Toy Problems
& Handling Stress at MakerSquare
-
Toy problems are mental coding exercises to teach you how to think about code.
-
These are also the same types of problems you will be expected to solve on a whiteboard when the pressure's on at an interview.
What is a Toy Problem?
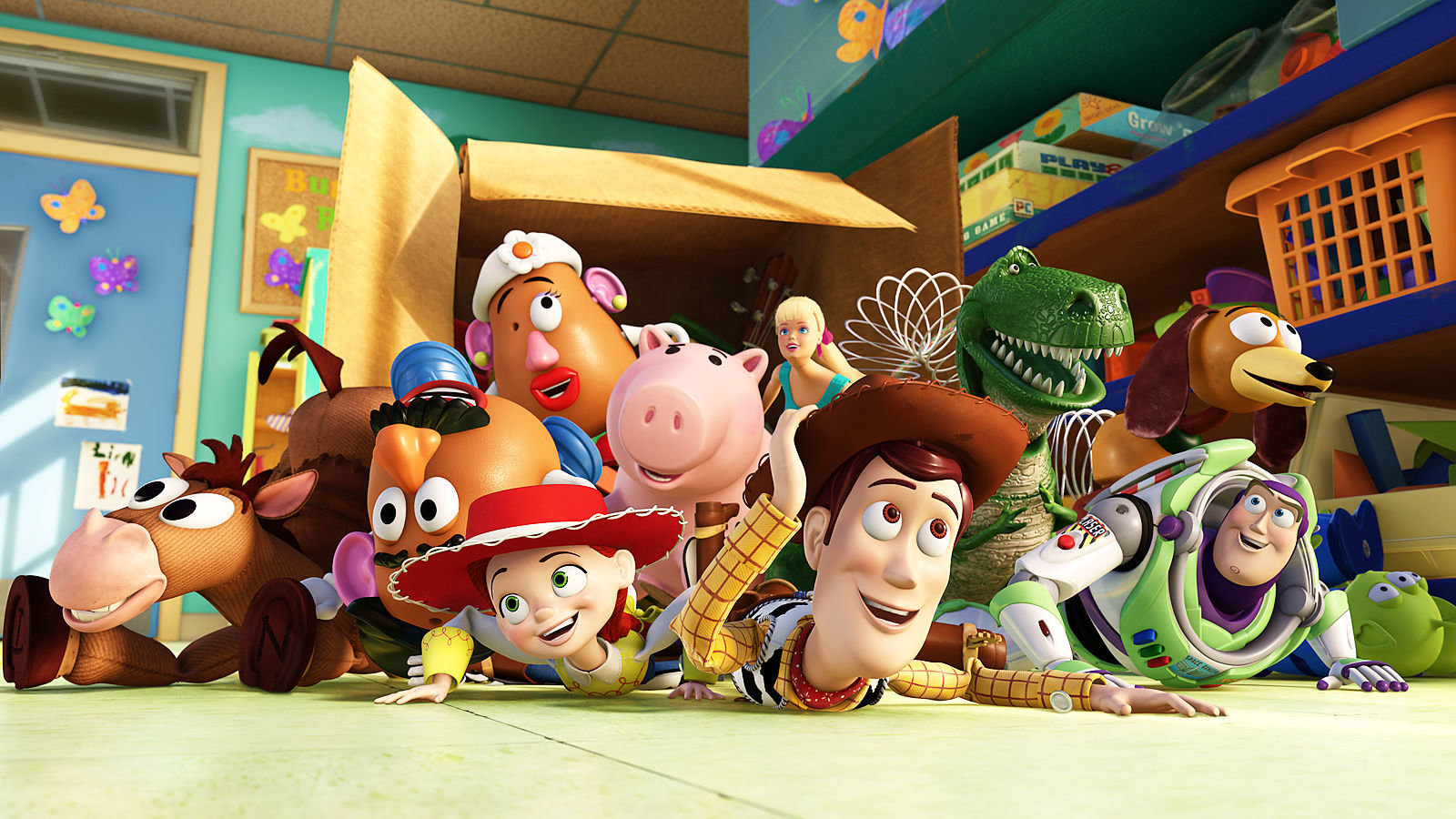
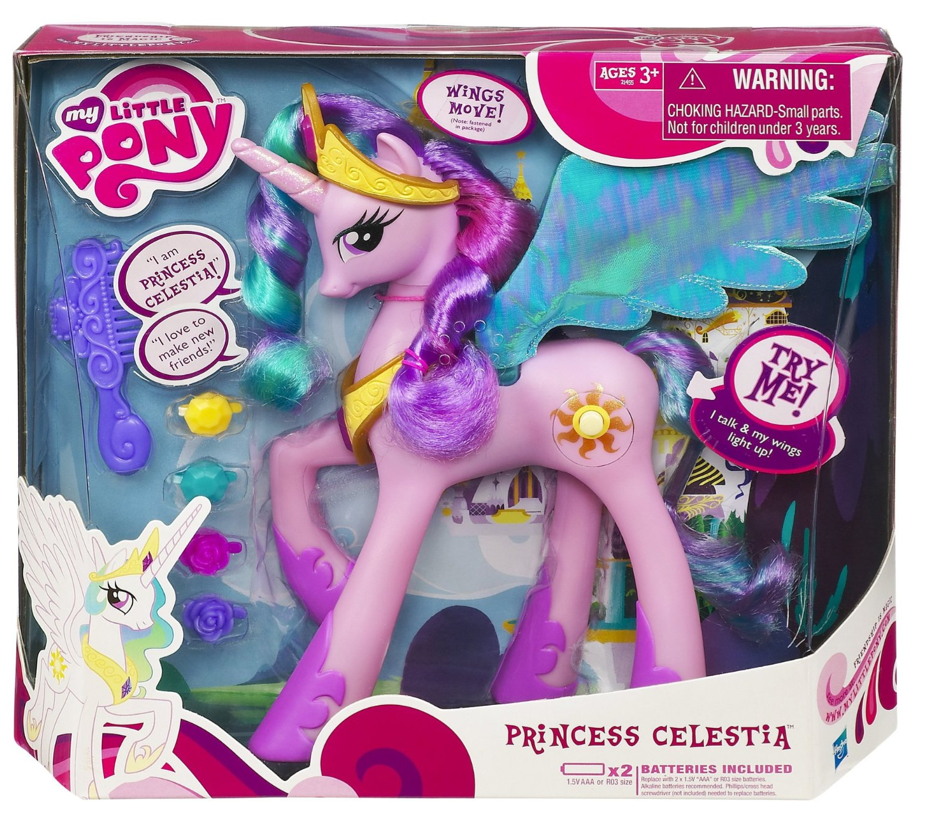
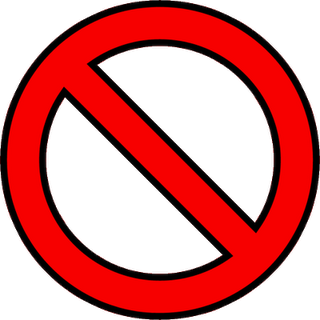
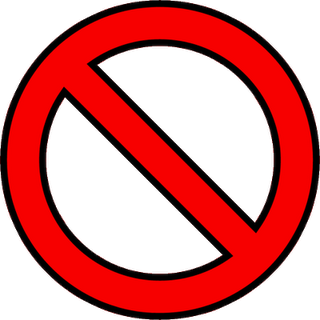
Toy Problem Examples
- Find all possible anagrams of a string
- Sort a string's characters in descending order by frequency, and ascending order alphabetically.
- Find the first item that occurs an even number of times in an array
- Find the longest palindrome in a string
- Check to see that a 9x9 string of digits is, or is not, a proper Sudoku solution.
Look and Say
Given a number n >= 1, write a function that returns the nth number in the "look-and-say" sequence as a string.
Examples
Input
1
2
3
4
5
6
Output
'1'
'11'
'21'
'1211'
'111221'
'312211'
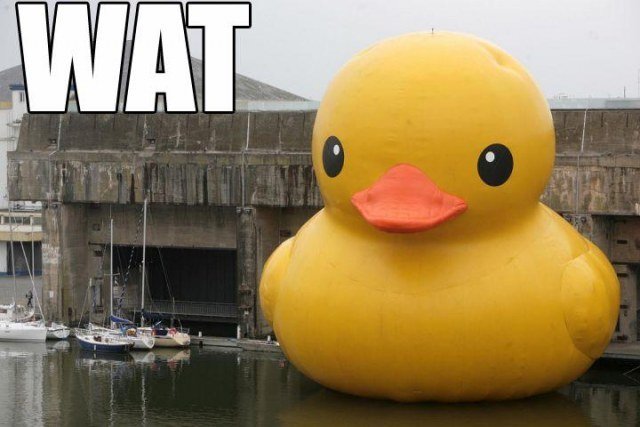
Given a number n >= 1, write a function that returns the nth number in the "look-and-say" sequence as a string.
Examples
Input
1
2
3
4
5
6
Output
'1'
'11'
'21'
'1211'
'111221'
'312211'
Steps to solve
- Identify what, exactly, the
problem is asking you to do.
- Come up with a plan how to
solve the problem.
(Whiteboarding helps.)
- Create pseudocode
Step-by-step instructions, in
English, to solve the problem.
- Code. And don't panic.
Handling Stress
Damned if I know...
We joined a course that will
have us working 10 hours a day
for over three months...
