See Gamedev in JS
One...
Two...
Three words
about me.
That's me
Student of Taras Shevchenko National University of Kyiv
Faculty: cybernetics

#100DaysOfCode
About myself:
A Girl with great ambitions and low budget
Where you can find me
Let's imagine the situation...
Web developer
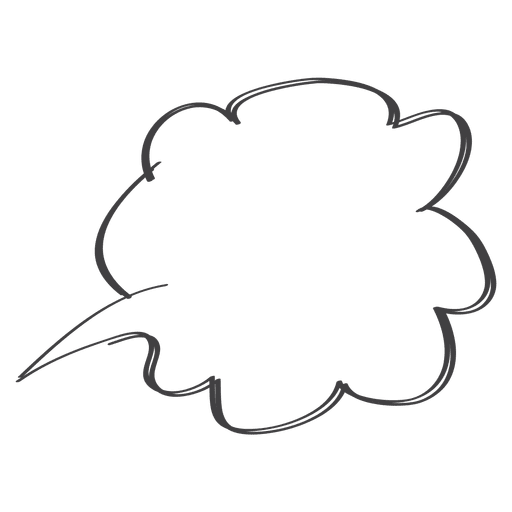
I don't want to be a web developer
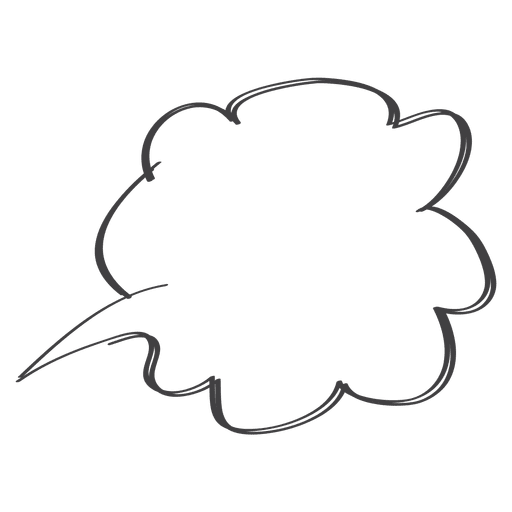
I want to be...
a game developer
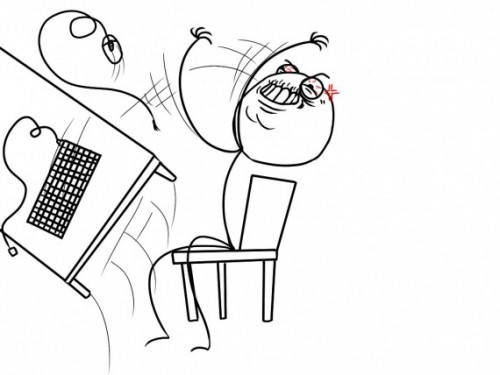
But before you start














Put your
devhats on
Let's the party begin

Chapter 1
Make a plan
Game





Speed
Physics
Sound
Animation
Platforms
Chapter 2
Let's choose language
Common choice
-
C++
-
Java
-
Swift
-
C#
-
HTML5
-
Unreal Engine
-
Unity
-
id Tech 4
-
Source
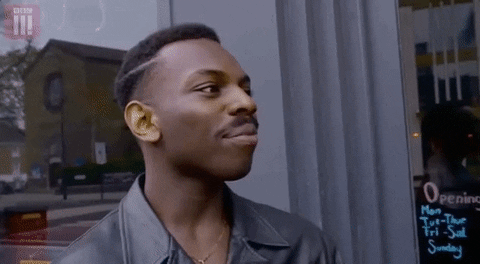
Why HTML5
Java Applets
Flash apps
Silverlight apps
Unity Web Player
<= require plugin
<= require plugin
<= require plugin
<= require plugin
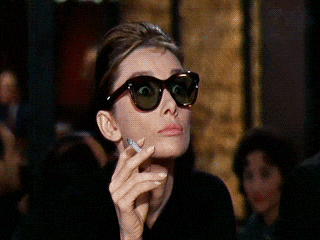
HTML5
<= no plugins, just browser!
Why to bother at all?
- build once and deploy everywhere
- no installs, no plugins, just instant gaming right in the browser
- millions of potential users/clients
- millions of potential developers
- huge database of ready-to-go solutions, libraries, engines and frameworks

