Nice to meet you,
React World
Who am I?
My concruption
- Developed by Facebook
- A view layer library
-
Not a framework like Backbone, Angular
etc . - You can't use React to build a fully-functional web app
Why was I developed?
- The complexity of two-way data binding
- Bad UX from using "cascading updates" of DOM tree
- A lot of data on a page changing over time
- The complexity of Facebook's UI architecture
- A shift from MVC mentality
Who uses me?
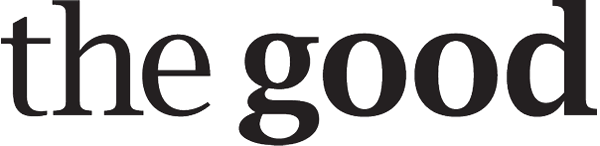
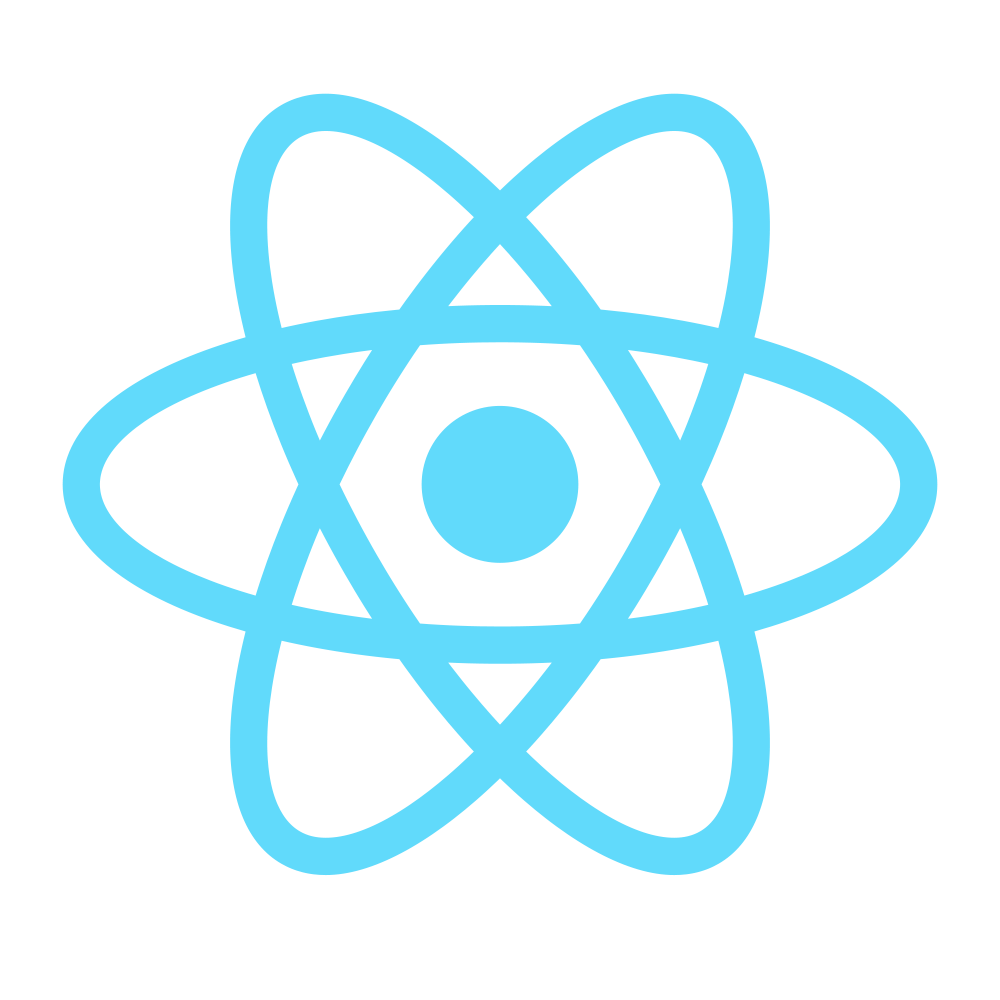
Easy to understand what a component will render
-
Declarative code → predictable code
React is fast!
