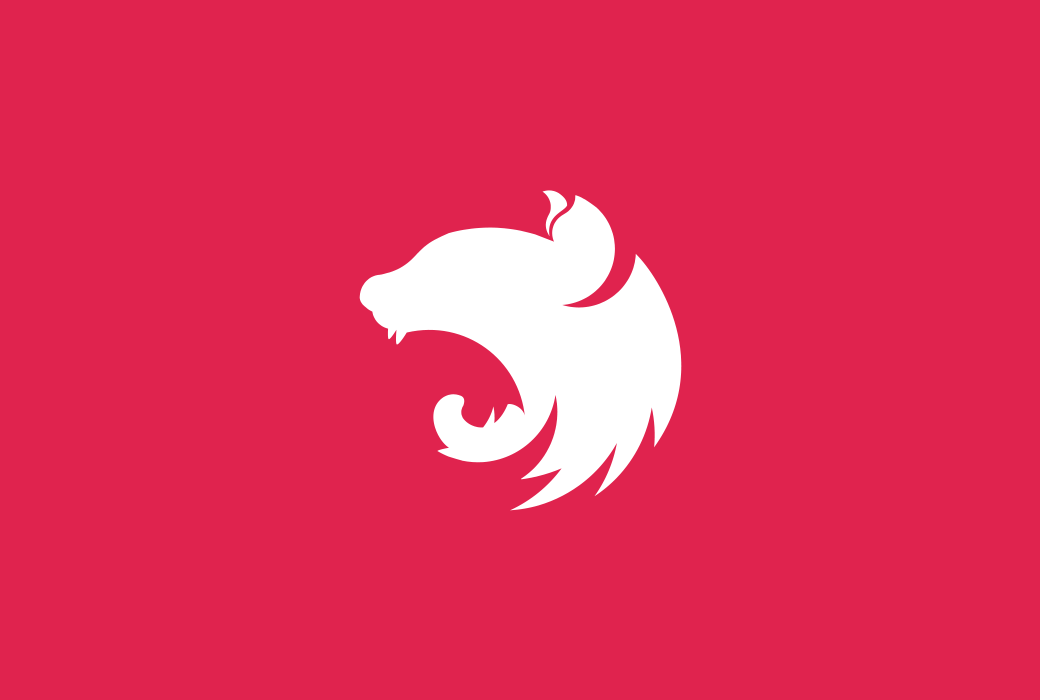
@AurelienLoyer && @EmmanuelDemey
@NestJS
NestJS
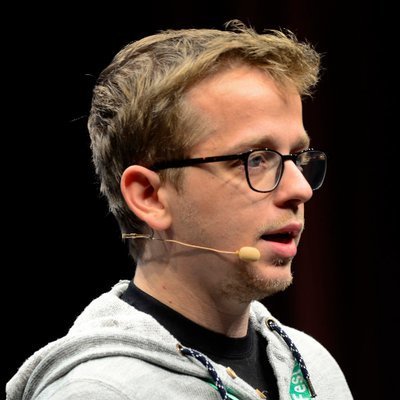
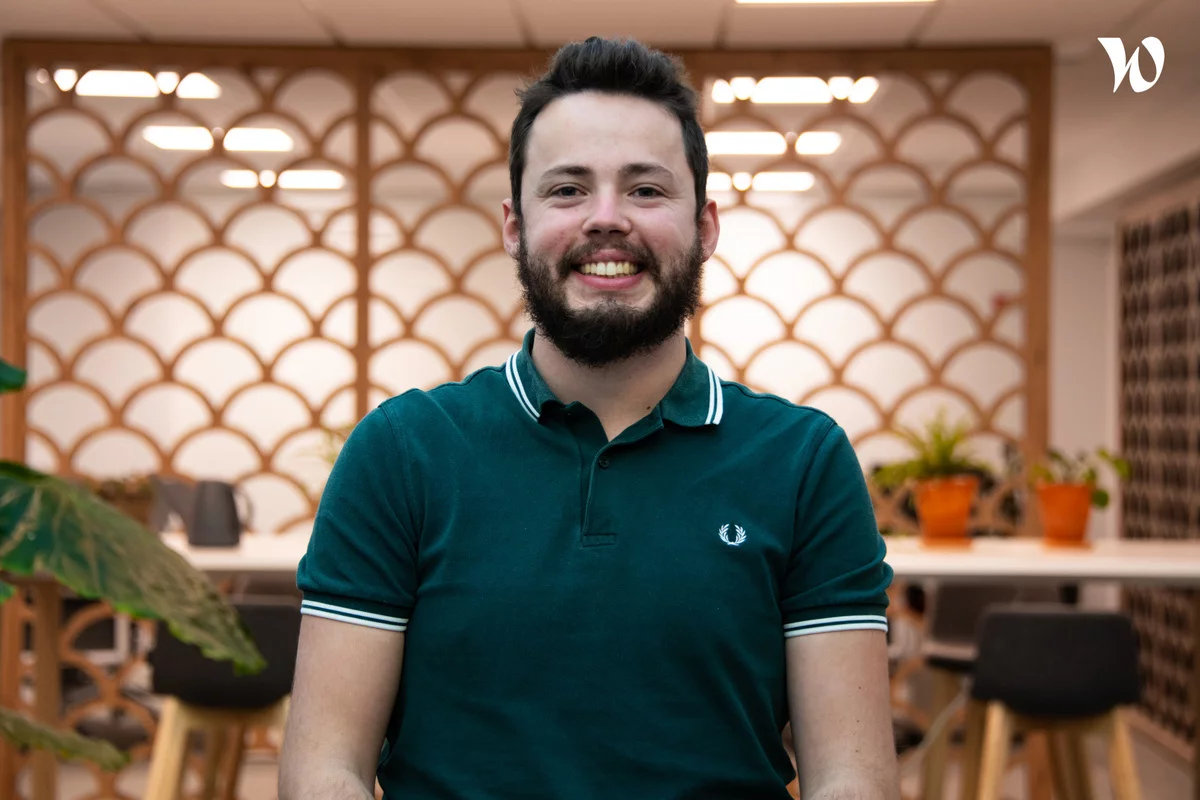
Emmanuel DEMEY
