Full-stack web application and RESTful web services
Daniel Popescu | Computer Scientist | Adobe Systems
"The secret to building large apps is never build large apps. Break your applications into small pieces. Then, assemble those testable, bite-sized pieces into your big application"
`Justin Meyer`
A little bit of history
Moving from this:
document.write('Hello World!');
to this:
var Book = function(price, author){
var _price = price;
var _author = author;
this.getAuthor = function(){
return _author;
}
};
var myBook = new Book(80, 'Douglas Crockford');
myBook.getAuthor(); // outputs 'Douglas Crockford'
Self-executing functions
(
function(name) {
alert('Hello ' + name);
}
)('John Doe')
Inner functions
function Person() {
function getName() {
return 'John Doe';
}
alert('Hello ' + getName());
}
alert('Hello ' + getName());
Self-rewriting functions
var sayName = function() {
alert('John Doe');
sayName = function() {
alert('Already did that');
}
}
Prototypes
var Person = function(firstName, lastName) {
var _firstName = firstName;
var _lastName = lastName;
this.getFirstName = function() {
return _firstName;
}
this.getLastName = function() {
return _lastName;
}
}
Person.prototype.getFullName = function() {
return this.getFirstName() + ' ' + this.getLastName();
}
Hoisting
Why U no FUN ?
var x = 12;
function getX() {
console.log(x);
var x = 3;
console.log(x);
}
getX();
// output:
// 'undefined'
// 3
Why?
Tools available today
RequireJS / CommonJS
define(function(require, exports)){
'use strict';
var Logger = require('util/Logger'),
PubSub = require('core/PubSub'),
MyModule = require('application/modules/MyModule');
var module = new MyModule();
module.start();
exports.getResult = module.processData();
}
Less / Sass
@bgColor: red;
@borderColor: black;
@alternateBgColor: green;
.decorator() {
background-color: @bgColor;
border: 1px solid @borderColor;
}
.myClass {
.decorator;
.myInnerClass {
background-color: @alternateBgColor;
}
}
Bower
A package manager for the web
Grunt
The JavaScript Task Runner

Jasmine / Mocha / Chai
describe("A suite", function() {
it("contains spec with an expectation", function() {
expect(true).toBe(true);
});
});
Object Oriented JS ?

JS - MVC - frameworks

<angular/>
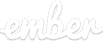
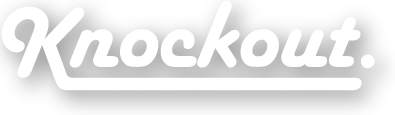
And "zillions" more...
Best Practices
Full-stack web application and RESTful web services
By Daniel Popescu
Full-stack web application and RESTful web services
Demonstrating best practices, design patterns and REST communication through a pilot web application
- 14