Let's learn to code
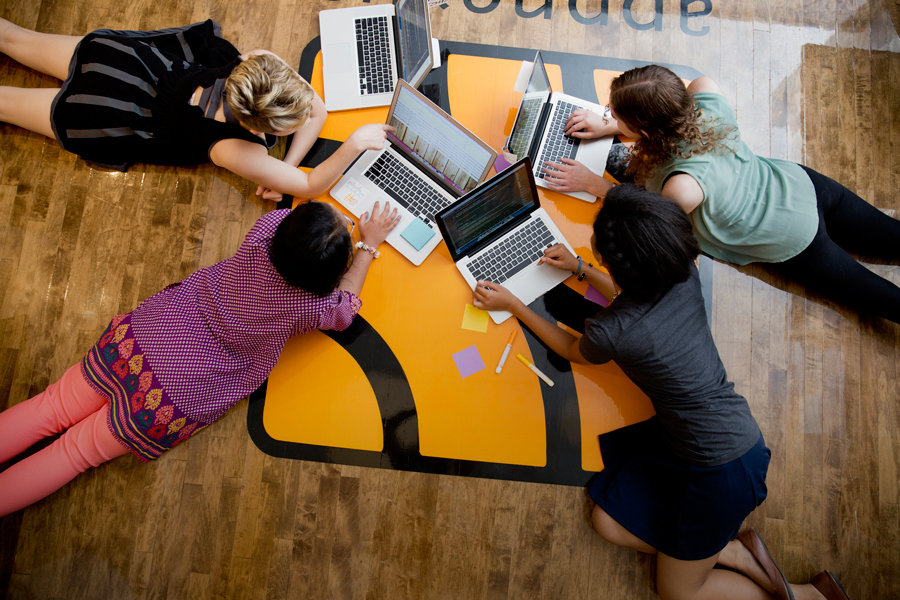
Apps are code
And fun.
So, let's build an app.
Starting with...
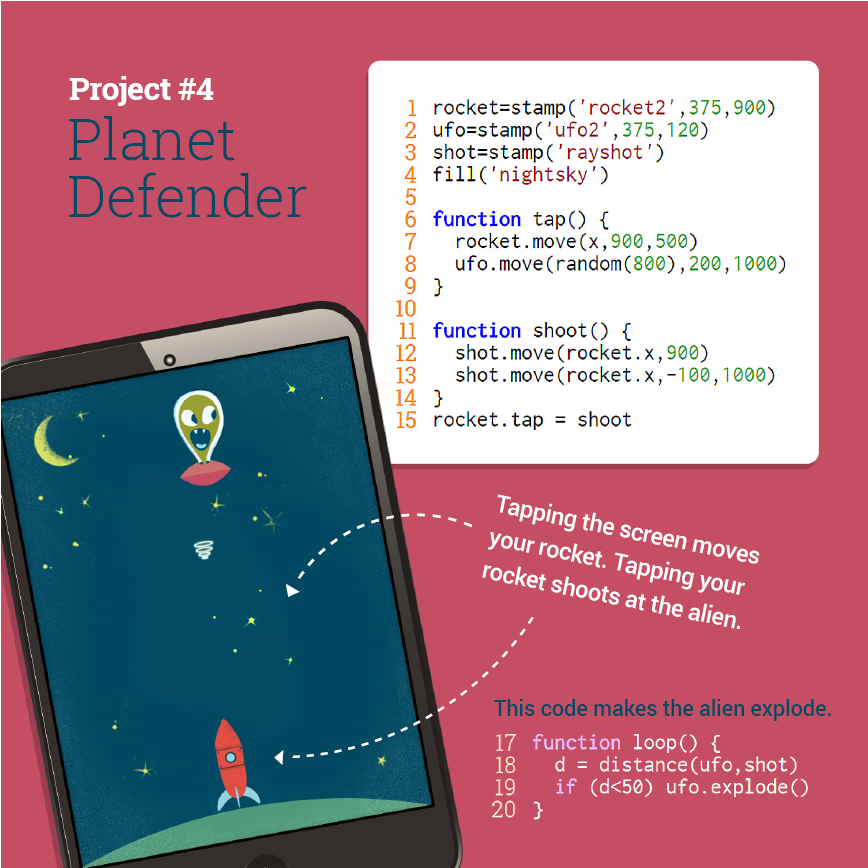
The Noisy
Reagan Eagle
But first... some learnin'
And a simple rule...
