Git and GitHub
Geoffrey Liu
Follow along!
While we're talking...
- Sign up for a free GitHub account: https://github.com/join
- Download and install GitHub Desktop client: https://desktop.github.com/
- Optional: log in on github.com and GitHub Desktop
Hi, I'm Geoffrey
- CSE major, music minor, c/o 2016
- Second time as a 154 TA
- Past: CDK Global, ASUW
- Future: Groupon
- Front-end web dev enthusiast
Life without version control
Have you done any of the following for a project?
- Emailing copies of files back and forth
- Working on code through Google Docs
- Sending code through IM
Life without version control
What if you need to...
- Go back to an older version of a file?
- View the history of the project? A file?
- Manage similar versions of the project?
- Easily share code with another developer?

Git, conceptually
At the highest level, a Git repository is a series of snapshots of files in a folder.
- Snapshots formally called commits (more later)
- You tell Git when to take a snapshot
- Like a time machine: can view any snapshot whenever you want!

time
Photo credit: gitref.org
Git, conceptually
There are three sections in a Git repository.
- Repository: all committed files, along with their contents at the time of commit
-
Staging area: all files declared to have been modified (staged), but not yet committed
- Also known as the index
- Working directory: all files whose contents have been modified, but not yet staged

Photo credit: Git-scm.com
Git, conceptually
- Git is what we call a distributed version control system (DVCS)
- There exists one "master" repo, called the origin
- Usually stored on a server
- In our case, stored on GitHub
- Each clone of the origin is called a working copy

Photo credit: CSE 331, UW CSE
One-time setup
Local setup, continued
- Log into the GitHub Desktop app with your account
- Open up "Git Shell"
- In Git Shell, type:
git --version
- You should see "
git version 2.X.X
" - If not, raise your hand or ask your neighbor
- You should see "
Git on PowerShell / cmd
(for Windows users only)
- Type %localappdata% in Explorer
- Go to GitHub/PortableGit_*/cmd
- Copy the filepath, which should look like this:
C:\Users\name\AppData\Local\GitHub\PortableGit_<uniqueId>\cmd
- Add that filepath to your PATH variable
Create the origin repo
- Go to https://github.com/new
- Give your repo a name and description
- (Leave all other settings as default)
- Click "Create Repository"
- Copy the Git URL (need this for next step)
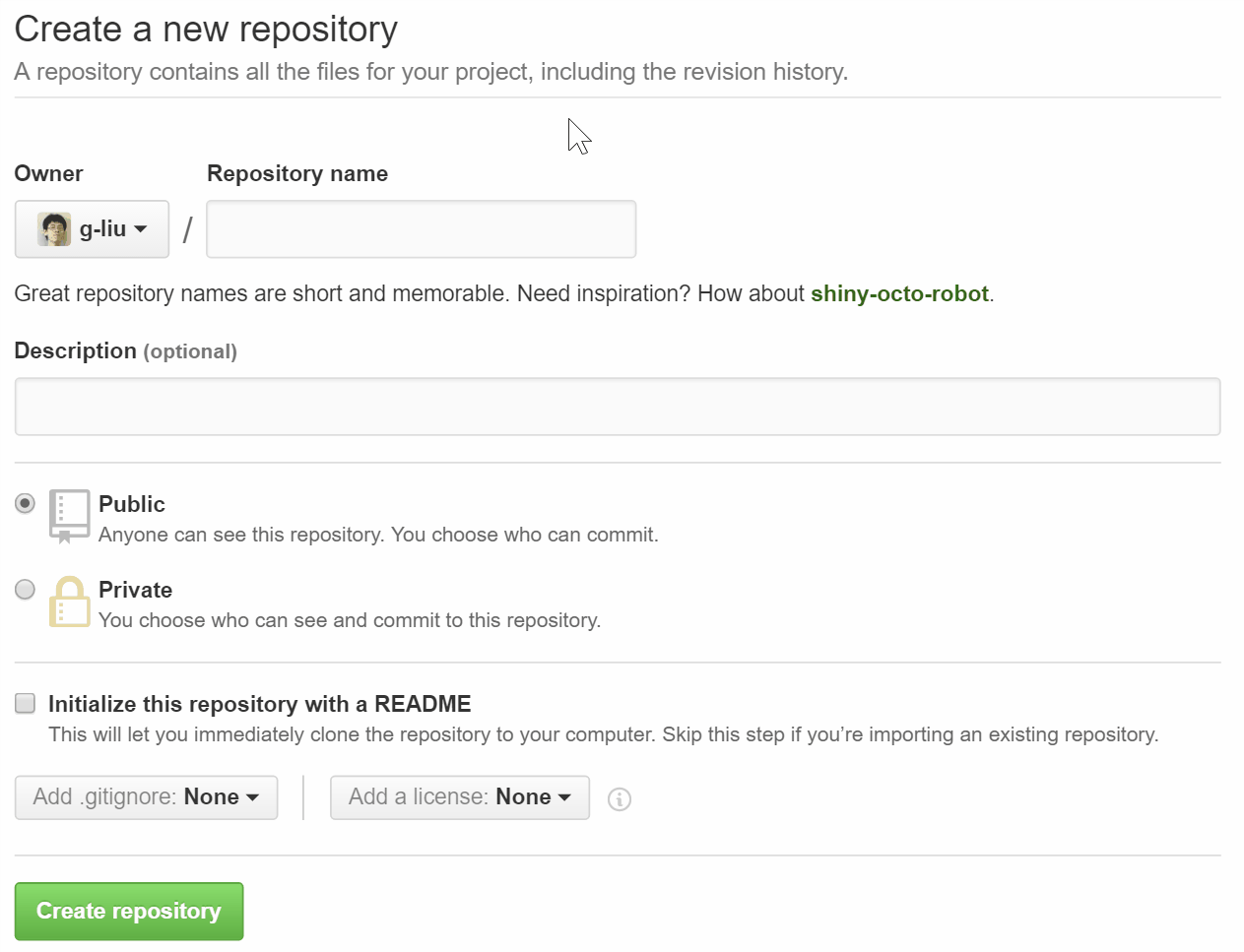
Clone your new repository
git clone git_url
- Open "Git Shell"
- Type the above command into the shell, pasting in the Git URL of your repository
- Note: May need to use Shift + Insert to paste

