RxJS library
RxJS - what is it
- Reactive programming
- Async / event based
- Observable sequences
- Observer pattern
-
Iterator pattern
- Functional programming
RxJS - based on
Observer pattern
Subject
Observers
Subject
Observers
state = 'A'
Subject
Observers
state = 'A'
Subject
Observers
Iterator pattern
Iterator
Container
Iterator
Container
Iterator
Container
Iterator
Container
Iterator
Container
Functional programming
x
y = filter( x % 2)
z = reduce( x + y)
RxJS - main classes
- Observable
- Observer
- Subscription
- Operators
- Subject
- Schedulers
RxJS - main actors
import { interval } from 'rxjs';
const observable = interval(1000);
const subscription = observable.subscribe( x => console.log(x) );
setTimeout(()=> { subscription.unsubscribe() }, 4000); //cancel stream in 4s
Observable
that emits 1 event per second
Observer
that prints out every event
Subscription
use of Subscription object
to cancel data stream
// output (one value every second): 0, 1, 2, 3
RxJS - main actors
import { interval } from 'rxjs';
const observable = interval(1000);
const subscription = observable2.subscribe( x => console.log(x) );
setTimeout(()=> { subscription.unsubscribe() }, 4000);
Observable
Observer
Subscription
// output (one value every second): 0.74, 0.23, 0.91, 0.47
import { map } from 'rxjs/operators';
const observable2 = observable.pipe( map( x=> Math.random() ) );
Operator
Observable
RxJS - multiple subscriptions
import { interval } from 'rxjs';
const observable = interval(1000).pipe( map( x=> Math.random() ) );
const s1 = observable.subscribe( x => console.log( "[obs1]: " + x ) );
const s2 = observable.subscribe( x => console.log( "[obs2]: " + x ) );
setTimeout(()=> { subscription1.unsubscribe() }, 4000);
setTimeout(()=> { subscription2.unsubscribe() }, 4000);
Subscription
import { map } from 'rxjs/operators';
Subscription
[obs1]: 0.047 [obs2]: 0.862
[obs1]: 0.653 [obs2]: 0.134
[obs1]: 0.752 [obs2]: 0.584
[obs1]: 0.714 [obs2]: 0.821
RxJS - subject
import { interval, Subject } from 'rxjs';
import { map } from 'rxjs/operators';
const observable = interval(1000).pipe( map( x=> Math.random() ) );
const s1 = subject.subscribe( x => console.log( "[obs1]: " + x ) );
const s2 = subject.subscribe( x => console.log( "[obs2]: " + x ) );
setTimeout(()=> { s1.unsubscribe() }, 4000);
setTimeout(()=> { s2.unsubscribe() }, 4000);
Subject is an observer
[obs1]: 0.106 [obs2]: 0.106
[obs1]: 0.645 [obs2]: 0.645
[obs1]: 0.125 [obs2]: 0.125
[obs1]: 0.749 [obs2]: 0.749
const subject = new Subject();
observable.subscribe(subject);
Subject
Subject is also an observable
RxJS - main actors
import { interval, Subject } from 'rxjs';
import { map } from 'rxjs/operators';
const observable = interval(1000).pipe( map( x=> Math.random() ) );
const s1 = subject.subscribe( x => console.log( "[obs1]: " + x ) );
const s2 = subject.subscribe( x => console.log( "[obs2]: " + x ) );
setTimeout(()=> { s1.unsubscribe() }, 4000);
setTimeout(()=> { s2.unsubscribe() }, 4000);
Observable
const subject = new Subject();
observable.subscribe(subject);
Subject
Subscription
Operator
Observer
RxJS - subscribe to execute
import { interval } from 'rxjs';
import { tap } from 'rxjs/operators';
const observable = interval(1000).pipe( tap ( x => console.log( x ) ) );
//output: [None]
- Observer pattern
-
Iterator pattern
- Functional programming
RxJS - based on
RxJS - observables work like iterators
import { interval } from 'rxjs';
const observable = interval(1000);
observable.subscribe( x=> console.log(x) );
Observer
every next(x) value
from Iterator
calls
console.log(x);
RxJS - observables work like iterators
import { interval } from 'rxjs';
import { take } from 'rxjs/operators';
const observable = interval ( 1000 ) .pipe ( take ( 4 ) );
const observer = {
next: x => console.log ( x ),
error: err => console.error ( err ),
complete: ( ) => console.log ( 'done' )
};
Observer
observable.subscribe( observer );
//output: 0, 1, 2, 3, done
RxJS - observable VS iterator
while( iterator.hasNext( ) ) {
Iterator
Observable.create( (observer) =>{
});
Observable
let value = iterator.next( ) ;
console.log( value ) ;
}
observer.next("value1");
observer.complete();
observer.next("value2");
RxJS - Observable is a PUSH system
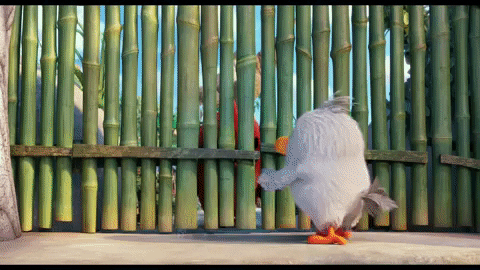
RxJS - Pull VS Push
Consumer
Pull
Producer
giveMeData();
return data;
giveMeData();
return data;
RxJS - Pull VS Push
Consumer
Push
Producer
subscribe();
sendSomeData();
sendSomeData();
sendSomeData();
RxJS - Observable is a PUSH system
import { interval } from 'rxjs';
const observable = interval ( 1000 ) ;
observable.subscribe( x => console.log( x ) );
RxJS - Observable is a PUSH system
import { Observable } from 'rxjs';
my custom interval observable
const observable = my5Interval ( 1000 ) ;
observable.subscribe( x => console.log( x ) );
//output: 0, 1, 2, 3, 4, 5, ...
function my5Interval(ms){
}
return Observable.create( (observer) => {
});
let count = 0;
const id = setInterval( ( )=>{
}, ms);
observer.next(count);
count++;
Observable
uses
observer.next
to push data stream
if(count >=5 ){
clearInterval( id );
observer.complete();
}
Observable
pushes end
of data with
observer.complete
- Observer pattern
-
Iterator pattern
- Functional programming
RxJS - based on
RxJS - main actors
- Observable
-
Operators
- Observer
- Subscription
- Subject

Aprende más en:
https://www.udemy.com/rxjs-nivel-pro/?couponCode=RXJS-SLIDESHARE
RxJS library
By Enrique Oriol
RxJS library
- 55