In the words of Bill Murray...
Accepting Your Inevitable Mental Breakdown
By week 5, I was on the verge of insanity and it was manifesting in my code. For example...
1. Feel free to get up and step away from the computer when you get frustrated.
2. If you need to take a walk, do it.
3. Excercise - even the minimum of walking 20 minutes a day, helps.
My Week 5 Look-And-Say Solution
function lookAndSay(n) {
// would you like to build a function?
// a function that returns a striiiing.
var lookSay = function(strNum) {
var sayArray = [];
var repeats = 1; // we check to see how many nums
var digit = 0; // and say the num
for (var i = 0; i < strNum.length; i++) {
if (!!strNum.charAt(i + 1) && strNum.charAt(i) === strNum.charAt(i +
1)) { // and if they are the saaaaaame?
repeats++; // if they are we take this, and increment
} else {
digit = strNum.charAt(i); // else digit is the char at IIIIII!
sayArray.push(repeats);
sayArray.push(digit);
repeats = 1;
}
}
return sayArray.join(""); // would you like to build a function.
// a for-loop non-recursive function...
};
// okay, bye.
var output = ''; // Would you like to have an output!
// have an output as a striiiing.
for (var i = 1; i <= n; i++) { // and now we're going to iterate
// say, ain't it great, we can count up to the thiiiing!
if (i === 1) {
output = '1'; // here we have our edge case.
// when i is 1
// and build from there to the sky!!!!
} else {
output = lookSay(output); // then we iterate the output...
}
}
// a stringy super-awesome output!
return output;
}
Thank you
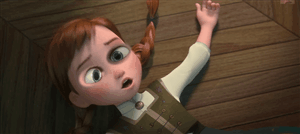
deck
By brianboyko
deck
- 972