

AutoMapper is an open source software library that automates the mapping of one class to another.
What are you?
SourceType sourceObject = new SourceType();
DestinationType autoMappedResult = Mapper.Map<DestinationType>(sourceObject);
In different words...
AutoMapper is a tool that will take as an argument an object of a specific type , then return an instance of a different type where the field values of that returned object will match (or will have been mapped from) the field values of the passed object.
The Mapping is based on a configuration.
SourceType sourceObject = new SourceType();
DestinationType autoMappedResult = Mapper.Map<DestinationType>(sourceObject);
Configuration:
AutoMapper maps values from one object to another based on it's configuration. Configuration, in it's most simple form, is done by matching field values that have the same name.
Mapper.Initialize(cfg =>
{
cfg.CreateMap<SourceType, DestinationType>();
});
CreateMap<PlayerProjection, Player>()
.ForMember
(
dest => dest.Decider,
opt => opt.MapFrom(src => src.IsDecider)
)
.ForMember
(
dest => dest.Game,
opt => opt.MapFrom(src => logic.getGame(src.Game.Id))
)
.ForMember
(
dest => mulliganCount,
opt => opt.MapFrom(src =>
src.HasMulligan ? 1: 0)
);
SourceType sourceObject = new SourceType();
DestinationType autoMappedResult = Mapper.Map<DestinationType>(sourceObject);
In the case where the destination type cannot be mapped to the source type by the default configuration profile, AutoMapper allows for a high degree of relatively sophisticated customization using .ForMember which allows values to be mapped:
- when the names don't match
- using the results of a provided calculation
- Mapping a destination value to a field value from within an object type field in the source class
Mapper.Initialize(cfg =>
{
cfg.CreateMap<SourceType, DestinationType>();
})
.ForMember
(
dest => dest.memberName1,
opt => opt.memberNameOne
);
No Profile
With Profile
Different Configuration styles
Mapper.Initialize(cfg =>
{
cfg.AddProfile<CardToCardProjection>();
});
public class CardToCardDTO : Profile
{
public CardToCardDTO()
{
CreateMap<CardInHand, CardDTO>()
.ForMember
(
dest => dest.CardType,
opt => opt.MapFrom(src => src.CardType.Name)
);
}
}
Mapper.Initialize(cfg =>
{
cfg.CreateMap<User, UserDTO>();
});
Testing
- Mapper.AssertConfigurationIsValid();
- You can run this after a configuration
- This will assert that every field in the destination class has a match source
What does it take to use it?
- Configure once per project
- Call configuration once per Domain
- Call Mapper.Map as much as you like
using System;
using AutoMapper;
namespace AutoMapperExample
{
public class Program {
static void Main(string[] args) {
User user = new User {
name = "Jane Doe",
ssn = "123-45-6789",
id = "31415",
numberOfChildren = 27
};
Mapper.Initialize(cfg =>
{
cfg.CreateMap<User, UserDTO>();
});
UserDTO userDTO = Mapper.Map<UserDTO>(user);
Console.WriteLine(userDTO.name );
Console.WriteLine("Press enter to close...");
Console.ReadLine();
}
}
}
THINGS TO BE MINDFUL OF
- Make sure that the AutoMapper NuGet package has been applied to the part of the solution you are working in
- Make sure that the configuration file has been called for the part of the solution you are working in
- There were some significant changes around version 4.2 and then again around version 5. This may affect the applicability of a large number of the examples you find on the Internet.
The changes were
- As of AutoMapper 4.2 Mapper.CreateMap() is now obselete and 'Mapper.Map<TSource, TDestination>(TSource, TDestination)' is obsolete.
- As of Version 5.0, 'The static API will be removed.
- These are referenced on the confluence page
How do I implement this in a project properly?
With regards to Numetrix
- Where to place the configuration file
- Where to place the call to the config file
-
Do we wish to use profiles (recommended), if so, we should pick a meaningful naming schema
In Summery...
AutoMapper is a library meant to make a tedious programming task easier. Once configured, it is extremely easy to use to instantiate an object that has prefilled values. Most of the "work" of AutoMapper is done in the configuration, which is is also where design pattern type choices can be me made.
