FIREFOX OS
WEB APIs & WEB ACTIVITIES
BORING STUFF
Fernando Campo
HTML5 Developer
Telefonica Digital


@ferkhamp
fcampo
firefox os 101

basics
- The web is the platform
- HTML5 / CSS3 / Javascript
- Open Web Apps
- Hosted VS Packaged
- manifest.webapp
{
"name": "My App",
"description": "My elevator pitch goes here",
"launch_path": "/",
"icons": {
"128": "/img/icon-128.png"
},
"developer": {
"name": "Your name or organization",
"url": "http://your-homepage-here.org"
},
"default_locale": "en"
}
everybody loves apis
OPEN API GROWTH

Expected around 7000 APIs in 2013
2013: 9888 APIs 7169 Mashups
BROWser apis

do we have enough?
nope!

new features,
new apis

it's a phone !
Telephony
Messages
Contacts
Battery
it's a smartphone!
Mobile connectivity
WiFi connectivity
Browser
Camera
Device Storage
Alarms
Keyboard
Settings
IT's a smartphone
full of sensors!
Vibration
Screen Orientation
Ambient Light
Proximity
Geolocation
Bluetooth
NFC
reminder: web standards
Mozilla uses standarized APIs when possible
If not, it proposes new APIs for standarization
Most of them will change with time, during the process
Using moz- prefix to avoid future collisions
firefox WEB apis
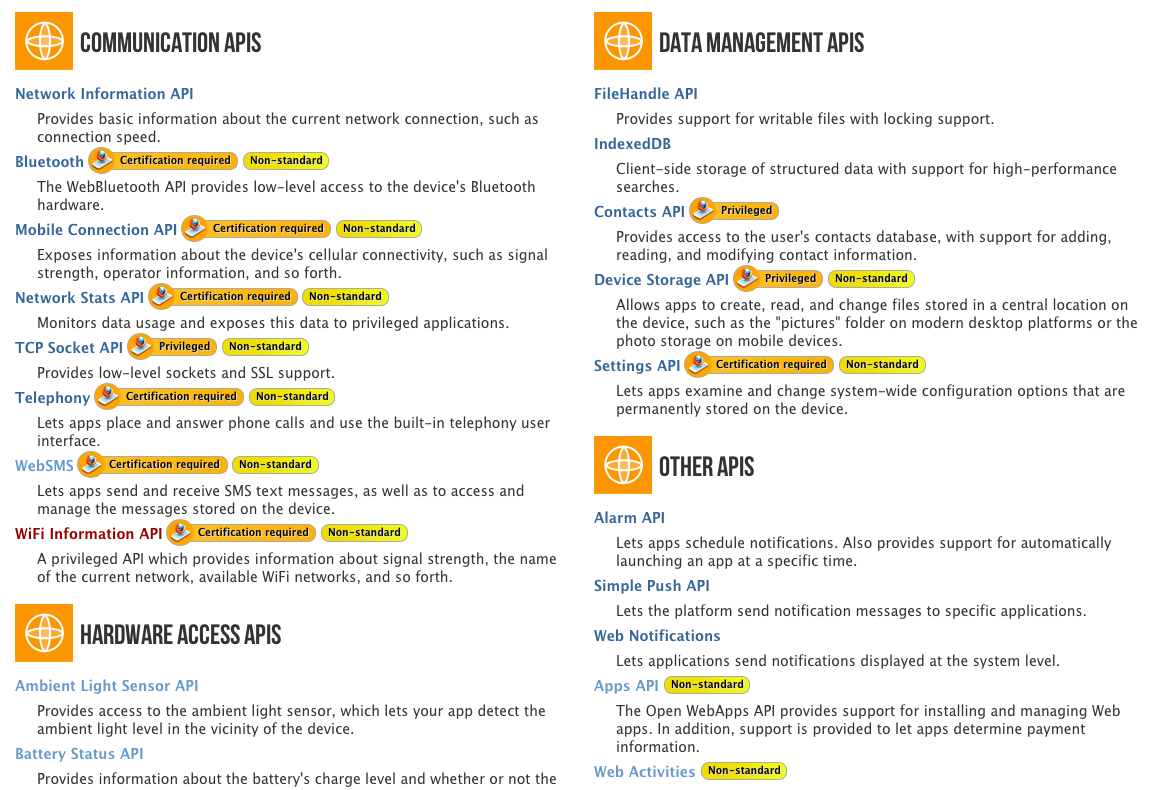
https://developer.mozilla.org/en-US/docs/WebAPI
access granted?
NOPE!

