Javascript high performance
Jan 30th, 2014

AGENDA
- Why?
- When?
- How?
- Load Time
- Data Storage
- DOM Scripting
- Algorithms and Flow Control
- Strings
- Tools
______________________________________________
Javascript High Performance

PRerequisites
- Too Late! No one is leaving!
- But if you insist, Javascript.
______________________________________________
Javascript High Performance

WHY?
- How many times did you give up browsing through a page because it was too slow?
- Browser's optimization won't ever handle everything
- Each day, a new kind of device gets access to the internet.
- Battery life
- Response time
______________________________________________
Javascript High Performance

WHEN?
-
Before your code is ready
- But in what kind of applications?
- All of them.
-
Most optimizations have no additional cost
- What if my code is done already?
- Effort
- Risk
- Benefit
______________________________________________
Javascript High Performance

HOW?
- Watch the rest of this presentation
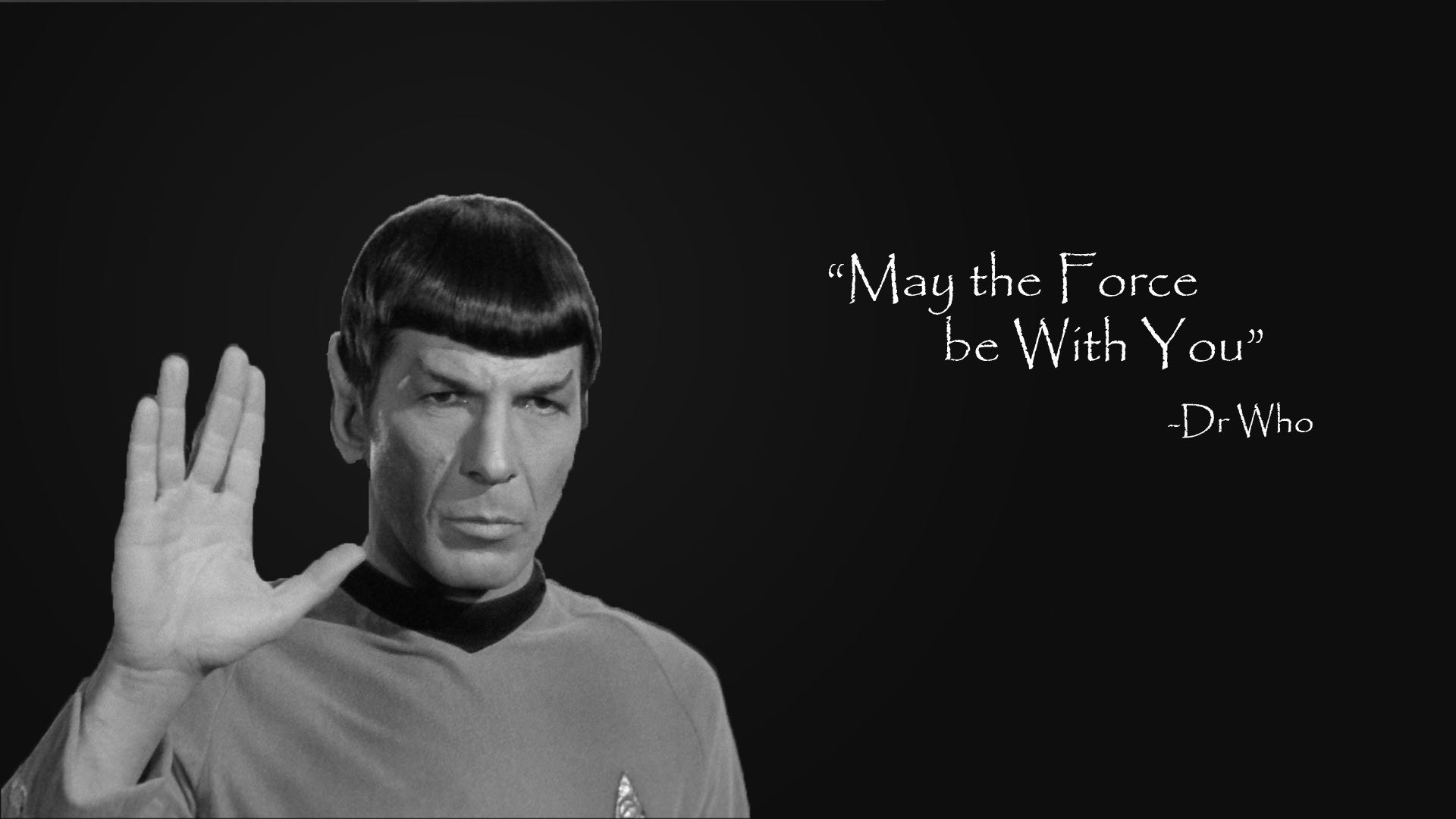
______________________________________________
Javascript High Performance

