CSS Magic 🧙♂️
&
Futuristic
Javascript 🤖
ECMAScript
Javascript
ECMAScript
- An implementation of the specification
- Reading its docs teaches you how to write code with that language
- Think: A physical Keyboard
- A Crowd-Sourced Specification
- Reading its docs teaches you how to write a language
- Think: The QWERTY standard
ECMAScript is...
- Implemented in multiple languages (Javascript is the BIG one)
- Open to proposals from the community
- On an annual release cycle since 2015
Adding Features to ES
TC39 👩⚖️👨⚖️
People Like Us 👨👩👧👦
In Spec 🖥
Stage 0 Strawman
Stage 1 Proposal
Stage 2 Draft
Stage 3 Candidate
Stage 4 Finished
Released
Some Proposal Metrics
Most | Least | |
---|---|---|
Time (Years) | Async Await (4+) | Dot-All flag for Regexps (1.5) |
Commits | Shared Memory & Atomics (364) | Lifting Template Literal Restriction (19) |
Time from Stage 0 to Stage 4
(as of 4/10/2018)
Some Proposals of Interest
A Quick Survey 🚌 🐯🦁🐼
Stage 4: Finished
Number in Stage: 8 (as of 4/6/18)
Indicate[s] that the addition is ready for inclusion in the formal ECMAScript standard.
Async Iteration
by Domenic Denicola
Allows one to sequentially map over an array of resolved asynchronous actions
* Can only be used inside an async body
const endpoints =
["/api/a", "/api/b", "/api/c"];
const fetches = endpoints.map(url =>
fetch(url)
);
for await (const data of fetches) {
// Access each asynchronous object
// one at a time, in sequence.
}
Stage 4 | Docs
Promise.prototype.finally
by Jordan Harband
Execute a callback at the end of a promise chain, no matter what
Similar to try...catch...finally
fetch("/a-land-down-under")
.then(() => doTheGood())
.catch(() => handleTheBad())
// Executed no matter what
.finally(() => cleanup());
Stage 4 | Docs
Stage 3: Candidate
Number in Stage: 14 (as of 4/6/18)
The proposal is mostly finished and now needs feedback from implementations and users to progress further.
import()
by Domenic Denicola
Allows javascript to not be loaded until you need it
Some Benefits:
- Faster app startup e.g. defer loading of non-essential modules
- Load only if needed
- Robustness e.g. app survives even if non-critical module fails to load
if (absolutelyNecessary) {
import("large-module.js");
}
Stage 3 | Docs
Global
by Jordan Harband
Allows code accessing the global object to be portable across runtime environments e.g. browsers, node, shell, etc.
// Current Way (doesn't work in all cases)
const getGlobal = () => {
if (typeof self !== 'undefined') {
return self;
}
if (typeof window !== 'undefined') {
return window;
}
if (typeof global !== 'undefined') {
return global;
}
throw new Error(
'unable to locate global object'
);
};
const global = getGlobal();
// New Way (Intended to work in all cases)
// Note: May not end up being called
// 'global' in the official spec
const global = global;
Stage 3 | Docs
Private Fields
by Daniel Ehrenberg & Kevin Gibbons
Private member variables & methods for Javascript classes (prefixed with a '#')
class Fetcher {
// PUBLIC
fetchAll = user =>
user.isAdmin
? this.#fetchAll()
: this.#fetchFiltered()
// PRIVATE
#serviceUrl =
"external-api/a?filter=all";
#filteredServiceUrl =
"external-api/a?filter=publicOnly";
#fetchAll = () =>
fetch(this.#serviceUrl);
#fetchFiltered = () =>
fetch(this.#filteredServiceUrl);
}
Stage 2: Draft
Number in Stage: 8 (as of 4/6/18)
A first version of what will be in the specification. At this point, an eventual inclusion of the feature in the standard is likely.
Decorators
by Daniel Ehrenberg
"...[lets] frameworks and libraries implement part of the behavior of classes..."
Similar space as Spring Boot Annotations
class Thing {
@notnull id;
@size('100') name;
constructor(id, name) {
this.id = id;
this.name = name;
}
}
Stage 2 | Docs
Stage 1: Proposal
Number in Stage: 41 (as of 4/6/18)
TC39 [is willing] to examine, discuss and contribute to the proposal. Going forward, major changes to the proposal are expected.
Optional Chaining
by Gabriel Isenberg & Claude Pache
Simplified null checking during object traversal
// Something like this...
const name = users
? users[id]
? users[id].names
? users[id].names.givenName
: undefined
: undefined
: undefined;
// Can become this...
const names = users?.[id]?.names?.givenName;
// The optional chaining
// operator '?.' is key.
// Can even be used for
// optional function calls...
myFunction?.(arg1, arg2);
Stage 1 | Docs
Realms
by Caridy Patiño & Jean-Francois Paradis
Isolated Javascript environments with their own copies of the global object, standard library, and the intrinsics
Some Benefits:
- Security isolation e.g. in-browser code editors
- Testing/mocking
Stage 1 | Docs
Temporals
by Maggie Pint & Matt Johnson
A better date and time standard for ES
Some Benefits:
- Support for non-local time zones
- More reliable parsing
- More intuitive computation APIs
// Civil Time Objects
// (no time zones)
new CivilDate();
new CivilTime();
new CivilDateTime();
// Construction
new CivilDate(year, month, day);
// Accessing the parts
let year = civilDate.year;
let month = civilDate.month;
let day = civilDate.day;
// Computations
myDate.add({ years: 1, months: 2 });
// Absolute Time Objects
// (includes time zones)
new Instant();
new ZonedInstant();

