Node for .NET Developers
August 2017
About Me
- David Fekke
- Chief Mobile Architect for Swyft Technology
- Co-organize JaxNode and JaxARCSIG
- Been in IT role since 1990
- Web, Database, Backend Services and
Mobile development - Commercial Pilot
JaxNode
- Node.js User Group
- Meet the third Thursday of each month
- jaxnode.com

A brief History

1995
Java
JavaScript



2009



Why learn Node.js
- Makes Concurrency easy
- Microsoft heavily invested in Node.js
- Broad cloud support from Amazon, Azure, IBM and Heroku
- Client side tools built with or use Node.js
- Solves the C10K problem
- Take advantage of vast Module ecosystem
- Single threaded and Asynchronous by default
You are already using Node
- Microsoft active contributor to Node.js
- Version of Node built on top of Chakra engine
- Visual Studio uses it for client side dependencies
- Gulp, Grunt and WebPack all use Node.js
- Many Azure services built with Node.js
- Mobile Central built with Node
- Run .NET code in Node applications
- Visual Studio Code built on top of Node.js
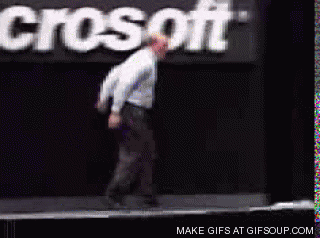
Misconceptions about JS
- JavaScript is browser only *
- JavaScript is slow *
- Node.js is interpreted *
- Not Object Oriented *
* None of these assumptions is true
The Truth
- Node.js runs on top of V8 and libuv
- Your code runs inside of a run loop
- Node.js is event driven
- Use clustering API to scale out your apps
- Each process only requires 20MB of RAM
Atwood's Law
Any application that can be written in JavaScript, will eventually be written in JavaScript

Node used for
- Web Frontend Development
- Mobile Development Cordova, React-Native
- Desktop Apps (Electron)
- Backend Services (AWS, Heroku, Azure, IBM)
- IoT Devices (Tessel 2, Galleo)
- Microsevices and APIs
Types of Apps
- Web Apps
- Service Apps REST/JSON
- MicroService Apps
- Socket Apps
- Command Line tools
- Message Queue and Bus Apps
- Desktop Apps with Electron
- Mobile Apps
Module System
- Units of functionality called Modules
- npmjs.org hosts over 500000 modules
- NPM command line tool to install
- Two types, native addon and pure JS
- Native modules extend V8s C++ framework
- Modules stored in 'node_modules' sub folder
- Import modules using require() function or import using ES module support

Package.json
- Used for configuration and meta information
- npm init will create package.json
- dependencies and devDependencies stored in this file
npm init
npm install
npm install module
npm install module --save
npm test
npm start
Useful NPM commands
walkthrough creating pkg.json
will grab your dependencies
installs individual module
installs includes in your dependencies
runs your unit tests
starts and runs your app
Package Clients
- NPM comes bundled with Node
- Yarn started last year as alternative, much faster
- Bower built for client-side
// Example from Nodejs.org/en/about
const http = require('http');
const hostname = '127.0.0.1';
const port = 3000;
const server = http.createServer((req, res) => {
res.statusCode = 200;
res.setHeader('Content-Type', 'text/plain');
res.end('Hello World\n');
}).listen(port, hostname, () => {
console.log(`CodeImpact Server running at http://${hostname}:${port}/`);
});
Node Gotchas
- Duct type language
- If Type system important TypeScript or Flow
- == not the same as ===
- Async, break work up in chunks
- Be careful with floating point math
- Avoid Callback hell
- Debugging has been a challenge, but much better
Functional JS
- JavaScript not a traditional OOP language
- Prototypical Inheritance
- Really more functional language like Lisp
- youtube.com/watch?v=zkFH1x-F_88
Avoiding Callback Hell
- Use named functions
- Use Promises
- Async/Await have been added to Node.js v8
ES6/ES2015/ES2016+
- JavaScript has many new language features
- LTS versions of Node have most of these features
- Babel and TypeScript transpilers allow almost all ES2016/17 features
Popular Frameworks
- Socket.IO
- Express
- Hapi.js
- Sails MVC
- Seneca MicroServices
Alternative Engines
- V8 default engine
- V8 version 6 introduced new Interpreter and compiler, Ignition and Turbofan
- Microsoft open sourced ChakraCore engine in Edge browser
- New Node bindings allow the JavaScript engine to be swapped out.
- Can use 'nvs' to switch between engines and versions of Node.
Demo
Resources
- nodejs.org
- Node Weekly
- jaxnode.com
- nodeschool.io
- github.com/jaxnode-UG/codeimpact2017
- github.com/jaxnode-UG/codeimpactchat
- github.com/Jaxnode-UG/node4es2015
- github.com/Jaxnode-UG/node6es2015
Questions?
Contact Me
- David Fekke at gmail dot com
- Skype: davidfekke
- Twitter: @davidfekke @jaxnode
- www.jaxnode.com
- www.meetup.com/Jax-Node-js-UG/
Node for .NET Developers
By David Fekke
Node for .NET Developers
These slides are for a presentation for CodeImpact 2017
- 1,563