DAta Load

-
Put scripts at the bottom.
- a script tag blocks rendering
-
defer attribute
- Load scripts together.
- 1 x 100kb is faster than 4 x 25kb
- Concatenate and minify.
- Extra: Use Sprites to combine Images
______________________________________________
Javascript High Performance

DATA LOAD
______________________________________________
Javascript High Performance

DATA STORAGE
- Where should we store our data?
- Variables and objects
- Local variables are the fastest!
- Avoid very long dot notations
______________________________________________
Javascript High Performance

DATA STORAGE


______________________________________________
Javascript High Performance

Data Storage
- Scope
- Scope chain
- avoid with, try-catch and eval()

______________________________________________
Javascript High Performance

DOM SCRIPTING
- DOM what?
- DOM = Document Object Model
-
The main library you're going to use to navigate through and modify elements of the page.
- A separate library from javascript itself.
______________________________________________
Javascript High Performance

DOM SCRIPTING


______________________________________________
Javascript High Performance

DOM SCRIPTING
- HTML collections can be expensive to use.


______________________________________________
Javascript High Performance
DOM SCRIPTING
______________________________________________
Javascript High Performance


DOM SCRIPTING
______________________________________________
Javascript High Performance


DOM SCripting
- Query Selectors
- Can save up a lot of time on complex queries
- Slower than simple getElementById
- Let's say you want all divs with 'warning' and 'notice' classes.
______________________________________________
Javascript High Performance

DOM SCRIPTING
- QuerySelectors provide a simpler and faster way

______________________________________________
Javascript High Performance

DOM Scripting
- Repaint / Reflow
- Repaint = Drawing pieces of the page that changed
- Reflow = Recalculation of the render tree
- Both are very expensive operations
- How to avoid?
- Use CSS classes instead of inline styles
- Avoid getting/setting layout informations multiple times. Same reason you should avoid length on an HTML Collection.
______________________________________________
Javascript High Performance

DOM SCRIPTING



______________________________________________
Javascript High Performance

DOM SCRIPTING
- Event Delegation
- How bad can this be?
- You could have hundreds or events in one page
- Or just 1.

______________________________________________
Javascript High Performance

DOM SCRIPTiNG

______________________________________________
Javascript High Performance

Algorithms and flow control
- Loops
- regular loops are the fastest ones
- for
- while
- do-while
- for-in loops can be 7 times slower than the regular ones
- function based iterations like forEach can be useful but should also be avoided
______________________________________________
Javascript High Performance

Algorithms and flow control
- How to optimize loops?
- reverse the iterator
- cache the length

______________________________________________
Javascript High Performance

Algorithms and flow control

______________________________________________
Javascript High Performance

Algorithms and flow control
- if-else vs switch
- if-else when there are only a few conditions
- for large number of conditions use switch
- how to optimize ifs?
- Put the most likely scenario first.
______________________________________________
Javascript High Performance

Algorithms and flow control


______________________________________________
Javascript High Performance

Algorithms and flow control
- But if you can use lookup tables...

______________________________________________
Javascript High Performance

Strings
- What's the best way to do String operations?
- Keep it simple and just use + and +=
- Very optimized by browsers but still expensive
- Try to do as few as possible.
- forget about String.prototype.concat
- forget about array joining.
______________________________________________
Javascript High Performance

TOOLS
- Some of the ones I liked the most
- http://jsperf.com/if-else-vs-switch-test
- chrome profiler
- YSlow
______________________________________________
Javascript High Performance

Conclusion
- Knowing how to optimize your code can make a big difference.
- My advice?

______________________________________________
Javascript High Performance

Learn more
- http://www.amazon.com/Performance-JavaScript-Faster-Application-Interfaces/dp/059680279X
- http://jonraasch.com/blog/10-javascript-performance-boosting-tips-from-nicholas-zakas
- https://developers.google.com/speed/articles/optimizing-javascript
- www.google.com
______________________________________________
Javascript High Performance

CHALLENGE
______________________________________________
Javascript High Performance

Javascript High Performance
By Avenue Code
Javascript High Performance
Learn to make your web application faster with a better response time.
- 3,305