Stage 1 | Docs
Pipeline Operator
by Daniel Ehrenberg
"...a useful syntactic sugar on a function call with a single argument."
// Some Functions
const doubleSay => str => {
return str + ", " + str;
}
const capitalize = str => {
return str[0].toUpperCase()
+ str.substring(1);
}
const exclaim = str => {
return str + '!';
}
// Traditional Way
let result =
exclaim(capitalize(doubleSay("hello")));
// result === "Hello, hello!"
// Using Pipeline Operator
let result = "hello"
|> doubleSay
|> capitalize
|> exclaim;
// result === "Hello, hello!"
Stage 1 | Docs
Transition
Javascript Only? 🧐
No! 🎊😆🎊
CSS😆
HTML😆
...😆
💡 ➡ (🕵️♂️</>)*↺ ➡ 🏅🖥 = 😆
CSS Houdini
What Is Houdini
The Extensible Web Manifesto
We—the undersigned—want to change how web standards committees create and prioritize new features. We believe that this is critical to the long-term health of the web.
We aim to tighten the feedback loop between the editors of web standards and web developers.
Today, most new features require months or years of standardization, followed by careful implementation by browser vendors, only then followed by developer feedback and iteration. We prefer to enable feature development and iteration in JavaScript, followed by implementation in browsers and standardization.
To enable libraries to do more, browser vendors should provide new low-level capabilities that expose the possibilities of the underlying platform as closely as possible.
Houdini... gives developers direct access to the browser’s CSS engine. This gives developers the power to create their own custom CSS features that run efficiently within the browser’s native rendering pipeline.
Houdini:
Who Is Behind Houdini?


