Functional
Programming
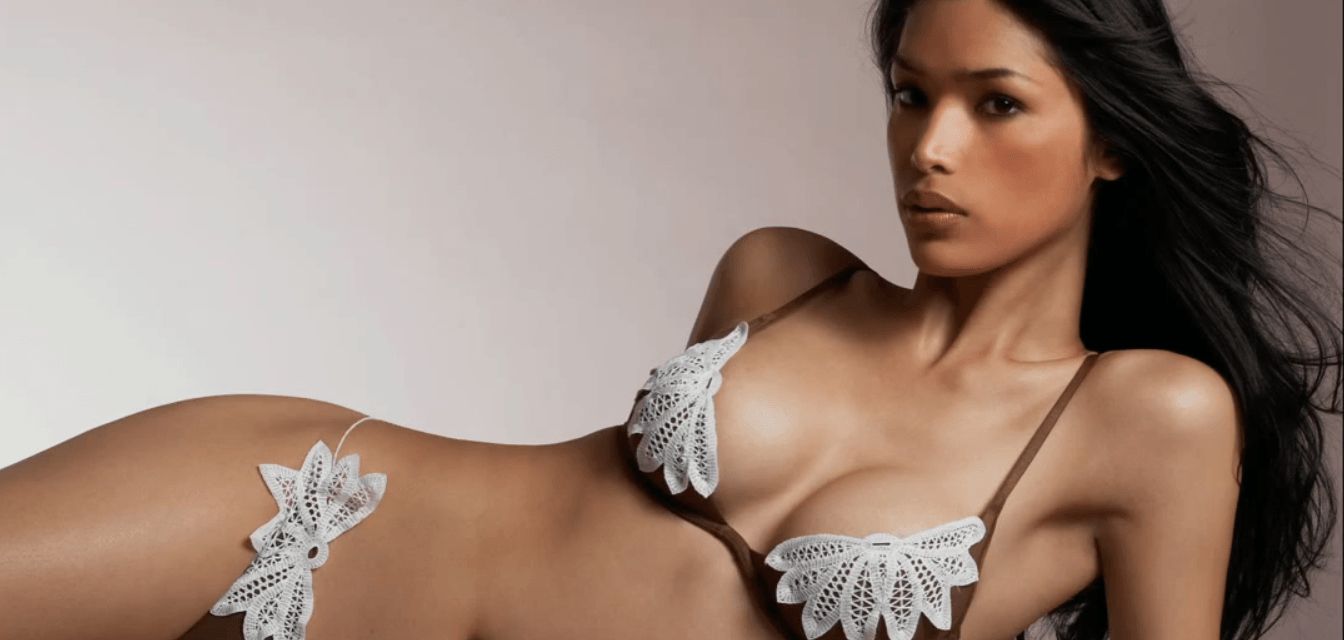
The sexy way to code!
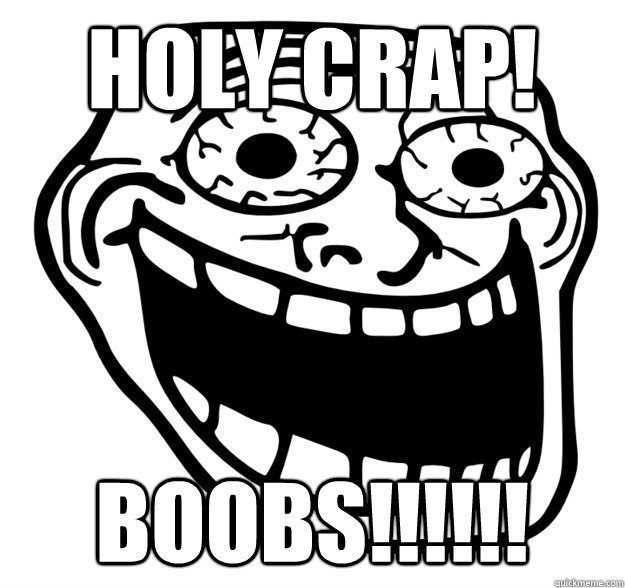
Imperative
Line by line
Input: values
Output: values
Modify memory registers/RAM
Functional
Definitions
Substitutions
e.g y(x) = x + 1
then y(y(x)) = (x + 1) + 1
Immutable values
State and Change
Do this, then that..
