Whats New in Node 10
JaxNode November 2018
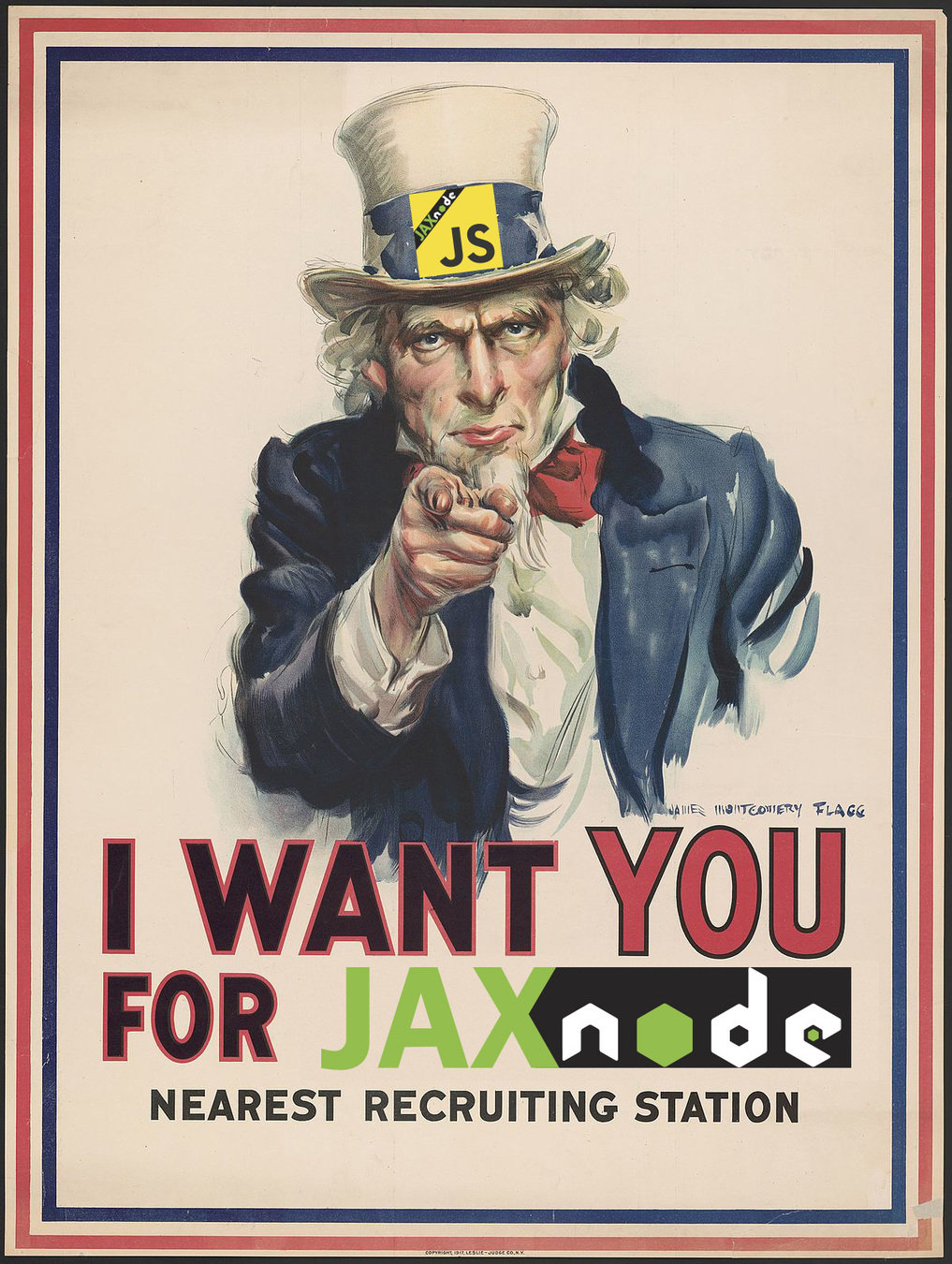
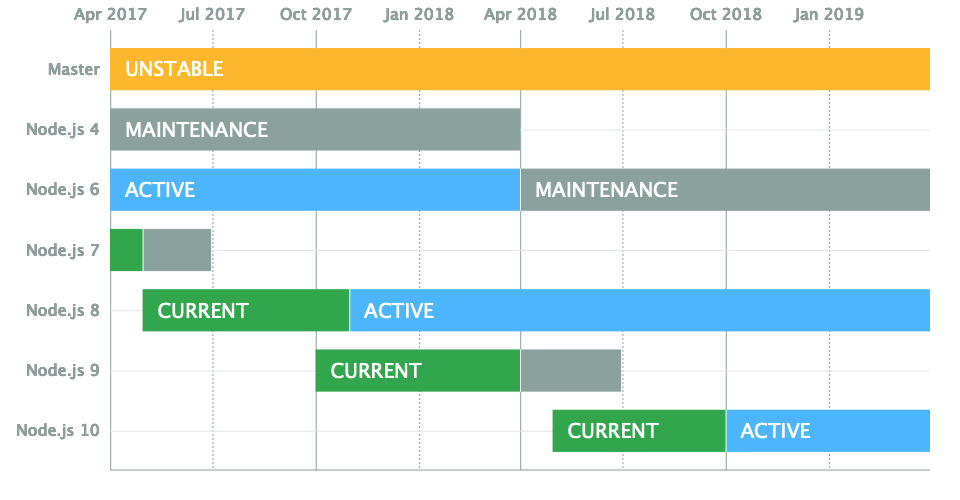
There is no Roadmap for Node!
