@fmuyassarov
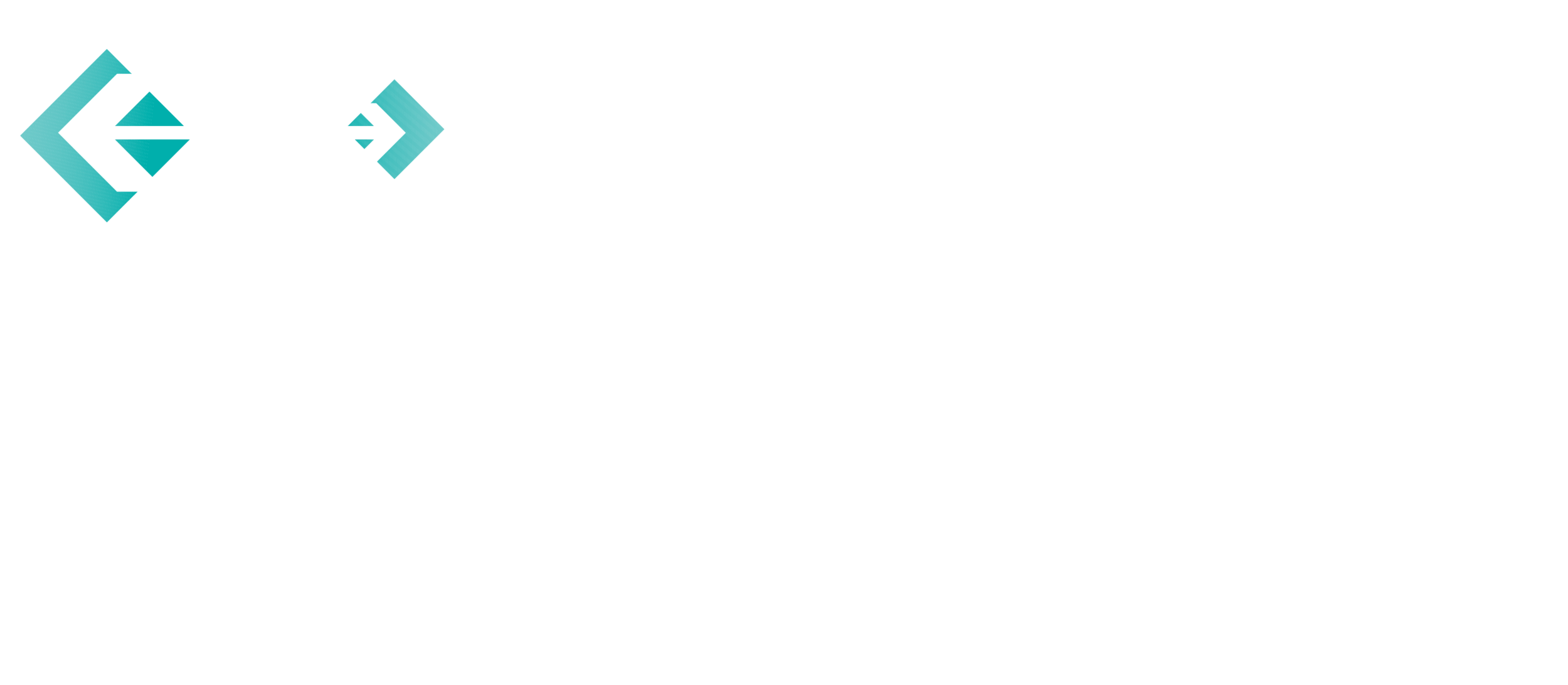
A communication system for handling request/response interactions and temporary streaming.
A client application can call a method on a server application running on another machine just like it would a local function.
Server implements the interface and runs a gRPC server to handle client requests.
Client (a.k.a.) stub, provides the same methods as the server.
Relies on Protocol Buffer for message serialization (can be replaced by JSON)
Relies on HTTP/2 as transport layer
Pluggable auth, tracing, load balancing and health checking
11 supported languages (C++, Go, Python, Java etc)
Open sourced by google in 2015 as the replacement for Stubby (micro services communication framework)