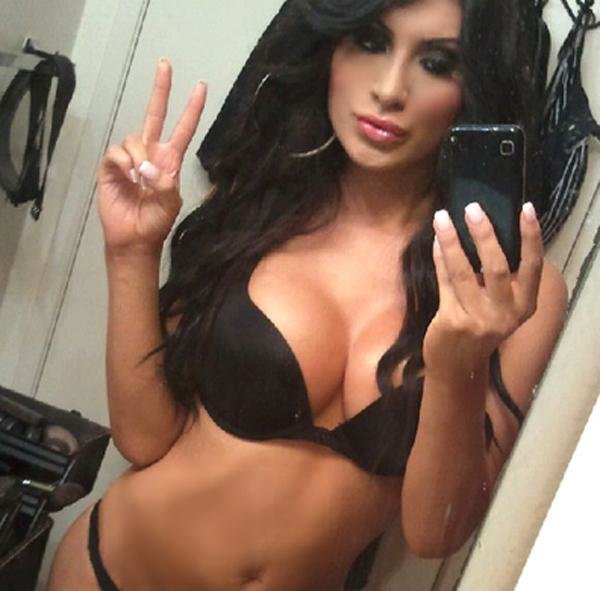
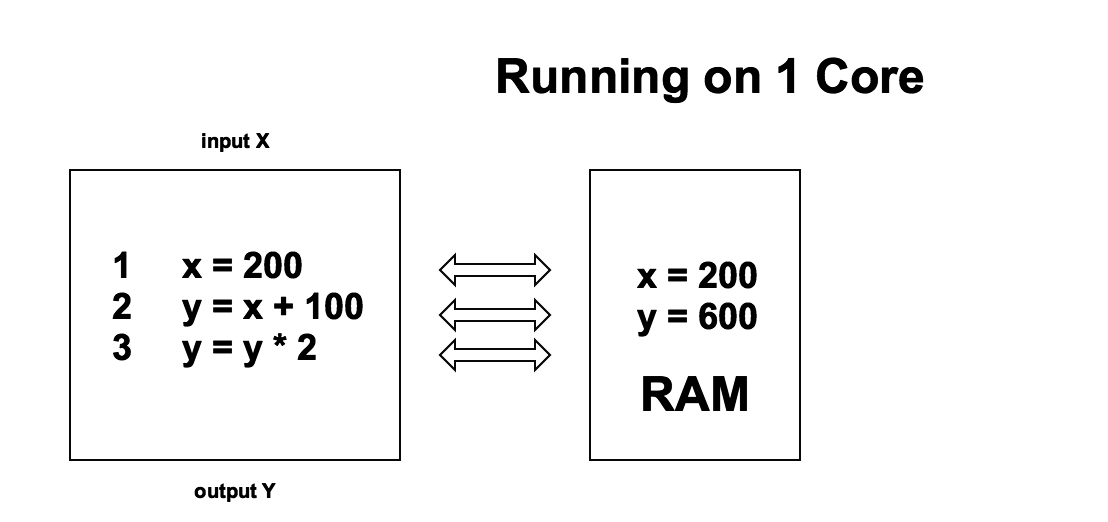
Line by line code
This was a great improvement on the
machine code that preceded it !