Navigate to your local copy
-
git clone
creates a new folder with the same name as your repository - Type
ls
to see all files/folders in the current directory - Type
cd repoName
, replacing repoName with the name of your repository, to navigate to your repo

Git basic workflow
Make some changes
Pretend you're working on a project. Maybe you need to:
- Create a new file/files?
touch filename
- Delete a file/files?
rm filename
- Edit a file?
vi filename
(or use your favorite editor). - What you do here is up to you!

See what's changed
git diff
- Shows comparison of working tree vs. staging area
git status
- Shows you a list of files modified

Add changed files

Describe the changes made
git commit -m "your commit message"
- Creates a snapshot of all changes made to staged files
- Use
git status
to see the files to be committed - Use
git log
to see all commits made so far

Commit messages
-
#1 rule: A commit message should be descriptive!
- Concisely describe changes made
- Pays off in the future
- Helps other developers understand your changes
- Git requires every commit to have a message
Good messages
Bad messages
asdf
fix bug
yeah
"Upload" the changes
git push
- Updates origin repo with the latest commit(s) made
- Makes changes available to other collaborators.
- Congratulations! You just made your first commit!

Workflow summary
- For any changes to a repository:
- Make changes to necessary file(s)
-
Add the files with
git add files...
-
Commit the files with
git commit -m "message"
-
Push the files to the origin with
git push
- The quintessential Git workflow; know this if nothing else!
Workflow summary

Undoing things
Reverting file changes
git stash
and git stash apply
- Saves all changes made so far and resets working copy to last commit
- Use
git stash apply
to bring back those changes
git checkout -- path1 path2 ...
- Reverts all changes to the files at the specified path(s)
- WARNING! Cannot be undone!
git reset --hard
- Reverts entire repository to the last commit
- WARNING! Cannot be undone! Use only as last resort!
Undo add
git reset HEAD path1 path2 ...
- Removes the specified files from the staging area
- File contents are not changed
git rm --cached path1 path2 ...
- Removes files from repository
- Keeps the untracked copy in your working directory
Trick: git status
tells you which one to use
Undo commit
git commit --amend -m "new message"
- Changes commit message of the last commit
git reset HEAD~1
- Undoes last commit, and keeps changes to files
git reset --hard HEAD~1
- Undoes commit, and discards all changes
- WARNING! Cannot be undone!
Undo push?
- A little more complicated...
- http://stackoverflow.com/questions/1270514/undoing-a-git-push
Lesson: don't push unless absolutely sure!
Need to peek back?
git checkout SHA
- Each commit has a unique identifer called an SHA
- You can find the SHA of each commit with
git log
- Useful for looking at code from a previous commit
- Can edit and play around with the code
- Cannot make commits here
- To go back to current:
git checkout master
†
†If you were on a different branch, replace "master
" with the branch you were on
Need to revert back?
git revert SHA
- Creates an "anti-commit" to rollback to the commit with the given SHA
- Push it as a normal commit:
git push

