Introduction to React
More About React :D
@GoyeSays

Carlos Goyeneche
@GoyeSays
https://github.com/Goye

DOM Refs
In the typical React dataflow, props are the only way that parent components interact with their children. To modify a child, you re-render it with new props. However, there are a few cases where you need to imperatively modify a child outside of the typical dataflow. The child to be modified could be an instance of a React component, or it could be a DOM element. For both of these cases, React provides an escape hatch.
- Managing focus, text selection, or media playback.
- Triggering imperative animations.
- Integrating with third-party DOM libraries
When to Use Refs
DOM Refs
DOM Refs
class CustomTextInput extends React.Component {
constructor(props) {
super(props);
this.textInput = null;
}
focus = () => {
// Explicitly focus the text input using the raw DOM API
this.textInput.focus();
}
render() {
// Use the `ref` callback to store a reference to the text input DOM
// element in an instance field (for example, this.textInput).
return (
<div>
<input
type="text"
ref={(input) => { this.textInput = input; }} />
<input
type="button"
value="Focus the text input"
onClick={this.focus}
/>
</div>
);
}
}
You may not use the ref attribute on functional components because they don't have instances:
Refs and Functional Components
DOM Refs
function MyFunctionalComponent() {
return <input />;
}
class Parent extends React.Component {
render() {
// This will *not* work!
return (
<MyFunctionalComponent
ref={(input) => { this.textInput = input; }} />
);
}
}
React Lifecycle