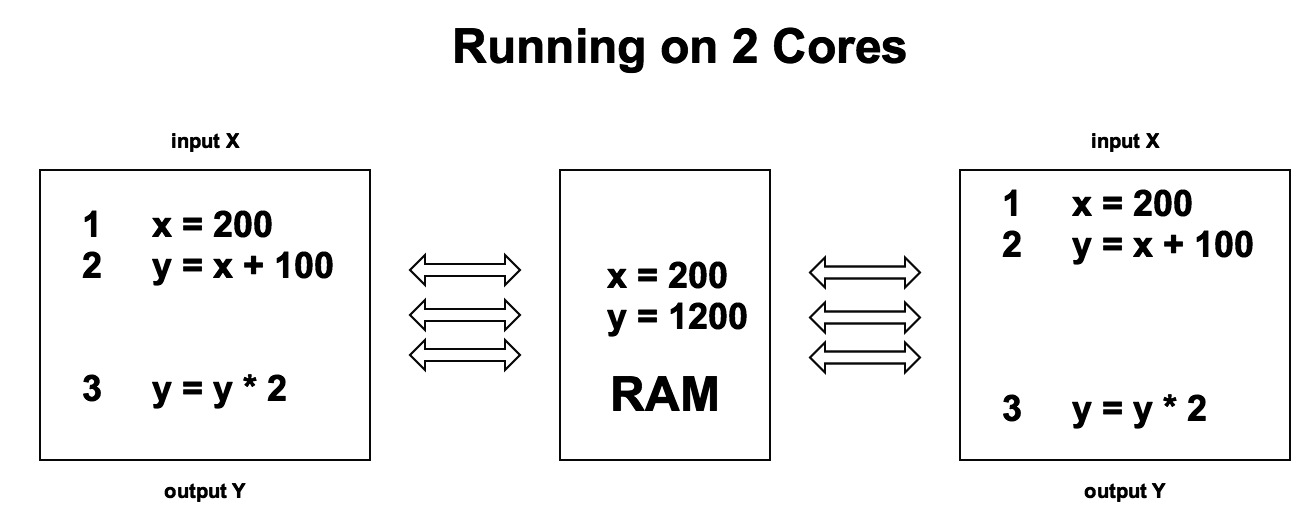
Bugs come from the assumption :
lines above the current one have done things in RAM that are needed
How Bugs Happen..
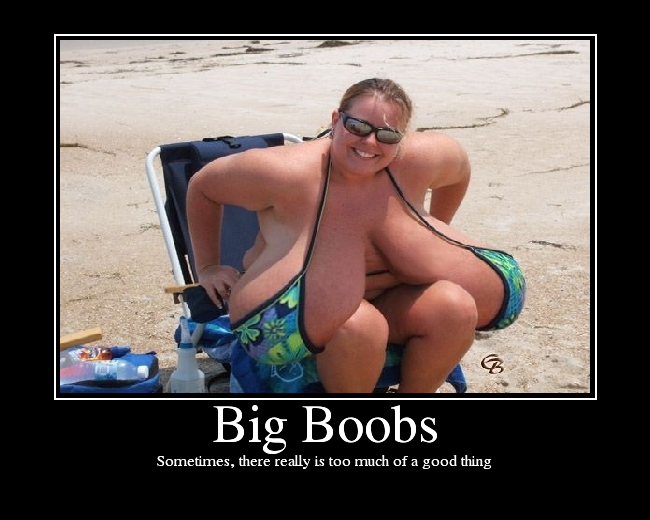
Pattern Matching
The 'Declarative' Way
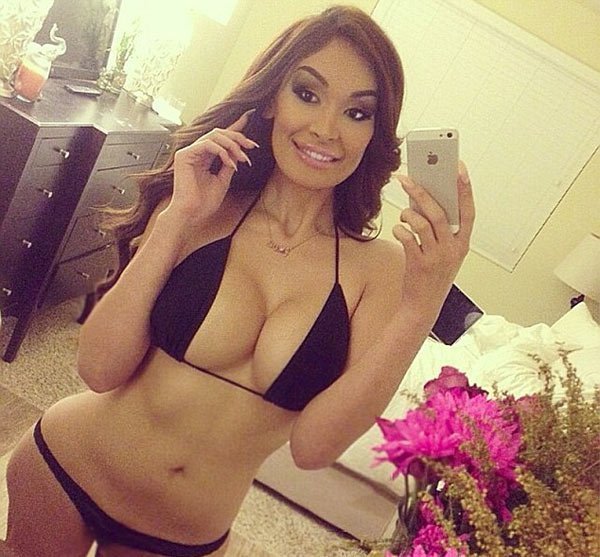
Declare the substitutions that can occur..
let secondElementFunction (a:(b:_)) = b
> [1..]
1,2,3,4,5,6,7,8,9,10,11,12,13......
Infinite Sequences!
> secondElement ( [1..] )
2
Can our function handle infinity?!
Lazy Evaluation
- Just make one code substitution
- Dont have to evaluate everything!