End AutoMapper Presentation
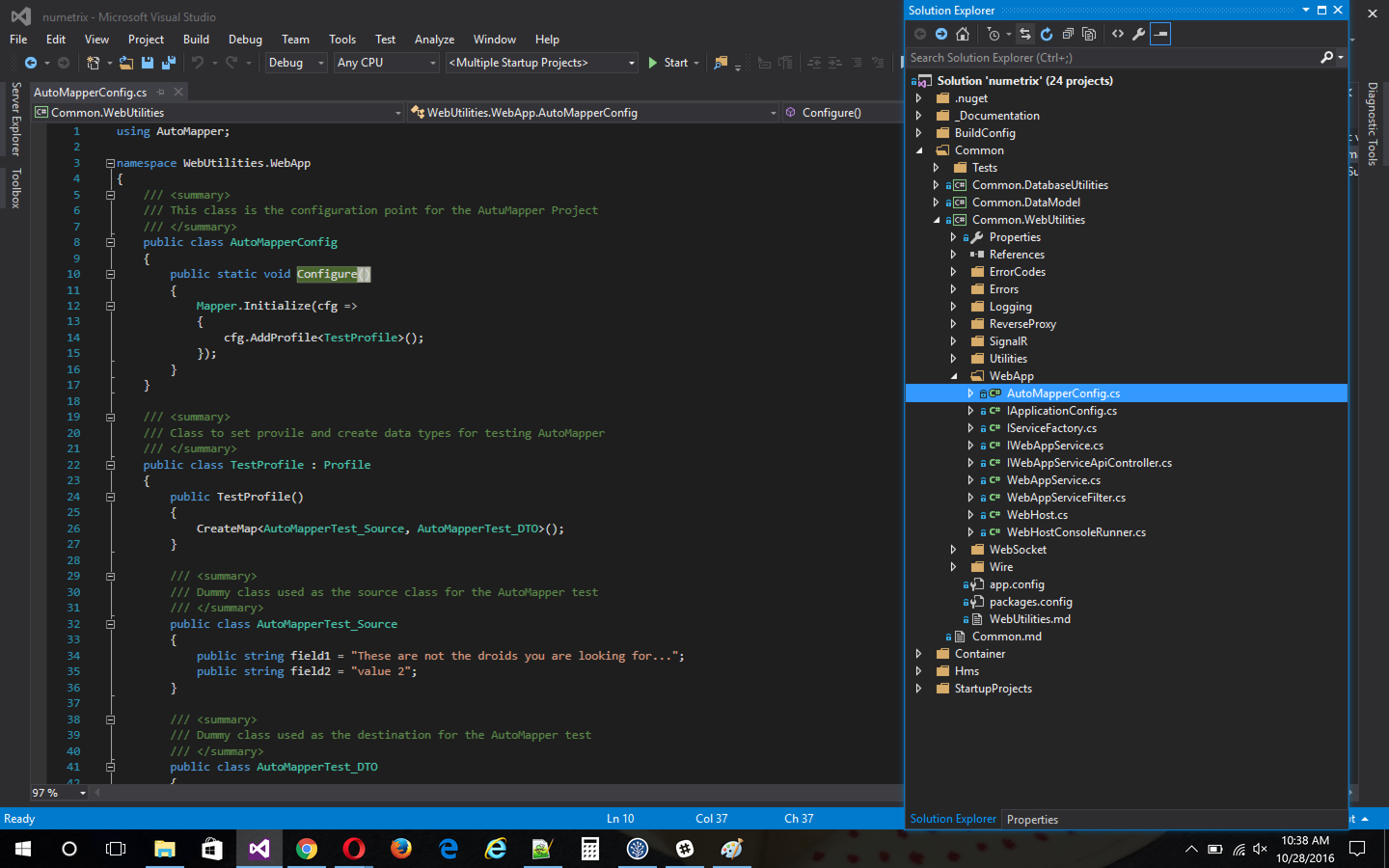
Config File
The config Call
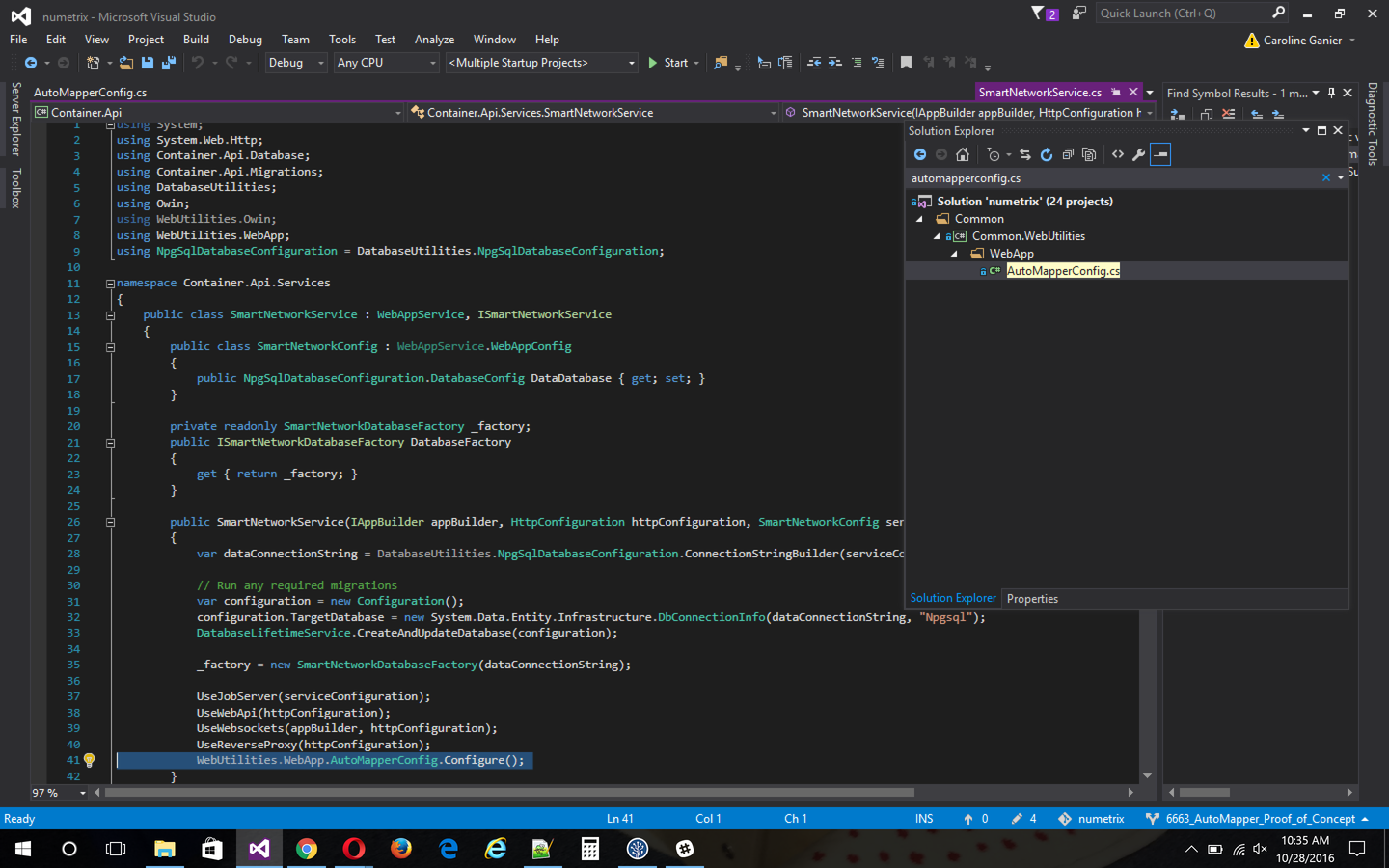
[Test]
public void TestAutoMapper()
{
AutoMapperConfig.Configure();
AutoMapperTest_Source testSourceObject = new AutoMapperTest_Source();
AutoMapperTest_DTO result = Mapper.Map<AutoMapperTest_DTO>(testSourceObject);
result.field1.ShouldContain("the droids you are looking for");
}
Using it
AutoMapper
By eightgate
AutoMapper
- 26