Serverless Development
In Traditional



Application Server
Database Server
Have to:
Knowledge about Linux
Docker or Systemd for Daemon
Port Proxy on nginx (Virtual Host)
Issue on:
System-wide Security
Surplus System Performance
In the feture


Java / Golang / Node.js / Python / Ruby / C#
Golang / Node.js / Python
Cost
Server
Serverless
hourly-use on VM
bandwidth
storage
requests
In Practice
// In Basic Model
const functions = require('firebase-functions');
exports.sampleFunction = functions.https.onRequest((req, res) => {
const {
requestSize,
} = req.body;
res.json({
text: `Received Request: ${requestSize}`,
});
});
Data Model
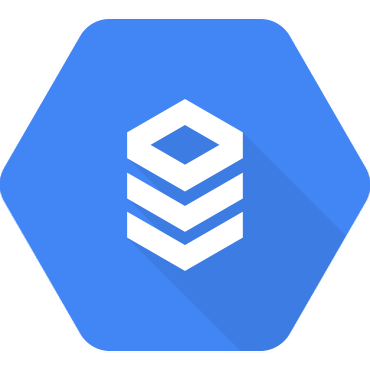

Cloud SQL
Traditional RDBMS
(MySQL / PostgresSQL...etc)
Cloud Firestore
No-SQL Database
Key-Value based scalable
In Practice
const functions = require('firebase-functions');
const admin = require('firebase-admin');
const moment = require('moment');
admin.initializeApp();
exports.listCaseCodes = functions.https.onRequest(async (req, res) => {
const snapshot = await admin
.firestore()
.collection('cases')
.where('numberCode', '>=', Number(`${moment().format('YYYYMM')}00001`))
.where('numberCode', '<', Number(`${moment().format('YYYYMM')}99999`))
.orderBy('numberCode', 'desc')
.get();
const responseList = [];
snapshot.docs.forEach(doc => {
const {
name,
numberCode,
creator,
} = doc.data();
responseList.push(`- *RC${numberCode}* ${name}${creator ? ` - ${creator}` : ''}`);
});
res.json(responseList);
});



Bot Webhook Handler
Issues
No shared memory to keep context
Establish connection to Firestore is very slow

https://www.serverless.com
One more thing
Flow Control
Talking about data
[map] N ⇢ N
[filter] N ⇢ N-M
[reduce] N ⇢ 1
Array
Promise
const asyncTask = new Promise((resolve, reject) => {
// ... working
});
asyncTask()
.then(() => {
// work after async task
})
.catch(() => {
// if error occured
})
.finally(() => {
// after task settled (no matter resolved or rejected)
});
In Practice
const payloadData = [1, 2, 3, 5, 8, 13];
// Parallels Request
Promise.all(payloadData.map(async (payload) => {
// do something async task
return payload * 2;
})).then((result) => {
// on all finish correctly;
console.log(result);
// [2, 4, 6, 10, 16, 26]
});
// Sequence Request
payloadData.map((payload) => async () => {
// do something async task
}).reduce((prev, next) => prev.then(next), Promise.resolve());
// Sequence Request with Response
payloadData.map((payload) => async (results) => {
// do something async task
return [
...results,
payload * 2,
];
}).reduce((prev, next) => prev.then(next), Promise.resolve([]))
.then((result) => {
// on all finish correctly
console.log(result);
// [2, 4, 6, 10, 16, 26]
});

https://caolan.github.io/async/v3/
Last thing 👻
CSS Variables

Scope w/ Components
import { css } from '@emotion/core';
const styles = {
title: css`
color: var(--title-color);
font-size: 20px;
`,
titleRed: css`
--title-color: red;
`,
titleBlue: css`
--title-color: blue;
`,
};
function Title({ children }) {
return (
<h3 css={styles.title}>
{children}
</h3>
);
}
function SampleComponent() {
return (
<div css={styles.wrapper}>
<div css={styles.titleRed}>
<Title>
Red Title
</Title>
</div>
<div css={styles.titleBlue}>
<Title>
Blue Title
</Title>
</div>
</div>
);
}
var with property
// @flow
import { css } from '@emotion/core';
import {
useRef,
useEffect,
} from 'react';
const styles = {
title: css`
color: var(--title-color);
font-size: 20px;
`,
titleRed: css`
--title-color: red;
`,
titleBlue: css`
--title-color: blue;
`,
titleDynamic: css`
--title-color: black;
`,
};
function Title({ children }) {
return (
<h3 css={styles.title}>
{children}
</h3>
);
}
function SampleComponent() {
const colorRef = useRef();
useEffect(() => {
funtcion onMove({ clientX }) {
const { current } = colorRef;
const width = document.body.clientWidth;
const percentage = clientX / width;
current?.style.setProperty('--title-color', `hsl(240, ${percentage * 100}%, 30%)`);
}
window.addEventListener('mousemove', onMove, false);
return () => window.removeEventListener('mousemove', onMove, false);
}, []);
return (
<div css={styles.wrapper}>
<div css={styles.titleRed}>
<Title>
Red Title
</Title>
</div>
<div css={styles.titleBlue}>
<Title>
Blue Title
</Title>
</div>
<div ref={colorRef} css={styles.titleDynamic}>
<Title>
Dynamic Color Title
</Title>
</div>
</div>
);
}
Au revoir
serverless-development
By Chia Yu Pai
serverless-development
- 351