Testes
&
Jest
Gabriel
Prates
@gabsprates

- Frontend Developer
- Translator
- Drummer
O
que é
"teste"?
TESTE
Prova; exame feito para testar, para avaliar as características ou qualidades de algo ou de alguém [...]
Todo mecanismo que busca verificar ou provar a verdade [...]
Teste de software...
Por quê?
Por que não?!
- Pegar o defeito antes do cliente
- Detectar problemas de design da aplicação
- Tornar o trabalho colaborativo mais seguro
- Reduz o tempo gasto em depuração e correção de bugs
- Auxilia testes de regressão
- Documentação pelos testes
- Refatoração constante
BENEFÍCIOS
QUALIDADE
Daiane Azevedo de Fraga
[...] teste de software visa garantir a qualidade, minimizando as incertezas e sistematizando os critérios de aceitação.
[...] teste não é um puro sinônimo de qualidade.
TIPOS
Performance
Unidade
Carga
Interface
Funcional
Caixa-branca
Caixa-preta
Regressão
Positivo-negativo
Operacional
Integração
Aceitação do usuário
Stress
Segurança
Testes de
RESPONSABILIDADE
DO DESENVOLVEDOR
-
UNIDADE
A palavra chave é:
AUTOMAÇÃO
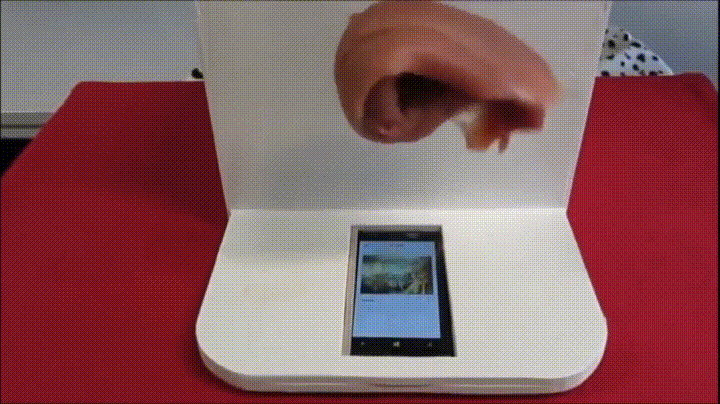
EM JAVASCRIPT


Jest
Zero configuration testing platform
$ mkdir testes && cd testes
# iniciar um projeto
$ npm init
# instalar o Jest
$ npm install --save-dev jest
// sum.js
function sum(a, b) {
return a + b;
}
module.exports = sum;
// sum.test.js
const sum = require('./sum');
test('adds 1 + 2 to equal 3', () => {
expect(sum(1, 2)).toBe(3);
});
// package.json
"scripts": {
- "test": "..."
+ "test": "jest"
},
$ npm test
> testes@1.0.0 test /path/to/project/testes
> jest
PASS ./sum.test.js
✓ adds 1 + 2 to equal 3 (4ms)
Test Suites: 1 passed, 1 total
Tests: 1 passed, 1 total
Snapshots: 0 total
Time: 1.324s
Ran all test suites.
PARA ENTENDER
O Jest é pré-configurado para ler testes de:
- diretório __tests__
-
arquivos:
- *.spec.js
- *.test.js
EXEMPLO
# projeto
playlist/
├── package.json
├── package-lock.json
└── src
├── playlist/
└── request/
// playlist/index.js
const request = require('../request');
class Playlist {
constructor() {
this.playlist = request.getPlaylist();
}
shuffle() {
const playlist = Array.from(this.playlist);
for (let i = playlist.length - 1; i > 0; i--) {
const newPosition = Math.floor(Math.random() * (i + 1));
const currentSong = playlist[i];
playlist[i] = playlist[newPosition];
playlist[newPosition] = currentSong;
}
this.playlist = playlist;
}
}
module.exports = Playlist;
// playlist/index.test.js
const Playlist = require('./index');
// cria um grupo de testes
describe('testing playlist', () => {
it('should to shuffle playlist', () => {
const playlist = new Playlist();
const initialOrder = playlist
.playlist.map(song => song.track);
playlist.shuffle();
const finalOrder = playlist
.playlist.map(song => song.track);
expect(initialOrder).not.toEqual(finalOrder);
});
});
MOCK
falsificado, imitado, simulado
In short, mocking is creating objects that simulate the behavior of real objects.