Benefits
Outsourced Innovation
Speed up Innovation in the CSS space 🔥🚀🔥
CSS Polyfills
Allow cool features to be used before they're officially implemented in the browser 😍
Normalization
normalize cross-browser differences
Basic Mechanics
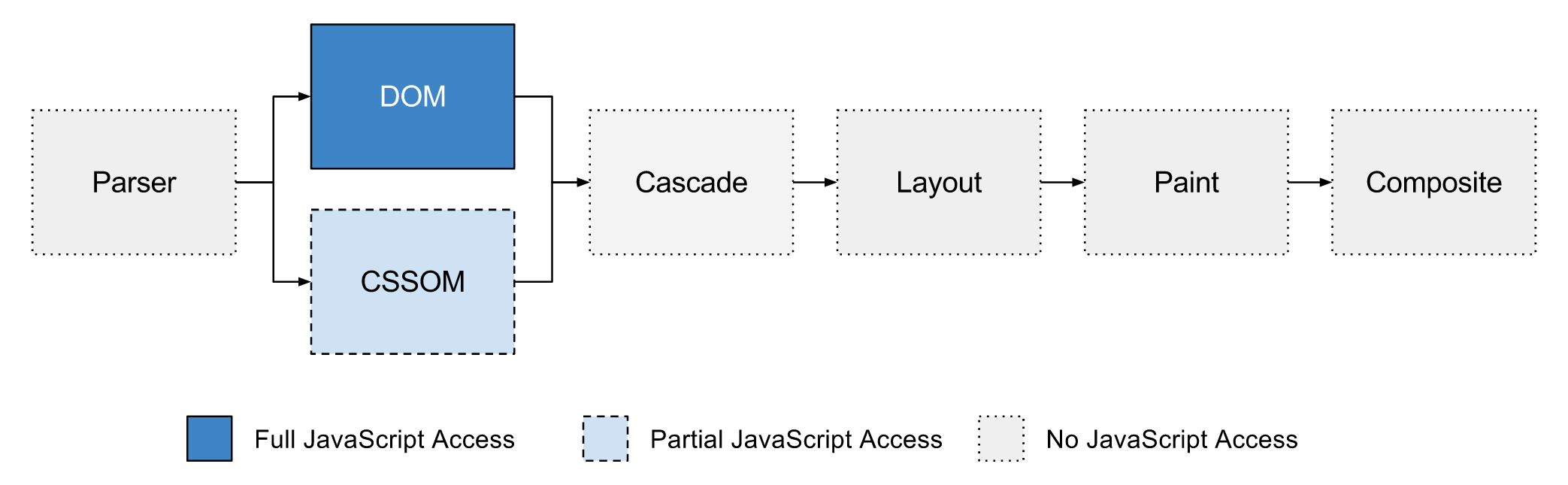
Current Access to The Rendering Pipeline

* As of 4/10/2018, there is now an AnimationWorklet W3C proposal and implementation in Chrome Canary
*
Access to The Rendering Pipeline With Houdini

{
class PlaceholderBoxPainter {
paint(ctx, size) {
ctx.lineWidth = 2;
ctx.strokeStyle = '#666';
// draw line from top left to bottom right
ctx.beginPath();
ctx.moveTo(0, 0);
ctx.lineTo(size.width, size.height);
ctx.stroke();
}
}
registerPaint('placeholder-box', PlaceholderBoxPainter);
Worklet
- Cached by browser
- Run by CSS engine, independent of main thread
- No access to DOM and many globals

if ('registerProperty' in CSS) {
// Properties and Values API
// is supported
CSS.registerProperty({
name: '--tooth-width',
syntax: '<length>',
initialValue: '40px'
});
}
Custom Property
- Typed
- Supports `calc()` expressions
- Useable by worklets
- Animatable by keyframes
Examples
Paint API: Placeholder Background
Paint API + Properties & Values API:
Toothed Border
Houdini + React 🎨
process.exit()
(The End)
References
- https://tc39.github.io/process-document/
- https://github.com/tc39/proposals
- https://babeljs.io/docs/plugins/preset-stage-3/
- https://babeljs.io/docs/plugins/preset-stage-2/
- https://babeljs.io/docs/plugins/preset-stage-1/
- https://medium.freecodecamp.org/whats-the-difference-between-javascript-and-ecmascript-cba48c73a2b5
- https://commons.wikimedia.org/
ECMAScript:
References Cont.
- https://extensiblewebmanifesto.org/
- https://ishoudinireadyyet.com/
- https://codersblock.com/blog/say-hello-to-houdini-and-the-css-paint-api/
- https://commons.wikimedia.org/
- https://www.smashingmagazine.com/2016/03/houdini-maybe-the-most-exciting-development-in-css-youve-never-heard-of/
- https://drafts.css-houdini.org/css-properties-values-api/#supported-syntax-strings
- http://slides.com/malyw/houdini-codemotion#/
CSS Houdini:
CSS Magic & Futuristic Javascript
By Evan Peterson
CSS Magic & Futuristic Javascript
There are some exciting up and coming features in Javascript and CSS in the next couple years, including CSS Houdini.
- 1,903