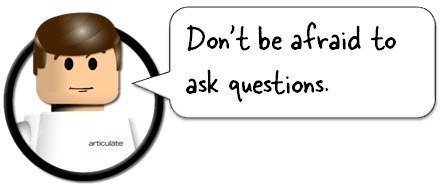
Everyone has questions
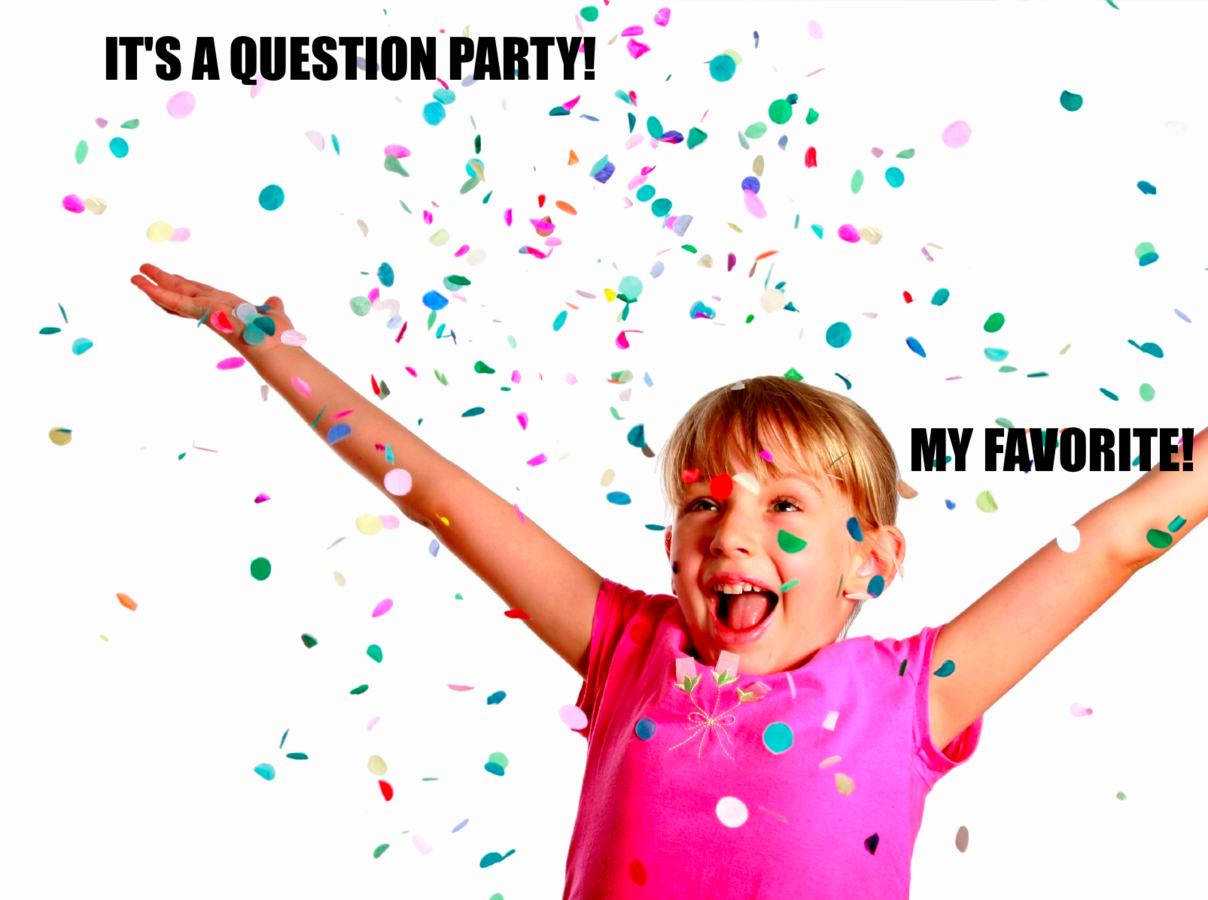
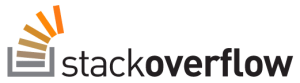
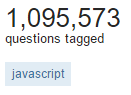
Now on with the learnin'
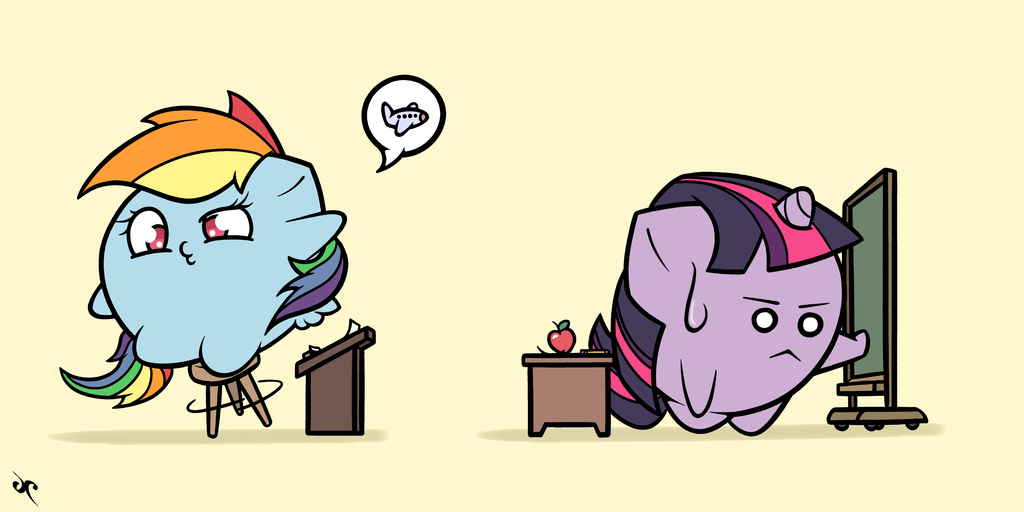
Functions
What is a function?
A set of instructions.
I need a volunteer...
It's just a fancy word for...
It's useful for not repeating yourself
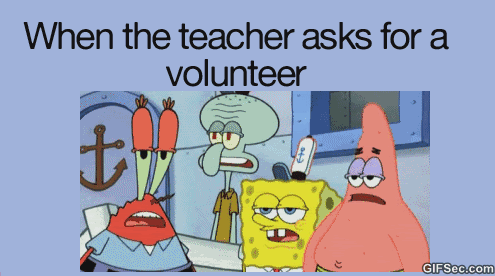
Anyone?
Do 10 jumping jacks
- Jump in the air and ...
- Spread your feet apart
- Put your arms above your head
- Jump in the air again and...
- Bring your feet together
- Bring your arms down by your side
We didn't have to say...
That's good. I hate typing.
