Introduction to React
React Basics
@GoyeSays

Carlos Goyeneche
@GoyeSays
https://github.com/Goye

Did you remember the homework?
+
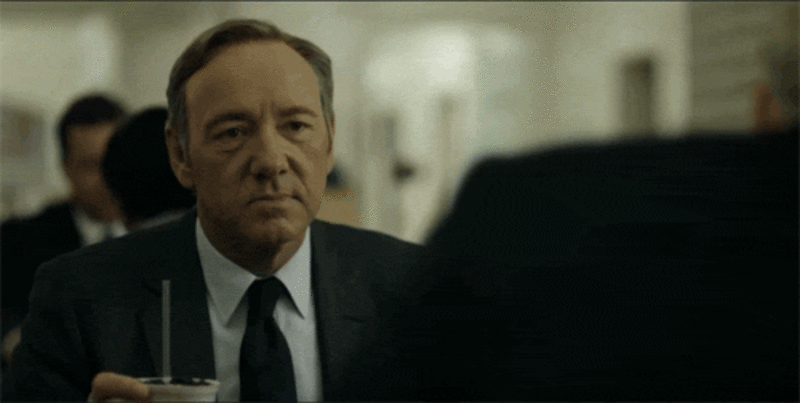
Function Composition in React
Inheritance
Inheritance is a concept in JavaScript which allows one class to inherit another class's properties to duplicate behavior and add more features
Is it javascript an inheritance language?
Function Composition in React

Function Composition in React
Inherited a Mess
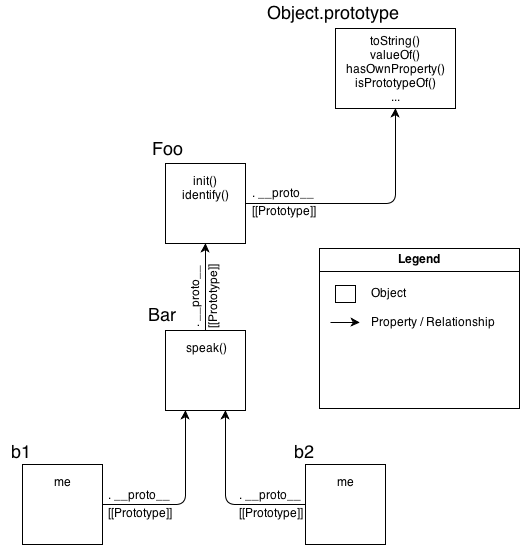
Function Composition in React
Behavior delegation" is a more accurate term to describe JavaScript's [[Prototype]]. This is not just a matter of word semantics, it's a fundamentally different type of functionality.
Inherited a Mess
Function Composition in React
Inheritance classic example
function Person(first, last, age) {
this.name = {
first,
last
};
this.age = age;
};
Person.prototype.greeting = function() {
alert('Hi! I\'m ' + this.name.first + '.');
};
function Teacher(first, last, age, subject) {
Person.call(this, first, last, age);
this.subject = subject;
}
Function Composition in React
Inheritance react example
class Label extends React.component {
constructor (props) {
super(props);
this.className = 'plain-label';
}
render () {
return (
<span className={this.className}>
{this.props.children}
</span>
);
}
}
class SuccessLabel extends Label {
constructor (props) {
super(props);
this.className = this.className + 'success-label';
}
}
class ErrorLabel extends Label {
constructor (props) {
super(props);
this.className = this.className + 'error-label';
}
}
class Main extends React.component {
render () {
return (
<div>
<Label> Plain label </Label>
<SuccessLabel> Success label </SuccessLabel>
<ErrorLabel> Error Label </ErrorLabel>
</div>
);
}
}
Function Composition in React
Composition
Composition is a technique which allows us to combine one or more components into a newer, enhanced component capable of greater behavior. Instead of extending another class or object, composition only aims at adding new behavior.
Function Composition in React
Composition react example
const Label = ({ children }) => {
return (
<span className={this.props.className + 'plain-label'}>
{children}
</span>
);
};
const SuccessLabel = ({ children }) => {
return (
<Label className='success-label'>
{children}
</Label>
);
};
const ErrorLabel = ({ children }) => {
return (
<Label className='error-label'>
{children}
</Label>
);
};
class Main extends React.component {
render () {
return (
<div>
<Label> Plain label </Label>
<SuccessLabel> Success label </SuccessLabel>
<ErrorLabel> Error Label </ErrorLabel>
</div>
);
}
}
Which one is better?
https://facebook.github.io/react/docs/composition-vs-inheritance.html