Ignoring files
Some files should not be committed:
- Passwords
- API tokens
- Sensitive information / data
- Compiled code
- System / OS generated files
A file named .gitignore is used to manage this!
The .gitignore file
- File name starts with a dot (.)
- May be hidden in your file explorer
- Create the file in the shell:
touch .gitignore
- Edit with your favorite text editor

.gitignore syntax
# Comments start with hash, and are ignored
# A gitignore file is a list of file-matching patterns
# Ignores any file in any directory with this name.
passwords.txt
# Ignores any directory with this name
dist/
# Ignores any file with this extension
*.class
# We can combine file-matching patterns
vendor/*.min.js
Read more: https://git-scm.com/docs/gitignore
.gitignore resources
Collaboration
Let's work on code together!
- "Share" the repository by adding others as collaborators
- Find a partner
- Exchange GitHub usernames
- Follow these instructions to add collaborators

Contribute to each other's repositories
- Exchange repository URLs
- Go back to your local GitHub folder:
cd ..
- Clone their repository:
git clone their_git_url
- Navigate to the cloned repo:
cd their_repo_name
- Most of the workflow is already familiar:
- Modify necessary files
git add paths...
git commit -m "commit message"
git push
Keep your copy up to date
git pull
- Go back to your repo's directory:
cd ../yourRepoName
- Type the above command
- Fetches the latest changes from upstream
- Merges changes into your local copy
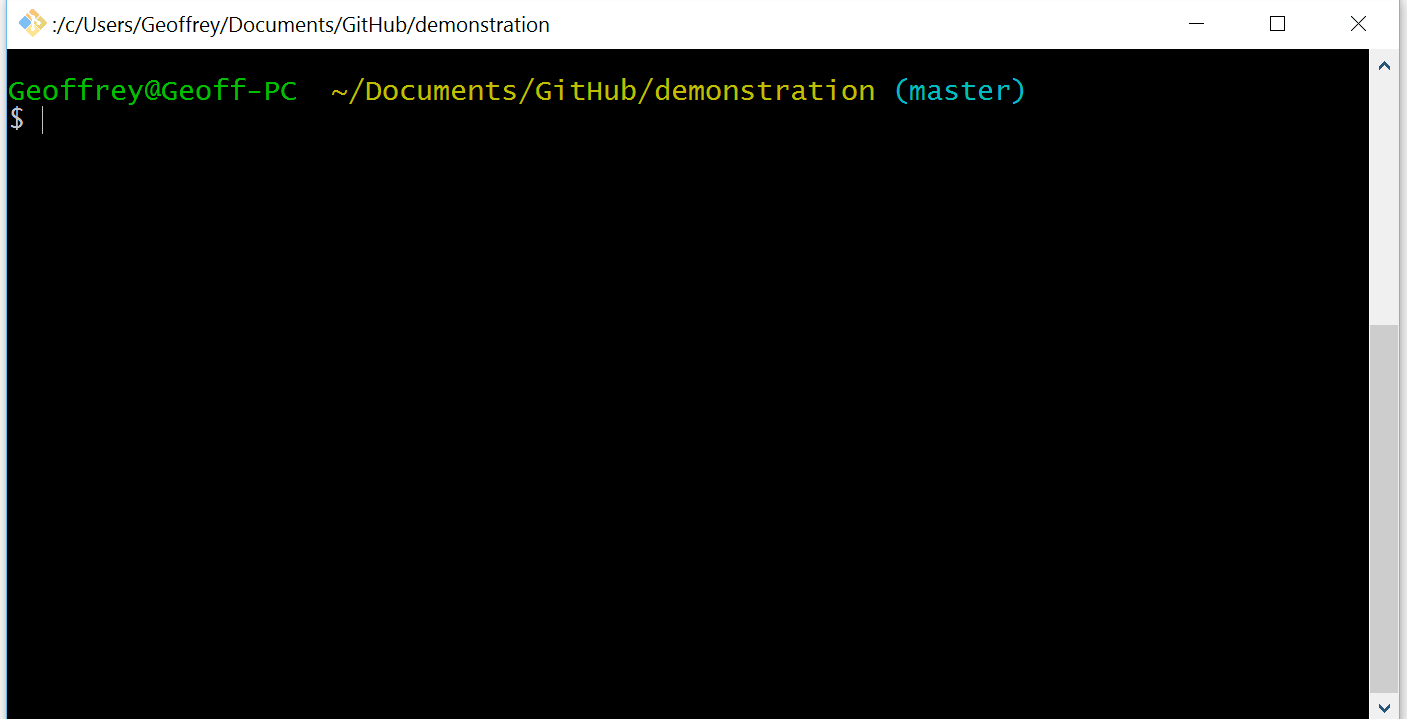
Git "errors"
- With many contributors, your local repo can fall behind.
- Most basic: need to get latest commits with
git pull
. - Even then, what if you and another user change the same file?
- These are the consequences of collaboration, but are fully justified.
- Be able to recognize and resolve these common issues
"non-fast-forward"
- Fancy speak for "your local repo isn't up to date"
- Usually occurs when git pushing some changes
- Fix by running
git pull
, followed bygit push