@EmmanuelDemey
Consultant WEB
AurΓ©lien LOYER
@AurelienLoyer
Software Engineer @QIMA
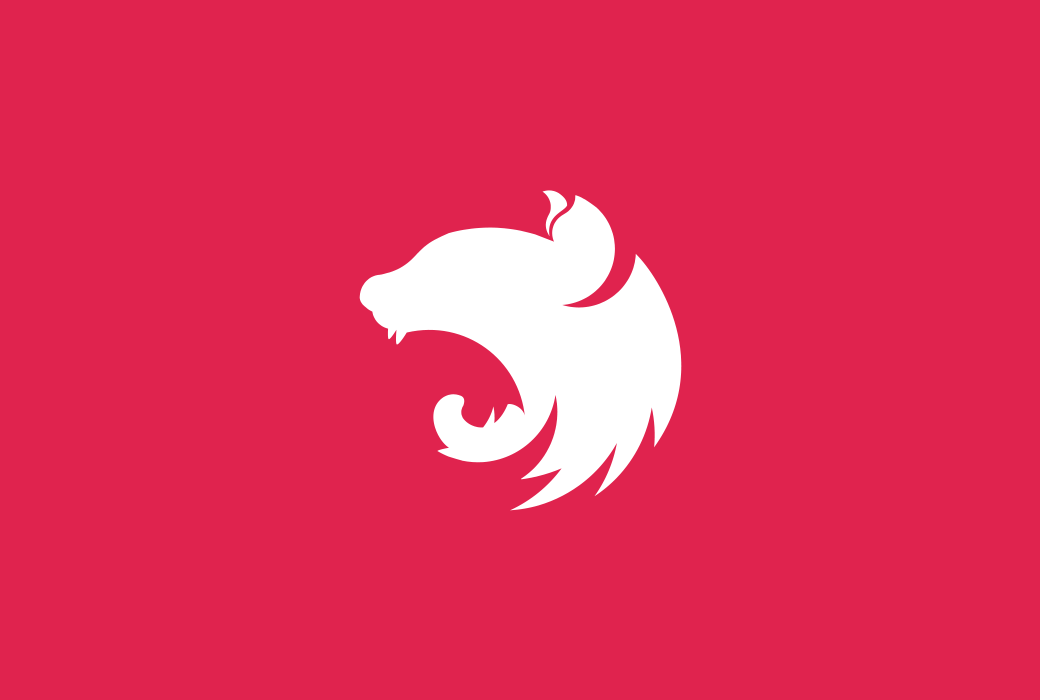
NestJS
You all already know NestJS or have heard of them!
