Let's Welcome
Vue 3!
Let's Welcome
Vue 3!
- Typescript
- Proxies
- Mostly Backwards Compatible
- Composition Function API
Vue 2 Is currently written in Flow,
a static type checking system from Facebook.
Vue 3 is completely rewritten from the ground up in Typescript.
(This will make the type hints in typescript better for YOU!)
The Vue 2 source is currently is very intertwined.
The Vue 3 source will be more modular.
This is will allow for:
- Different rendering engines (Server Side, Browser, NativeScript, etc)
- Tree Shaking (only use the code you need)
Vue 2 has a reactivity based on Object.defineProperty
Vue 3 will have a reactivity system Based around Proxies.
ES6 Proxies
The Proxy object is used to define custom behavior for fundamental operations (e.g. property lookup, assignment, enumeration, function invocation, etc).
In other words: Fancy new JavaScript stuff
What's a Proxy?
BUT WHY PROXIES??
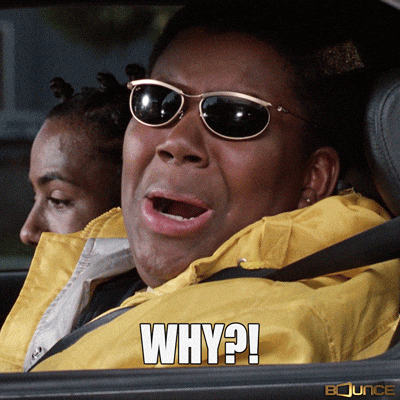
Have you ever...?
// Setting an array item by index
Vue.set(this.myArray, index, newValue)
// Adding a new property to an object
Vue.set(this.myObject, key, value)
// Deleting a property from an object
Vue.delete(this.myObject, key)
Vue 2
Well Now....
// Setting an array item by index
Vue.set(this.myArray, index, newValue)
// Adding a new property to an object
Vue.set(this.myObject, key, value)
// Deleting a property from an object
Vue.delete(this.myObject, key)
Vue 2
// Setting an array item by index
this.myArray[index] = newValue
// Adding a new property to an object
this.myObject[key] = value
// Deleting a property from an object
delete this.myObject[key]
Vue 3
Here's the thing though...
Proxies cannot be polyfilled
in Internet Explorer.
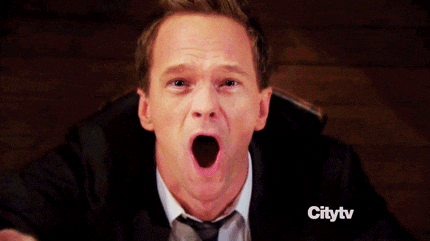
Good news!
There will be an option to do a backwards compatible build!
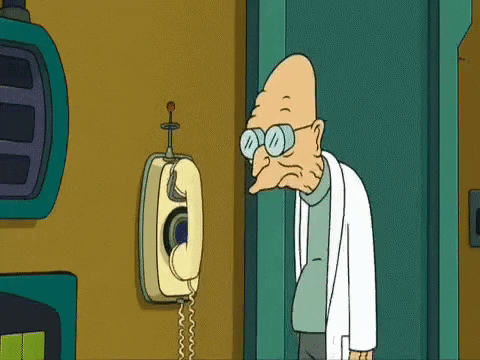
Okay, So let's talk code.
Vue 2 Syntax
<template>
<div id="app">
<h1>{{ msg }}</h1>
</div>
</template>
<script>
export default {
data () {
return {
msg: 'Hello World';
},
},
};
</script>
<style>
body {
font-family: Helvetica, sans-serif;
}
</style>
<template>
<div id="app">
<h1>{{ msg }}</h1>
</div>
</template>
<script>
export default {
data () {
return {
msg: 'Hello World';
},
},
};
</script>
<style>
body {
font-family: Helvetica, sans-serif;
}
</style>
Vue 2 Syntax
<template>
<div id="app">
<h1>{{ msg }}</h1>
</div>
</template>
<script>
export default {
data () {
return {
msg: 'Hello World';
},
},
};
</script>
<style>
body {
font-family: Helvetica, sans-serif;
}
</style>
<template>
<div id="app">
<h1>{{ msg }}</h1>
</div>
</template>
<script>
export default {
data () {
return {
msg: 'Hello World';
},
},
};
</script>
<style>
body {
font-family: Helvetica, sans-serif;
}
</style>
Vue 3 Syntax

Most of your components will just work.
Things that will change
- Filters - removed
- $listeners will be merged into $attrs
Let's talk about the Composition Function API
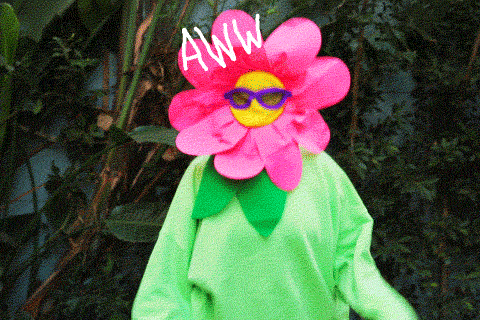
A Bit of History
(Or... how i saw it)
-
October 25th, 2018: Dan Abramov demos React Hooks at React Conf
- October 27th, 2018 12:38 am EST: Evan You Tweets:
- Ok I can’t really help myself… use hooks in Vue (via userland lib in under 100 LOC): https://codesandbox.io/s/jpqo566289
WARNING
Vue 3 is in ALPHA as of March 24th, 2020. The API might change before release.
So what does it look like?
Classic Component
<template>
<button @click="increment">
Count is: {{ count }}, double is: {{ double }}
</button>
</template>
<script>
export default {
data(){
return { count: 0};
},
computed:{
double(){
return this.count * 2;
},
},
methods:{
increment() {
this.count++;
},
},
};
</script>
Component with reactive
<template>
<button @click="increment">
Count is: {{ state.count }}, double is: {{ state.double }}
</button>
</template>
<script>
import { reactive, computed } from 'vue';
export default {
setup() {
const state = reactive({
count: 0,
double: computed(() => state.count * 2)
});
function increment() {
state.count++
}
return {
state,
increment
};
},
};
</script>
Component with ref
<template>
<button @click="increment">
Count is: {{ count }}, double is: {{ double }}
</button>
</template>
<script>
import { ref, computed } from 'vue';
export default {
setup() {
const count = ref(0);
const double = computed(() => count.value * 2);
function increment() {
count.value++
}
return {
count,
double,
increment,
};
},
};
</script>
Demo!
Why would I want this?


Other Nifty Things!
- Fragments!
- Reactive Dependency Injection
- Portals
- Suspended Component Rendering
Questions?
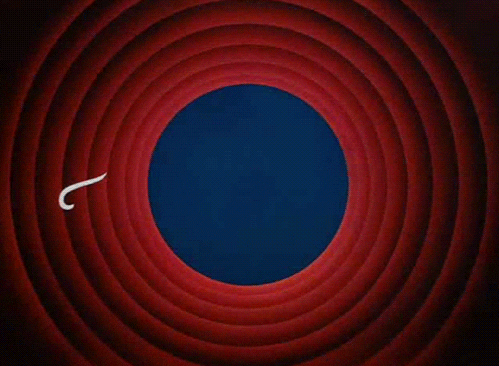
Slides: https://slides.com/fimion/lets-welcome-vue-3
More information:
Let's Welcome Vue 3
By Alex Riviere
Let's Welcome Vue 3
- 599