security by default

firefox web app
Hosted app
-
Internet origin
-
Remote resources
- Local manifest
- Access to safe APIs
Packaged app
-
Installed on device
-
Local resources (zip file)
- Different levels of security
api safety
Web
-
Signed by app store, no authentication
-
Same as hosted, access only safe APIs
Privileged
- Approved by Mozilla Marketplace
- Access to sensitive APIs
- Tight CSP (no inline scripts)
Certified
- Approved by vendor
- Access to critical APIs
- Loosened CSP
permissions
Now that you have clearance...what do you want?
{
"name": "My App",
"description": "My elevator pitch goes here",
"launch_path": "/",
"icons": {
"128": "/img/icon-128.png"
},
"developer": {
"name": "Your name or organization",
"url": "http://your-homepage-here.org"
},
"type": "privileged",
"permissions": {
"alarms": {},
"browser":{},
"power":{},
"fmradio":{},
"webapps-manage":{},
"mobileconnection":{},
"bluetooth":{},
"telephony":{},
"voicemail":{},
"device-storage:sdcard":{ "access": "readonly" },
"device-storage:pictures":{ "access": "readwrite" },
"device-storage:videos":{ "access": "readwrite" },
"device-storage:music":{ "access": "readcreate" },
"device-storage:apps":{ "access": "readonly" },
"settings":{ "access": "readwrite" },
"storage":{},
"camera":{},
"geolocation":{},
"wifi-manage":{},
"desktop-notification":{},
"idle":{},
"network-events":{},
"embed-apps":{},
"background-sensors":{},
"permissions":{},
"audio-channel-notification":{},
"audio-channel-content":{},
"cellbroadcast":{},
"keyboard":{}
}
}
Full list with needed privileges on
[https://developer.mozilla.org/en-US/docs/Web/Apps/App_permissions]
it's business time
battery
First, get the object
var battery = navigator.mozBattery;
Check the current level
var batteryLevel = battery.level * 100 + "%";
Listen to events
battery.addEventLister("levelchange", setStatus, false);
battery.addEventLister("chargingchange", setStatus, false);
vibration
navigator.vibrate(1000); //one second
navigator.vibrate([1000, 200, 500]); //pattern
navigator.vibrate(0); //stop the vibration
ambient light
window.addEventListener("devicelight", function(event) {
// event.value contains the detected lux values
// consider 'dim' when below 50, 'bright' above 10000
});
device storage
Available storages: 'music', 'pictures', 'videos', 'apps', 'sdcard'
var sdcard = navigator.getDeviceStorage('sdcard');
var request = sdcard.get("my-file.txt");
request.onsuccess = function () {
var file = this.result;
console.log("Found the file: " + file.name);
}
request.onerror = function () {
console.warn("Unable to get the file: " + this.error);
}
We can also check for free space
var videos = navigator.getDeviceStorage('videos');
var request = videos.usedSpace();
request.onsuccess = function () {
var size = this.result / 1000000; // Bytes to Mb
console.log("Your videos are using " + size.toFixed(2) + "Mb");
}
contacts
get the ContactManager object
var contactsAPI = navigator.mozContacts;
create a new contact
var contact = new mozContact();
contact.givenName = ["John"];
contact.familyName = ["Doe"];
contact.nickName = ["No kidding"];
save new contact into the database
var request = contactAPI.save(contact);
saving.onsuccess = function() {
console.log('New contact created');
};
saving.onerror = function(err) {
console.error(err);
};
telephony
Get the Telephony object
var tel = navigator.Telephony;
Place a call!
var call = tel.dial(“123456789”);
Listen to events
call.onstatechange = function (event) {
/* Different values for event.state
* "dialing", "ringing", "busy", "connecting", "connected",
* "disconnecting", "disconnected", "incoming"
*/
};
call.onconnected = function () {};
call.ondisconnected = function () {};
MEssages
Get the Message object
var messageManager = navigator.mozMobileMessage;
Send SMS or MMS
var sms = messageManager.sendSMS(number,messageContent);
var mms = messageManager.sendMMS(mmsParameters);
...and wait for events
sms.onsucces = mms.onsuccess = function(evt) {
console.log('Thanks for paying');
};
sms.onerror = mms.onerror = function(evt) {
console.log('Problems ahead');
};
too tight?
How can I do privileged actions without access
to the API I need?
interaction
web activities