So where are those games?
-
mobile games
-
games markets & promo sites
-
Steam
-
Playable ads
Duelyst


Heroes of Paragon
Kopanito All-Stars Soccer
Let's have a look
http://10.0.0.238:3000
To do list
-
Language -
Physic
-
Sound
-
Animation
-
Speed
Chapter 3
Let's create a game
HTML5
JS
CSS
DOM
var getRandomNumber = function (size) {
return Math.floor(Math.random() * size);
};
//calculate distance between click(event) to treasure (target)
var getDistance = function (event, target) {
var diffX = event.offsetX - target.x;
var diffY = event.offsetY - target.y;
return Math.sqrt((diffX * diffX) + (diffY * diffY));
};

Canvas
function startGame() {
myGamePiece = new component(30, 30, "red", 10, 120);
myGamePiece.gravity = 0.05;
myScore = new component("30px", "Consolas", "black", 280, 40, "text");
myGameArea.start();
}
var myGameArea = {
canvas : document.createElement("canvas"),
start : function() {
this.canvas.width = 480;
this.canvas.height = 270;
this.context = this.canvas.getContext("2d");
document.body.insertBefore(this.canvas, document.body.childNodes[0]);
this.frameNo = 0;
},
clear : function() {
this.context.clearRect(0, 0, this.canvas.width, this.canvas.height);
}
}

But...
It's rather boring...
We need some tools!!!
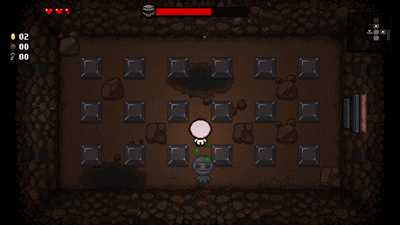
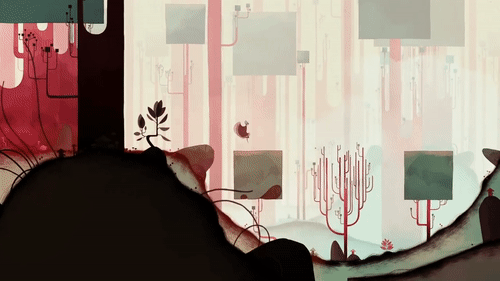
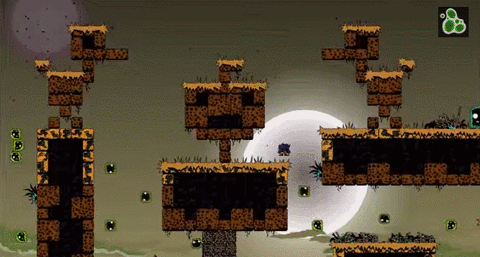
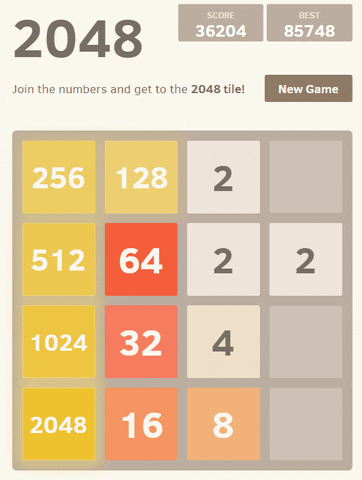
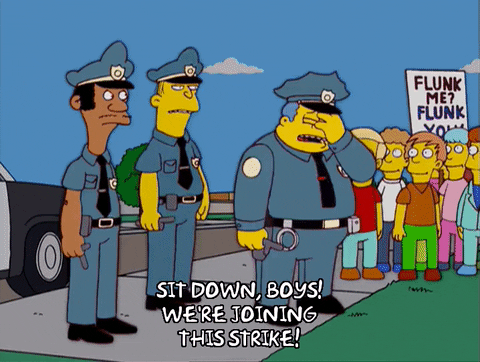