The "fill" function
fill('red')
The "stamp" function
fill('red')
stamp('eagle')
The "sound" function
fill('red')
stamp('eagle')
sound('fox')
Read the Manuals
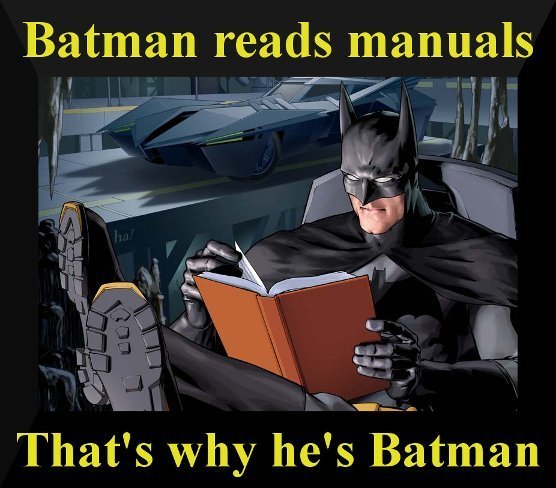
Being picky
Some functions have more options to help your vision
stamp('cat') //In the middle
stamp('cat', 1000) //In the middle, but bigger
stamp('cat', 200, 300, 50) //At X 200, Y 300, size 50
Tap
Drag
Loop
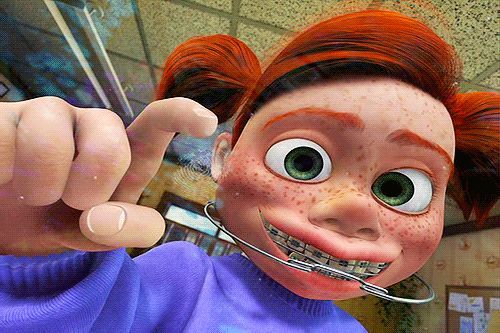
Tap Function
What happens when you tap the screen?
function tap() {
stamp('pig', x, y)
}
Drag Function
Loop Function
What happens when you drag your finger or mouse on the screen?
function drag() {
stamp('flare', x, y)
}
function loop() {
stamp('splat', 50).move()
}
What should happen over and over again and again?
Make your own function
Create, then use
// Create...
// Name the function anything you want
function newPet(pet) {
stamp(pet).move()
}
// Then use...
newPet('cow')
newPet('pig')
newPet('duck')
newPet('elephant')
newPet('dolphin')
// Typing the same long thing in
// over and over again
stamp('cow').move()
stamp('pig').move()
stamp('duck').move()
stamp('elephant').move()
stamp('dolphin').move()
Change your function
Just change it in a single spot
// Now make your pets sing
function newPet(pet) {
// Just add a .sing() to the end
stamp(pet).move().sing()
}
// No changes needed below
newPet('cow')
newPet('pig')
newPet('duck')
newPet('elephant')
newPet('dolphin')
// Here we need to add .sing() 5 times
// Imagine if you had an entire zoo!
stamp('cow').move().sing()
stamp('pig').move().sing()
stamp('duck').move().sing()
stamp('elephant').move().sing()
stamp('dolphin').move().sing()
Variables
The imaginary box you put thing in
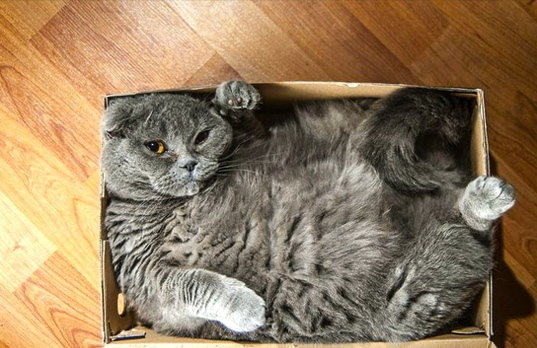
Name your box
Create a variable name,
put something in there,
do something with it
myPet = stamp('cat')
// We now have a variable called myPet
// Let's do something with it
myPet.sing()
function tap() {
// We can change the type of pet to something else
myPet.change(prompt('What kind of pet do you want?'))