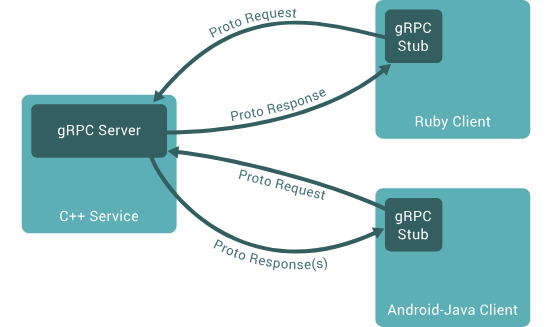
Facts about gRPC...
Basic components
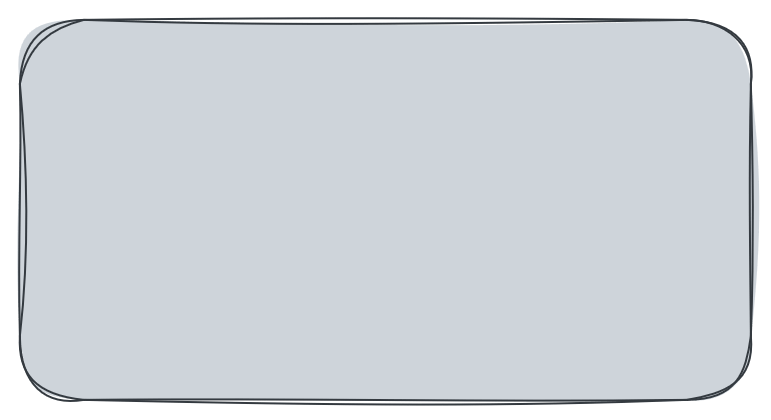
000110101011011101
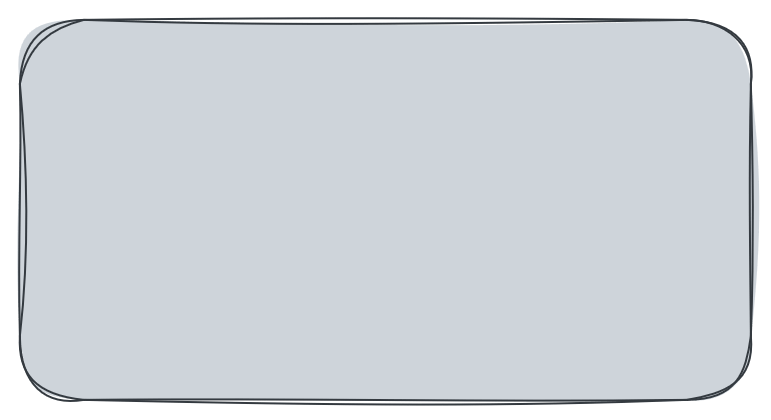
gRPC
client
HTTP/2
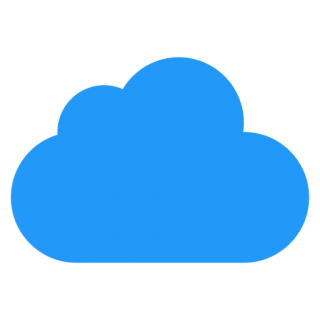
network
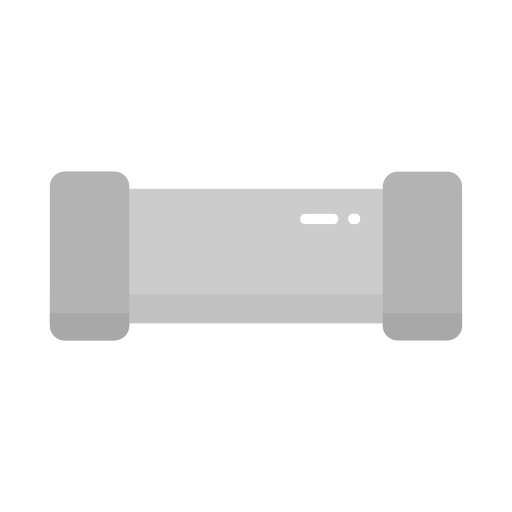
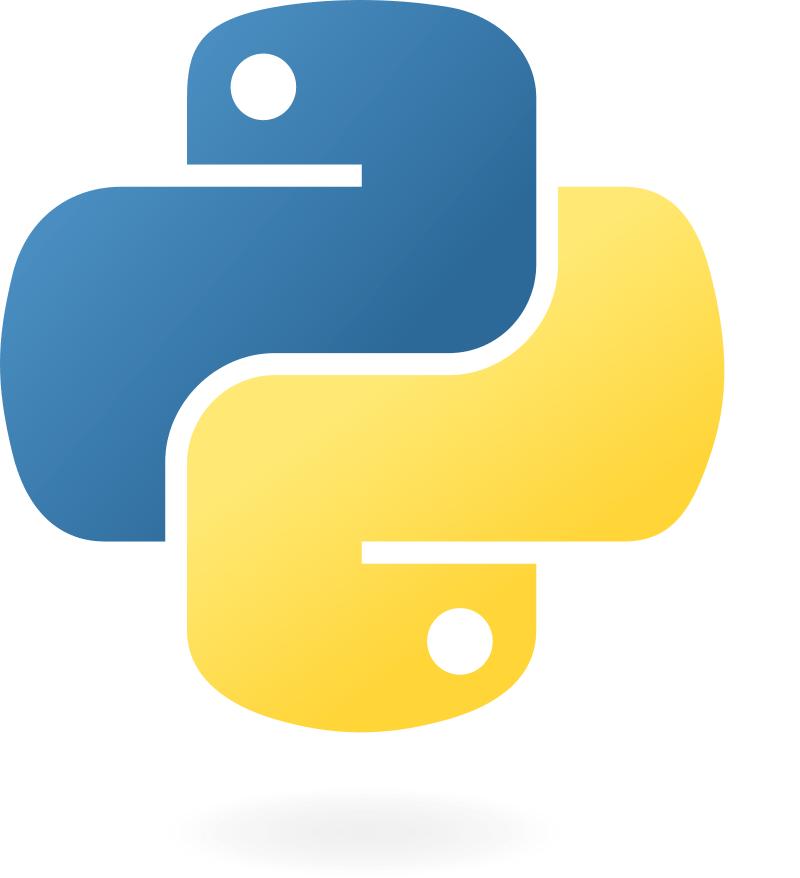
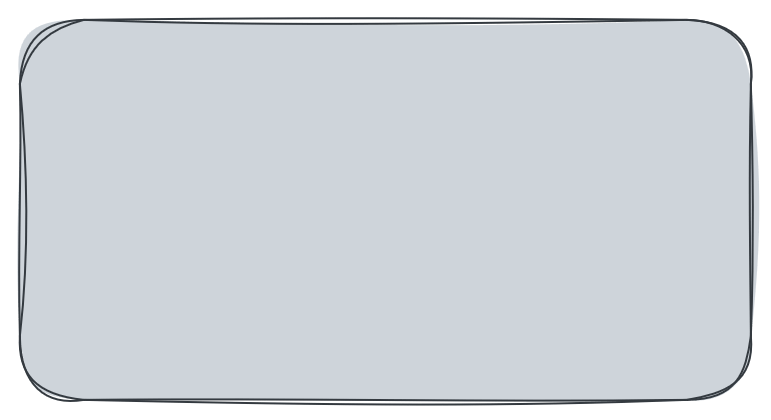
gRPC
server
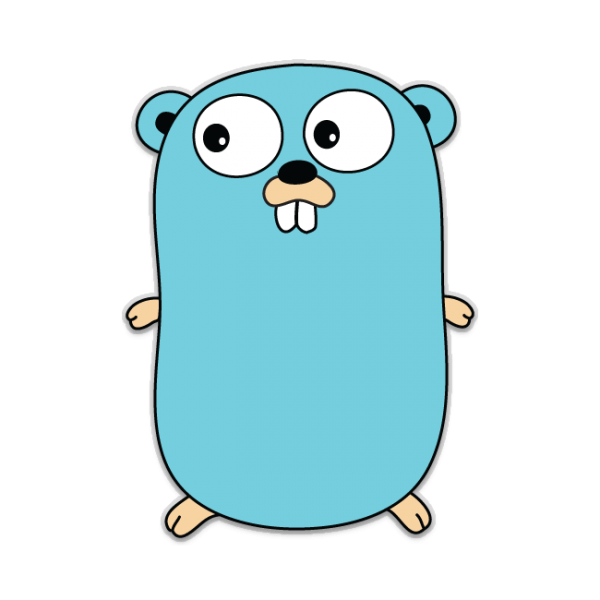
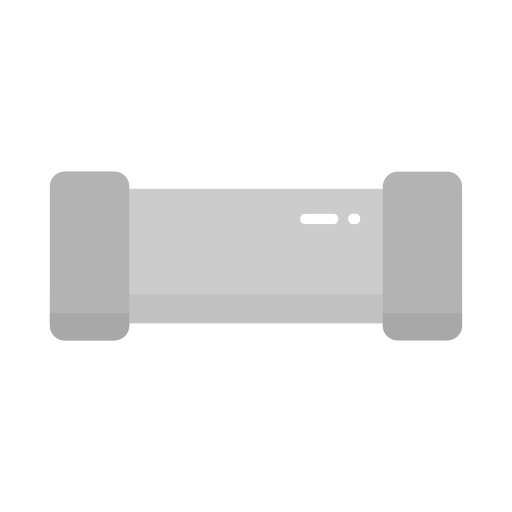
- protocol buffer
- HTTP/2
-
channel
- a virtual connection
- stub
- service methods
var opts []grpc.DialOption
opts = append(opts, grpc.WithTransportCredentials(insecure.NewCredentials()))
conn, err := grpc.NewClient(*serverAddr, opts...)
