Leveraging Azure Cloud Services with Unity3D and WebAssembly
JaxNode October 2017
John Lyons
David Fekke
Speakers
Hosting Node on Azure
Game server
Unity3D
WebAssembly Intro
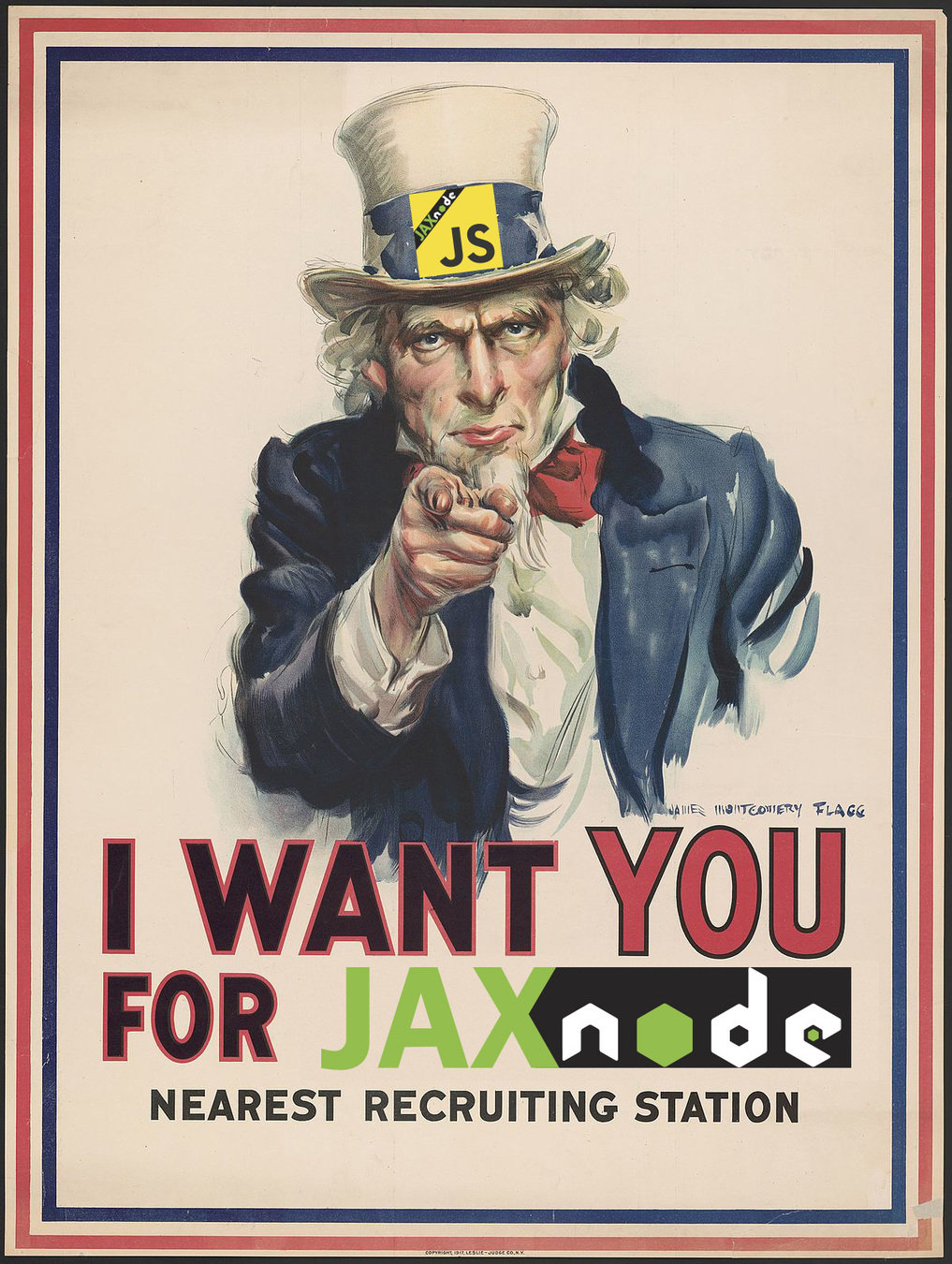
JAXNODE IS LOOKING
FOR SPEAKERS!!!