myPet.sing()
}
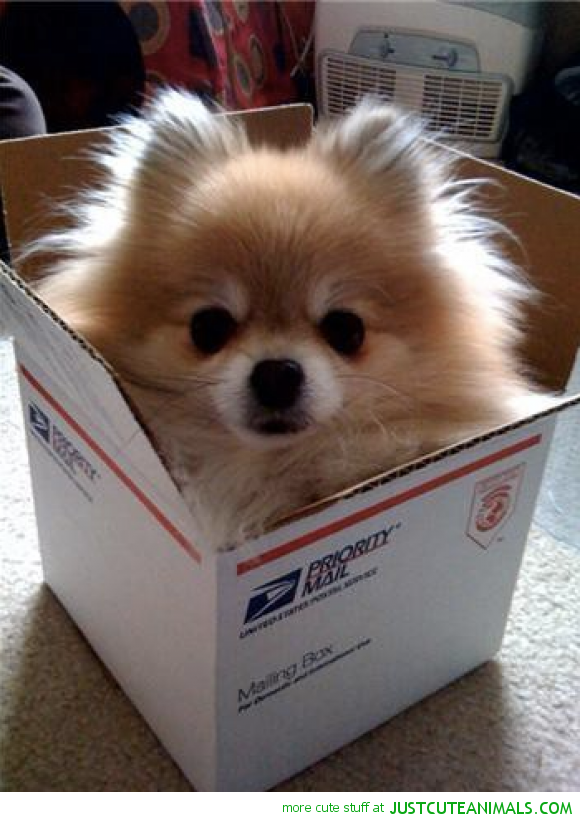
Logic
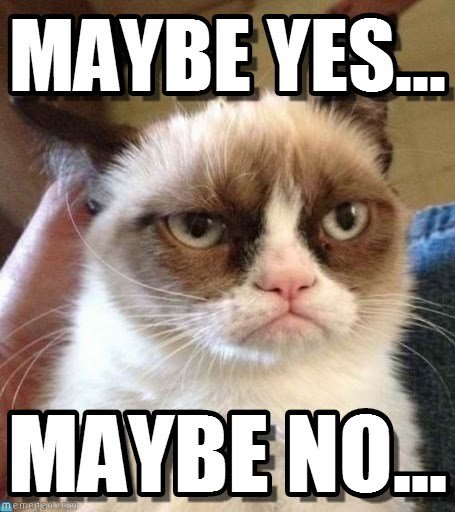
If/Else If/Else
What to do when something is true
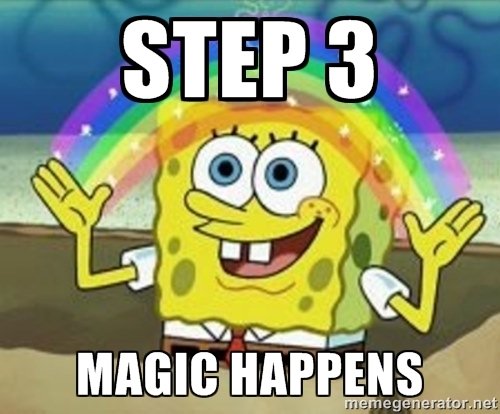
n = random(10)
if (n < 5) {
text('It was a low number')
} else if (n == 10) {
text('It was 10')
} else {
text('It was between 6 and 9')
}
Funny Mus{aches && s+uff
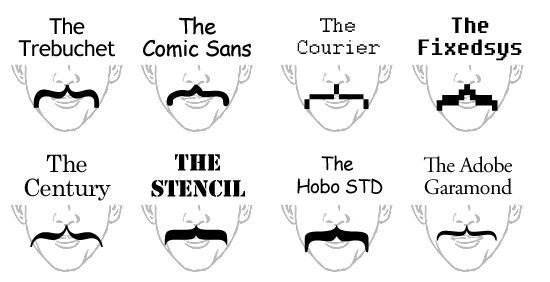
What's with the goofy symbols?
=
Make something be something
==
Don't worry about it :-)
= vs == vs ===
name = 'Derek'
//Now name is Derek
===
Check if something is the same as something else
winningScore = 10
if (score == winningScore) {
text('Hooray! You Won!!!')
}
&& means AND
Are both things true?