Merge conflicts
- Happens when two users change the same file
- File now contains changes from both you and others
- Need to choose how to resolve conflicts
- For simple fixes: open text editor, resolve conflicts
- More complex fixes:
git mergetool
(may require additional software installation)

Resolving merge conflicts
- Open file in text editor
- Look for parts of the file that look like this:
<<<<<<< HEAD
// This is the code that you have written
...
=======
// This is the code that they have written
...
>>>>>>> b56a88aab1e13f7c3402fd71a5f890d0368ea3ec
Resolving merge conflicts
- Edit each merge conflict accordingly
- For each conflict, decide which parts to keep
- When done, all lines with
<<<<<
,=====
,>>>>>
should be gone
-
git add conflicted-file
to mark resolution -
git commit
to conclude merge git push

Resolution shortcuts
A quick way to say, "their changes are all correct", or "my changes are all correct"
git checkout --theirs file
- Accept all changes made by other author(s) to file
git checkout --ours file
- Accept all changes made by you to file
GitHub.com features
Git and GitHub, so far
- So far, the focus has been on
Git
- What is Git, conceptually?
- How do you use Git commands?
- What is the basic Git workflow?
- Now: Focus on GitHub, a "wrapper" around Git
- Go to your repository on GitHub.com:
http://github.com/your_username/repo_name
GitHub is a wrapper
Along with hosting your Git repository, GitHub provides the following infrastructure:
Issue tracking
- Each repository has a built-in issue tracker, at
github.com/username/repo/issues
An issue is a task to accomplish on a project. Examples:
- Bug report (most common)
- Feature request
- Enhancement
Creating an issue
- Give the issue a descriptive title
- Describe the issue (more to come)
- Give the issue a label (recommended)
- Give the issue a milestone (optional)
- Assign the issue to someone (recommended)
Writing good bug reports
Can't just say "this button doesn't work, plz fix!!"
A good bug report should contain at least:
- A concise description of the issue
- The environment on which the bug appeared. Examples:
- Operating system and version
- Browser version
- Application version
- Hardware info (CPU, screen resolution, etc.)
- The steps required to reproduce the issue
- The expected bug-free behavior
Bug report examples
Guidelines for issues
- Each issue should be categorized and assigned to a user
- Assignees and issue authors should keep the issue progress up to date
- Referencing issues in commits is a lightweight way to track progress on issues
- A good project is always closing issues
GitHub issue features
More overhead, but can help keep your project tidy!
- Reference an issue in another issue
- Reference a specific commit in an issue
- Mention user(s) in an issue
- Close an issue with a single commit
See Mastering Issues
Forking
Say you want to contribute to Twitter Bootstrap
- Problem: You don't have contributor access
-
Solution: Copy of the Bootstrap repo to your account by forking it
- Make changes to your fork
- Request those changes to be integrated into the main Bootstrap repo (next slide)
Pull requests
- Used to tell others about changes you've made to a repository on GitHub.
- Only contributors with push access can accept pull requests!
- Read more on Using pull requests
Wikis
- Like an internal Wikipedia for your project
- Use it to keep track of information about your project:
- Installation
- Known bugs
- Configuration
- Software architecture diagrams
- Detailed documentation
- Use cases
Stats and graphs
Note about homework code
- GitHub repos are by default public
- Homework solutions are for CSE 154 only!
- In short: don't upload your 154 homeworks to GitHub
- If you must, make a private repo for homework
What you know now
- How to set up a repo on GitHub
- Basic Git workflow
- Basic Git "debugging"
- Collaborating with a partner
- Using GitHub features
What you should do next
- Learn about branching and merging
- Request a free upgrade to a student account
- Unlimited private repos (as of 11 May 2016)
- Good for your entire time here at UW
- Apply here: https://education.github.com/
- Start moving your personal projects to GitHub
- Use Git and/or GitHub for future personal projects
Follow me on GitHub!
Additional resources
Questions?
Git workshop
By Geoffrey Liu
Git workshop
- 1,556