Basic components of gRPC
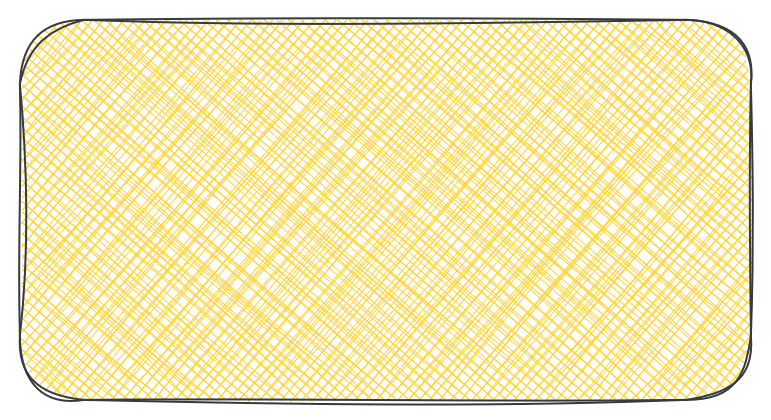
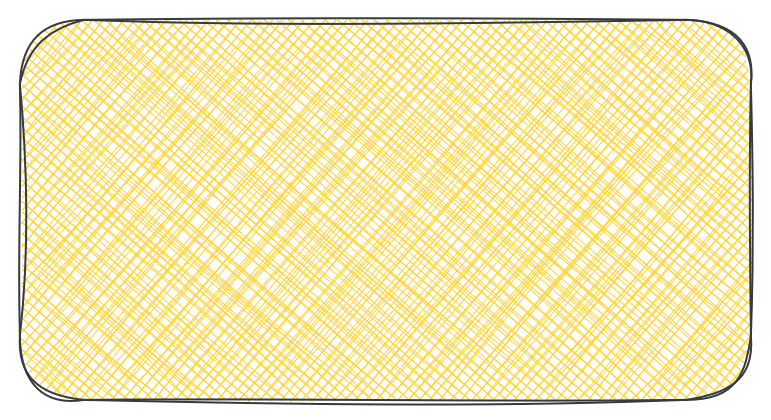
gRPC
client
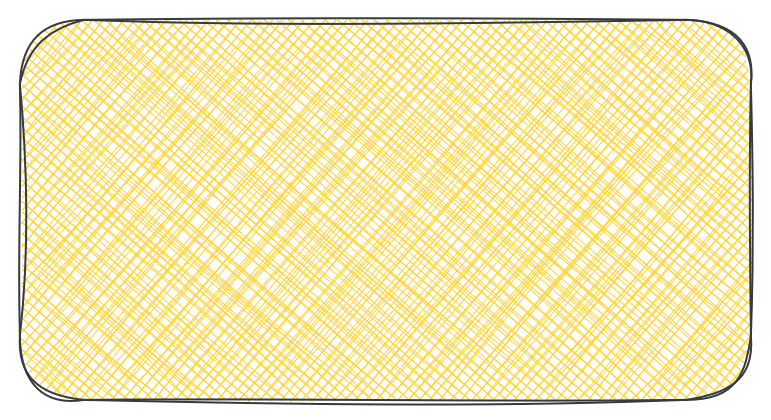
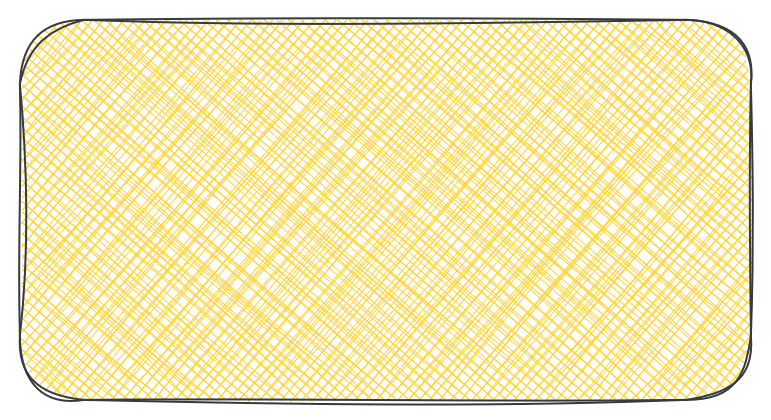
gRPC
server


request
Unary RPC
response
rpc SayHello(HelloRequest) returns (HelloResponse);
- protocol buffer
- HTTP/2
- channel
- stub
- service methods
Basic components of gRPC
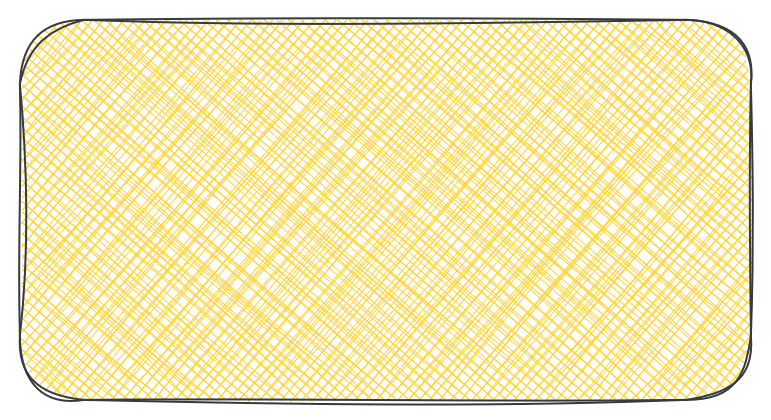
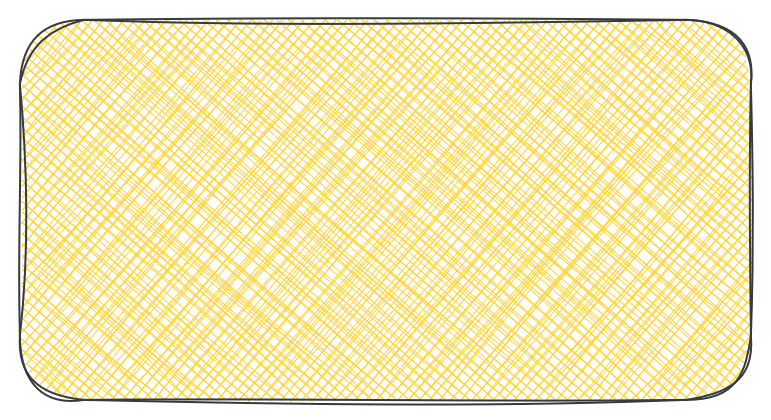
gRPC
client
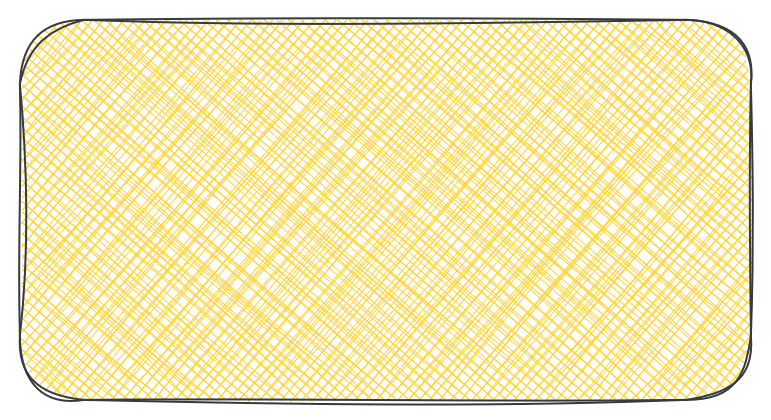
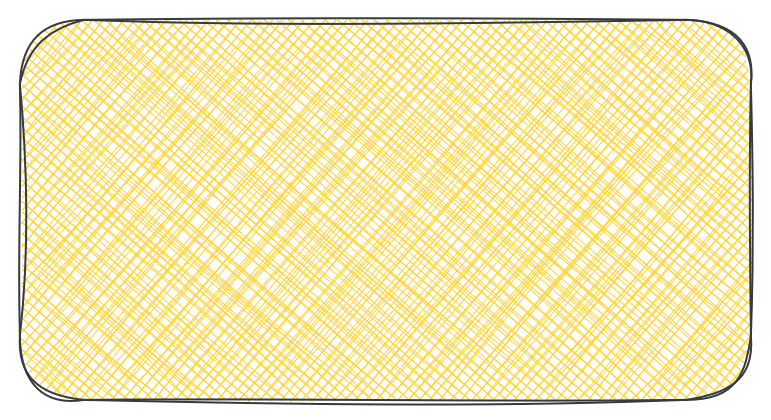
gRPC
server

request
response

Server streaming RPC
rpc LotsOfReplies(HelloRequest) returns (stream HelloResponse);
- protocol buffer
- HTTP/2
- channel
- stub
- service methods
Basic components of gRPC
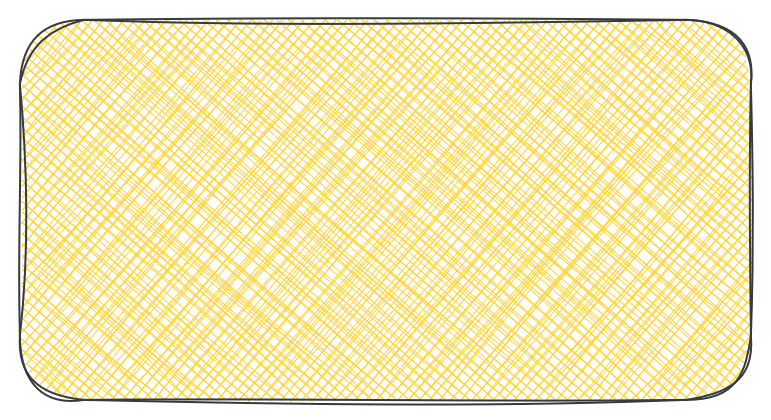
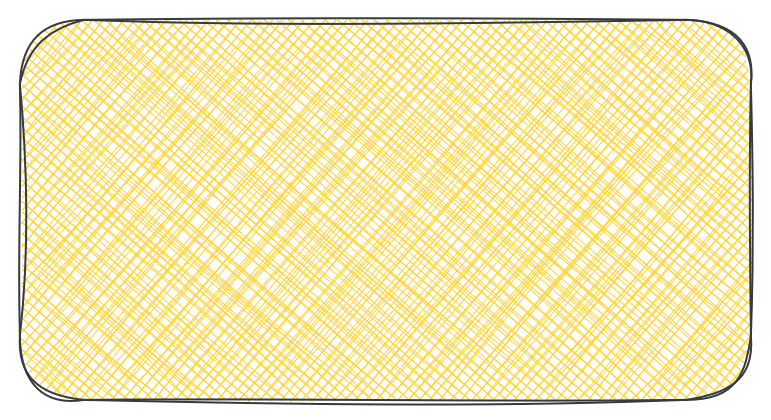
gRPC
client
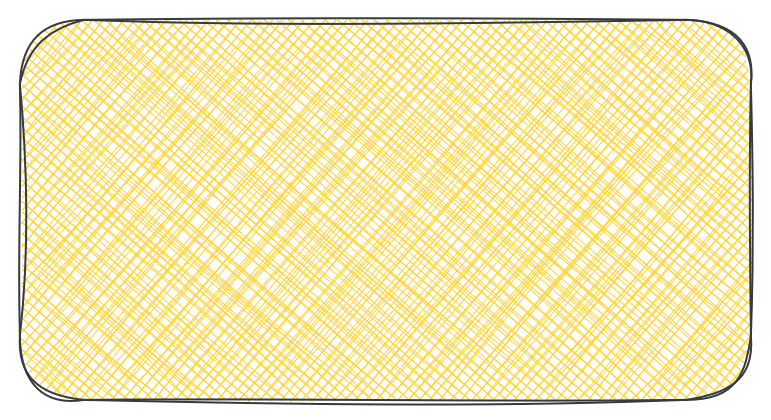
