ngular 2
An Introduction
Chad Jewsbury
@chadjewsbury
(but I don't tweet much)

OVERVIEW
Nuts & Bolts
Demo
Who am I?
- Front End Engineer @Groupon
- Online Booking Team
- Midwesterner
- New to Santa Cruz (July)
- New Father
www.chadjewsbury.com

My
Experience
- Internal Onboarding Tool
- New Project
- Started in June on RC1
- V1 released in December

OVErview
Angular.js (v1)
- 2010 - Original Release
- v1.6.1 - Current stable release
- 54.4k - Stars on Github
History
- Sep-2014 - Announced @ ng-Europe
- Apr-2015 - Developer Preview
- Dec-2015 - Beta
- May-2016 - First Release Candidate
Angular 2
September 14, 2016
Angular v2.0 Official Release
( ... only two years after originally announced ... )
- Improve Performance
- Mobile / Universal - (Bootstrap is platform specific)
- Simpler to reason about
Motivations & Goals
-
$scope, $scope.apply(), $scope.digest() -
Controllers - Zone.js
- RxJS
- @Decorators (ES2017)
- Component based design
- Typescript
What's new?
execution context that persists across async tasks
- Inspired by a feature in Dart
- Wrap functionality (sync or async) in a context
- Add hooks at start and end of execution
- Monkey-patch native async event handlers
Zone.js
function foo() {...}
function bar() {...}
function baz() {...}
foo();
bar();
baz();
Naive Example: Profiling
*Example adapted from Thougtram.io blog & Brian Ford's zone.js talk
/* start */
start = Date.now();
foo();
bar();
baz();
/* stop */
time = Date.now() - start;
/* log */
console.log(time);
Measure time elapsed
/* start */
start = Date.now();
foo();
setTimeout(bar, 2000);
/* ^^^ ??? */
baz();
/* stop */
time = Date.now() - start;
/* log */
console.log(time);
... But what about Async?
/* Execution order... */
start = Date.now();
foo();
setTimeout(bar, 2000);
/* ^ Added to event queue */
baz();
time = Date.now() - start;
console.log(time);
/* ... 2000 ms later */
bar();
function main() {
foo();
setTimeout(bar, 2000);
baz();
}
var myZoneSpec = {
beforeTask: function () {
console.log('Before task');
/* start timer */
},
afterTask: function () {
console.log('After task');
/* end timer */
}
};
var myZone = zone.fork(myZoneSpec);
myZone.run(main);
Hooray Zones!
Monkey-patched hooks
Zone.setInterval()
Zone.alert()
Zone.prompt()
Zone.requestAnimationFrame()
Zone.addEventListener()
Zone.removeEventListener()
NgZone
- Fork of Zone.js global zone
- Extended with Angular Behaviors
- Exposes RxJS Observables to trigger change detection / re-rendering
Reactive Extensions for JavaScript
- "Observable" sequences
- Asynchronous data streams
- Combine, filter, create multiple observables
RxJS





functions that modify JavaScript classes
- Attach metadata & functionality to classes
- Used everywhere in Angular...
@NgModule({ ... })
@Component({ ... })
@Pipe({ ... })
@Injectable()
@Decorators
function superhero(target) {
target.isSuperHero = true;
target.power = 'flight';
}
@superhero
class MySuperHero() {}
console.log(MySuperHero.isSuperHero);
// true
hierarchy of UI elements
- React-like structure
- Encapsulate templates, styles, functionality, and ui state in discrete components
- Compositional
Components

<subscribeComponent></subscribeComponent>
<!-- subscribeComponent -->
<div class="subscribe-container">
<h2>Subscribe</h2>
<myInputComponent [labelText]="Name">
</myInputComponent>
<myInputComponent [labelText]="Email" [inputType]="email">
</myInputComponent>
<myButton>Subscribe</myButton>
</div>
<!-- myInputComponent -->
<label>
{{labelText}}
<input type="{{inputType || 'text'}}"/>
</label>
Typed superset of Javascript
- Complies to plain javascript
- Adds static typing
Typescript
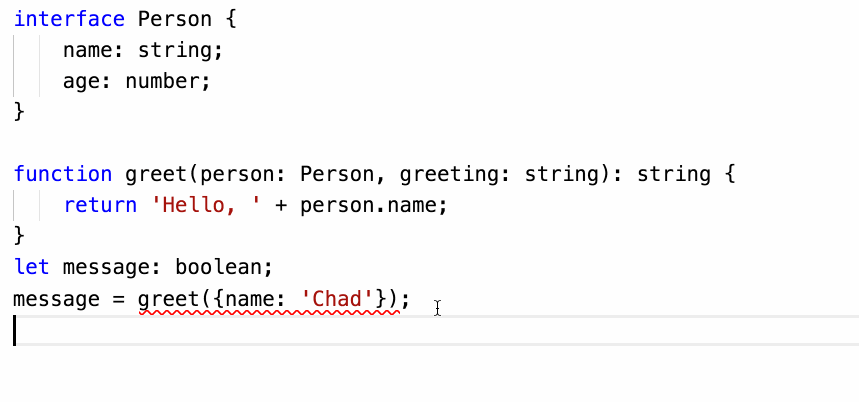
- Better tooling and feedback
- Great for large projects and/or teams
function Greeter(greeting) {
this.greeting = greeting;
}
Greeter.prototype.greet = function() {
return "Hello, " + this.greeting;
}
let greeter = new Greeter({message: "world"});
/*
No Errors! Totally Valid! :(
"Hello, [object Object]" instead of
"Hello, world"
*/
Classic Javascript
function Greeter(greeting: string) {
this.greeting = greeting;
}
Greeter.prototype.greet = function() {
return "Hello, " + this.greeting;
}
let greeter = new Greeter({message: "world"});
/*
Compilation Error!
Argument of type '{ message: string; }'
is not assignable to parameter of type 'string'.
Editor can highlight errors as you work!
*/
Typescript
class Student {
fullName: string;
constructor(public firstName,
public middleInitial,
public lastName) {
this.fullName = firstName + " "
+ middleInitial + " "
+ lastName;
}
}
interface Person {
firstName: string;
lastName: string;
}
function greeter(person : Person) {
return "Hello, "
+ person.firstName + " "
+ person.lastName;
}
var user = new Student("Jane", "M.", "User");
console.log(greeter(user)); // "Hello Jane User"
Nuts & Bolts

Demo
... but there's so much more
- Ahead-of-time compilation
- Animations
- Testing
- Angular Material
- Internationalization
- ngRouter
- ng-content (projection)
- ngForms
- Redux & Immutable.js
- NGRX or ng2-redux
Thanks!
http://slides.com/chadjewsbury/angular-2
https://github.com/jewsroch/angular-talk
Angular 2 An Introduction
By Chad Jewsbury
Angular 2 An Introduction
Angular 2 Talk at Santa Cruz JS Meetup. January 2017
- 595