ReactiveX RxSwift
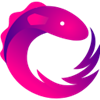
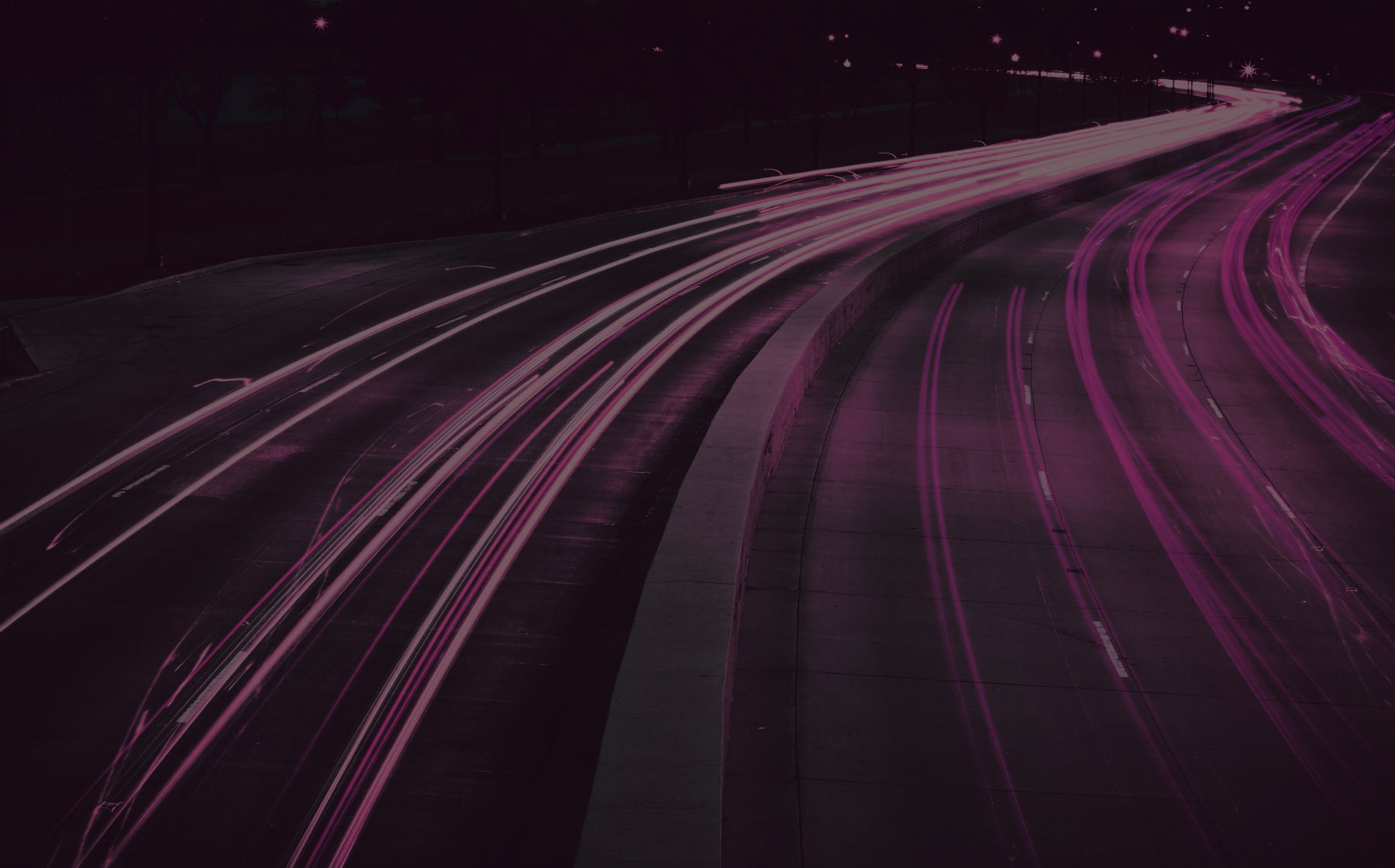
Calvin Huang
iOS 攻城蝨 at pipimy inc.
- Ruby
- JS ......(一拖拉庫的新東西是要學到哪時候,聽說昨天FB又出了新工具,是有什麼毛病XD) https://code.facebook.com/posts/1840075619545360/yarn-a-new-package-manager-for-javascript/
- PHP
The Observer pattern done right
ReactiveX is a combination of the best ideas from
the Observer pattern, the Iterator pattern, and functional programming
Here are some toptip
Observerable pattern
Reactive programming
Functional programming
Functional programming
struct Student {
let name: String
let age: Int
init(name: String, age: Int) {
self.name = name
self.age = age
}
}
let students = [
Student(name: "Calvin", age: 25), Student(name: "Fenny", age: 23),
Student(name: "Bob", age: 32), Student(name: "Tian", age: 35)
]
Functional programming
var ageBelow30 = [Student]()
for student in students {
if student.age < 30 {
ageBelow30.append(student)
}
}
var names = [String]()
for student in students {
names.append(student.name)
}
var ageBelow30Names = [String]()
for student in students {
ageBelow30Names.append(student.name)
}
Functional programming
let ageBelow30 = students.filter { $0.age < 30 }
let names = students.map { $0.name }
let ageBelow30Names = students.filter { $0.age < 30 } .map { $0.name }
Functional programming
let ageBelow30Names = students.reduce([]) { (result, student) -> [String] in
if student.age < 30 {
var nextResult = result
nextResult.append(student.name)
return nextResult
}
return result
}
Functional programming
More!!
- 不可變的狀態, 更少的副作用 (Immutable State, Side Effect Free)
- Concurrency 更抽象簡化
- Declarative
- ...等等
Reactive programming

以 Data Flow 為中心,有事件發生就及時反應的編程思維
Observerable pattern
ReactiveX is a library for composing asynchronous and event-based programs by using observable sequences.