- Real DOM is slow
- JavaScript is fast
- Using virtual DOM objects enables fast batch updates to real DOM, with great productivity gains over frequent cascading updates of DOM tree
No complex two-way data flow
- Uses simple one-way reactive data flow
- Easier to understand than two-way binding
- Uses less code
React dev tools
- React Chrome extension makes debugging so much easier
It's okay to see errors in React like this
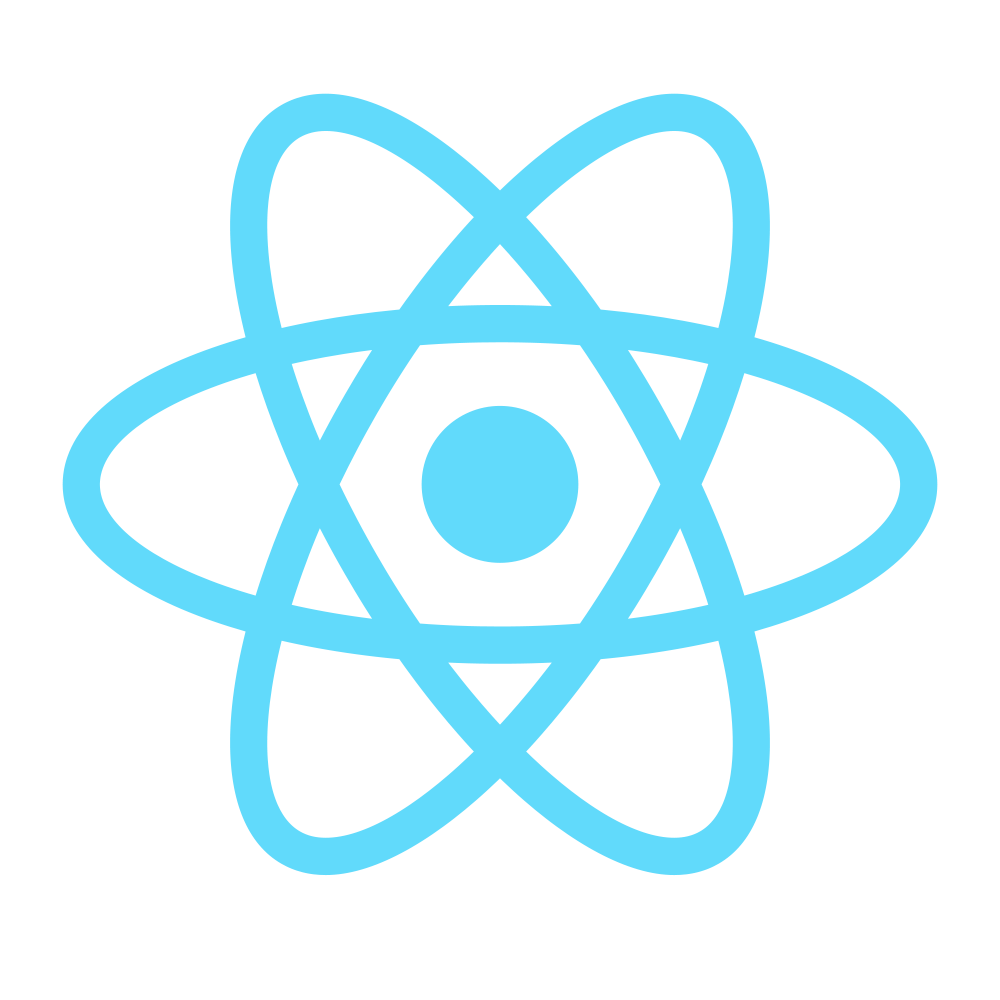
the Bad
React is nothing but the view
- No events
- No XHR
- No data / models
- No promises / deferreds
- No idea how to add all of the above
No support for older browsers
- React won't work with IE8
- There some polyfills / shims that help
Why should I use?
- Easy to read and understand views
- Concept of components is the future of web development
- If your page uses a lot of fast updating data or real time data - React is the way to go
- Once you and your team is over the React's learning curve, developing your app will become a lot faster
Fundamentals
Most important terms in React
abstract away all the basic React.js needs of configuration
“create-react-app”
Why do we need Babel?
Answer is easy —for latest ECMAScript syntax support (like ES5, ES6).
Why do we need Webpack?
Webpack is most widely used and an accepted module bundler and task runner through out React.js community.
All the API are available on the React.*.