Wow...Slow down
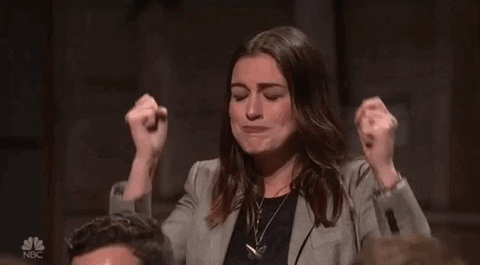
Chapter 4
Frameworks
Physic
Goal:
-
Simple collision detection
-
Simple manifold generation
-
Impulse resolution
- etc.
How to reach success?
Solution
-
Write your own code with the help of formulas
-
Use frameworks
-
Use libraries
Frameworks

-
Arcade Physics
-
P2
-
Ninja Physics

Example:

is a 2D physics engine for the web

A lightweight and simple 3D physics engine for the web.

Look an example
Load the physics shapes and sprites
function preload() {
// Load sprite sheet generated with TexturePacker
this.load.atlas('sheet', 'assets/fruit-sprites.png', 'assets/fruit-sprites.json');
// Load body shapes from JSON file
//generated using PhysicsEditor
this.load.json('shapes', 'assets/fruit-shapes.json');
}
Load magic line for physic
this.matter.world.setBounds(0, 0,
game.config.width, game.config.height);
// add some objects
this.matter.add.sprite(200, 50, 'sheet', 'crate',
{shape: shapes.crate});
this.matter.add.sprite(250, 250, 'sheet', 'banana',
{shape: shapes.banana});
this.matter.add.sprite(360, 50, 'sheet', 'orange',
{shape: shapes.orange});
this.matter.add.sprite(400, 250, 'sheet', 'cherries',
{shape: shapes.cherries});
Now add some more objects in the scene:
By click add more bananas
this.input.on('pointerdown', function (pointer) {
this.matter.add.sprite(pointer.x, pointer.y,
'sheet', 'banana', {shape: shapes.banana});
}, this);
}
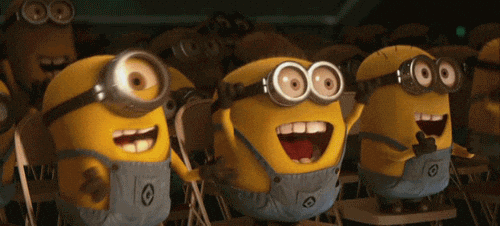
-
Language -
Physic -
Sound
-
Animation
-
Speed
To do List
Chapter 5
Frameworks
Sounds
Difficulties
-
Autoplay
-
Volume
-
Concurrent audio playback
-
Testing and support
Ways to work around the issues
-
Audio sprites
-
HTMLMediaElement
-
XMLHttpRequest
-
WebAudio
-
Libraries
Library

- Zero Dependencies
- Audio Sprites
- Works Everywhere
Most basic
var sound = new Howl({
src: ['sound.mp3']
});
sound.play();
Define and play a sound sprite:
var sound = new Howl({
src: ['sounds.webm', 'sounds.mp3'],
sprite: {
blast: [0, 3000],
laser: [4000, 1000],
winner: [6000, 5000]
}
});
// Shoot the laser!
sound.play('laser');
-
Language -
Physic -
Sound -
Animation
-
Speed
To do List
Chapter 6
Frameworks
Animation
Considerations
-
CSS transform
-
requestAnimationFrame
-
A take-away
var startTime = -1;
var animationLength = 2000; // Animation length in milliseconds
function doAnimation(timestamp) {
// Calculate animation progress
var progress = 0;
if (startTime < 0) {
startTime = timestamp;
} else {
progress = timestamp - startTime;
}
// Do animation ...
if (progress < animationLength) {
requestAnimationFrame(doAnimation);
}
}
// Start animation
requestAnimationFrame(doAnimation);
Libraries





