First Example in Java
Advanced Programming
SUT • Spring 2019
Outline
-
Review
-
First program in java
-
Variables
-
Methods
-
Conditions
-
Loops
First Example
Review
-
Java is
-
Simple
-
object oriented
-
Robust
-
And popular
-
-
Java is platform independent.
-
Write Once, Run Anywhere!
-
First program in java
-
Create a file named First.java
-
Java class files have .java extension
-
Note to naming convention
-
-
Copy this lines to the file
-
Note: File name and class name should be the same.
-
public class First{
public static void main(String[] args){
System.out.println("Hello World!");
}
}
First Example
- Run javac First.java
- Run java First
- We don’t use any IDE now.
- To highlight compile and run stages.
- Lets watch it in real world!
behnam@behnam-UX430UNR:~$ mkdir test
behnam@behnam-UX430UNR:~$ cd test/
behnam@behnam-UX430UNR:~/test$ cat > First.java
public class First{
public static void main(String[] args){
System.out.println("Hello World!");
}
}
behnam@behnam-UX430UNR:~/test$ ls -lah
total 12K
drwxr-xr-x 2 behnam behnam 4.0K فوریه 4 10:55 .
drwxr-xr-x 22 behnam behnam 4.0K فوریه 4 10:55 ..
-rw-r--r-- 1 behnam behnam 116 فوریه 4 10:56 First.java
behnam@behnam-UX430UNR:~/test$ javac First.java
behnam@behnam-UX430UNR:~/test$ java First
Hello World!
behnam@behnam-UX430UNR:~/test$
Java Programs
-
A simple java program is a file
-
The file contains one class
-
-
The class name equal to the file name
-
The names are case sensitive
-
-
The class contains a main method
-
When we run the program, the main method is executed
Variables
Variables
- What is a variable?
- A piece of memory
- Holds data
- For example a number, string or Boolean
- Java variables have a fixed size
- Platform independence
Java Primitive Types
Primitive type | Size | Min | Max | Wrapper Type |
---|---|---|---|---|
boolean | - | - | - | Boolean |
char | 16 bits | Unicode 0 | Unicode 2^16-1 | Character |
byte | 8 bits | -128 | 127 | Byte |
short | 16 bits | -2^15 | 2^15-1 | Short |
int | 32 bits | -2^31 | 2^31-1 | Integer |
long | 64 bits | -2^63 | 2^63-1 | Long |
float | 32 bits | IEEE754 | IEEE754 | Float |
double | 64 bits | IEEE754 | IEEE754 | Double |
void | - | - | - | Void |
Java Primitive Types
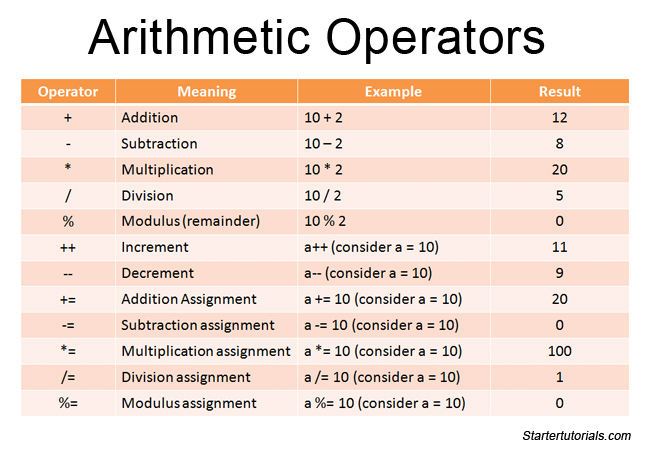
Operator Precedence

Equality and Relational Operators
Operator | Java | Sample | meaning |
---|---|---|---|
= | == | x == y | x is equal to y |
≠ | != | x != y | x is not equal to y |
> | > | x > y | x is greater than y |
< | < | x < y | x is less than y |
≥ | >= | x >= y | x is greater than or equal to y |
≤ | <= | x <= y | x is less than equal y |
Associativity
- When two operators with the same precedence the expression is evaluated according to its associativity.
- x = y = z = 17
- is treated as x = (y = (z = 17))
- since the = operator has right-to-left associativity
- 72 / 2 / 3
- is treated as (72 / 2) / 3
- since the / operator has left-to-right associativity
A simple program
public class SimpleProgram{
public static void main(String[] args){
int a;
a = 12;
a += 2;
int b;
b = 4;
b++;
b = a*b;
System.out.println(b);
}
}
Example
- A method is like a machine
- Zero or more inputs
- Zero or one output
- Other names
- Function
- Procedure
method
inputs
output
Example
public class Example{
static double add(double a, double b){
double result = a + b;
return result;
}
public static void main(String args[]){
double x = 3;
double y = 4;
double add = add(x,y);
System.out.println(add);
}
}
Parameter Passing
-
Local variables
-
Java passes the parameters by value
public class ParameterPassing{}
public static void main(String[] args) {
double x = 3;
double y = 4;
double add = add(x,y);
System.out.println(add);
System.out.println(x);
}
static double add(double a, double b){
a = a + b;
return a;
}
}
Conditions
public class Main{
public static void main(String[] args){
int x = 2;
int y = 3;
if(x>y){
System.out.println("X is greater than Y");
} else if(x==y){
System.out.println("X is equal to Y");
} else {
System.out.println("Y is greater than X");
}
boolean condition = x>y;
if(condition){
System.out.println("X is greater than Y");
}else{
System.out.println("Y >= X");
}
}
}
Loops
public class Loop{
public static void main(String[] args){
int counter = 0;
while(counter < 10){
counter++;
System.out.println(counter);
}
counter = 0;
do{
counter++;
System.out.println(counter);
}while(counter < 10);
for (int i = 1; i <= 10; i++) {
System.out.println(i);
}
}
}
For Loop vs. While Loop
for (X; Y; Z) {
body();
}
X;
while(Y){
body();
Z;
}
goto
-
goto is a reserved word in java
-
But forbidden!
Title Text

First Example in Java
By Behnam Hatami
First Example in Java
First Example in java / Advanced Programming Course @ SUT, Spring 2019
- 1,130