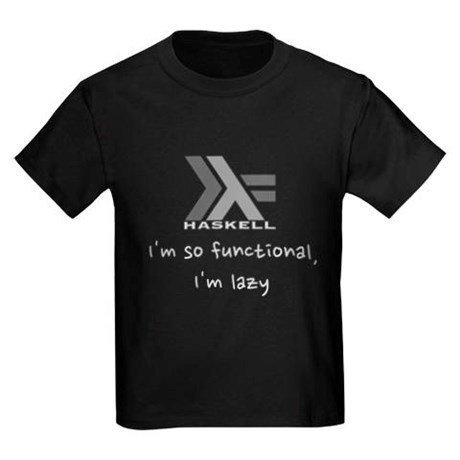
Functional Composition
Life after 'line-by-line'
'First-Class' Functions
or 'Lambdas'
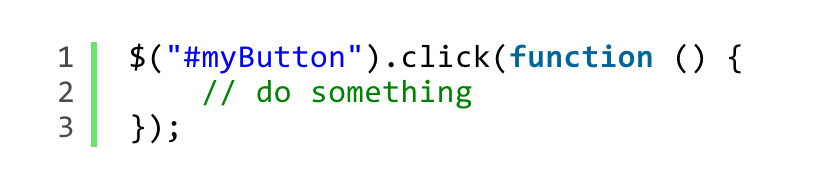
Just means to be able to
pass functions as parameters
Functional Composition
asyncCall()
.then(function(data1){
// do something...
return anotherAsyncCall();
})
.then(function(data2){
// do something...
return oneMoreAsyncCall();
})
.then(function(data3){
// the third and final async response
})
.fail(function(err) {
// handle any error resulting from any of the above calls
})
.done();
The alternative to line by line control flow
Declare how functions feed into each other rather than using: shared memory, parameters and return values
Immutability
No mutants allowed!
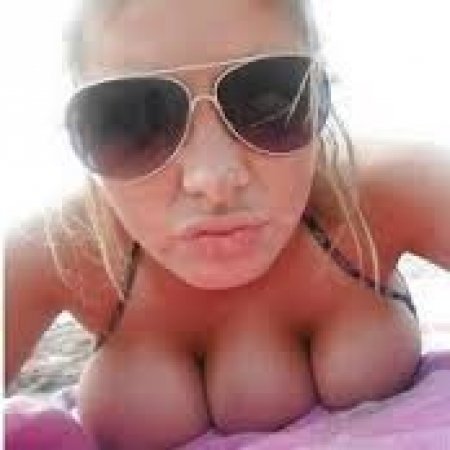
- Have to copy/patch to change
- Garbage collection
- What about I/O?! (argh MONADS!)
Pros
Cons
- No "Side effects"
- Threadsafe / parallelisable