- Electron
- Go Lang
- Kubernetes, Swarm or Mesos
- Client Side frameworks
- NAPI
- Any other JavaScript topic
WebAssembly
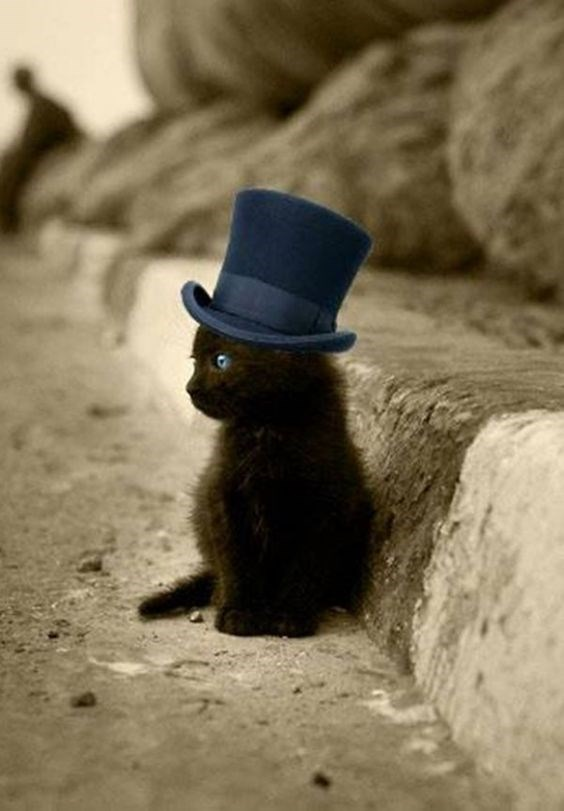
WebAssembly
- What the Heck is It?
- Direct Successor to ASM.js
- Compiler Target for the Web
- Runs on Web and Node.js
WebAssembly history
- ASM.js tool from Mozilla to compile their C/C++ code into a low-level JS format
- Effort began a couple of years ago to incorporate a binary executable format in all browsers
- Firefox added support in March 2017
- All major browser vendors now support it as of October 2017
WASM format
- Compiles to binary format
- Has an intermediate WAST format
int add(int a, int b) {
return a + b;
}
C code
WAST format
(module
(table 0 anyfunc)
(memory $0 1)
(export "memory" (memory $0))
(export "_Z3addii" (func $_Z3addii))
(func $_Z3addii (; 0 ;) (param $0 i32) (param $1 i32) (result i32)
(i32.add
(get_local $1)
(get_local $0)
)
)
)
Wasm Assembly
wasm-function[0]:
sub rsp, 8 ; 0x000000 48 83 ec 08
mov ecx, esi ; 0x000004 8b ce
mov eax, ecx ; 0x000006 8b c1
add eax, edi ; 0x000008 03 c7
nop ; 0x00000a 66 90
add rsp, 8 ; 0x00000c 48 83 c4 08
ret ; 0x000010 c3
DEMO
Web use cases
- Eliminate need for plugins
- Support custom codecs
- Games
- Image processing
- AI/Machine Learning
Node use cases
- Replace native modules with WASM
- No need to create separate executables for each chip architecture or OS
- Replace processor intensive pure JS modules
How can we use today
- Unity3D has web export
- Unreal engine can run in WASM
- emscripten to compile to WASM
What is missing in 1.0?
- No native Web API support
- Single Threaded
- No GC
- No SIMD support
Questions?
And Now,
John Lyons!
NodeJS, Websockets, Unity3d
Websockets
WebSockets, part of the HTML5 specification, enables two-way communication between web pages and a remote host. (https://tools.ietf.org/html/rfc6455)
- Reduce unnecessary network traffic and latency using full-duplex through a single connection (instead of two)
- Streaming through proxies and firewalls, supporting simultaneously upstream and downstream communication
- Backward compatible with the pre-WebSocket world by switching from an HTTP connection to WebSockets (degrades)
The good (read bad) old days of browser based games
Long Polling - server holds the request open until new data is available. Once available, the server responds and sends the new information.
Flash Socket uses a flash object to establish a connection to the WebSocket server and communicates using the WebSocket protocol. This means an additional Flash object (SWF files) will need to be leveraged.
Short Polling - an XHR request is made from the browser it is answered immediately and closes immediately
WebSocket Upgrade Path
- http(s) handshake
- Upgrade to Websocket connection
- Full duplex (bidirectional comms) over TCP
Websocket vs UDP/TCP
-
The overhead induced by TCP/IP and TLS in particular can dwarf the overhead of WebSocket itself.
-
Applications can use application-level message batching to effectively reduce the overhead that can be induced by TCP/IP and TLS.
-
WSS gives you easy encryption
-
HMAC-SHA256 gives you payload signing
-
Websockets make streaming easy.
-
Cell phone IP's aren't static there proxied
-
Sends strings, buffered arrays, and blobs
-
No need for port mapping , UPNP, etc.
Benchmarks Here:
https://crossbario.com/blog/Dissecting-Websocket-Overhead/
Another Great write up:
https://stackoverflow.com/questions/13040752/websockets-udp-and-benchmarks
Common Websocket implementations
- socket.io
- pomelo
- ws
A word on Azure App Services
References
- http://www.websocket.org/aboutwebsocket.html
- http://www.websocket.org/quantum.html
- http://buildnewgames.com/websockets/
- http://ithare.com/tcp-and-websockets-for-games/
- https://crossbario.com/blog/Dissecting-Websocket-Overhead/
- https://github.com/NetEase/pomelo
- https://github.com/websockets/ws
- https://tools.ietf.org/html/rfc6455
- https://developer.valvesoftware.com/wiki/Latency_Compensating_Methods_in_Client/Server_In-game_Protocol_Design_and_Optimization
I'm BORED , let's look at some code.
Unity3D and WebAssembly
By David Fekke
Unity3D and WebAssembly
- 2,496