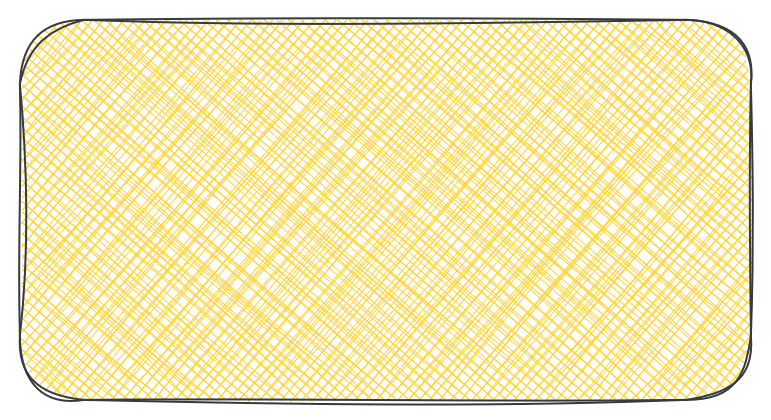
gRPC
server

request
response

Client streaming RPC
rpc LotsOfGreetings(stream HelloRequest) returns (HelloResponse);
- protocol buffer
- HTTP/2
- channel
- stub
- service methods
Basic components of gRPC
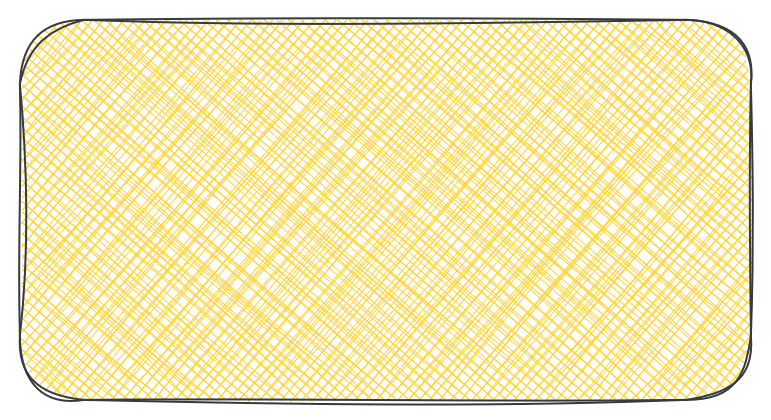
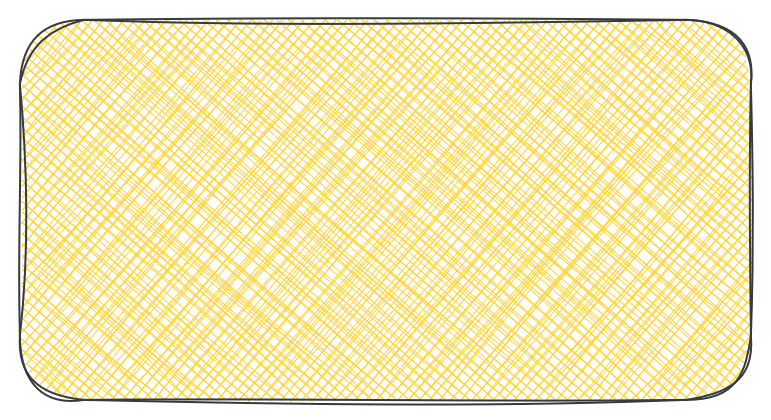
gRPC
client
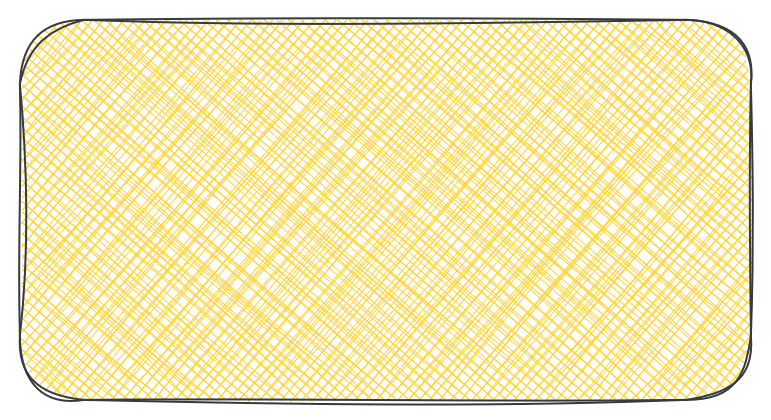
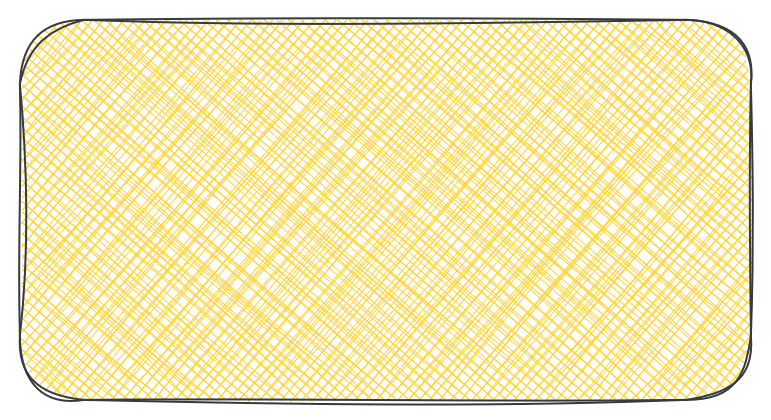
gRPC
server

request
response

Bidirectional streaming RPC
rpc BidiHello(stream HelloRequest) returns (stream HelloResponse);
- protocol buffer
- HTTP/2
- channel
- stub
- service methods
Basic components of gRPC
- protocol buffer
- HTTP/2
- channel
- stub
- service methods
- synchronous vs asynchronous
- protocol buffer
- HTTP/2
- channel
- stub
- service methods
- synchronous vs asynchronous
- Timeouts/Deadline
- Timeouts and Deadlines control how long a gRPC call should wait before failing.