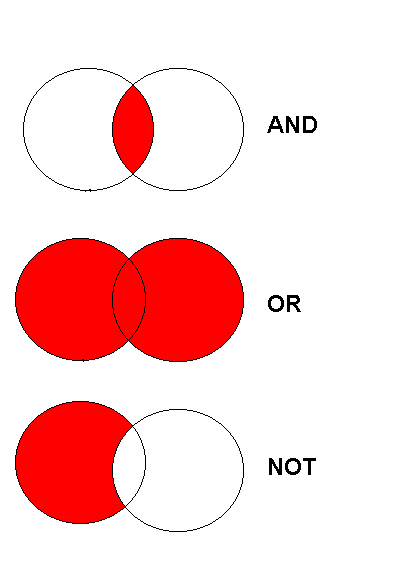
|| means OR
! means NOT
n = 10
if (isEven(n) && isTen(n))
n = 8
if (isEven(n) || isTen(n))
n = 8
if (isEven(n) && !isTen(n))
Is the thing NOT true?
Are either thing true?
Parens ()
( Parens ) are like bubbles. They keep things together.
Curly braces {}
{ Curly braces } are like walls. They keep things together.
What are all the things you want to happen when a player wins your game?
// If the player won
if (score == winningScore) {
text('Hooray! You Won!!!')
stamp('treasurechest')
sound('claps')
song('fanfare')
}
What are all the things you want to be true?
// If the player won
if (
( day == 'Wednesday'
|| day == 'Friday'
)
&&
( month == 'Oct'
|| month == 'Feb'
)
) {
text('Hooray! You Won!!!')
}
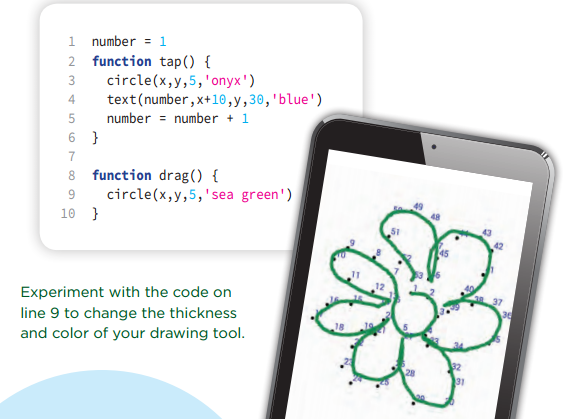
Connect the Dots
number = 1
function tap() {
circle(x,y,5,'onyx')
text(number,x+10,y,30,'blue')