Each component has several "lifecycle methods" that you can override to run code at particular times in the process. Methods prefixed with will are called right before something happens, and methods prefixed with did are called right after something happens.
React Lifecycle
These methods are called when an instance of a component is being created and inserted into the DOM:
- constructor()
- componentWillMount()
- render()
- componentDidMount()
React Lifecycle
Mounting
An update can be caused by changes to props or state. These methods are called when a component is being re-rendered:
- componentWillReceiveProps()
- shouldComponentUpdate()
- componentWillUpdate()
- render()
- componentDidUpdate()
React Lifecycle
Updating
This method is called when a component is being removed from the DOM:
- componentWillUnmount()
React Lifecycle
Unmounting
Other APIs
Each component also provides some other APIs:
- setState()
- forceUpdate()
React Lifecycle
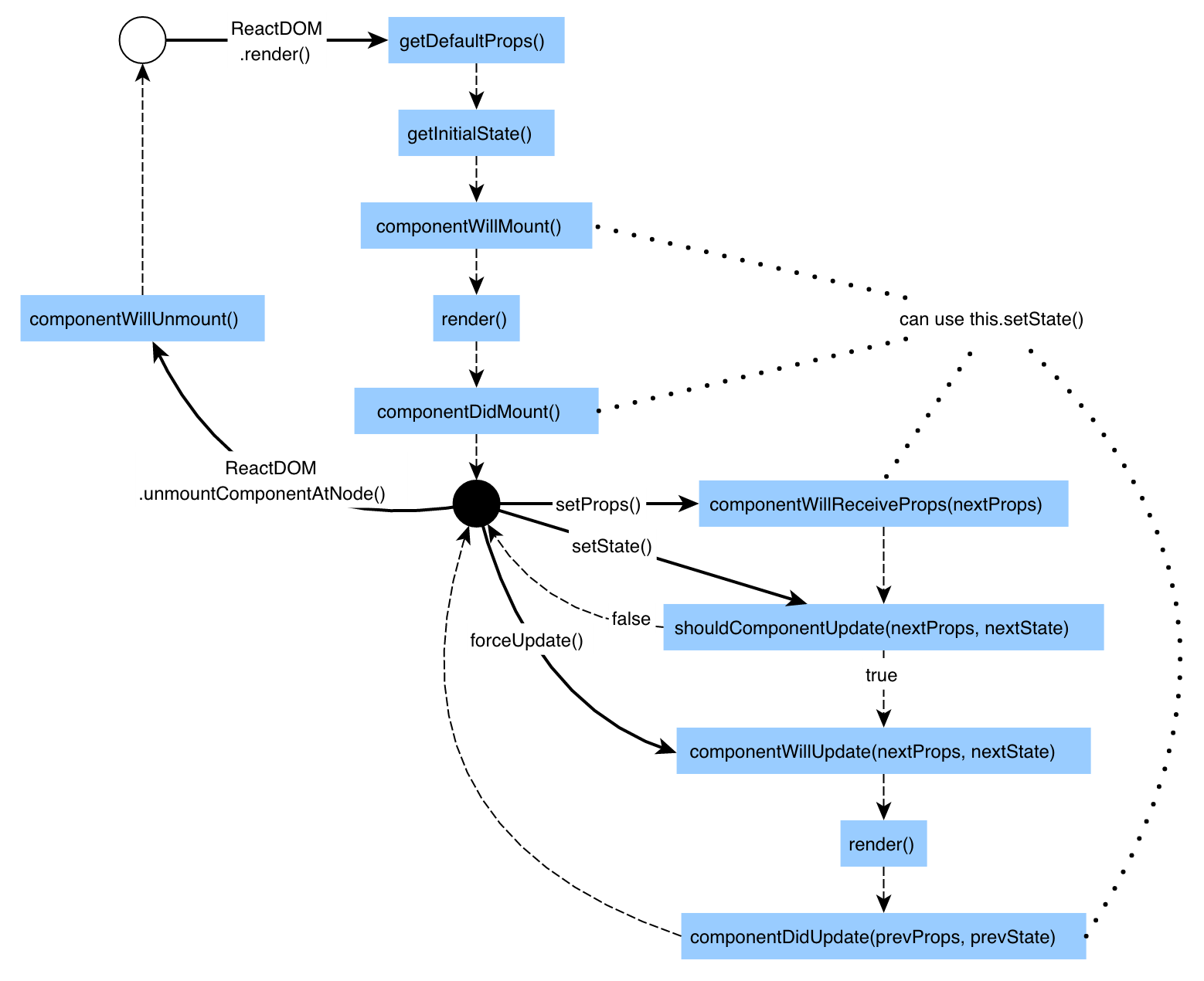
Fragments
A common pattern in React is for a component to return multiple elements. Fragments let you group a list of children without adding extra nodes to the DOM.
Quick example
Fragments
import React, { Fragment } from 'react';
const Header = (props) => (
<Fragment>
<Logo />
<Title />
</Fragment>
);
Problem
import React from 'react';
const Header = (props) => (
<div>
<Logo />
<Title />
</div>
);
SSR (Server Side Rendering)
Server-Side Rendering — SSR from here on — is the ability of a front-end framework to render markup while running on a back-end system.
Applications that have the ability to render both on the server and on the client are called universal apps.
SSR (Server Side Rendering)
Why bother?
This is tightly coupled with the rise of the Single Page Application — SPA from here on. SPAs offer great advantages in speed and UX over traditional server-rendered apps.
So the idea is to render your app on the server initially, then to leverage the capabilities of SPAs on the client.
SSR + SPA = Universal App
SSR (Server Side Rendering)
Cons of Rendering React on the Server
- SSR can improve performance if your application is small. But it can also degrade performance if it is heavy.
- It increases response time (and it can be worse if the server is busy).
- It increases response size, which means the page takes longer to load.
- It increases the complexity of the application.
SSR (Server Side Rendering)


Hydrate()
Same as render(), but is used to hydrate a container whose HTML contents were rendered by ReactDOMServer. React will attempt to attach event listeners to the existing markup.
React expects that the rendered content is identical between the server and the client. It can patch up differences in text content, but you should treat mismatches as bugs and fix them.
Hydrate()
Real example
import Article from './Article';
const appRoot = document.getElementById('app-root');
Loadable.preloadAll().then(() => {
const page = (
<AppContainer>
<Article
page={window.__INITIAL_STATE__.data}
/>
</AppContainer>
);
ReactDOM.hydrate(page, appRoot);
});
Portals
Portals provide a first-class way to render children into a DOM node that exists outside the DOM hierarchy of the parent component. (Added on React 16 version)
Portals
Example
const modalRoot = document.getElementById('modal-root');
class Modal extends React.Component {
constructor(props) {
super(props);
this.el = document.createElement('div');
}
componentDidMount() {
// The portal element is inserted in the DOM tree after
// the Modal's children are mounted
modalRoot.appendChild(this.el);
}
componentWillUnmount() {
modalRoot.removeChild(this.el);
}
render() {
return ReactDOM.createPortal(
this.props.children,
this.el,
);
}
}
Questions?
https://github.com/Goye

Thanks a lot!
https://github.com/Goye
ReactJS Season Four
By Carlos Goyeneche
ReactJS Season Four
ReactJS
- 278