- A Deadline is the specific time by which a request must complete (e.g., 5 seconds from now).
- A Timeout is the maximum duration a call can take (e.g., 5 seconds).
- If a request exceeds the deadline or timeout, gRPC cancels the call and returns a DEADLINE_EXCEEDED error.
- Clients set deadlines, and servers can check them to avoid unnecessary processing.
Basic components of gRPC
- protocol buffer
- HTTP/2
- channel
- stub
- service methods
- synchronous vs asynchronous
-
Timeouts/Deadline
- Timeouts and Deadlines control how long a gRPC call should wait before failing.
- A Deadline is the specific time by which a request must complete (e.g., 5 seconds from now).
- A Timeout is the maximum duration a call can take (e.g., 5 seconds).
- If a request exceeds the deadline or timeout, gRPC cancels the call and returns a DEADLINE_EXCEEDED error.
- Clients set deadlines, and servers can check them to avoid unnecessary processing.
- RPC termination
- can be independently terminated either by client or server
Basic components of gRPC
Protocol Buffer (language)
Protobuf
- A mechanism for serializing structured data (think of it similar but better version of JSON)
- Binary format - it’s more compact than text-based JSON with a lot of overhead (brackets, keys, etc.).
- Faster serialization/de-serialization – since Protobuf is binary, encoding and decoding are much faster compared to JSON, which requires parsing text.
-
Strongly typed – enforces data types (int32, float, string)
-
Backward compatibility – allows to add/remove fields without breaking older clients
-
Unlike JSON (gzip), it doesn't require extra tooling for reducing the payload size
-
Since it is in binary format, it is NOT human readable nor a self describing/human readable language
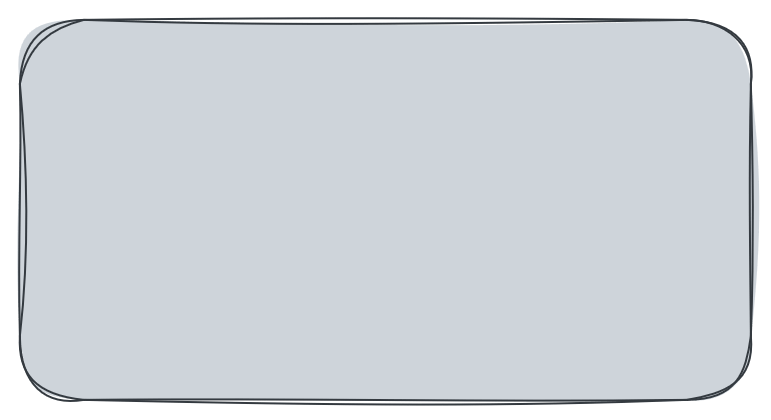
000110101011011101
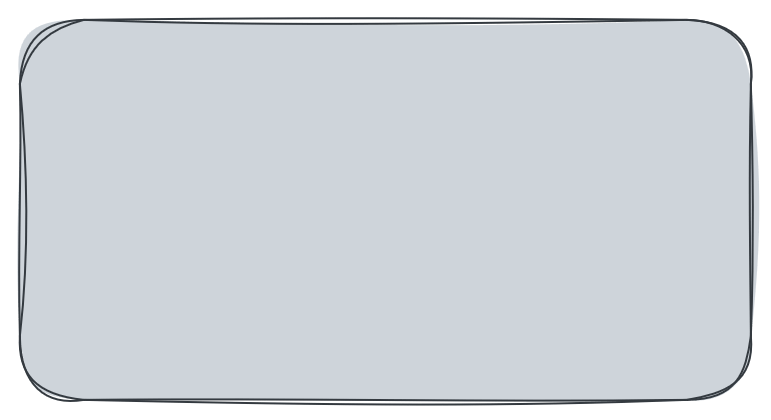
gRPC
client
HTTP/2
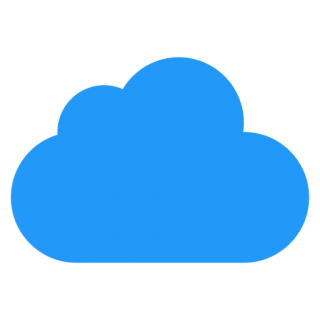
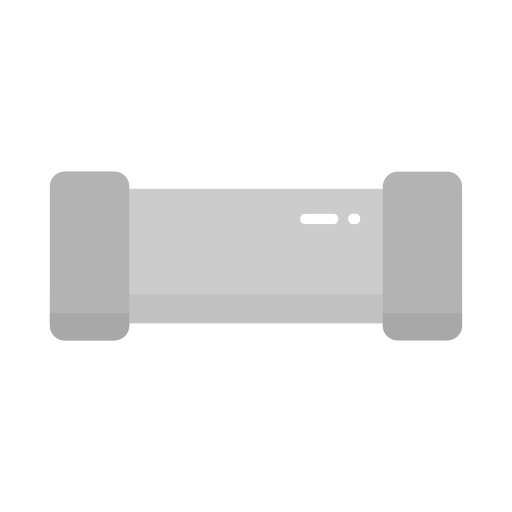
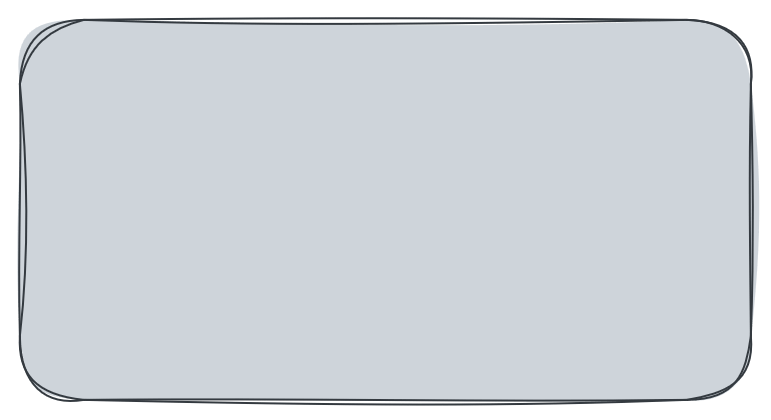
gRPC
server
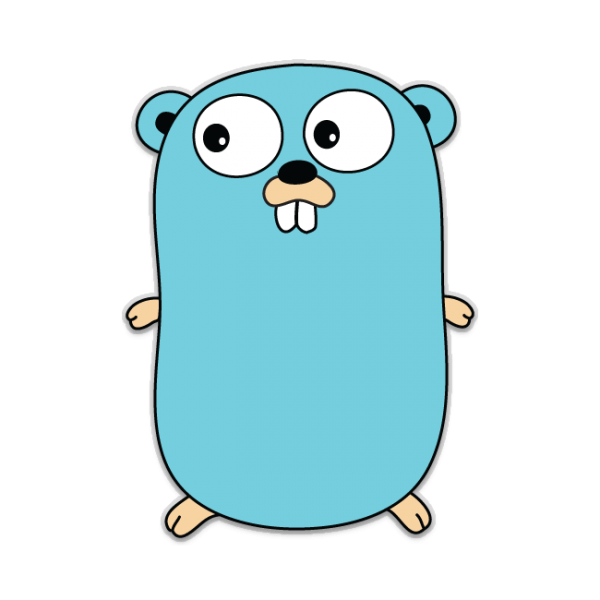
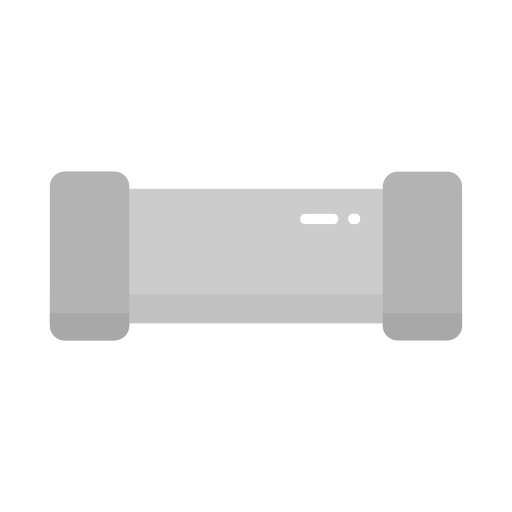
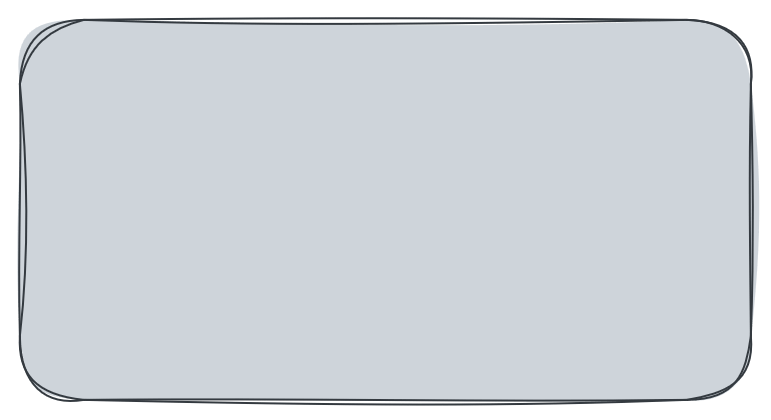
Scheme
.proto
network
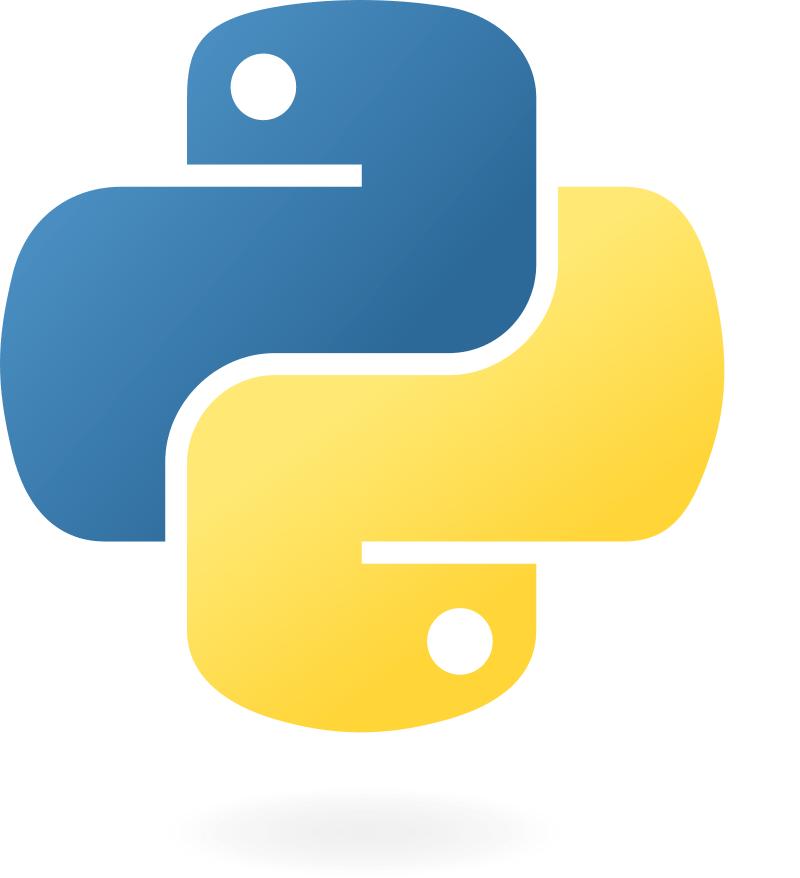
Let's build ...
-
protoc - a compiler that reads
.proto
files and generates code in different languages with the help of plugins. -