// Em nosso exemplo:
// playlist/index.js
this.playlist = request.getPlaylist();
# projeto
projeto/
├── package-lock.json
├── package.json
└── src
├── playlist
│ ├── index.js
│ └── index.test.js
└── request
├── __mocks__
└── index.js
// request/__mocks__/index.js
const request = {
getPlaylist: () => Array(8)
.fill(null)
.map((_, i) => ({
track: i,
title: `song ${i}`
}))
};
module.exports = request;
// playlist/index.test.js
const Playlist = require('./index');
// faz mock do módulo request
jest.mock("../request")
describe('testing playlist', () => {
it('should to shuffle playlist', () => {
// ...
});
});
PARA ENTENDER
O Jest é pré-configurado para ler mocks de:
- diretório __mocks__
Deve ser colocado no mesmo nível do módulo
CONFIGURAÇÃO
Zero configuration testing platform
Mas às vezes as configurações padrão não atendem aos casos de uso.
# criar arquivo de configuração
$ ./node_modules/.bin/jest --init
// jest.config.js
module.exports = {
// Automatically clear mock calls and instances between every test
clearMocks: true,
// An array of glob patterns indicating a set of files
// for which coverage information should be collected
collectCoverageFrom: [
"**/*.{js,jsx}",
],
// An array of regexp pattern strings used to skip coverage collection
coveragePathIgnorePatterns: [
"/node_modules/",
"/coverage/"
],
// A list of reporter names that Jest uses when writing coverage reports
coverageReporters: [
"json",
"text",
"html",
],
// The root directory that Jest should scan for tests and modules within
rootDir: "./src",
// The test environment that will be used for testing
testEnvironment: "node",
// Indicates whether each individual test should be reported during the run
verbose: true
};
// package.json
"scripts": {
- "test": "jest"
+ "test": "jest --config=jest.config.js"
},
COVERAGE
Martin Fowler
[...] Well it helps you find which bits of your code aren't being tested.
You are rarely hesitant to change some code for fear it will cause production bugs.
// playlist/index.js
const request = require('../request');
class Playlist {
// ...
shuffle() {
if (!this.playlist.length) {
return;
}
// ...
}
}
module.exports = Playlist;
# executar testes com coverage
$ npm test -- --coverage
> playlist@1.0.0 test /path/to/project/testes/playlist
> jest --config=jest.config.js "--coverage"
PASS src/playlist/index.test.js
testing playlist
✓ should to shuffle playlist (7ms)
-----------|----------|----------|----------|----------|-------------------|
File | % Stmts | % Branch | % Funcs | % Lines | Uncovered Line #s |
-----------|----------|----------|----------|----------|-------------------|
All files | 68.75 | 50 | 50 | 73.33 | |
playlist | 91.67 | 50 | 100 | 91.67 | |
index.js | 91.67 | 50 | 100 | 91.67 | 12 |
request | 0 | 100 | 0 | 0 | |
index.js | 0 | 100 | 0 | 0 | 1,2,8 |
-----------|----------|----------|----------|----------|-------------------|
Test Suites: 1 passed, 1 total
Tests: 1 passed, 1 total
Snapshots: 0 total
Time: 1.411s
Ran all test suites.
EXTRA
Só pra quem veio
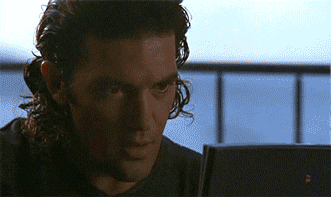
PERGUNTAS?
REFERÊNCIAS
Testes & Jest
By Gabriel Prates
Testes & Jest
- 1,398