-
Component
-
PureComponent
-
createElement(type, [props], [...children])
-
cloneElement(element, [props], [...children])
-
createFactory(type)
-
isValidElement(object)
-
Children // map, forEach, count, only, toArray
-
Fragment
React Top-Level API
React DOM
JSX
Is Syntax extension for JavaScript code which looks lot like HTML, it has full power of JavaScript. See the below snippet.
render(){
return(
<div>Hello</div>// JSX
)
}
Component
Components are self-contained reusable building blocks of web application.
React components are basically just idempotent functions (same input produces same output).
They describe your UI at any point in time, just like a server-rendered app.
import React, { Component } from 'react';
Class App extends Component {
Render(){
returns(
<div>Hello</div>
)
}
}
export default App
Components
Stateful vs Stateless
StateFul component — are written using ES6 classes instead of function
import React, { Component } from 'react';
Class App extends Component {
Render(){
returns(
<div>Hello</div>
)
}
}
export default App
Stateless or Functional Component — are written using arrow function.
import React, { Component } from 'react';
Const App = () => <div>Hello</div>
export default App
OR
import React, { Component } from 'react';
Const App = () =>
render() {
return (
<div>Hello</div>
)
}
export default App
Components
Dump vs Smart
-
Smart components are stateful. They may have a state and lifecycles. So, the component will re-render every time you set the new state or receiving new props.
- Dump components don’t have a state or lifecycles at all and will re-render every time then prop changes.
Props
- Passed down to component from parent component and represents data for the component
-
accessed via this
.props
...
var News = React.createClass({
render: function() {
var data = this.props.data;
var newsTemplate = data.map(function(item, index) {
return (
<div key={index}>
<p className="news__author">{item.author}:</p>
<p className="news__text">{item.text}</p>
</div>
)
})
console.log(newsTemplate);
return (
<div className="news">
{newsTemplate}
</div>
);
}
});
...
props.children
this.props.children is a special prop, typically defined by the child tags in the JSX expression rather than in the tag itself.
State
State is the place where the data comes from. We should always try to make our state as simple as possible and minimize the number of stateful components.
import React from 'react';
class App extends React.Component {
constructor(props) {
super(props);
this.state = {
header: "Header from state...",
content: "Content from state..."
}
}
render() {
return (
<div>
<h1>{this.state.header}</h1>
<h2>{this.state.content}</h2>
</div>
);
}
}
export default App;
Props vs State
Lifecycle
If you create a react class component, you will be able to use component’s lifecycle methods.
What is "key" in React?
Keys help React identify which items have changed (added/removed/re-ordered). To give a unique identity to every element inside the array, a key is required.
function ListComponent(props) {
const listItems = myList.map((item) =>
<li key={item.id}>
{item.value}
</li>
);
return (
<ul>{listItems}</ul>
);
}
const myList = [{id: 'a', value: 'apple'},
{id: 'b', value: 'orange'},
{id: 'c', value: 'strawberry'},
{id: 'd', value: 'blueberry'},
{id: 'e', value: 'avocado'}];
ReactDOM.render(
<ListComponent myList={myList} />,
document.getElementById('root')
);
Tips of advice
Pulling in API Data
fetch and axious
Axious
is promise-based and thus we can take advantage of async and await for more readable asynchronous code.
import React from 'react';
import axios from 'axios';
export default class PersonList extends React.Component {
state = {
persons: []
}
componentDidMount() {
axios.get(`https://jsonplaceholder.typicode.com/users`)
.then(res => {
const persons = res.data;
this.setState({ persons });
})
}
render() {
return (
<ul>
{ this.state.persons.map(person => <li>{person.name}</li>)}
</ul>
)
}
}
Fetch is a new-ish, promise-based API that lets us do Ajax requests without all the fuss associated with XMLHttpRequest.
const myPost = {
title: 'A post about true facts',
body: '42',
userId: 2
}
const options = {
method: 'POST',
body: JSON.stringify(myPost),
headers: {
'Content-Type': 'application/json'
}
};
fetch('https://jsonplaceholder.typicode.com/posts', options)
.then(res => res.json())
.then(res => console.log(res));
Practical part
Let's build an
YouTube Searcher
(using API)
Conception
Our Result
Create a project in Google API Dashboard
Look for the service YouTube -> YouTube Data API v3:
Create API
Nice to meet you,
By Khrystyna Landvytovych
Nice to meet you,
- 263