Chapter 7
Brief look
PixiJS
Create great interactive graphics for Games and Web

What isn't PiXi
A game engine
No built-in :
- keyboard input
- collision detection
- sound engine
- animation engine Particles

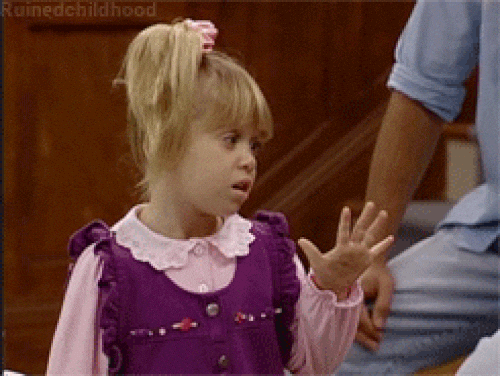
Then...What is Pixi?
- Wrapper for WebGL
- Multimedia engine
- Interactive

What PiXi gives us
- Sprites
- Easy loading
- Flexible data structure
- Cross-device compatibility
- JavaScript!

Chapter 8
Usage of PiXiJS
Setting up
$> npm install pixi.js
/** CDN Install (via cdnjs) **/
<script src="https://cdnjs.cloudflare.com/ajax/libs/pixi.js/4.5.1/pixi.min.js"></script>

Create background
var PIXI = require('pixi.js');
var app = new PIXI.Application(800, 600, {backgroundColor : 0x6889ff});
document.body.appendChild(app.view);

Result

Create Sprite Animation
let roleImages = ["images/mario1.png","images/mario2.png","images/mario3.png","images/mario4.png"];
let textureArray = [];
roleImages.forEach((role) => {
let texture = PIXI.Texture.fromImage(role);
textureArray.push(texture);
});
let mario = new PIXI.extras.AnimatedSprite(textureArray);
mario.animationSpeed = 0.1;
mario.play();
mario.interactive = true;
mario.position = new PIXI.Point(0, 538);
app.stage.addChild(mario);




Result

Layer
let tilingSprite =
new PIXI.extras.TilingSprite.fromImage(
'images/ground.png',
app.screen.width,
app.screen.height
);
tilingSprite.y = app.screen.height - 32;
tilingSprite.scale = new PIXI.Point(2, 2);
app.stage.addChild(tilingSprite);

Result

-
Language -
Physic -
Sound -
Animation -
Speed
To do List
Chapter 9
Future of HTML5
Games need performance!
JS is sloooow !
How about some speedup?
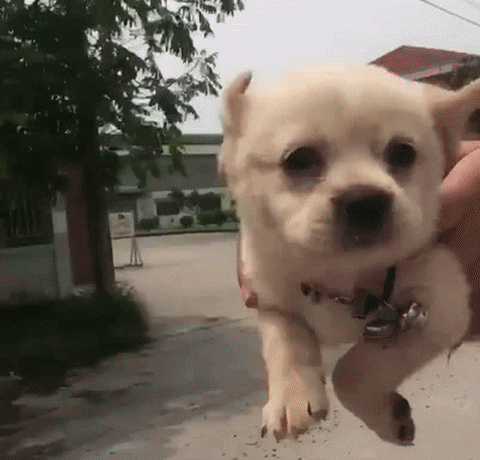
asm.js
- speed race since V8 by Google
- JS: dynamic, untyped, and interpreted
JIT - Just In Time Compiler
- compilation at execution
- adaptive optimization
- asm.js - strict subset of JS, generated by
source to source compilers
Result?
Up to 66% of native speed
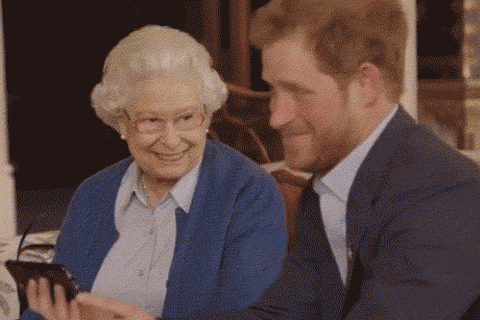
On a browser!!!
Who uses it?
- as a proof of
concept Mozilla portedsimple
Unreal Engine game (asm .js+WebGL interface)
- some heavy-performance JS libs use it
- game engines like Unity, Godot or Unreal allow
to export game to HTML5
Is that all?
Nope
Web Assembly is on the way
= native browser sandbox to run bytecode
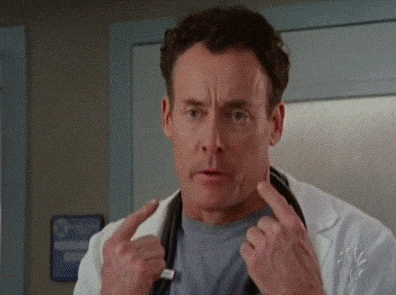











