ getContext(element),
getListeners: ƒ getListeners(element),
getOwningComponent: ƒ getOwningComponent(elementOrDir),
getHostElement: ƒ getHostElement(componentOrDirective),
getInjector: ƒ getInjector(elementOrDir),
getRootComponents: ƒ getRootComponents(elementOrDir),
getDirectives: ƒ getDirectives(element),
applyChanges: ƒ applyChanges(component)
}
Before we start...what is $0?
$0
<p class="some-element"></p>
<div class="some-div">
<p class="some-element">
Hey there text!
</p>
</div>
most recent selection from the Elements Inspector or from using Inspect Element
Get Angular Elements
ng.getComponent
ng.getDirectives
ng.getListeners
ng.getComponent
ng.getDirectives
ng.getListeners
retrieves the component from an HTML element
retrieves an array of directives from an HTML element
returns an array of both html (ex. click) and host listeners for an HTML element
ng.getComponent
<app-parent class="some-div">
<app-child class="some-element">
Hey there text!
</app-child>
</app-parent>
ng.getComponent($0)
ChildComponent {...}
ng.applyChanges
let component = ng.getComponent($0)
component.value = 10;
ng.applyChanges($0)
<app-element class="some-div">
<p class="some-element">
5
</p>
</app-parent>
<app-element class="some-div">
<p class="some-element">
10
</p>
</app-parent>
triggers change detection for the component or directive
ng.getOwningComponent
ng.getContext
ng.getOwningComponent
returns the parent component for the HTML element
ng.getContext
returns the context of an *ngIf or *ngFor for the HTML element
Other Methods
ng.getRootComponents
ng.getInjector
What these APIs won't do?
- Replace using breakpoints in Chrome
- Debug interactions between components
- Debug complex state issues with Observables or NgRx
- Magically fix every problem in your app
Note from the Angular Team
The current API is a work in process. These methods are the starting point to a way more powerful set of debug tools than ng.probe. More methods and better functionality will be coming in the future.
Demo
Next Steps
- Experiment with the new methods
- Spend some time pair programming with another developer
- Help someone in the community with a problem they can't solve
- Always keep learning!
Thank You!
Debugging Like a Boss in Angular 9
By Anthony Humes
Debugging Like a Boss in Angular 9
- 2,596