what?
Web Activities define a way for apps to
delegate actions on other apps.
WAIT, I'VE SEEN THIS BEFORE.
YES
Intents on Android
WebIntents on Chrome
WebActivities for Mozilla
WHO can USE IT?
WebActivities API is considered a SAFE one
So any app can use it
I NEED...
To receive data from another app, we should
start a MozActivity, then wait for the response
var activity = new MozActivity({
// Ask for the "pick" activity
name: "pick",
// Provide the data required by the filters of the activity
data: {
type: "image/jpeg"
}
});
activity.onsuccess = function() {
var picture = this.result;
console.log("A picture has been retrieved");
};
activity.onerror = function() {
console.log(this.error);
};
I OFFER...
Any app can handle activities.
Just register on the manifest.
{
// [...] Other Manifest related stuff
// Activity registration
"activities": {
"pick": { // name of the activity
"href": "./pick.html", // page opened when handling
"disposition": "inline", // how is the page presented
"filters": {
"type": ["image/*","image/jpeg","image/png"] // data
},
"returnValue": true // expect a value on the response
}
}
}
handling
Once our app is registered as a handler,
we need to decide how are we gonna do it.
navigator.mozSetMessageHandler('activity', function(activityRequest) {
var option = activityRequest.source;
if (option.name === "pick") {
// Do something to handle the activity
}
});
responding
Because giving back is always nice
navigator.mozSetMessageHandler('activity', function(activityRequest) {
[...]
// Send back the result
if (picture) {
activityRequest.postResult(picture);
} else {
activityRequest.postError("Unable to provide a picture");
}
}
});
examples
Make a call...
"dial": {
type: "webtelephony/number", // ...with the phone
number: {
regexp:/^[\\d\\s+#*().-]{0,50}$/
}
}
Create new...
"new": {
type: "webcontacts/contact" // ...contact
type: "mail" // ...email
type: "websms/sms", // ...sms
number: {
regexp:/^[\\d\\s+#*().-]{0,50}$/
}
}
examples
Open an app...
"open": {
type: "webcontacts/contact" // ...contacts
type: [ // ...gallery
"image/jpeg",
"image/png",
"image/gif",
"image/bmp"
]
type: [ // ...music
"audio/mpeg",
"audio/ogg",
"audio/mp4"
]
}
Record media...
"record": {
type: ["photos", "videos"] // ...with the camera
}
COLLISIONS
Camera app
"pick": {
"filters": {
"type": ["image/*", "image/jpeg", "image/png"]
}
}
Gallery app
"pick": {
"filters": {
"type": ["image/*", "image/jpeg", "image/png"]
}
}
Wallpaper app
"pick": {
"filters": {
"type": ["image/*", "image/jpeg", "image/png"]
}
}
USER CHOOSE
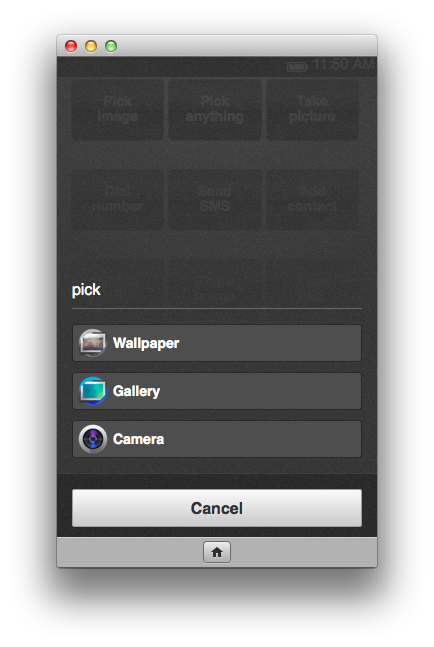
THANK YOU!
where CREDICT'S DUE
API GROWTH GRAPH
http://blogs.kuppingercole.com/burton/2011/10/28/more-on-the-open-api-revolution/
OPEN API NUMBERS
http://www.programmableweb.com/
BROWSER APIs
http://platform.html5.org/
FIREFOX OS APIs
https://developer.mozilla.org/en-US/docs/WebAPI
WEB ACTIVITIES
https://developer.mozilla.org/en-US/docs/WebAPI/Web_Activities
Firefox OS: APIs & Activities
By Fernando Campo
Firefox OS: APIs & Activities
Get the basics about FirefoxOS, access the new APIs and learn to interact between apps through web activities.
- 7,800