-
Parsing — the time it takes to process the source code into something that the interpreter can run.
-
WebAssembly doesn’t need to go through this transformation because it is already a bytecode.
-
Compiling + optimizing — the time that is spent in the baseline compiler and optimizing compiler. Some of the optimizing compiler’s work is not on the main thread, so it is not included here.
-
The compiler doesn’t have to spend time running the code to observe what types are being used before it starts compiling optimized code.
-
More optimizations have already been done ahead of time in LLVM
-
Re-optimizing — the time the JIT spends readjusting when its assumptions have failed, both re-optimizing code and bailing out of optimized code back to the baseline code.
-
In WebAssembly, things like types are explicit, so the JIT doesn’t need to make assumptions about types based on data it gathers during runtime.
-
Execution — the time it takes to run the code.
-
Many of the optimizations that JITs make to JavaScript just aren’t necessary with WebAssembly.
-
Garbage collection — the time spent cleaning up memory.
-
For now, WebAssembly does not support garbage collection at all. Memory is managed manually
Chapter 10
Final
Creating games is not so hard as it seems
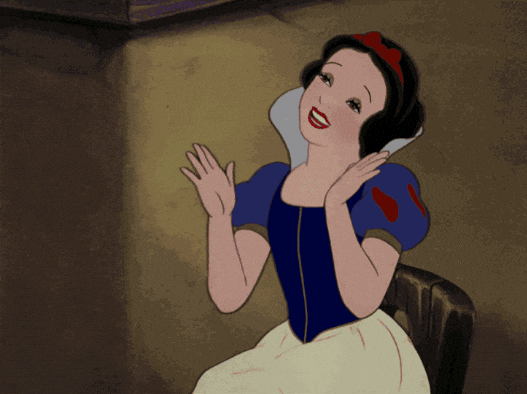
Results??!
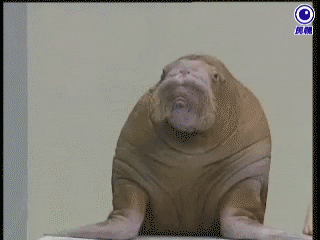
Give some little magic
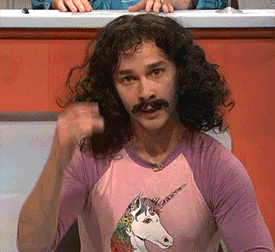
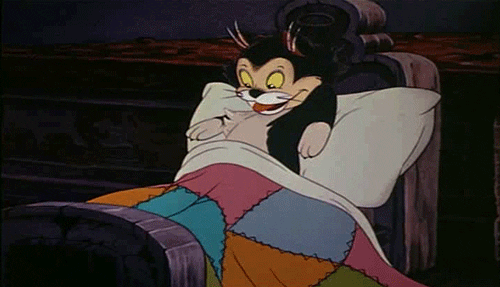
Thank you for attention
Gamedev in JS
By Khrystyna Landvytovych
Gamedev in JS
- 891