- Well defined contracts
No Memory Modifications
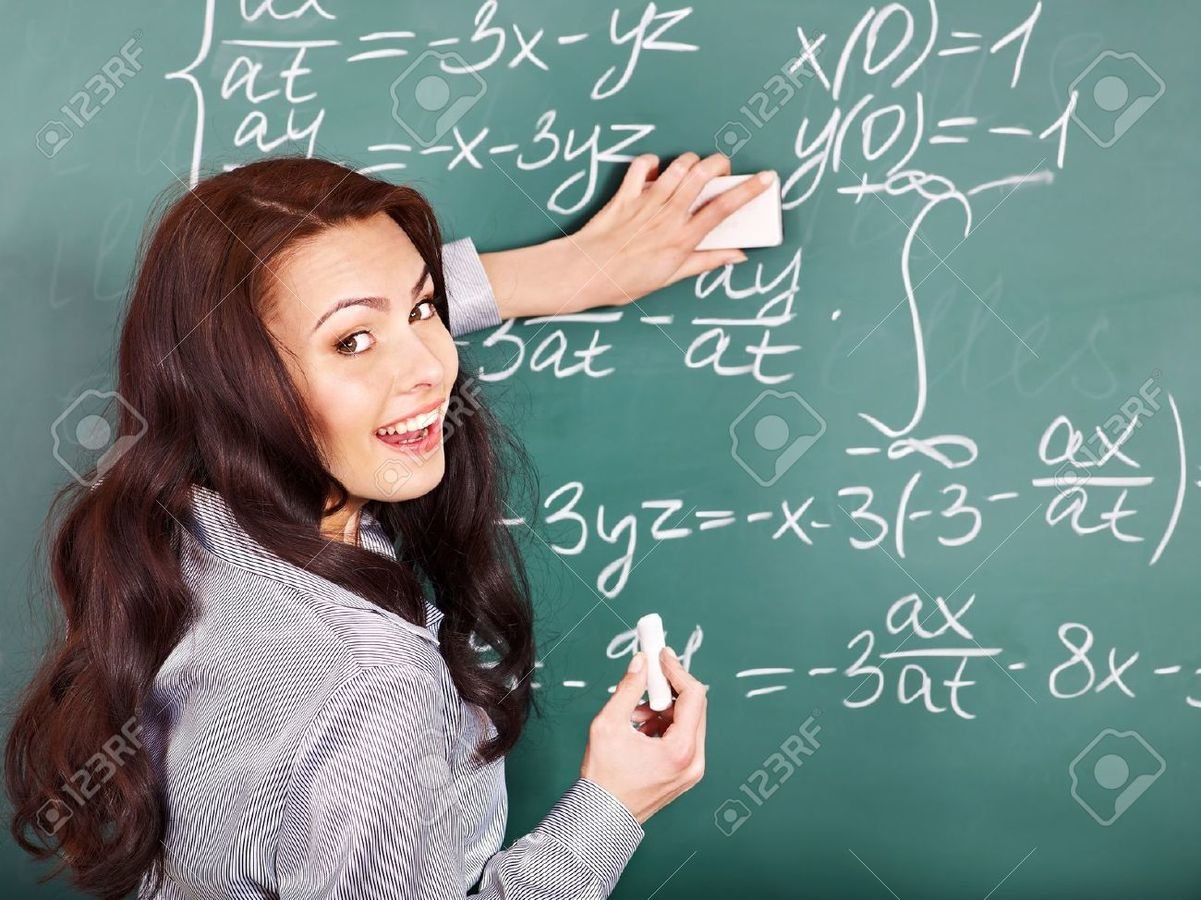
Streaming
To Infinity and Beyond!
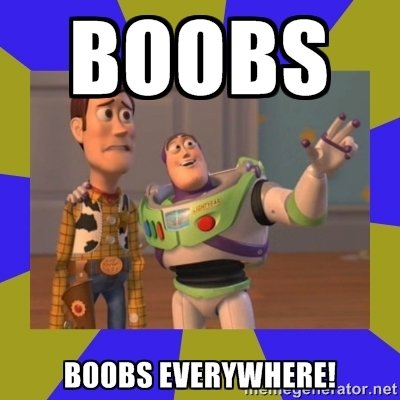
Substitution vs Iteration
Because we can substitute a list with
its head and then 'the rest' we can reason
about INFINITE data structures!
Reactive
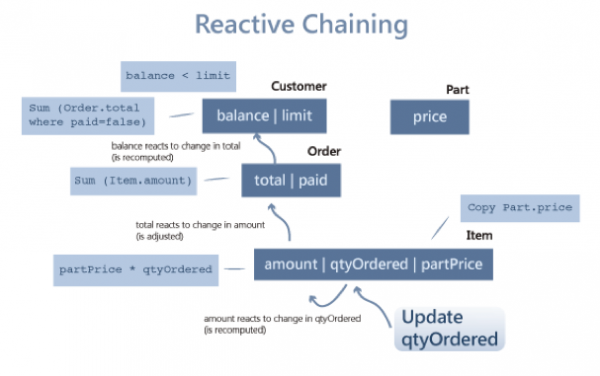
Sorry!
I wanted to give more examples in PHP
Here are some references..
Sorry!
Most of the ladies in this presentation are transexuals
PHP References
- http://code.tutsplus.com/tutorials/functional-programming-in-php--net-35043
- http://www.php5dp.com/php-functional-programming-part-ii-oop-immutable-objects/
- http://www.garfieldtech.com/blog/functional-php-tour
- https://www.palantir.net/madison-php-2014
- http://www.sitepoint.com/functional-programming-and-php/
- http://www.garfieldtech.com/presentations/dcmunich2012-fp/#1
Functional Programming
By Ben Ritchie
Functional Programming
- 226