CreateClass vs ES6 Classes
Syntax difference
React.createClass
import React from 'react';
const Contacts = React.createClass({
render() {
return (
<div></div>
);
}
});
export default Contacts;
React.component
import React from 'react';
class Contacts extends React.Component {
constructor(props) {
super(props);
}
render() {
return (
<div></div>
);
}
}
export default Contacts;
CreateClass vs ES6 Classes
State differences
React.createClass
import React from 'react';
const Contacts = React.createClass({
getInitialState () {
return {
};
},
render() {
return (
<div></div>
);
}
});
export default Contacts;
React.component
import React from 'react';
class Contacts extends React.Component {
constructor(props) {
super(props);
this.state = {
};
}
render() {
return (
<div></div>
);
}
}
export default Contacts;
CreateClass vs ES6 Classes
"This" differences
React.createClass
import React from 'react';
const Contacts = React.createClass({
handleClick() {
console.log(this); // React Component instance
},
render() {
return (
<div onClick={this.handleClick}></div>
);
}
});
export default Contacts;
React.component
import React from 'react';
class Contacts extends React.Component {
constructor(props) {
super(props);
this.handleClick = this.handleClick.bind(this);
}
handleClick() {
console.log(this); // React Component instance
}
render() {
return (
<div onClick={this.handleClick}></div>
);
}
}
export default Contacts;
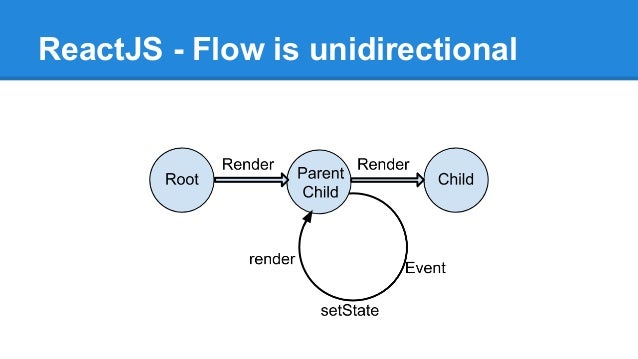
Unidirectional Dataflow
Unidirectional Dataflow
Example
class Label extends React.Component{
constructor(props) {
super(props);
this.state = {
message: 'everything works as expected (that's not truth)'
};
}
render(){
return <SuccessLabel
message={this.state.message}
/>
}
}
class SuccessLabel extends React.Component{
render(){
const { message } = this.props;
return <span className='success-label'>{message}</span>;
}
}
class Main extends React.Component{
render(){
<Label> Plain Label </Label>
<hr/>
}
}
JSX In Depth
Fundamentally, JSX just provides syntactic sugar for the React.createElement(component, props, ...children) function
<MyButton color="blue" shadowSize={2}>
Click Me
</MyButton>
React.createElement(
MyButton,
{color: 'blue', shadowSize: 2},
'Click Me'
)
JSX In Depth
User-Defined Components Must Be Capitalized
When an element type starts with a lowercase letter, it refers to a built-in component like <div> or <span> and results in a string 'div' or 'span' passed to React.createElement. Types that start with a capital letter like <Foo /> compile to React.createElement(Foo) and correspond to a component defined or imported in your JavaScript file.
JSX In Depth
User-Defined Components Must Be Capitalized
import React from 'react';
// Wrong! This is a component and should have been capitalized:
function hello(props) {
// Correct! This use of <div> is legitimate because div is a valid HTML tag:
return <div>Hello {props.toWhat}</div>;
}
function HelloWorld() {
// Wrong! React thinks <hello /> is an HTML tag because it's not capitalized:
return <hello toWhat="World" />;
}
JSX In Depth
Props in JSX
<MyComponent foo={1 + 2 + 3 + 4} />
JavaScript Expressions as Props
String Literals
<MyComponent message="hello world" />
<MyComponent message={'hello world'} />
Props default to "true"
<MyTextBox autocomplete />
<MyTextBox autocomplete={true} />
Spread attributes
function App2() {
const props = {firstName: 'Ben', lastName: 'Hector'};
return <Greeting {...props} />;
}
JSX In Depth
Props in JSX
<MyContainer>
<MyFirstComponent />
<MySecondComponent />
</MyContainer>
JSX Children
JavaScript Expressions as Children
<MyComponent>foo</MyComponent>
<MyComponent>{'foo'}</MyComponent>
Virtual DOM

Virtual DOM
How did you do that?
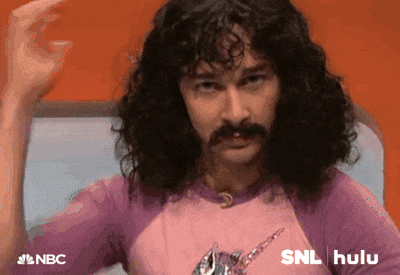
Virtual DOM
Reconciliation
https://reactjs.org/docs/reconciliation.html
The Diffing Algorithm
When diffing two trees, React first compares the two root elements. The behavior is different depending on the types of the root elements.
Virtual DOM
Examples
https://reactjs.org/docs/reconciliation.html
<div className="before" title="stuff" />
<div className="after" title="stuff" />
<div style={{color: 'red', fontWeight: 'bold'}} />
<div style={{color: 'green', fontWeight: 'bold'}} />
DOM Elements Of The Same Type
Questions?
https://github.com/Goye
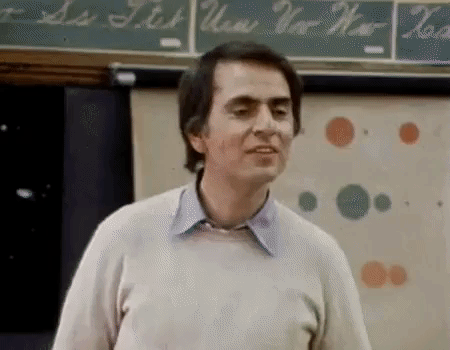
Thanks a lot!
https://github.com/Goye
ReactJS Season Two
By Carlos Goyeneche
ReactJS Season Two
ReactJS (Season Two)
- 331