Or maybe you just know Angular ?!
NestJS
overview in
~10 minutes !!
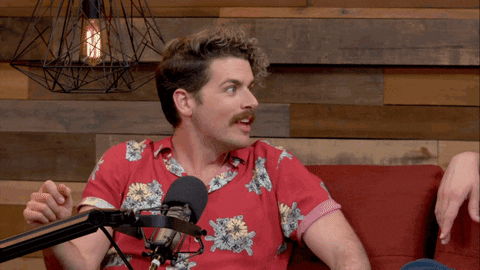
Nest (NestJS) is a framework for building efficient, scalable Node.js server-side applications
-
Node.js
-
TypeScript
-
Express or Fastify
- "Plugins": GraphQL, WebSockets, ...
- Inspired by the Angular framework
- An awesome CLI π
npm install -g @nestjs/cli nest new my-nest-project // OR npx @nestjs/cli new my-nest-project
@nestjs/cli
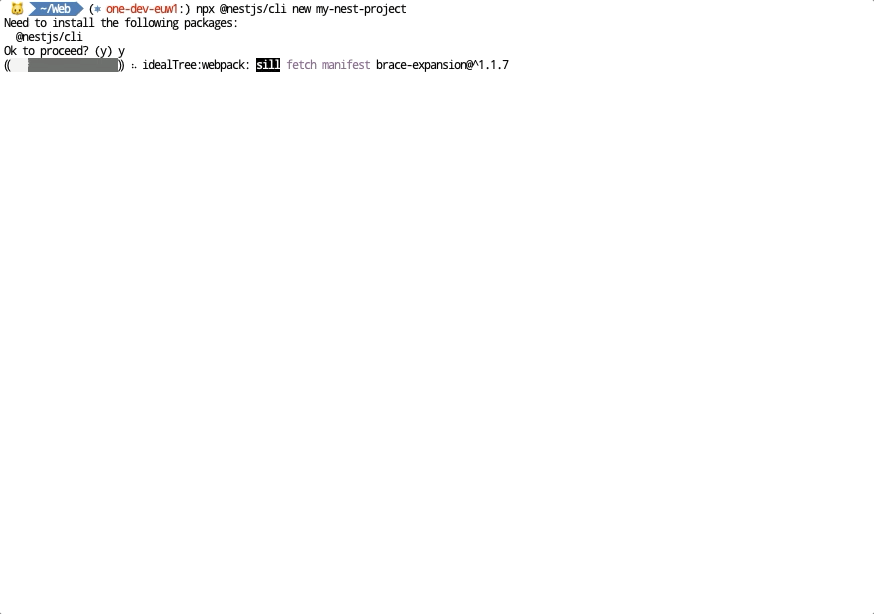
@nestjs/cli new app
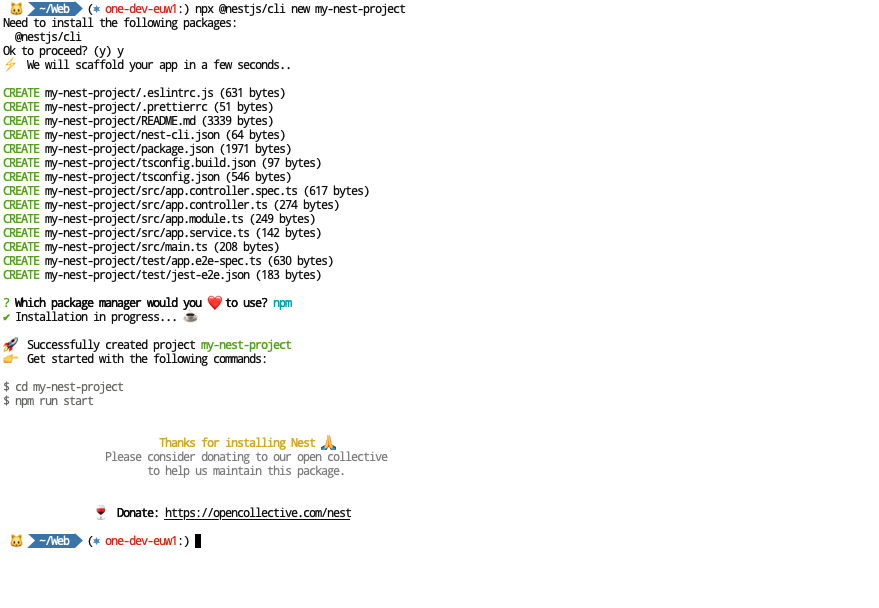
@nestjs/cli new app
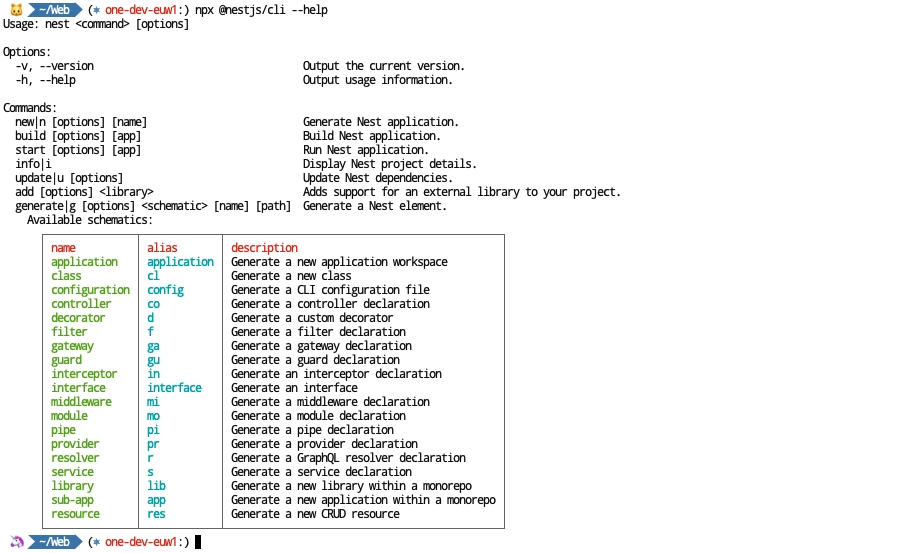
@nestjs/cli --help
@nestjs Project Structure
. |-- node_modules |-- src | |-- app.controller.ts | |-- app.module.ts | |-- app.service.ts | `-- main.ts |-- nest-cli.json |-- package.json |-- tsconfig.json `-- .eslintrc.js
. |-- node_modules |-- src | |-- app.controller.ts | |-- app.module.ts | |-- app.service.ts | `-- main.ts |-- nest-cli.json |-- package.json |-- tsconfig.json `-- .eslintrc.js
@nestjs main.ts
import { NestFactory } from '@nestjs/core'; import { AppModule } from './app.module'; async function bootstrap() { const app = await NestFactory.create(AppModule); await app.listen(3000); } bootstrap();
import { NestFactory } from '@nestjs/core'; import { AppModule } from './app.module'; async function bootstrap() { const app = await NestFactory.create(AppModule); await app.listen(3000); } bootstrap();
-
Bootstrap our application
-
Main module definition
-
Port
-
And more...