number = number + 1
}
function drag() {
circle(x,y,5,'sea green')
}
text('By Derek', 650, 980, 20, 'blue', 'console', RIGHT)
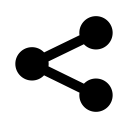
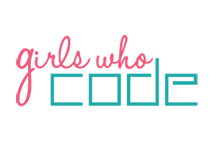
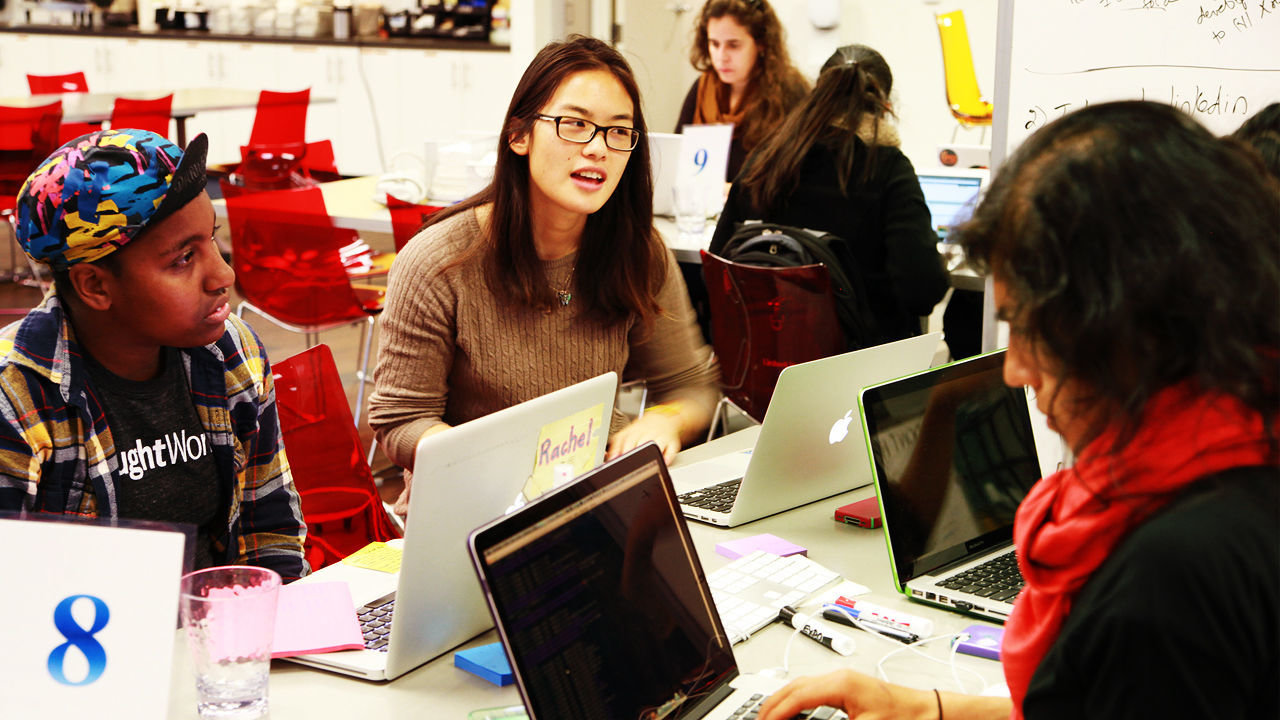
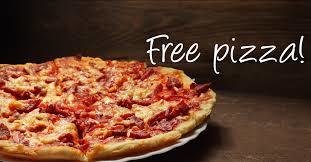
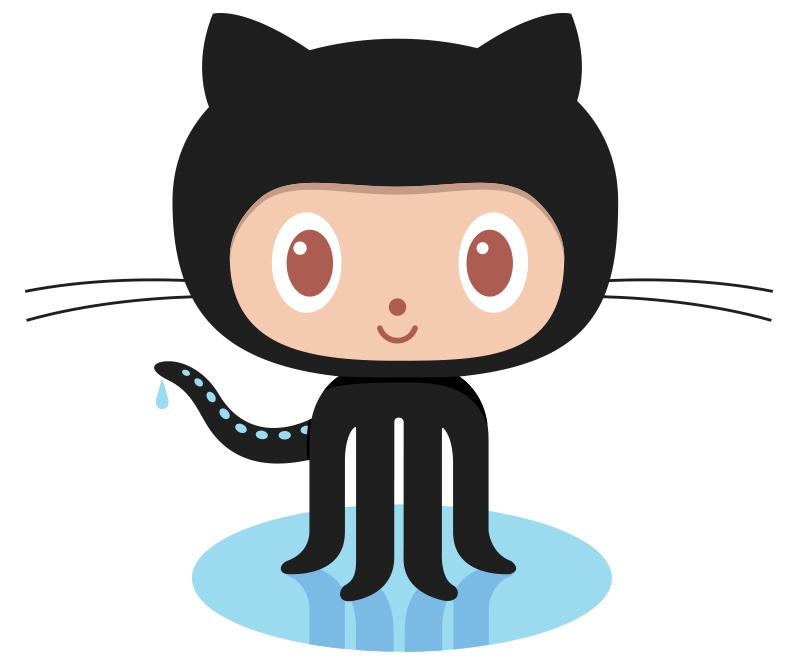
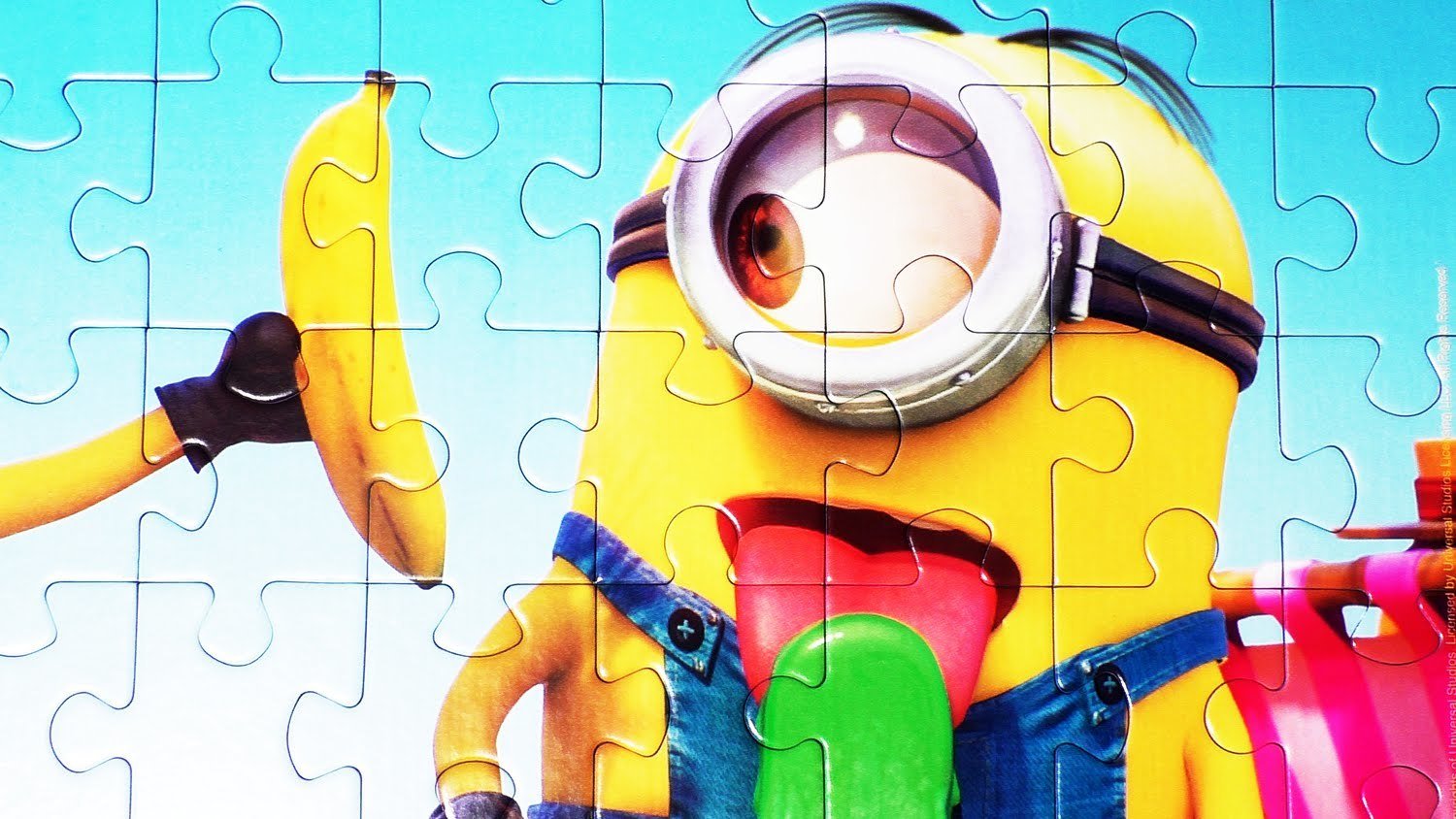
GS Coding April 2016
By Derek Eskens
GS Coding April 2016
- 2,058