Observerable pattern
整個 RX 都是 Obserbable

ReactiveX
An async , event based framework
query.rx.text
.filter {string in
return string.characters.count > 3
}
.debounce(0.51, scheduler: MainScheduler.instance)
.map {string in
let apiURL = URL(string: "https://api.github.com/q?=" + string)!
return URLRequest(URL: apiURL)
}
.flatMapLatest { request in
return URLSession.shared.rx.data(request)
}
.map { data —> [AnyObject] in
let json = try JSONSerialization.JSONObject(with: data, options: [])
return json as! [AnyObject]
}
.map {object in
return Repo(object: object)
}
.bindTo(tableView.rx.items(cellIdentifier("Cell"))
ReactiveX
v.s
PromiseKit

其實不太一樣,待稍後提提 (有機會再說)
ReactiveX
Text

ReactiveX

ReactiveX

ReactiveX
- 用到多少元件就會有N個 (或N以上個) Extension,因為功能都在Extension。
- Shared State 讓狀態管理一團糟 (要是想要讓另一個元件共用需要大概只能存成 Instance Property,但這只會讓事情更糟)
- Extension 摘到別的檔案裡面,完了,找不到 Property 從哪來。
ReactiveX

ReactiveX

ReactiveX
- 動作都透過 Observer 傳遞,寫法強制單一化
- 事件從哪來就到哪去都
-
No More Delegate!!!!
ReactiveX
超短改寫 Present
Observable
In ReactiveX an observer subscribes to an Observable. Then that observer reacts to whatever item or sequence of items the Observable emits. This pattern facilitates concurrent operations because it does not need to block while waiting for the Observable to emit objects, but instead it creates a sentry in the form of an observer that stands ready to react appropriately at whatever future time the Observable does so.
This page explains what the reactive pattern is and what Observables and observers are (and how observers subscribe to Observables). Other pages show how you use the variety of Observable operators to link Observables together and change their behaviors.
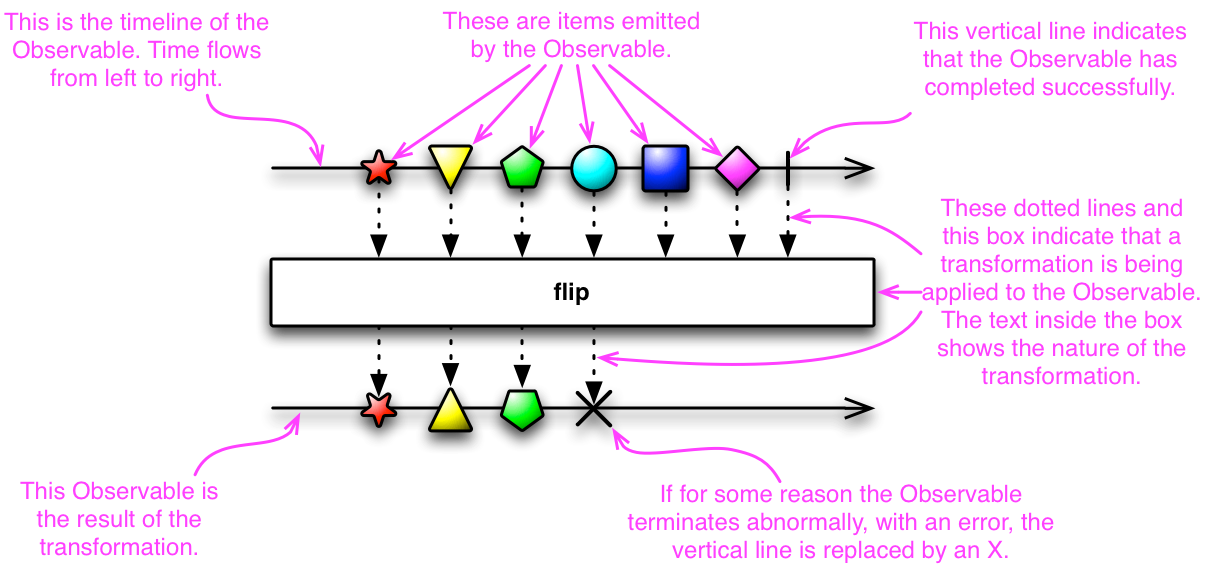


GitBookReader
死命趕也來不及在今天 Present 的 Project
iOS - RxSwift, Server - Express

其實後面有改 cocoaheads
IOS工程師(創新)
OMG歐買尬遊戲派對
茂為歐買尬數位科技股份有限公司


RactiveX short talk
By Calvin Huang