@nestjs Controllers
import { Controller, Get } from '@nestjs/common'; @Controller('plants') export class PlantsController { @Get() findAll(): string { return 'This action returns all plants'; } }
import { Controller, Get } from '@nestjs/common'; @Controller('plants') export class PlantsController { @Get() findAll(): string { return 'This action returns all plants'; } }
-
Use classes and decorators
-
Incoming requests
-
Returning responses
-
CRUD
@nestjs Providers
import { Injectable } from '@nestjs/common'; import { Plant } from './interfaces/plant.interface'; @Injectable() export class PlantsService { private readonly plants: Plant[] = []; create(plant: Plant) { this.plants.push(plant); } //.. }
import { Injectable } from '@nestjs/common'; import { Plant } from './interfaces/plant.interface'; @Injectable() export class PlantsService { private readonly plants: Plant[] = []; create(plant: Plant) { this.plants.push(plant); } //.. }
-
Use classes and decorators
-
Point of contact with the data
-
Injected as dependency
constructor(private catsService: CatsService) {}
@nestjs Modules
import { Module } from '@nestjs/common'; import { PlantsController } from './plants.controller'; import { PlantsService } from './plants.service'; @Module({ controllers: [PlantsController], providers: [PlantsService], exports: [PlantsService], }) export class PlantsModule {}
import { Module } from '@nestjs/common'; import { PlantsController } from './plants.controller'; import { PlantsService } from './plants.service'; @Module({ controllers: [PlantsController], providers: [PlantsService], exports: [PlantsService], }) export class PlantsModule {}
-
Use classes and decorators
-
Organize the application structure
-
Controllers / Providers / Exports
@nestjs ...
@Injectable() export class LoggerMiddleware implements NestMiddleware { use(req: Request, res: Response, next: NextFunction) { console.log('Request...'); next(); } }
@Injectable() export class LoggerMiddleware implements NestMiddleware { use(req: Request, res: Response, next: NextFunction) { console.log('Request...'); next(); } }
-
Middleware
@Get(':id') async findOne(@Param('id', ParseIntPipe) id: number) { return this.catsService.findOne(id); }
@Get(':id') async findOne(@Param('id', ParseIntPipe) id: number) { return this.catsService.findOne(id); }
-
Pipes
-
Pipes
-
Guards
-
Interceptors
-
...
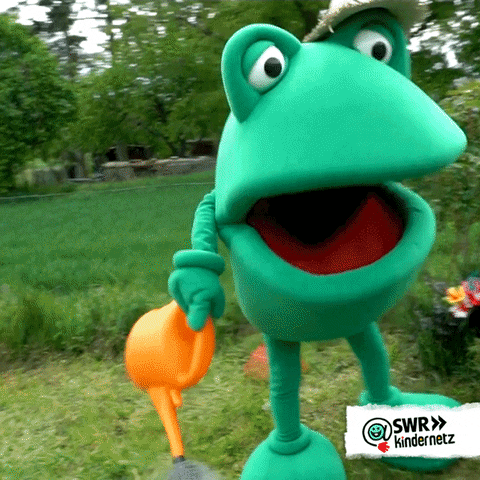
π¨π»βπ» Live Coding Session!
Gardener!
@AurelienLoyer && @EmmanuelDemey @NestJS NestJS
NestJS - Level up !
By Aurelien Loyer
NestJS - Level up !
- 932