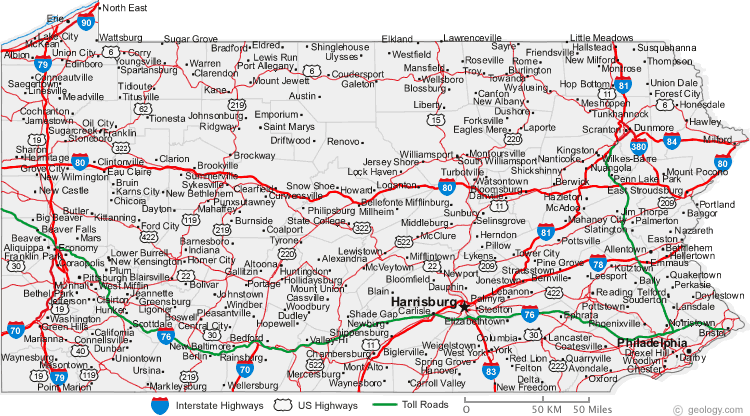
Node versions
- Node LTS version 10
- Node 11
- openssl 1.1.0i
- v8 v6.8.275.24
- Node 11 v8 now at v7.0
Engines
- V8 (Google)
- ChakraCore (Microsoft)
- JSCore (Apple)
New features
- Async Hooks
- Promise based modules
- N-API
- http/2
- Time Travel Debugging
- ESM (Ecmascript modules)
- Worker Threads
2 Versions of Node?
- 10 is the LTS version
- Long Term Support
- Ready for Production
- 11 is experimental release
Async Hooks
- Create callbacks that execute around system events
- Used to provide additional info around system and can execute other behavior
- Once created can be disabled or enabled
- Experimental
class MyAsyncCallbacks {
init(asyncId, type, triggerAsyncId, resource) { }
destroy(asyncId) {}
}
class MyAddedCallbacks extends MyAsyncCallbacks {
before(asyncId) { }
after(asyncId) { }
}
const asyncHook = async_hooks.createHook(new MyAddedCallbacks());
Promise based Modules
- Promise based versions of core Node APIs
- File System
- DNS
- Domains
-
const fsPromises = require('fs').promises;
const fsPromises = require('fs').promises;
async function openAndClose() {
let filehandle;
try {
filehandle = await fsPromises.open('thefile.txt', 'r');
} finally {
if (filehandle !== undefined)
await filehandle.close();
}
}
Addons N-API
- Native C/C++ api for using native code
- Abstraction layer
- Will work with different engines
- V8, ChakraCore, JSCore
- Most methods are experimental
http/2
- http v1.1 very verbose
- http v1.1 is request/response based
- http/2 allows multiplexing
- Can serve multiple files over same connection
- Stable
http/2 gocthas
- http/2 does not require TLS, but browsers do
- Very easy to add certificates to http/2 server
- Express requires plugin module to run http/2
- Hapi supports http/2
const http2 = require('http2');
const fs = require('fs');
const server = http2.createSecureServer({
key: fs.readFileSync('localhost-privkey.pem'),
cert: fs.readFileSync('localhost-cert.pem')
});
server.on('error', (err) => console.error(err));
server.on('stream', (stream, headers) => {
// stream is a Duplex
stream.respond({
'content-type': 'text/html',
':status': 200
});
stream.end('<h1>Hello World</h1>');
});
server.listen(8443);
// Generate certs for example
openssl req -x509 -newkey rsa:2048 -nodes -sha256 -subj '/CN=localhost' \
-keyout localhost-privkey.pem -out localhost-cert.pem
Time Travel Debugging
- Can go forwards and backwards with debugging
- Available in ChakraCore
- Used in conjunction with VSCode
ESM Modules
- Allows for use of `import` and `export` keywords
- Part of the ES6/2015 spec
- React has been using this for years
- ESM modules use *.mjs extension
- Node currently differentiates between CJS/MJS
- No one is happy with ESM modules
Demo
New Language Features
- ES2015/16/17
- Most features added in Node 4 and 6
- Async/Await came in Node v8
ES2015 Features in Node 4
Let and Const
Iterators and Generators
Arrow => functions
Reflection
New literals
Template Strings
Classes
Lexical Scoping
Maps, Set, WeakMap
ArrayBuffer
Promises
Octal support
Node 6 features
- ...Spread Operator
- New Buffer
- Default Parameters
- Destructuring
Resources
- https://medium.com/@atulanand94/beginners-guide-to-writing-nodejs-addons-using-c-and-n-api-node-addon-api-9b3b718a9a7f
- https://hackernoon.com/node-js-tc-39-and-modules-a1118aecf95e
- https://medium.com/webpack/the-state-of-javascript-modules-4636d1774358
Questions?
Whats New in Node 10
By David Fekke
Whats New in Node 10
There are a lot of changes that have come to Node over the last year. We will explore some of the new features released in Node 10.
- 1,112