intro to
functional programming
with php7
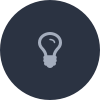

WHO am i?
Chris Langton
- Speaker for meetups
- Python: Django, Pandas, Hadoop
- PHP: Symfony ZF2 CI Doctrine
- Raspberry Pi: My fish can sms me!
- Blogger with publications in WDW, Pi Weekly, Openshift
- chrislangton84@gmail.com
- github.com/chrisdlangton
- www.nodewiz.biz
- @chrisdlangton
So what is Functional Programming?
Functional Programming allows for complexities to be trivial to follow
PHP however is Imperative fundamentally,
but it is also quite flexible
Don't be Shy
There are no dumb questions
only ones you don't ask
What Will Go Wrong?
Everything
If debugging is the practice of removing bugs from software... then programming must be the practice of adding them.
You will have to make mistakes to learn how to fix them
Key Concepts
Immutability and statelessness are core to functional programming and are important to understand, but don’t worry if they don’t quite make sense yet.
-
Statelessness is simple;
perform every task as if for the first time
-
Immutability often trips people up;
Functions should operate only on data passed in as arguments and will never rely on outside values to perform their calculations. Furthermore, any definition be it a variable or otherwise should be set once only and never change.
Continued..
Recursion and Data structures are equally important,
-
Recursion means no loops;
perform every loop instead as a recursive call
-
There are 4 types of structs to be aware of;
Dictionary, Set, Ordered Lists, and Unordered List. Any array in PHP is essentially one of these structs unfortunately, so we need to find our build blocks for this to work.
New Features in PHP7
Massive performance enhancements as well as;
-
Strict mode and more scalar types for param and return value hinting;
Particularly important for your pure functions to behave with known inputs and outputs. -
Constant arrays using define();
In terms of immutable data we will rely on define() more so this is a very welcome change. -
Closure::call;
The ability to bind a context to any closure can be beneficial but also harmful.
More new features
- Changes to list accepting ArrayAccess
- Generator delegation and Return Expressions
- Null coalescing operator
- Spaceship operator
- Anonymous classes
- Unicode codepoint escape syntax
- Exception Hierarchy
These are nice features, but not specifically needed to perform functional programming
Closures
A closure is a function which remembers it's environment in which it was created.
function closure() {
return function() {
echo "I'm a Closure!";
};
}
It has no access to anything in the scope of where it was called or even the function it is defined;
This pattern demonstrates Higher Order functions.
function closure($say) {
// can access $say
return function() {
echo "$say"; //can't access $say
};
}
Higher Order Functions
To take a function as a parameter, return a function, or both
In our last higher order function we lost access to data outside the closure scope.
Let's look at Early binding to address that constraint;
function closure($say) {
return function() use ($say) {
echo "$say"; //can access $say
};
}
Early binding is used for importing
$say
variable into the created closure returned by the higher order function.
For true closures with late binding one should use a reference when importing.
Immutability
An immutable object cannot be modified by accident.
Because all objects are safe from modification by default, and all changes are explicit, a significant amount of cognitive load can be reduced by not having to follow complex method chains to see where changes occur.
Together these constraints actually give us greater freedom to do complex operations while worrying less about object state.
Structs
These implement Iterable and Countable
Also Immutable once their value is set
Dictionary
- An associative array that consists of a key and a value.
OrderedList
- A list that contains any number of values in a defined order.
- a list that contains any number of values in an undefined order.
Set
- A set of values that contains no duplicates.
Data Types
PHP is fairly unique among popular programming languages in that the Array type can be used in so many ways
$dictionary = [
'rabbit' => 'fun pet',
'szechuan' => 'spicy deliciousness',
'php7' => 'not php as you know it'
];
$orderedList = ['amazing', 'functional', 'functional', 'programming'];
$unorderedList = [0, 8, 2, 32, 4];
$set = ['total war', 'sins of a solar empire', '0 AD'];
Flexible!
However, by using more strict data structures, we can avoid cognitive problems and have more testable code
However, by using more strict data structures, we can avoid cognitive problems and have more testable code
What next
There are tools you must first provide PHP with before you can get started employing Functional Programming.
I've put the entire project on GitHub for you;

Intro to Functional Programming with PHP7
By Chris Langton
Intro to Functional Programming with PHP7
- 1,108