jsf 2.0 introduction
http://docs.oracle.com/javaee/5/tutorial/doc/bnaph.html
Java Server faces
- JavaServer Faces technology is a server-side user interface component framework for Java technology-based web applications.
-
The main components of JavaServer Faces technology are as follows:
- An API for representing UI components and managing their state; handling events, server-side validation, and data conversion; defining page navigation; supporting internationalization and accessibility; and providing extensibility for all these features
- Two JavaServer Pages (JSP) custom tag libraries for expressing UI components within a JSP/xhtml page and for wiring components to server-side objects
What Is a JavaServer Faces Application?
- A set of pages (although you are not limited to using JSP pages as your presentation technology)
- A set of backing beans, which are JavaBeans components that define properties and functions for UI components on a page
- An application configuration resource file (faces-config.xml)
- A deployment descriptor (a web.xml file)
- Possibly a set of custom objects created by the application developer. These objects might include custom components, validators, converters, or listeners.
- A set of custom tags for representing custom objects on the page
facelets
Facelets is a page declaration language that is used to build JavaServer Faces views using HTML style templates and to build component trees .
It includes:
-
Use of XHTML for creating web pages
-
Support for Facelets tag libraries in addition to JavaServer Faces and JSTL tag libraries
-
Support for the Expression Language (EL)
-
Templating for components and pages
Tag Libraries Supported by Facelets
Tag Library |
Prefix |
Example |
Contents |
---|---|---|---|
JavaServer Faces Facelets Tag Library |
ui: |
ui:component ui:insert |
Tags for templating |
JavaServer Faces HTML Tag Library |
h: |
h:head h:body h:outputText h:inputText |
JavaServer Faces component tags for allUIComponentobjects |
JavaServer Faces Core Tag Library |
f: |
f:actionListener f:attribute |
Tags for JavaServer Faces custom actions that are independent of any particular render kit |
JSTL Core Tag Library |
c: |
c:forEach c:catch |
JSTL 1.2 Core Tags |
JSTL Functions Tag Library |
fn: |
fn:toUpperCase fn:toLowerCase |
JSTL 1.2 Functions Tags |
Facelets Templating Tags
Tag |
Function |
---|---|
ui:component |
Defines a component that is created and added to the component tree. |
ui:composition |
Defines a page composition that optionally uses a template. Content outside of this tag is ignored. |
ui:debug |
Defines a debug component that is created and added to the component tree. |
ui:decorate |
Similar to the composition tag but does not disregard content outside this tag. |
ui:define |
Defines content that is inserted into a page by a template. |
ui:fragment |
Similar to the component tag but does not disregard content outside this tag. |
ui:include |
Encapsulate and reuse content for multiple pages. |
ui:insert |
Inserts content into a template. |
ui:param |
Used to pass parameters to an included file. |
ui:repeat |
Used as an alternative for loop tags, such as c:forEach or h:dataTable. |
ui:remove |
Removes content from a page. |
facelets example
java server faces architecture
- JSF Includes:
- A set of UIComponent classes for specifying the state and behavior of UI components
- A rendering model that defines how to render the components in various ways
- An event and listener model that defines how to handle component events
- A conversion model that defines how to register data converters onto a component
- A validation model that defines how to register validators onto a component
User Interface Component Model
- JavaServer Faces UI components are configurable, reusable elements that compose the user interfaces of JavaServer Faces applications. A component can be simple, such as a button, or compound, such as a table, which can be composed of multiple components.
ui components
- Are used into the xtml/jsp pages
<html xmlns:h="http://java.sun.com/jsf/html" xmlns:f="http://java.sun.com/jsf/core">
<head>
<title>Ejemplo</title>
</head>
<body>
<h:form>
<h:commandButton value="Login"/>
<commandLink value="hyperLink"/>
</h:form>
</body>
</html>
Tag |
Rendered As |
---|---|
commandButton |
|
commandLink |
|
Model convertion
- When component is bound to an object, the application has two views of the component’s data:
- The model view , in which data is represented as data types, such as int or long.
- The presentation view , in which data is represented in a manner that can be read or modified by the user.
For example a java.util.Date might be represented as a text string in the format mm/dd/yyyy or as a set of three text string.


JSF Standard converters
- BigDecimalConverter
- BigIntegerConverter
- BooleanConverter
- ByteConverter
- CharacterConverter
- DateTimeConverter
- DoubleConverter
- FloatConverter
- IntegerConverter
- LongConverter
- NumberConverter
- ShortConverter
converters example
Event and Listener Model
- An Event object identifies the component that generated the event and stores information about the event.
- To be notified of an event an application must provide an implementation of the Listener class and must register it on the component that generates the event.
-
When the user activates a component, such as by clicking a button, an event is fired. This causes the JavaServer Faces implementation to invoke the listener method that processes the event.
kind of events
-
Action event
- Value change event
- Data model event
<h:selectOneMenu value="#{country.localeCode}" onchange="submit()">
<f:valueChangeListener type="com.mkyong.CountryValueListener" />
<f:selectItems value="#{country.countryInMap}" />
</h:selectOneMenu>
public void processValueChange(ValueChangeEvent event) {
//do something
}
event example
validation model
JavaServer Faces technology supports a mechanism for validating the local data of editable components (such as text fields).
This validation occurs before the corresponding model data is updated to match the local value. Like the conversion model, the validation model defines a set of standard classes for performing common data validation checks.
standard validator
- f:validateDoubleRange A component value is within a specified range.