protoc-gen-go - a Go plugin for
protoc
that generates Go structures and serialization methods for Protocol Buffers (but without gRPC support).
Let's build ...
-
protoc - a compiler that reads
.proto
files and generates code in different languages with the help of plugins. -
protoc-gen-go - a Go plugin for
protoc
that generates Go structures and serialization methods for Protocol Buffers (but without gRPC support).
message User {
string name = 1;
int32 age = 2;
}
type User struct {
Name string
Age int32
}
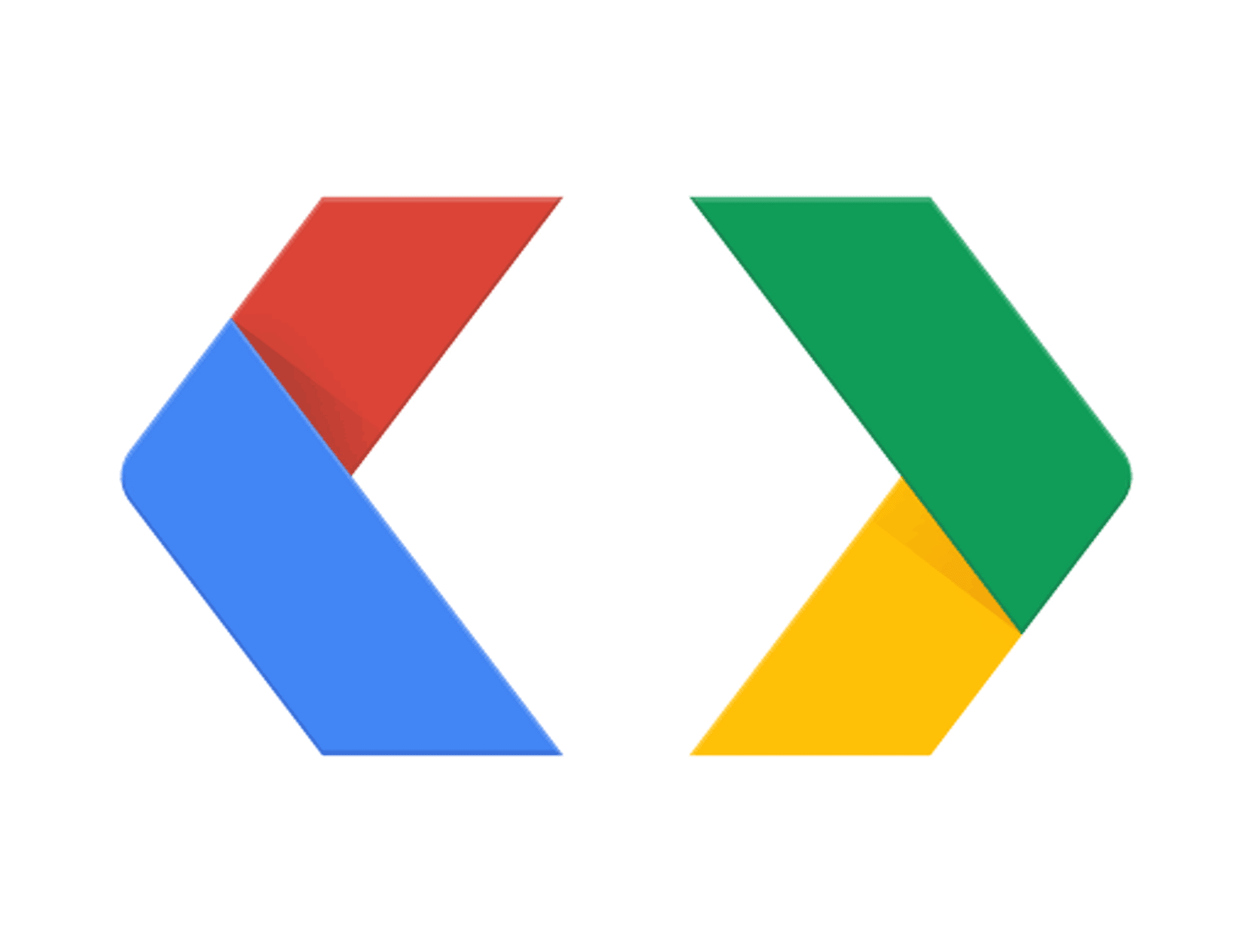
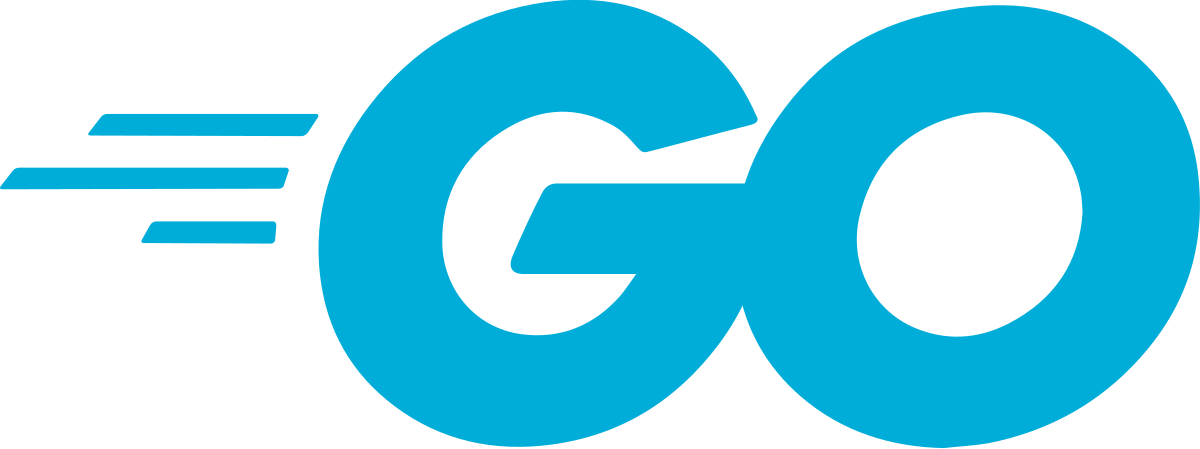
Let's build ...
- protoc - a compiler that reads
.proto
files and generates code in different languages with the help of plugins. - protoc-gen-go - a Go plugin for
protoc
that generates Go structures and serialization methods for Protocol Buffers (but without gRPC support). -
protoc-gen-go-grpc - a gRPC plugin for
protoc
that generates Go code specifically for gRPC server and client stubs from.proto
files.
message User {
string name = 1;
int32 age = 2;
}
type User struct {
Name string
Age int32
}
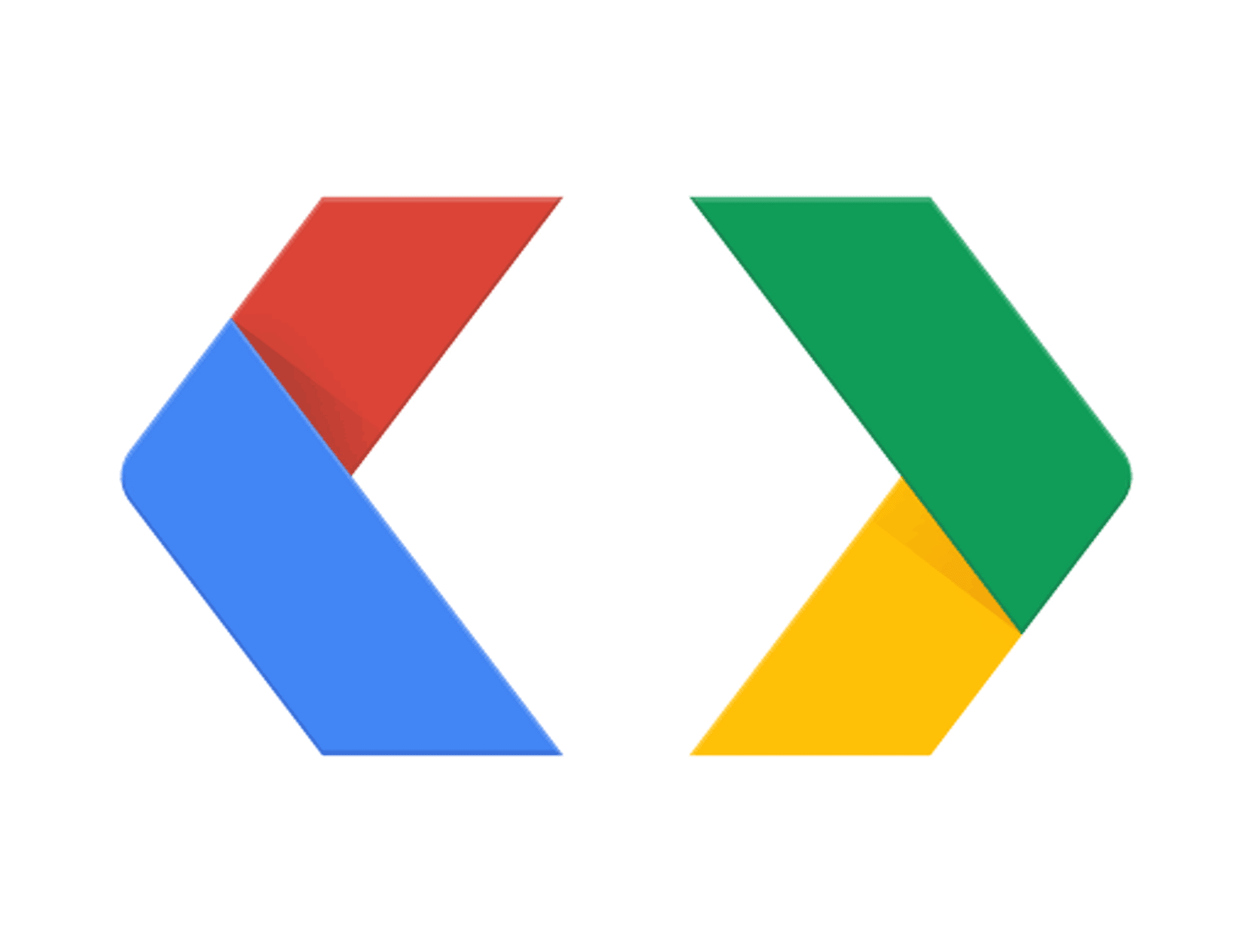
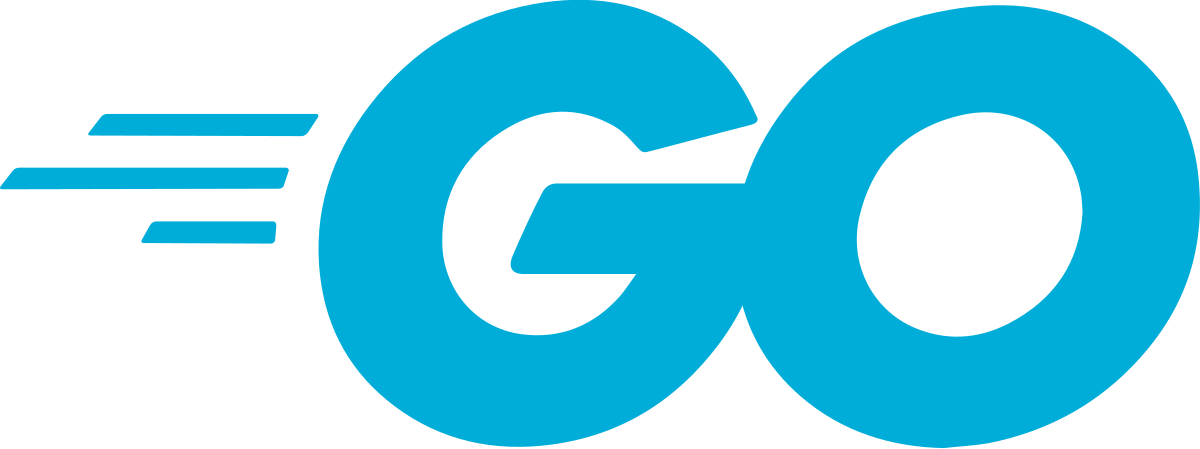
Let's build ...
