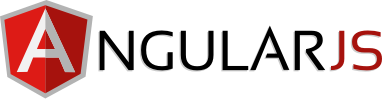
Part II: Electric Webaloo
Overview
- Me
-
Why NodeJS + Express
- Why AngularJS
-
Node + Express example
- Angular + Node + Express
- More advanced examples
-
NextStep.io use case
- Questions/Discussion
- List of resources
My Background
- BS in Computer Engineering, University of Illinois
- (Embedded) Software Engineer, Rockwell Collins
- Co-Founded RecBob, Jan 2012
- Co-Founder of NextStep.io
- Email: chris@nextstep.io || cquartier@gmail.com
where (not) to find me
@cquartier
http://cquartier.com
Why NodeJS?
-
Javascript all the things!
- asynchronicity
-
multi-platform capable (in my experience)
-
modularization from the start
- familiar debugging that actually works!
- Express simplifies Node API
- AWS OpsWorks supports Node servers
- personal reasons
Why use AngularJS?
- rapid prototyping (thanks Sam)
- decent for mobile (Phonegap/Trigger.io)
- greatly simplifies web app construction
- 2-way data binding is magical
-
its new, therefore buggy and less proven
-
changing RAPIDLY
- learning curve
NodeJS + Express Hello World
(requires node + npm)
> npm install -g express
...output...
> mkdir hello-world && cd hello-world
> express --sessions --ejs
...output...
> npm install
...output...
> node app.js
doesn't get much easier than that
Adding AngularJS
-
Get Angular Libray (or use Google CDN)
- bootstrap Angular
- try a simple expression
<!DOCTYPE html>
<html ng-app="fooApp">
<head>
<title><%= title %></title>
<link rel="stylesheet" href="/stylesheets/style.css" />
<script src="/javascripts/lib/angular.min.js"></script>
</head>
<body>
<h1><%= title %></h1>
<p>Welcome to {{ "hello-world" + (1 + 1) }}</p>
</body>
</html>
Doing More
That last example was pretty simple.
I'm about to go from 0 to 60 pretty fast
hold on to your butts
Let's look at a more complex example
Repository here:
https://github.com/cquartier/angular-example
Knowledge is Power
If you want to use AngularJS in a full-fledged app, you will want to understand the following:
- $scope
- dependency injection
- routing / templating
- $http or $resource
$scope
Understanding $scope is essential to any angular app
- I could spend all my time on this alone
-
some directives create their own scope
- ng-repeat, ng-switch, ng-include, ng-view, ng-controller
- inherits parent scope prototypically
- 2-way data binding in these isolate scopes does not behave the way you might initially assume when using primitive types (number, string, bool). This is because of prototypical inheritance!
- please read Understanding Scopes, then fail, then read it again, then seriously read it this time if you ever want to build your own app
Dependency Injection
- How does Angular know what $scope is in the first example below?
- in normal JS functions, order and type of parameters matter
- Angular cares about the name of the parameter, i.e. $scope is always $scope
- also helps with code modularization.
Inferred: Angular automagically injects dependencies by scanning your functions
function FooCtrl($scope, barService, ...) { ... }
Problem: minifiers will break your app Solution: Declare dependencies inline
fooApp.controller('FooCtrl', ['$scope', ..., function($scope, ...) {
...
}]);
Routing & Templating
-
without any front end framework, routing will happen server side
- EJS/Jade/etc for templating
- IMHO, this leads to custom js per page, reusability concerns
Angular can take over both routing and templating
- fun animations when transitioning pages!
- server side concerns simplified
- DB connection
- serving JSON data to Angular
$http or $resource
- both are used for ajax to backend
- note: same domain restrictions
- $http for non-RESTful endpoints
- example: /login, /logout
- manually set request type
- manually process response
- $resource for RESTful endpoints
- baked-in CRUD via REST
- less work for you on front-end
- requires RESTful routes on backend
NextStep.io
- MEAN stack (Mongo/Express/Angular/Node)
- AWS OpsWorks
-
mongolab
-
API server (api.nextstep.io)
- Node + Restify
- Oauth2orize
- mongoose
- Application server (nextstep.io)
- Node + Express
- Angular + ui-router
- jQuery (ugh) for Flot charts
Questions / Discussions
Resources
-
angularjs.org
- API documentation "ok" (lacks good examples)
- Tutorial good for core concepts ( http://docs.angularjs.org/tutorial)
- yearofmoo.com
- very helpful for animations in Angular
- egghead.io
- in-depth videos on core and advanced Angular concepts
- todomvc.com
- compare Angular to most other frontend frameworks
-
Plunker (http://plnkr.co/)
- use it for any and all prototyping
- good for collaboration
Angular.js Part II: Electric Webaloo
By cquartier
Angular.js Part II: Electric Webaloo
Angular.js is excellent for web prototyping: no server is needed to create an interactive web experience. But how do you begin to run Angular for a web app running on a full-blown server?
- 1,124