- f:validateLength: The length of a component value is within a specified range.
-
f:validateLongRange: A component value is within a specified range.
<h:inputText value="#{bean.property}">
<f:validateDoubleRange minimum="0" maximum="100"/>
<f:validateLength maximum="5" minimum="5"/>
<f:validator validatorId="validadorSemestre" />
</h:inputText>
validators example
Navigation Model
Navigation is a set of rules for choosing the next page to be displayed after a button or hyperlink is clicked
-
Define the rules in the application configuration resource file. (faces-config.xml)
-
Refer to an outcome String from the button or hyperlink component’s action attribute. This outcome String is used by the JavaServer Faces implementation to select the navigation rule.
<h:commandButton
action="#{bean.navigation}" value="Submit" />
<navigation-rule>
<from-view-id>/greeting.jsp</from-view-id>
<navigation-case>
<from-outcome>success</from-outcome>
<to-view-id>/response.jsp</to-view-id>
</navigation-case>
</navigation-rule>
public String navigation(){
Usuario userAct = getUsuario();
executeLogicMethdo();
return"evxVerReporteT.xhtml";
}
navigation example
backing bean
-
A typical JavaServer Faces application includes one or more backing beans, each of which is a JavaServer Faces managed bean that is associated with the UI components used in a particular page.
-
Managed beans are JavaBeans components (see JavaBeans Components) that you can configure using the managed bean facility.
-
In addition to defining a no-arg constructor, as all JavaBeans components must do, a backing bean class also defines a set of UI component properties and possibly a set of methods that perform functions for a component.
backing bean
Each of the component properties can be bound to one of the following:
- A component’s value
- A component instance
- A converter instance
- A listener instance
- A validator instance
Example
public class ExampleBB {
private Integer userNumber = null;
private InputText inputText;
public ExampleBB() {
super();
}
public void setUserNumber(Integer user_number) {
userNumber = user_number;
}
public Integer getUserNumber() {
return userNumber;
}
public String getResponse() {
doSomething();
return "response.xhtml";
}
}
<h:inputText value="#{exampleBean.userNumber}"/>
<h:inputText binding="#{exampleBean.inputText;}"/>
backing bean
The most common functions that backing bean methods perform include the following:
-
Validating a component’s data
-
Handling an event fired by a component
-
Performing processing to determine the next page to which the application must navigate
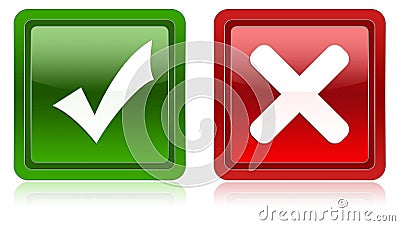
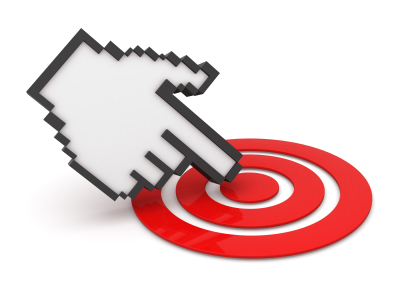
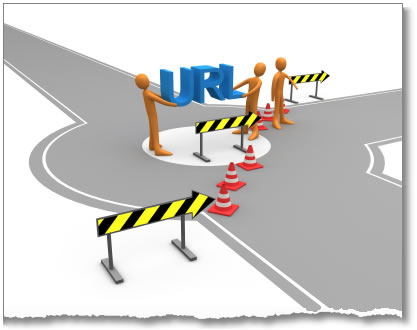
Using the Unified EL to Reference Backing Beans
To reference the backing bean from UI component tags, page authors use the unified expression language (EL) . Some of the features this language offers are.
- Deferred evaluation of expressions
- The ability to use a value expression to both read and write data
- Method expressions
<h:inputText id="userNo" value="#{UserNumberBean.userNumber}" validator="#{UserNumberBean.validate}" />
<inputText binding="#{UserNumberBean.userNoComponent}" />
backing bean scopes
- None
- Request
- View *
- Session
-
Application
The Life Cycle of a JavaServer Faces Page
The Life Cycle of a JavaServer Faces Page
-
A JavaServer Faces page is also different from a JSP page in that it is represented by a tree of UI components, called a view.
-
During the life cycle, the JavaServer Faces implementation must build the view while considering state saved from a previous submission of the page.
-
When the client submits a page, the JavaServer Faces implementation performs several tasks, such as validating the data input of components in the view and converting input data to types specified on the server side.
JavaServer Faces Standard Request-Response Life Cycle
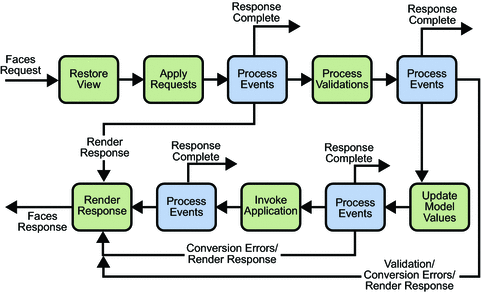
http://docs.oracle.com/javaee/5/tutorial/doc/bnaqq.html
jsf 2.0 Introduction
By Diego Montoya
jsf 2.0 Introduction
A Brief introduction to JSF 2.0 Based in the official oracle tutorial
- 924