Adapting to Change
JSDC Taiwan 2016 / #jsdc2016 / @blakenewman
Plan A

Plan V

By Blake Newman
Front-end please stop!
Frameworks...
Task Runner tools...
Build tools...
All the things™...

JSDC Taiwan 2016 / #jsdc2016 / @blakenewman
Imperative JavaScript
Declarative CSS
HTML
JSDC Taiwan 2016 / #jsdc2016 / @blakenewman
JavaScript is Fragile
JSDC Taiwan 2016 / #jsdc2016 / @blakenewman
Keeping in control
Sacrificial Architecture
Development experience
JSDC Taiwan 2016 / #jsdc2016 / @blakenewman
Dependency Management done wrong:
{
"name": "sample-package-json",
"dependencies": {
"babel-runtime": "^6.0.0",
"vue": "^1.0.0",
"vue-resource": "^0.8.0",
"vue-router": "^0.7.0",
"vuex": "^0.6.0",
"vuex-router-sync": "^1.0.0"
}
}
https://docs.npmjs.com/misc/semver
JSDC Taiwan 2016 / #jsdc2016 / @blakenewman
The problem with `Caret Ranges`
^1.0.0
1.0.0 <= 2.0.0
=
JSDC Taiwan 2016 / #jsdc2016 / @blakenewman
Use the Direct Version
1.0.17
1.0.17
=
JSDC Taiwan 2016 / #jsdc2016 / @blakenewman
Keep in control
$ npm i -g npm-check-updates
$ ncu
eslint 2.12.0 → 2.13.1
vue-hot-reload-api 1.3.1 → 1.3.2
JSDC Taiwan 2016 / #jsdc2016 / @blakenewman
Sacrificial Architecture
JSDC Taiwan 2016 / #jsdc2016 / @blakenewman
A
B
C
JSDC Taiwan 2016 / #jsdc2016 / @blakenewman
A
B
C
JSDC Taiwan 2016 / #jsdc2016 / @blakenewman
A
B
C
JSDC Taiwan 2016 / #jsdc2016 / @blakenewman
B
A
B
C
Store
State A
State C
JSDC Taiwan 2016 / #jsdc2016 / @blakenewman
A
C
Store
State A
State C
JSDC Taiwan 2016 / #jsdc2016 / @blakenewman
Scalability

https://addyosmani.com/scalablejs/
JSDC Taiwan 2016 / #jsdc2016 / @blakenewman
Maintainability

JSDC Taiwan 2016 / #jsdc2016 / @blakenewman
Modularisation
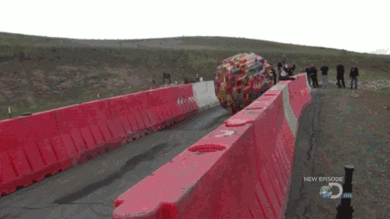
JSDC Taiwan 2016 / #jsdc2016 / @blakenewman
JSDC Taiwan 2016 / #jsdc2016 / @blakenewman
LIFT Principles
JSDC Taiwan 2016 / #jsdc2016 / @blakenewman
Locate
JSDC Taiwan 2016 / #jsdc2016 / @blakenewman
Identify
JSDC Taiwan 2016 / #jsdc2016 / @blakenewman
Flat
JSDC Taiwan 2016 / #jsdc2016 / @blakenewman
T-DRY
(Try to Stick to DRY)
JSDC Taiwan 2016 / #jsdc2016 / @blakenewman
Problems are with Frameworks
JSDC Taiwan 2016 / #jsdc2016 / @blakenewman
Choose your framework as if you will need to change it after six months
JSDC Taiwan 2016 / #jsdc2016 / @blakenewman
Don't be a slave to Frameworks
JSDC Taiwan 2016 / #jsdc2016 / @blakenewman
// getUsers factory
getUsers.$inject = ['$http'];
function getUsers($http) {
return $http.get('api/v1/users').then(function (response) {
return response.data;
});
}
app.factory('getUsers', getUsers);
// ----
// Home controller
homeController.$inject = ['$scope', 'getUsers'];
function homeController($score, getUsers) {
getUsers().then(function (users) {
$scope.users = users;
});
}
app.controller('home', homeController);
JSDC Taiwan 2016 / #jsdc2016 / @blakenewman
// getUsers es6 module
export default async function getUsers(){
const { json } = await fetch('api/v1/users');
return json();
};
// ----
// Home controller
import getUsers from './getUsers';
homeController.$inject = ['$scope'];
async function homeController($scope) {
$scope.users = await getUsers();
}
app.controller('home', homeController);
JSDC Taiwan 2016 / #jsdc2016 / @blakenewman
Development Experience
JSDC Taiwan 2016 / #jsdc2016 / @blakenewman
The best code that you can write now, probably won't be in a few years
JSDC Taiwan 2016 / #jsdc2016 / @blakenewman
The best way to cope with change is to increase productivity
JSDC Taiwan 2016 / #jsdc2016 / @blakenewman
Hot module replacement in action

JSDC Taiwan 2016 / #jsdc2016 / @blakenewman
HMR (Hot Module Replacement)
Patch part of dependency tree with the new code
Changes appear live without page reloading
Compiler
HMR Server
Bundle Server
Compile
Change
code
HMR runtime
bundle
JSDC Taiwan 2016 / #jsdc2016 / @blakenewman
Exploring Vue.js

JSDC Taiwan 2016 / #jsdc2016 / @blakenewman
What does Vue deliver?
Library for the View layer
Components with reactivity
Extendable via plugins
Lightweight
Simplicity
Testable
JSDC Taiwan 2016 / #jsdc2016 / @blakenewman
How does Vue.js work?
Reactive data-binding system for a Data-driven view
DOM in sync with data
Object.defineProperty

JSDC Taiwan 2016 / #jsdc2016 / @blakenewman
How does Vue.js work?
Component System helps with small abstraction layer
Component loosely modeled after the Web Components spec
Implements the Slot API and the special attribute is

JSDC Taiwan 2016 / #jsdc2016 / @blakenewman
What is Reactivity?

JSDC Taiwan 2016 / #jsdc2016 / @blakenewman
Vue.js & Vuex == Sacrificial Architecture!
A
B
Mutation A
State B
State A
Actions
JSDC Taiwan 2016 / #jsdc2016 / @blakenewman
C
Vue.js Components
JSDC Taiwan 2016 / #jsdc2016 / @blakenewman
Vue.component('DemoComponent', {
template: '<div> {{ msg }} </div>',
// Data initialization
data() {
return {
msg: 'Vue is cool'
};
},
computed: { },
methods: { },
mounted() {
// Execute logic on mounted hook
},
});
// -------
<DemoComponent />

JSDC Taiwan 2016 / #jsdc2016 / @blakenewman

Vue.js 2.0
JSDC Taiwan 2016 / #jsdc2016 / @blakenewman
const vnode = {
tag: 'div',
children: [
{ tag: 'my-component' }
]
};
<div>
<my-component></my-component>
</div>
Virtual DOM
JSDC Taiwan 2016 / #jsdc2016 / @blakenewman
Thanks
Chat to me on twitter: @blakenewman
OR
Chat to me in the real world :)
JSDC Taiwan 2016 / #jsdc2016 / @blakenewman
Adapting to change #jsdc2016
By Blake Newman
Adapting to change #jsdc2016
Best practises to ensure you can adapt to change. Sacrificial Architecture can ensure you can constantly adapt your code and tooling. We will take a look at Vue.js, to see what it is trying to solve. Seeing how it can help you now and in the future.
- 2,331