- protoc - a compiler that reads
.proto
files and generates code in different languages with the help of plugins. - protoc-gen-go - a Go plugin for
protoc
that generates Go structures and serialization methods for Protocol Buffers (but without gRPC support). -
protoc-gen-go-grpc - a gRPC plugin for
protoc
that generates Go code specifically for gRPC server and client stubs from.proto
files.
service UserService {
rpc GetUser (UserRequest) returns (UserResponse);
}
type UserServiceServer interface {
GetUser(context.Context, *UserRequest) (*UserResponse, error)
}
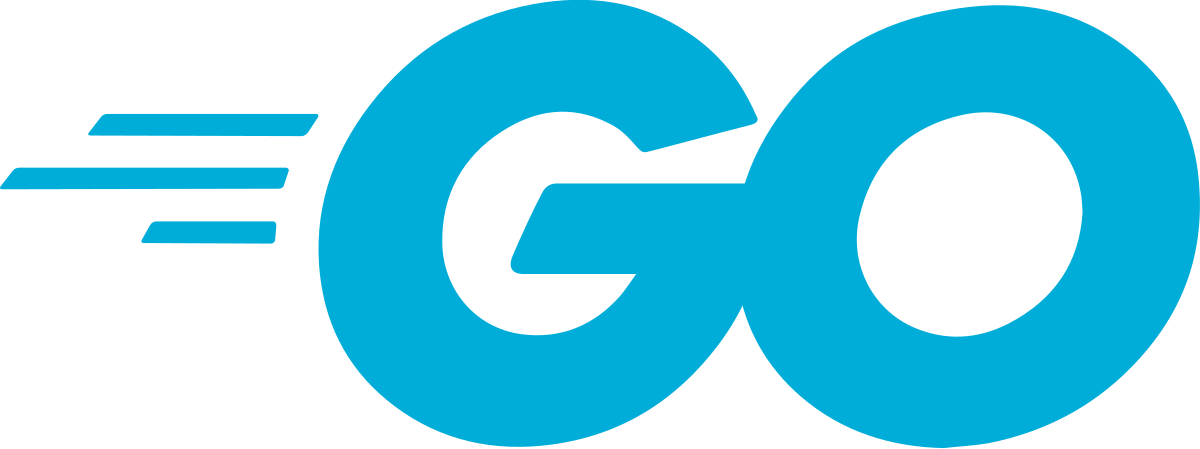
At this point, we have a Go interface, but we still need to implement it.
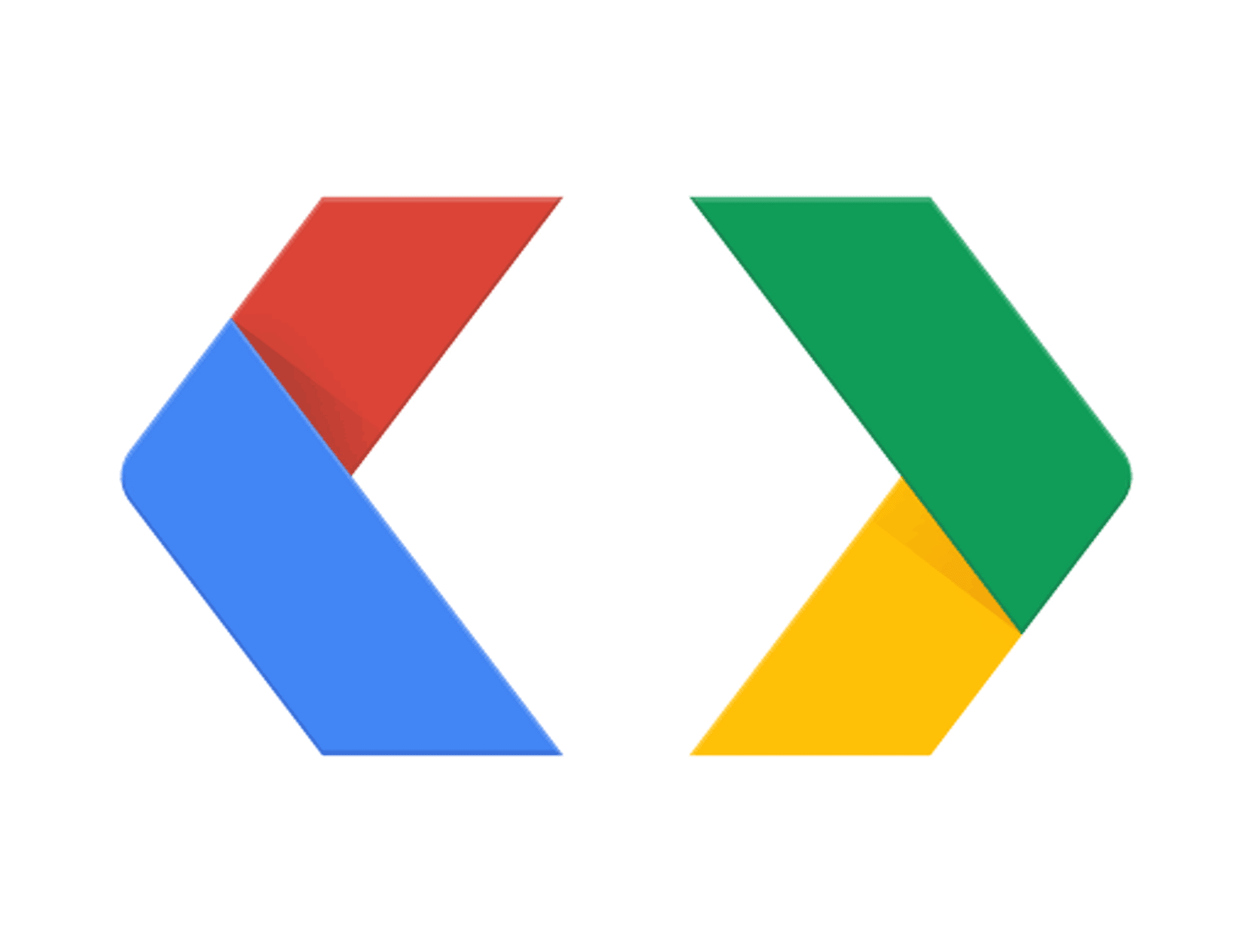
protoc hello/hello.proto \
--go_out=. \
--go-grpc_out=. \
--go_opt=paths=source_relative \
--go-grpc_opt=paths=source_relative
protoc hello/hello.proto \
--go_out=. \
--go-grpc_out=. \
--go_opt=paths=source_relative \
--go-grpc_opt=paths=source_relative
ls -l hello
total 40
-rw-r--r--@ 1 est staff 6127 Feb 24 15:57 hello.pb.go
-rw-r--r--@ 1 est staff 277 Feb 24 15:43 hello.proto
-rw-r--r--@ 1 est staff 4688 Feb 24 15:57 hello_grpc.pb.go
-
hello.pb.go - interface types for servers to implement
-
hello_grpc.pb.go - protocol buffer code (for populating, serializing and retrieve messages.
demo time ...
gRPC
By fmuyassarov
gRPC
- 16